325
|
Clear Undo/Redo queue (method 2)
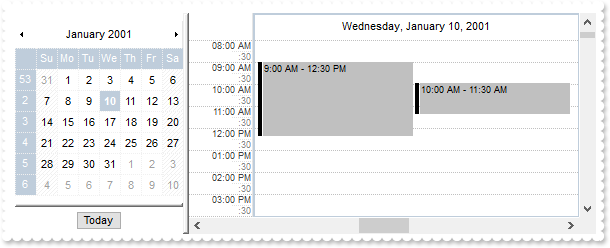
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
c := UndoRedoQueueLength;
UndoRedoQueueLength := 0;
UndoRedoQueueLength := c;
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
324
|
Clear Undo/Redo queue (method 1)
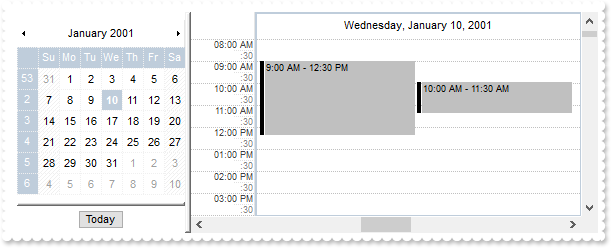
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
AllowUndoRedo := True;
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
323
|
Removes Redo operations
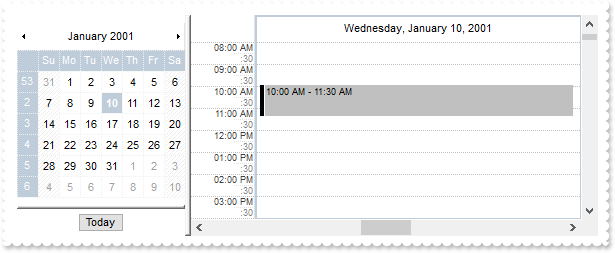
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
Undo();
RedoRemoveAction(TObject(13),Nil);
OutputDebugString( get_RedoListAction(Nil,Nil) );
EndUpdate();
end
|
322
|
Removes Undo operations
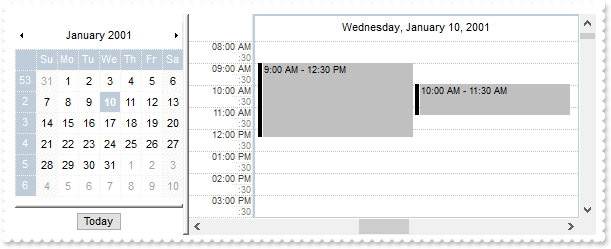
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
UndoRemoveAction(TObject(13),Nil);
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
321
|
Record the UI operations as a block of undo/redo operations
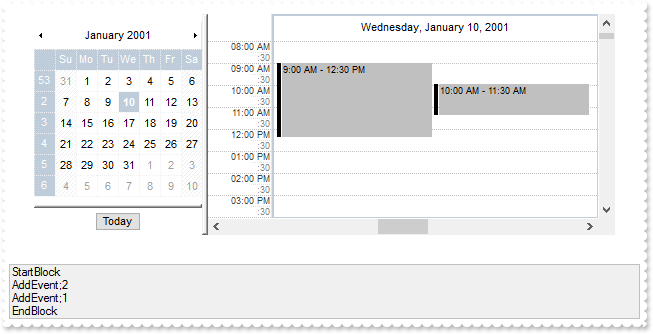
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
StartBlockUndoRedo();
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
EndBlockUndoRedo();
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
320
|
Groups the next to current Undo/Redo Actions in a single block
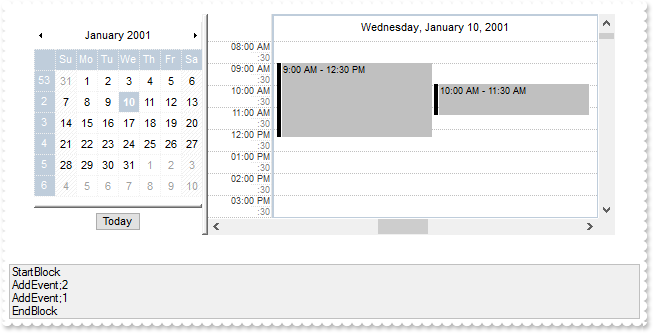
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
GroupUndoRedoActions(2);
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
319
|
Limits the number of entries within the Undo/Redo queue
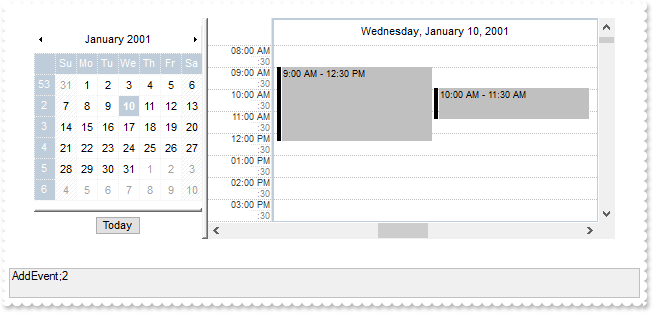
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
UndoRedoQueueLength := 1;
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
318
|
Lists the Redo actions that can be performed on the control
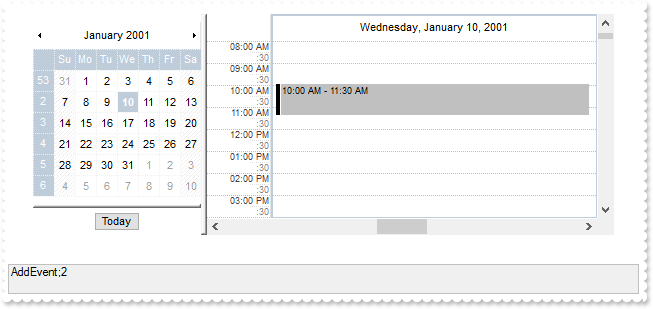
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
Undo();
OutputDebugString( get_RedoListAction(Nil,Nil) );
EndUpdate();
end
|
317
|
Lists the Undo actions that can be performed on the control
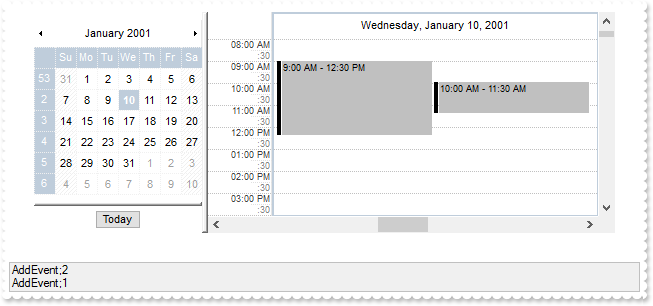
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
OutputDebugString( get_UndoListAction(Nil,Nil) );
EndUpdate();
end
|
316
|
Checks whether the Undo operation is possible
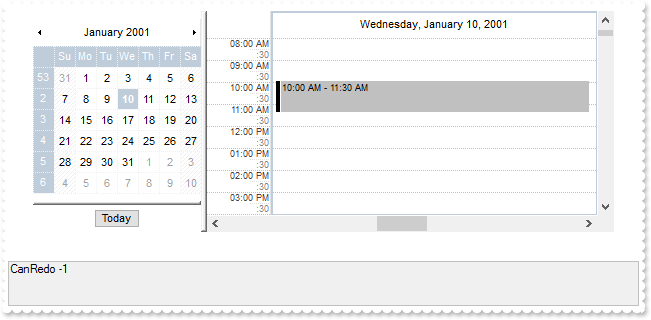
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
Undo();
OutputDebugString( 'CanRedo' );
OutputDebugString( CanRedo );
EndUpdate();
end
|
315
|
Call Redo by code
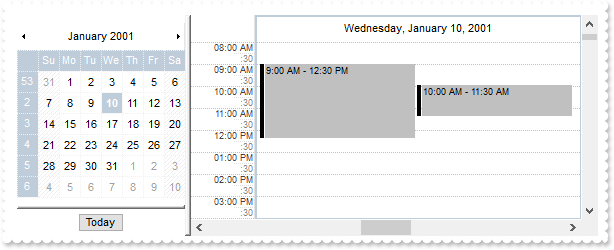
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
Undo();
Redo();
EndUpdate();
end
|
314
|
Checks whether the Undo operation is possible
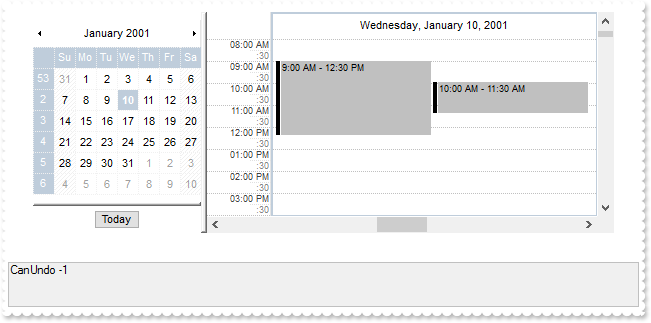
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
OutputDebugString( 'CanUndo' );
OutputDebugString( CanUndo );
EndUpdate();
end
|
313
|
Call Undo by code
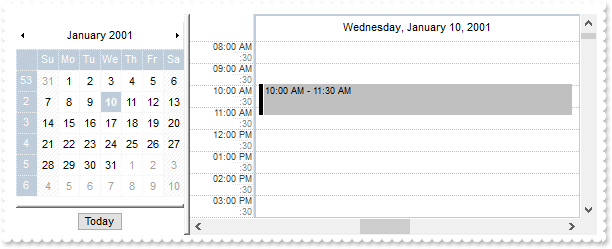
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
Undo();
EndUpdate();
end
|
312
|
Save the calendar-event's properties for Undo/Redo operations, by code
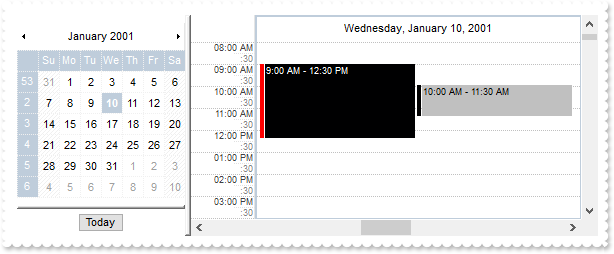
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
Events.Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
StartBlockUndoRedo();
with Events.Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM') do
begin
h := StartUpdateEvent;
BodyBackColor := $10000;
BodyForeColor := $ffffff;
StatusColor := $ff;
EndUpdateEvent(h);
end;
EndBlockUndoRedo();
EndUpdate();
end
|
311
|
No color is restored for the calendar-event when Undo/Redo operation is performed
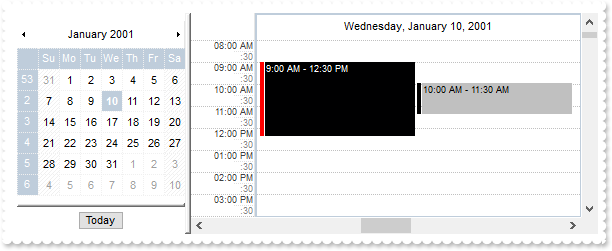
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
Events.Add('1/10/2001 10:00:00 AM','1/10/2001 11:30:00 AM');
StartBlockUndoRedo();
with Events.Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM') do
begin
h := StartUpdateEvent;
BodyBackColor := $10000;
BodyForeColor := $ffffff;
StatusColor := $ff;
EndUpdateEvent(h);
end;
EndBlockUndoRedo();
EndUpdate();
end
|
310
|
How can I ensure that a specified calendar-event fits the control's visible area
with AxSchedule1 do
begin
with Events do
begin
with Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').BodyPattern do
begin
Type := EXSCHEDULELib.PatternEnum.exPatternBDiagonal;
Color := $e0e0e0;
end;
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').EnsureVisible();
end;
end
|
309
|
LayoutEndChanging(exUndo), LayoutEndChanging(exRedo) or LayoutEndChanging(exUndoRedoUpdate) notifiy your application once a Undo/Redo operation is executed (CTRL+Z, CTRL+Y) or updated
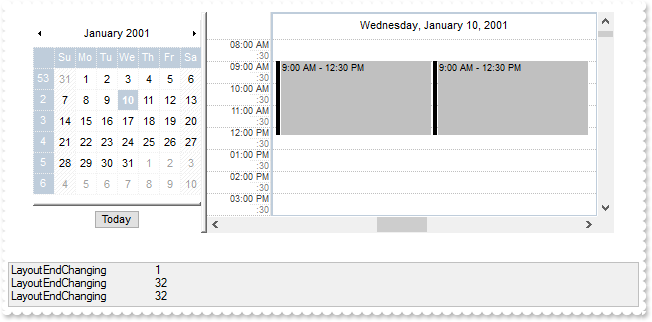
// LayoutEndChanging event - Notifies your application once the control's layout has been changed.
procedure TWinForm1.AxSchedule1_LayoutEndChanging(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_LayoutEndChangingEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( 'LayoutEndChanging' );
OutputDebugString( e.operation );
end
end;
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
EndUpdate();
end
|
308
|
Turn on the Undo/Redo feature
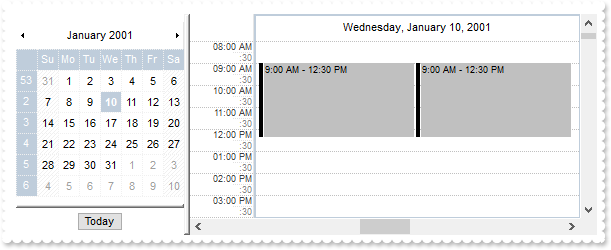
with AxSchedule1 do
begin
BeginUpdate();
AllowUndoRedo := True;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
end;
EndUpdate();
end
|
307
|
How can I make the header (date/group) always visible, so it stays on the top while the user scrolls the chart
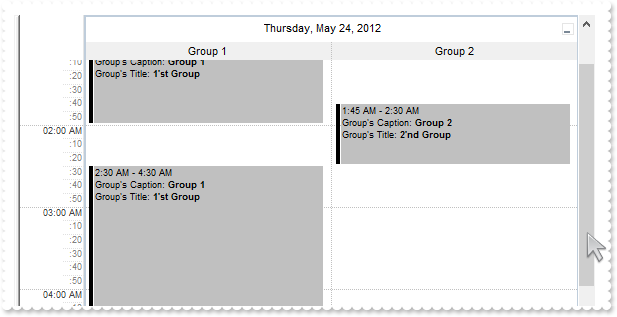
// LayoutEndChanging event - Notifies your application once the control's layout has been changed.
procedure TWinForm1.AxSchedule1_LayoutEndChanging(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_LayoutEndChangingEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( e.operation );
DayViewHeight := 2016;
end
end;
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
SelectDate['5/20/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
end;
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exVertical;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRowLockHeader;
DayViewHeight := 2016;
TimeScales.Item[TObject(0)].MinorTimeRuler := '00:10';
DayStartTime := '00:00';
DayEndTime := '24:00';
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
Calendar.Selection := '5/24/2012';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
ApplyGroupingColors := False;
with Groups do
begin
with Add(1,'Group 1') do
begin
Visible := True;
EventBackColor := $808080;
Title := '1''st Group';
end;
with Add(2,'Group 2') do
begin
Visible := True;
EventBackColor := $ff;
Title := '2''nd Group';
end;
end;
DefaultEventLongLabel := '<%=%256%><br>Group''s Caption: <b><%=%262%></b><br>Group''s Title: <b><%=%263%></b>';
DefaultEventShortLabel := DefaultEventLongLabel;
with Events do
begin
Add('5/24/2012 1:00:00 AM','5/24/2012 2:00:00 AM').GroupID := 1;
Add('5/24/2012 1:45:00 AM','5/24/2012 2:30:00 AM').GroupID := 2;
Add('5/24/2012 2:30:00 AM','5/24/2012 4:30:00 AM').GroupID := 1;
end;
EndUpdate();
end
|
306
|
It appears that Width property of the Group does not what. What am I doing wrong
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '1/10/2001';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
with Groups do
begin
with Add(1,'Group 1') do
begin
Title := 'First';
Visible := True;
end;
with Add(2,'Group 2') do
begin
Title := 'Second';
Visible := True;
end;
with Add(3,'Group 3') do
begin
Title := 'Third';
Visible := True;
end;
end;
DayViewWidth := 144;
Groups.Item[TObject(1)].Width := 48;
Groups.Item[TObject(2)].Width := 48;
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').GroupID := 1;
Add('1/10/2001 10:00:00 AM','1/10/2001 1:00:00 PM').GroupID := 2;
end;
EndUpdate();
end
|
305
|
ImageSize property on 32 (specifies the size of control' icons/images/check-boxes/radio-buttons)
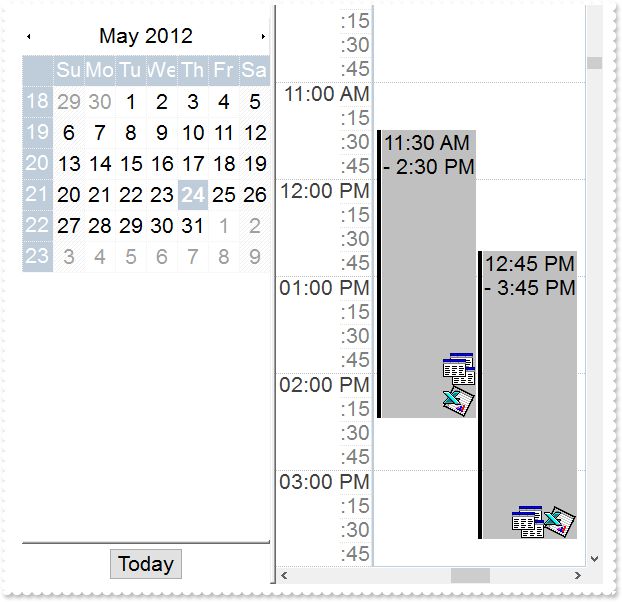
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
ImageSize := 32;
Font.Size := 16;
EventsFont.Size := 16;
TimeScaleFont.Size := 16;
TimeScales.Item[TObject(0)].Width := 128;
Images('gBJJgBAIDAAEg4AEEKAD/hz/EMNh8TIRNGwAjEZAEXjAojKAjMLjABhkaABAk0plUrlktl0vmExmUzmk1m03nE5nU7nk9miAoE+oVDolFo1HpFJpU5h8Sf9OqFNqUOqN' +
'UqdPq9VrFWrlbr1QpdhAFAkFis1ntFptVrtkrpszrNvmVxqk3uVtm1kmF3sdBvF/wGBmV+j9BYGHwWJulfxdax2NyFdx2JlV6l9Nw7AAGZymdz2Cy2GxErvWcz9ivlwy' +
'V21cuxugwktzGIzmvwtl0+53U5y0a0Wazmmyu/3dCyOMyXHx/J5nIr9q3uyqnBxFN3G46ma4vb7mD2Ng4nZze00fDkHC7t7us2rOX5tguetpHRlmz4HVqnXk1PjHO+CM' +
'Po9MBMC+j2vC8j7wS8cFNI4kBo05UIvfCT/NsnsApU+0Fqg/T+oy/kPxC0sEQfErKQK96+w28UWRI8UGvO8sTLS9r2PWmsMJTDTask3CsIbIEQRA3shOXEEAO/GclJ9F' +
'EKrrA8FRbKMXRIlb0JxCkjS1LMswhCcvuel0cv26cSMa8Ufx+2sQwhEUoSXOCjSbLcnxjKc7sdKUVyq28NtVI71P9P7JxtQEapjQ6fzfM8zPfNE2PhIsLL63E40slk5y' +
'7N89LcyU9SvMb3SdUc6VJLj5VLVLfO/PS9KzNFHUa/0XyBD0dxlS9cxhMlTRSoNXypPErWDPyfNS+MwprRNO0FD8wVVZ1AI08URwVRjtJ1WCn21QkkUrXVLVPQS/XIkF' +
'gTxT9iONZ9xVTdq+L1eKg3kkF6Upe68XtfV51/MtrVjBlwYFL1ev8y1/P6/lyzzYl02wntj0RVFmS1Qa+M5as93QxEUW9e993rfmQ2+vy65M/mL1lhl/2bj2ByVduMtN' +
'hCJT9hdz41nN14Ld12Z9UjfI/oUAaGseiw6+uFLLhcVabJOS5RqOE0BHlZ5VnEr5fOMs3st+aa/bbRzrJGV51Y0b0DbqaWXZD90hIsPbjWu52+6Wyadpe66hhO+P/Xio' +
'W5rD8ZbrUZuVg6n1dsE/cXmewu1m9PVwnd35/nueXho/NaJzmjc61W76esuT77eG8pTquy9TwWH8LEzG8RDfFalx3Gcfvna9rvG/cptGLd9tuI6TZOP5Fiqi99vea+X4' +
'VRcBq/JZZtVQ9cwSs5lsXE372+a9z7PbfB3VVqHyvMctLto8uob6eV0m/cD6MN2v+T33t6sBut42vdv2bJ8a997x2maFJfK+qArbGJPEKE+1qTflMsIdW/GCJX17KcT6' +
'/czr/X+u1g29B7j/4BQfWkkx4zIHisjhPCmE0K4SwtXM+d4BvHRwNZOoBph9IJvPek9d40FoMJxf691jj2ywQQcHEWET4XJwkTszlVqm2GokewxtBT1DpQjRxDN0rUVD' +
'NKdC3lb6tzNOwh6upMSSYfv4YBCl/bsn9PxiFCEo7SI6Obc9HeOrnY8x4jtHtdpN4GRbaorhsbu18Pph5CiHymI0RpSXGJ/z2oUOxYxG858AyiI+bfJtuTcG5yelBJyT' +
'8okhqFd4a5yxL0rvulYtKCsZiWxWkc1s1cRoxxwhA31DLE0mR9l9HqX8fJgTDmFMVH0MIsRzVYnwnMi1dyzmhLt2kS2pxIiU62Wj5ptQGlSYFakLonTUJNLKaM5Wzlff' +
'EkuFkk5wTrhVO2eE7G6lJhxFFYUZ55zmn0WuBCD4pzhirFCKkbomsOoIYmZx5p90LoYWGPdD5g0QmJRKYxbZ6zYoVQ2jVGylSak7KSkFH6RSjpHKFuU+YMyNo5SulkC6' +
'I0vonTCitMXPoEpVS2H5FQfEqp2R1opIgAEkJISYARTCukOhmPNI5Ex/wzGHUsicMwA1LHgQ90Y/KpoQHAD+pB/R4NzIaMAB9Xaw1gqaAOsh/A/ptIkWUfhGK1kZH8Rg' +
'H5GqvgArqRmt4AAPrTroRofBGADkqr6Rmu4D7CEaHARiwpJrEEZsXXwlVjyMWRsaRqwdkLGNBABZmytmyMnaINZqyVpLR2ftKAAAdd6h2osbaskdiq4EZtgSmyNcbVWR' +
'JNXe3AA7REar3b0stlAAXBtoRmvJGLjEYAHUWsFcwCD/rnaop9aEICMAPdK5hT6xpeuzdOtAgKuJeGfdq6ggEbkTvAP+p9UCHXrvKkcgIA==');
with Events do
begin
Add('5/24/2012 11:30:00 AM','5/24/2012 2:30:00 PM').Pictures := '1/2';
Add('5/24/2012 12:45:00 PM','5/24/2012 3:45:00 PM').Pictures := '1,2';
end;
EndUpdate();
end
|
304
|
ImageSize property on 16 (default) (specifies the size of control' icons)
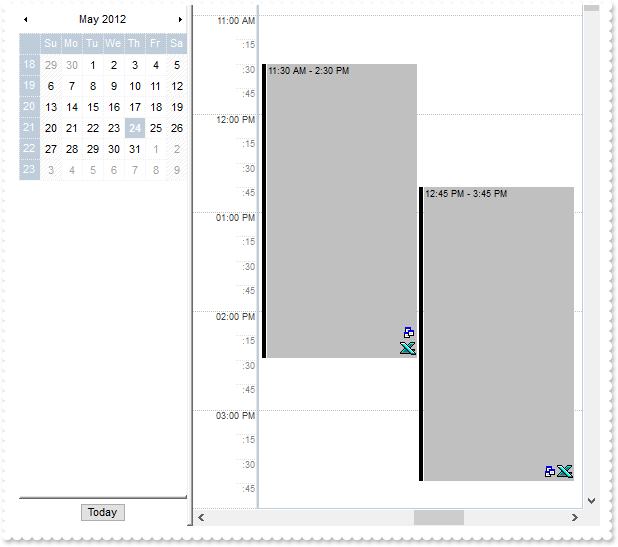
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
ImageSize := 16;
Images('gBJJgBAIDAAEg4ACEKAD/hz/EMNh8TIRNGwAjEZAEXjAojJAjMLjABAAgjUYkUnlUrlktl0vmExmUzmk1m03nE5nU7nkrQCAntBoVDolFo1HoM/ADAplLptImdMYFOqd' +
'SqlXq1QrVbrlGpVWsFNrNdnNjsk7pQAtNroFnt0sh8Yr9iulTuNxs1Eu8OiT/vsnsNVutXlk/oGGtVKxGLxWNtsZtN8iUYuNvy0Zvd+xNYwdwvl4p870GCqc8vOeuVtt' +
'mp1knyOayWVy+WzN/ze1wOElenm+12WUz/Bv2/3UyyWrzeutux2GSyGP2dQ33C1ur3GD3M4zUNzHdlWjq/E3nGzVpjWv4HA7fRy/Tv2IrN8rPW6nZ3ve7mUlfu20Z8ac' +
'vQyb+vY9jasYoDwMm+LytVBDqKG3z8O3Cb8P+mkAuY9cCQ2uL4KaxDKvkp8RNLEjqugnrwQo/UWPzFyeQw5sNLZFENrI4kOqU66pw8uzmOKvTqNqjULJvGL1JO48GtTG' +
'sbLdEL3scxLlyiw8dQeoUVxdLTtyKmUjwGlslRPJsnK1HbAKbKCrsQo8uQk/CeP44iaR/ATnTNPLvyxPU+z9P9AUDQVBowiofJXQ6Oo+kKMpIkjztE4TKn4P6JowfgPn' +
'wD5/nAjB8AOeAPo0eAA1IAFH07UhAIMpYAVIYFHqBUhwVjV1S1EtQAHxW65V0AZwAeuQAnwB5gAPYViEDVhwAHTQBkCjB4gOhwDmCyhH0sACAg==');
with Events do
begin
Add('5/24/2012 11:30:00 AM','5/24/2012 2:30:00 PM').Pictures := '1/2';
Add('5/24/2012 12:45:00 PM','5/24/2012 3:45:00 PM').Pictures := '1,2';
end;
EndUpdate();
end
|
303
|
Is it possible to show a different background color for alternate days
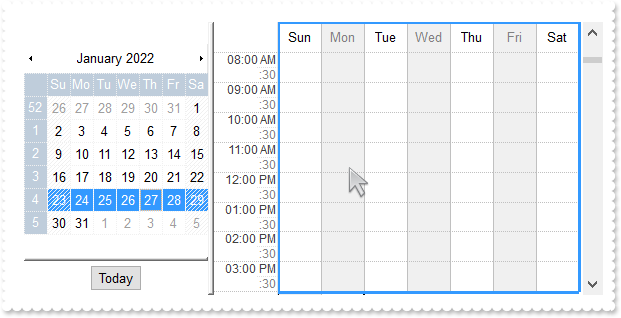
with AxSchedule1 do
begin
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleDayHeaderBackColor,$0);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleDayHeaderForeColor,$0);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleDayBackColorAlternate,$f0f0f0);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleDayForeColorAlternate,$808080);
Calendar.Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
end
|
302
|
How can I select all events
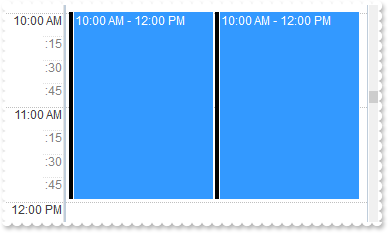
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
end;
SelectAll();
EndUpdate();
end
|
301
|
How can I unselect all events
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
end;
SelectAll();
Selection := '';
EndUpdate();
end
|
300
|
How do I immediately select a newly added event
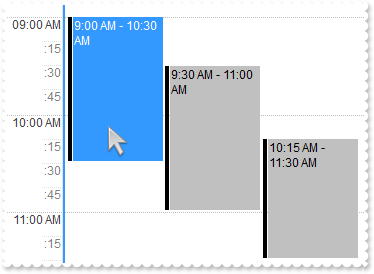
// AddEvent event - Notifies your application once the a new event is added.
procedure TWinForm1.AxSchedule1_AddEvent(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_AddEventEvent);
begin
// Ev.Selected = True
end;
|
299
|
I would like to know if this allows me to setup a number of room(column). Let's say, i need to go up to 10 rooms is it possible. Also, the possibility to have a complete week of that 10 rooms.
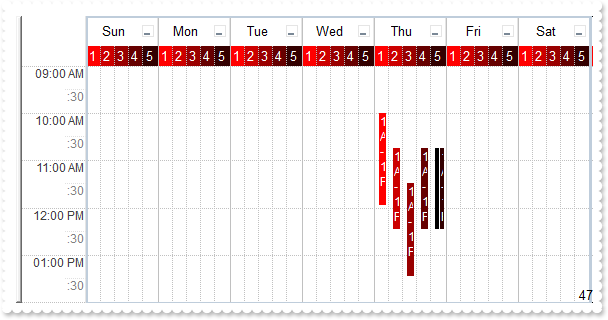
with AxSchedule1 do
begin
BeginUpdate();
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
DisplayGroupingButton := True;
ShowGroupingEvents := True;
BodyEventForeColor := Color.FromArgb(255,255,255);
DayStartTime := '09:00';
DayEndTime := '14:00';
with Calendar do
begin
SelectDate['5/24/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
end;
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exNoScroll;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRow;
with Groups do
begin
with Add(1,'1') do
begin
Visible := True;
EventBackColor := $ff;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(2,'2') do
begin
Visible := True;
EventBackColor := $cc;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(3,'3') do
begin
Visible := True;
EventBackColor := $99;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(4,'4') do
begin
Visible := True;
EventBackColor := $66;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(5,'5') do
begin
Visible := True;
EventBackColor := $33;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
end;
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM').GroupID := 1;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 2;
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM').GroupID := 3;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 4;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 5;
end;
EndUpdate();
end
|
298
|
I would like to know if this allows me to setup a number of room(column). Let's say, i need to go up to 10 rooms is it possible
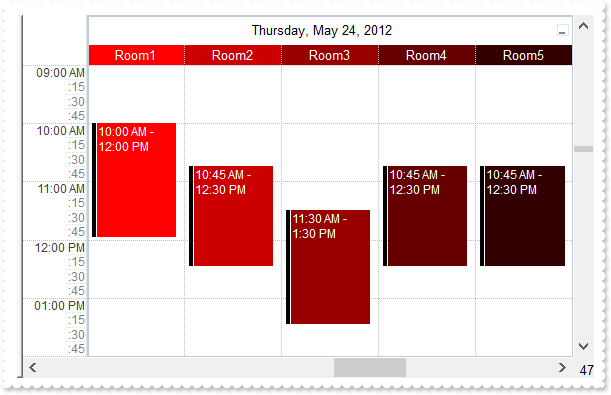
with AxSchedule1 do
begin
BeginUpdate();
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
Calendar.Selection := '5/24/2012';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
BodyEventForeColor := Color.FromArgb(255,255,255);
DayStartTime := '09:00';
DayEndTime := '14:00';
with Groups do
begin
with Add(1,'Room1') do
begin
Visible := True;
EventBackColor := $ff;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(2,'Room2') do
begin
Visible := True;
EventBackColor := $cc;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(3,'Room3') do
begin
Visible := True;
EventBackColor := $99;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(4,'Room4') do
begin
Visible := True;
EventBackColor := $66;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
with Add(5,'Room5') do
begin
Visible := True;
EventBackColor := $33;
HeaderBackColor := EventBackColor;
HeaderForeColor := $ffffff;
end;
end;
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM').GroupID := 1;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 2;
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM').GroupID := 3;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 4;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 5;
end;
EndUpdate();
end
|
297
|
Please could you let me know if it is possible to change the increment when the user scrolls the mouse wheel as its to slow by default
with AxSchedule1 do
begin
VerticalScrollWheel := 3;
end
|
296
|
I am using the DefaultEventLongLabel property to specify the event's label. Is it possible to change the way the event's label is displayed when it is an all day event (sample 2)
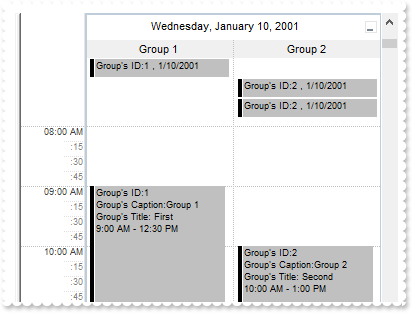
with AxSchedule1 do
begin
BeginUpdate();
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
AllowAllDayEventScroll := Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventWheelScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventMax4);
DefaultEventLongLabel := '<%=%><%= ( %3 = 0 ? (`Group''s ID:` + %4 + `<br>Group''s Caption:` + %262 + `<br>Group''s Title: ` + %263 + `<br>` + %256 ) : ( (`' +
'Group''s ID:` + %4 + ` , ` + %256 ) replace `<br>` with `,` ) ) %>';
DefaultEventShortLabel := '<%=%><%= ( %3 = 0 ? (`Group''s ID:` + %4 + `<br>Group''s Caption:` + %262 + `<br>Group''s Title: ` + %263 + `<br>` + %256 ) : ( (' +
'`Group''s ID:` + %4 + ` , ` + %256 ) ) replace `<br>` with `\r\n` ) %>';
Calendar.Selection := '1/10/2001';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
HeaderGroupHeight := 1;
ShowAllDayHeader := True;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
with Groups do
begin
with Add(1,'Group 1') do
begin
Title := 'First';
Visible := True;
end;
with Add(2,'Group 2') do
begin
Title := 'Second';
Visible := True;
end;
end;
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').GroupID := 1;
Add('1/10/2001 10:00:00 AM','1/10/2001 1:00:00 PM').GroupID := 2;
with Add('1/10/2001','1/10/2001') do
begin
GroupID := 1;
AllDayEvent := True;
end;
with Add('1/10/2001','1/10/2001') do
begin
GroupID := 2;
AllDayEvent := True;
end;
with Add('1/10/2001','1/10/2001') do
begin
GroupID := 2;
AllDayEvent := True;
end;
end;
EndUpdate();
end
|
295
|
I am using the DefaultEventLongLabel property to specify the event's label. Is it possible to change the way the event's label is displayed when it is an all day event (sample 1)
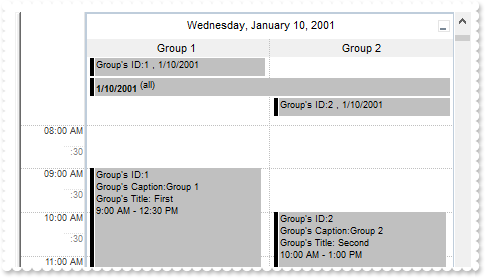
with AxSchedule1 do
begin
BeginUpdate();
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
DefaultEventLongLabel := '<%=%><%= %4 < 0 ? `<b>` + %256 + `</b> <off -4>(all)` : ( (`Group''s ID:` + %4 + `<br>Group''s Caption:` + %262 + `<br>Group''s T' +
'itle: ` + %263 + `<br>` + %256 ) replace ( %3 ? `<br>` : ``) with `,` ) %>';
DefaultEventShortLabel := '<%=%><%= %4 < 0 ? %256 : ( %3 = 0 ? (`Group''s ID:` + %4 + `<br>Group''s Caption:` + %262 + `<br>Group''s Title: ` + %263 + `<br>' +
'` + %256 ) : ( (`Group''s ID:` + %4 + ` , ` + %256 ) ) replace `<br>` with `\r\n` ) %>';
Calendar.Selection := '1/10/2001';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
HeaderGroupHeight := 1;
ShowAllDayHeader := True;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
with Groups do
begin
with Add(1,'Group 1') do
begin
Title := 'First';
Visible := True;
end;
with Add(2,'Group 2') do
begin
Title := 'Second';
Visible := True;
end;
end;
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').GroupID := 1;
Add('1/10/2001 10:00:00 AM','1/10/2001 1:00:00 PM').GroupID := 2;
with Add('1/10/2001','1/10/2001') do
begin
GroupID := 1;
AllDayEvent := True;
end;
with Add('1/10/2001','1/10/2001') do
begin
GroupID := -1;
AllDayEvent := True;
end;
with Add('1/10/2001','1/10/2001') do
begin
GroupID := 2;
AllDayEvent := True;
end;
end;
EndUpdate();
end
|
294
|
The Event.Caption does not support HTML, and so if using in DefaultEventLongLabel/DefaultEventShortLabel no HTML is applied, instead HTML tags are displayed as plain text. What can be done
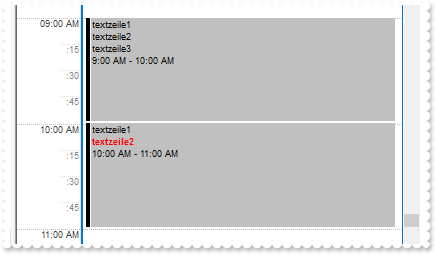
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
DefaultEventLongLabel := '<%=%><%=%5%><br><%=%256%>';
with Events do
begin
Add('5/24/2012 9:00:00 AM','5/24/2012 10:00:00 AM').Caption := 'textzeile1<br>textzeile2<br>textzeile3';
Add('5/24/2012 10:00:00 AM','5/24/2012 11:00:00 AM').Caption := 'textzeile1<br><fgcolor-FF0000><b>textzeile2</b></fgcolor>';
end;
EndUpdate();
end
|
293
|
Please could you let me know how I can remove/hide the time scale/marks from the scheduler. I am creating a month view that only requires a box for the day and no time markers required
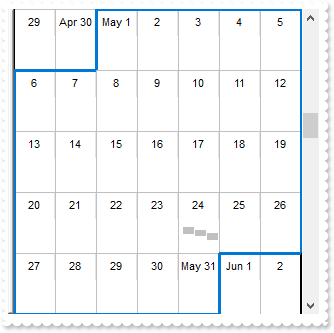
with AxSchedule1 do
begin
BeginUpdate();
TimeScales.Item[TObject(0)].Visible := False;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
with Calendar do
begin
Selection := '5/24/2012';
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectMonth);
end;
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').ShowStatus := False;
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM');
end;
EndUpdate();
end
|
292
|
Is it possible to lock down the view to allow resizing of the days column but not to allow the scrolling outside of the dictated time zone
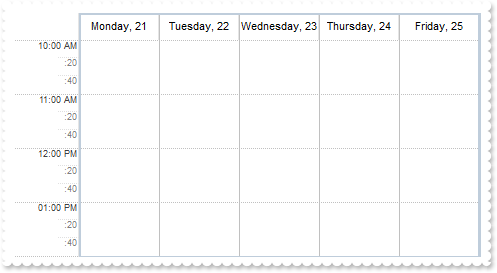
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '5/21/2012';
Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
end;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exVertical;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRow;
AllowMoveSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowExchangePanels := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMoveTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMultiDaysEvent := False;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColor,get_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColorUnFocus));
TimeScales.Item[TObject(0)].MinorTimeRuler := '00:10';
DayStartTime := '10:00';
DayEndTime := '14:00';
EndUpdate();
end
|
291
|
I would also like to control the column view to only show 5 days at a time with a side scroll, how would I achieve this please
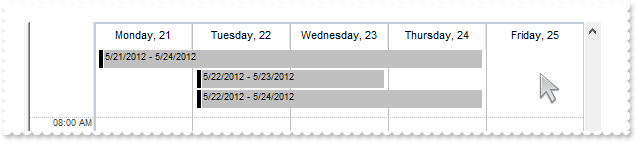
with AxSchedule1 do
begin
BeginUpdate();
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exVertical;
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
290
|
Can I force the schedule grid to only show a single day and then to step through each day using either a custom button click or using the built in schedule calendar
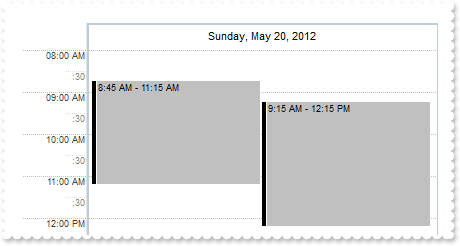
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
SelectDate['5/20/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectFocusDay);
end;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exNoScroll;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRow;
AllowMoveSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowExchangePanels := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMoveTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMultiDaysEvent := False;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColor,get_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColorUnFocus));
EndUpdate();
end
|
289
|
Can I colour the background of the schedulers grid from a time point to another EG 9:00 to 12:00. This is to show users that they can only book appointments in this time zone
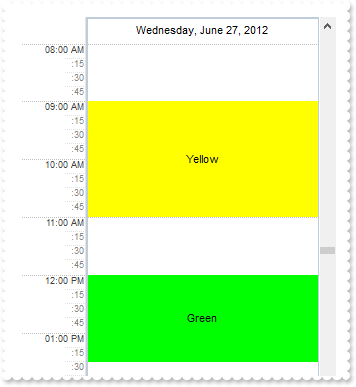
with AxSchedule1 do
begin
BeginUpdate();
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
Calendar.Selection := '6/27/2012';
with MarkZones.Add('zoneA','6/27/2012 9:00:00 AM','6/27/2012 11:00:00 AM') do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternEmpty;
LongLabel := 'Yellow';
BackColor := $ffff;
end;
with MarkZones.Add('zoneB','6/27/2012 12:00:00 PM','6/27/2012 1:30:00 PM') do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternEmpty;
LongLabel := 'Green';
BackColor := $ff00;
end;
EndUpdate();
end
|
288
|
My programming language has the following format for date 2012-05-24-13.04.06.810000 every other format returns a compiler error. Is there a possibility to use a string for the date
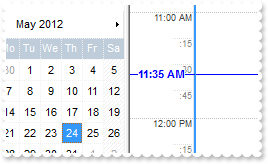
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := AxSchedule1.ExecuteTemplate('#5/24/2012#');
with MarkTimes do
begin
with Add('timer',AxSchedule1.ExecuteTemplate('#5/24/2012 11:35#')) do
begin
Movable := True;
LineColor := $ff0000;
StatusEventBackColor := $ff0000;
TimeScaleLineColor := $ff0000;
TimeScaleLabel := '<fgcolor=0000FF><b><%hh%>:<%nn%> <%AM/PM%>';
end;
end;
EndUpdate();
end
|
287
|
How do I display a picture with transparency
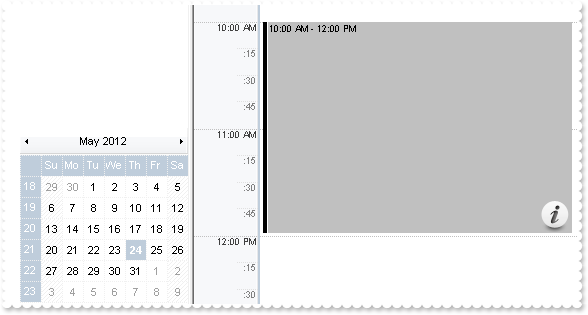
// PictureClick event - Occurs when the user clicks a picture within an event ( Event.Pictures/ExtraPictures ).
procedure TWinForm1.AxSchedule1_PictureClick(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_PictureClickEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( e.key );
end
end;
with AxSchedule1 do
begin
BeginUpdate();
ShowSelectEvent := False;
Calendar.Selection := '5/24/2012';
with Pictures do
begin
Add('pic1','gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa' +
'2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdP' +
'yyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QW' +
'k4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW' +
'4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByE' +
'QGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2Cydh' +
'Gg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIie' +
'RIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpP' +
'hyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaG' +
'wnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBM' +
'GQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxT' +
'AmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+Asf' +
'wMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB' +
'4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0Hgfx' +
'niuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQ' +
'XQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCy' +
'EkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJ' +
'h4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6H' +
'sXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2D' +
'eE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo' +
'8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0' +
'CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5Ag' +
'jtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS' +
'43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtipG' +
'0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtA' +
'uBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKg' +
'FBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZB' +
'IAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnB' +
'dAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB' +
'7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhvB' +
'PlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfA' +
'bAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpB' +
'ig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA' +
'2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/h' +
'EhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9h' +
'IgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hzB' +
'7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGg' +
'KBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA' +
'/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAAChpA' +
'kgiAggqAsAEhigrAkgmASAygKB/BVhVB7hTBNg3BNA2hQBOg4BAAAgiBhB1BIA8BMgCAxBxAGAbhBAJgJgHBnAkhyhQBjBhgmAoBBgwgaAPBghJgLgShogYAxAIAZAZA' +
'ghLBhhQAEgPAEAwgBAwhIgkBBBwhwAYBhhsBoBhhtAbAqArgrh8hehLBVAqA7grgXhPA+Bkglh+h6i4hfh7hehygvgMB9hPhEh9gXh/AKgihUhBh/A1hwA1gwAdh8ASg' +
'4AEB+ADh8AAB+AFh8AThHBxg7B4BmhFBZABAABjgbgwh2gBg4A8grArB/3UgDgQBfgzhpBzhpgUhOYogKBlBZB+ARgAA+NxgDhZgWBcgKg9BfgjBRhogngj4zBCg6AfA' +
'pAzBwB+OiBtA2AbA/hHqYg6ADBZBMBNgfgfAfBfByh4g8BUAiAkgyBMBAg4AcA+BsgyAZBGgRgFA/gbAIh/h6h2AMh/huheg9hbB6h6g6hJhA1zAc5RhhgN5TBLASB3g' +
'IXagQAMAGBdAghJhUAvhahohBAiBshzBzBTB4A9AzhTgDh9AOAZBjBfAPAOAeA2ZlBKAJB61xhBE+gmhKATiWhnBvhlhrAcgMghhGg2hBAQA4B85xBWBQBQXiBIAchoh' +
'khih/gN36hyBqhiDRgABQgoB0AJAKBvAbAkAxhlBzAVgVAVBJA2gbANg5gdAOAWBrh2gzAW6HBIiagwh9hRhiBTBGAVgAgNB3g5giB/grARBlA6B/gsBhhjaTB2A0AaB' +
'Qhahah6hWBVBVBlAUB+hNAEh2BVAtkNhSgFBxAAOjBOg2gxBhBTgnAzB9ADABAAh/BohRgjgzBPhrhVgaAaAagihWArSxA0gGhzB3gBhgAdhVPTgOhgA8gShjAsgcgjh' +
'UhcBdg+zcBshsgsgtgvAvAPA5hdguBWBUs1LxBbh7gsBahLhzBtjjBpg9AABDgjA6hVBtB0hygt7IAhAz7Jg3AkgkhEtvh5hYhRBhAhBzB6g9AsgwA3hng0AFgNB6hHB' +
'7hRBrgxAcBaAGVWhYgehXgjhLgcBvBththxhd7egugB7gBThoAzh57igHA5A3BYgsW8gshMgiAgBZgQh0AKghA3hLsfAGAwAWB0ARgagNBXhWBWAEB+7yBTga7zhpB6B' +
'qBKBmh0g6AZAxBdhuhnAvWAB6huA0BGhIhGBzuRgcgThpAZAABigYAUhThRhbcEgEBmgpgHgWcHgsAUh+g6B8AsBoA+B6gGgGgxBTB6hVA2h2ACZYAxhSAAhIASB0gng' +
'QAphyg0hf3pAJgrAMBwg4BZAYgVAihihKgVhzgMBhg/h9AAAqBRh0BbgTsbgHhwAbhFATANhNBMhMhYgwhlhihRAThT6WBahYh6AzhZgLBAA7h6ALhJgLBZBnhzhthAh' +
'AhGB7h7htOfA0hzh4gxhJgBg6hThzBlg4hchyBFc/AsA0hIBihGEnB8BQgvAkgBggAhAmBvBrgngNBGhTgTAThWg1B5gsgAhABRBTgZB+AYAZBxBMBMhPBbJ9BohlhIB' +
'/Awh+g/gOAAAuBhhBhUB2gXBSgagKgXgXAXAtA5AXAdBvhCBJBKgrgqBEAHhjBjhO7dBthhBGh7B7BaAdgAzWgxBthXBTACAoAuAuAOBBBxgXgpF2AFhEhtgHgDACg1A' +
'fARAiVxAHA4Af9zhc0CB3BOAoATApBWhCp0gJA+hTA7gnAxhWgEhqgEgQB+BzBweFANBGBahVgZB6oehXBwBrAphiAkBCAhhbB0AEBLA3guBfg/AtArgvgXhVGUhfBPh' +
'wBlHFBYhcBlh0AxAYgb6phyA0A3g3hPgAAEA9ACgpgqBNAPAPAWBuB1B1BVBUgNBbgvBUhvgPhqgdheBoBxgUAFgiAsAPgN8VhvhxBWg4ANhRBSA+hNB8AwASAjh+B8B' +
'8AcAMhMg3A0DThhgxhRgGBahxhggBApBEWFBmhS+/BBBhhwgIB4BgAYAJhJgOA2BDARgAhmF6A4gGB8Bo/Jgrg/Bfh/hXBnA8gOAEAWgZAUAIH8ApAshmA8Bjgcg5/Ug' +
'ehh/WB3gxhNhugiAaA7AcBFgcgRADhSBWAThPBzgyBPALgSAABHABBRhCA72hBpBpApA/4BBrgBhKBFgnBfAZgYA2grgMhWBAgkAChgBBBWhzBiBkh0BghxeEZdALg9i' +
'NBvbw7whhhvBbgwgIhygrBfgrBTA6AhgvhjAYg+haBAF4vH4cze70OQyenwMnkQoiQrzCFQA9SUXGy9CMMAuywARTOoQYIFaLgw9RErSKcCginiCw7MHqeDoXjsuW69i' +
'WxWKDgAjGAaQQvTiMBuN1ugSmcgaaDsOSyDjA/Tm2FEN2E33AMEwTlUtAACAgAVAM2yhh0O0gVFuJCQHnogCO4QonwkVR4ShoaiOHmq/iCPTwiQmuBmgXGjgqjh2RA2H' +
'CUfHoiES4gi7EgcyU/xymE4UwSNCU3EMVA2YikFCiSycVCIBBGR2CdGQUBU9wqWVswAUgRIwGwIlkBFmImECyGcEUL3SESm1HIOFS9kgxRQsQIJCosyAmQg8HIslA+RY' +
'OEI6EwtS2cHyiiYJHAlAC0iiAUmEzEiksmHUrWUKBqAoih0OMyAIAAKSQFwnRTAEmS9Mwa36GQOhhCc5BcA8gAcFAfTJCEOSiDEsSaNEuC0EMrjRD8XB4LYziiOIJgNA' +
'CNAAEkRROEQrhCGAgkBA');
end;
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM').Pictures := 'pic1';
end;
EndUpdate();
end
|
286
|
I need a border around each event/item on scheduler. Can you direct me to propery to use (to all)
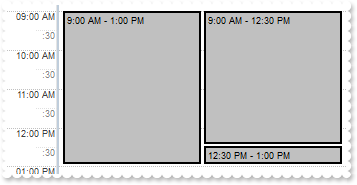
// AddEvent event - Notifies your application once the a new event is added.
procedure TWinForm1.AxSchedule1_AddEvent(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_AddEventEvent);
begin
// Ev.BodyBackgroundExt = "[frame=RGB(0,0,0),framethick]"
end;
with AxSchedule1 do
begin
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
Calendar.Selection := '1/10/2001';
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
StatusEventSize := 0;
set_DefaultEventPadding(EXSCHEDULELib.PaddingEdgeEnum.exPaddingAll,4);
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM');
Add('1/10/2001 9:00:00 AM','1/10/2001 1:00:00 PM');
Add('1/10/2001 12:30:00 PM','1/10/2001 1:00:00 PM');
end;
end
|
285
|
I need a border around each event/item on scheduler. Can you direct me to propery to use (distinct)
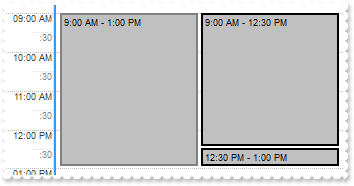
with AxSchedule1 do
begin
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
Calendar.Selection := '1/10/2001';
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
StatusEventSize := 0;
set_DefaultEventPadding(EXSCHEDULELib.PaddingEdgeEnum.exPaddingAll,4);
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').BodyBackgroundExt := '[frame=RGB(0,0,0),framethick]';
Add('1/10/2001 9:00:00 AM','1/10/2001 1:00:00 PM').BodyBackgroundExt := '[frame=RGB(128,128,128),framethick]';
Add('1/10/2001 12:30:00 PM','1/10/2001 1:00:00 PM').BodyBackgroundExt := '[frame=RGB(0,0,0),framethick]';
end;
end
|
284
|
How can I change/specify the caption of the groups, when the user clicks the drop down button
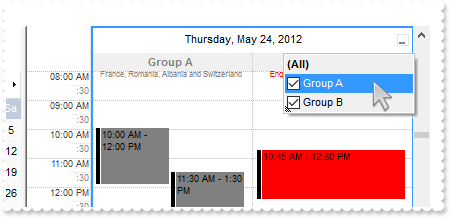
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
with Groups do
begin
with Add(1,'Group A') do
begin
Caption := '<fgcolor=808080><c><b>Group A</b><c><br><font ;6>France, Romania, Albania and Switzerland';
Visible := True;
EventBackColor := $808080;
end;
with Add(2,'Group B') do
begin
Caption := '<fgcolor=FF0000><c><b>Group B</b><c><br><font ;6>England, Russia, Wales and Slovakia';
Visible := True;
EventBackColor := $ff;
end;
end;
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM').GroupID := 1;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 2;
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM').GroupID := 1;
end;
EndUpdate();
end
|
283
|
Is it possible to hide the group header, but still display the groups/captions
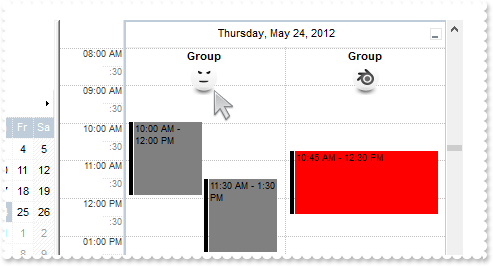
with AxSchedule1 do
begin
BeginUpdate();
set_HTMLPicture('pic1','gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa' +
'2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdP' +
'yyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QW' +
'k4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW' +
'4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByE' +
'QGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2Cydh' +
'Gg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIie' +
'RIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpP' +
'hyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaG' +
'wnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBM' +
'GQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxT' +
'AmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+Asf' +
'wMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB' +
'4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0Hgfx' +
'niuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQ' +
'XQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCy' +
'EkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJ' +
'h4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6H' +
'sXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2D' +
'eE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo' +
'8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0' +
'CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5Ag' +
'jtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS' +
'43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtipG' +
'0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtA' +
'uBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKg' +
'FBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZB' +
'IAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnB' +
'dAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB' +
'7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhvB' +
'PlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfA' +
'bAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpB' +
'ig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA' +
'2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/h' +
'EhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9h' +
'IgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hzB' +
'7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGg' +
'KBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA' +
'/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAACB5g' +
'kgiAggqAsAEhigrglg0ASAqgKBWgng7hSg9gdBPyphph0gQgeoOgyBNBMBIBMBnhjBsgEBJgMACAIgSBhAQAzAwg7BXA6A4BKgUBHB8Agg4BJgIgBA3ASAQAFAIhkhhA' +
'EA/ABRgBIhQAFgTgQhFARAEgsgkhngmAMAdhJh6A3gdBUhXBHhzhaB5hFB1HEgNgasJAOB1Bq3OB3Aeh3vth3A9gSgkh/g5hJ01A/hthbB9hQB/gCB4DPgCA9BAAeBYA' +
'Gh2AAhvgBAvhkBZBOBiB5gsB2gsACBwB8AFBWBegGA6AOLkgvgiBvAABIhvh/AnAvhUhShIBmgzBRhLAyg+gDABB3gBgOhAA6AmAuBDBvA7hTA0hfAagfgfhXhrB5A4g' +
'yACAHAHgHhdh+A/B/gTh8AcAvBlhgOnhfBngyAZK2A4BMAlgqBohVAJAUAKAFALgaAmgthBA7AlhigLh/ghBAA0LxBchch8g6A7g7hbh3B+heBBBgB3B3A3ARhtBLgsB' +
'fgXBXh5A/g+B/hqBzh5heh0BagohIgBg4B/ApAatAg6BdBBBWBrhMA8AbgGAhhlByg5AvB+gzhFhihxBxghAjgeBlgEByB5h8g5gMA8BQgtBRAGh6B7gjgTZzANArArg' +
'rh+hquoAlhSgpB5B6B6A6BYBb57g858hcghAHhbAvhWhMhKAkBeKBguh/hRgNAjAjgjh6h0BshZg7AWALBZhD6KvyAtgtg7husuhJhJh7gYAzAihRhohJBnBtBGgNg9h' +
'3A4hogfgcAcA8ApBmBPBnhZAEAwALBngwBtp5gYAZgZhJg9h6BpBSgxA1g1hVga6XgsA2A3g3wIA9AR6pA9ARBIgYBnhjhxgTBRBIB2h3B0gYhuBxhRAHhPgnhciZh1g' +
'CAzAEgGBWBdADgPgoA/gXgIAEAuAKBbA+hRBBhRBohSgUAygAgIghgTh+hGAYBrghBQhoA2gNA7AEAQB5hxgmBSBpg0B4AJhChPg5ABAIBUBTgi4jAHBEBEgdAIhAB5h' +
'hA0gIggAhB5ACAWgahDgABFBjh3hThKABgJB5B2gzAagBBfhwB4AIh5BFAThbhohjBjBDT3BIgXgSgoBEhtgkAyAHARhkAtBoBrBrAzAqgMBcAXhgBbgkh7hghyAiBQB' +
'ShSgShEgphFhzh7hIgzhgB2B2A2A4A0g0hUgXAMAGAlA6hNATB8k5gAgMgdBjBWB0BuBOBfA/AegBgBAjh8hTh3BZA9hoBUgBgKBRBvAvgChhhrhuAFBvA/AZBMgqB7B' +
'7h7hKh2h2g2gPg1A9hEgkANgOAjhhAwh9gxARAwg8B6heh3gQAdPFgiA/beAZABAd8lgQgKBEgPggBBAzhhBEgbhnAPBwg/hnA2hcBWB5AQBCA8gUBehjhIAfAABMAkB' +
'6BbhuhnBsgXgRggAAhhB5j+A/qYgEhbhrkOgqBuAIhVhuhrgyBuAuBLAdolAXBBggAGAyNFAABCgnhRALBUh9gdAFB6AOguhlAYB5hHhIByB3grB9hrAlhAAHBKBChCg' +
'CgNB8BdBf9XgngqhqhDgkAAAoAw7ShThwhsBrBoB7hRgvgBByg+AjhBBUgABkgJAuAjAMhEh+gggeAPA/gJg7BOhuh1ATBxBOgIvMAthpgv9wcYg1A1AFBnhmhmguh27' +
'7ZQhiAGcchkgIgOhcBGhYBXgUBZbABVc5gTB1BEgAhJBUha+BB0h4+Chng4hjgyBygd7agAA1hCAQUPgRg8A7hNAQBQBwhaAFBQh0BCB4g4h1hoeRBhgp+Shnhxi8hhg' +
'ygagABrAZAWBcBkgtgQA0Ahr4gkhLgvdwB/hzBtBagrgfBfA6h1hnAmguAiA8ANgLACA8AUgagOg3h0d5iBgBBCBjbIhWAGgYANAggsBthwBQAOzogx+yBHr+AGAuA0A' +
'0A2AGBPZoBnBAAwAIgnB5hcBmB3BWhWBWB+BdBcBcB8AZhsU6hxBuBgh7g7gwgVgBhuABiEh1giAmgBASAiABBWBkhAgZg0AEA1BRhQgNAwhyAgAMA0hsAxBNg7BsAbB' +
'mgQBxAUJZB1hQgMBZAVAPrchOBYgyg8gPg0hYA3gUAzAtBpB6gaB2Qvhhg/gEBoA8gshMg7AlAYgDBsBcAbACAKg6hWCthtqBAAhXgvhDgsA9hDAZAygthLADAVgSAkh' +
'hBsANBLAAhZgdBTArhAAQBDgTh6gEBnBNBHAVhrAPBAFkUnmLgChRECkYhG03G4rBi83imysh3uoBMaTqulIkxwIyAHSCmCcCGclnAegWGTOzSSUVurCrHwKymKmBA5E' +
'eSAivxCGV8rEopTmNwCOnA+mkIQ6sSeiEQlkKHTkp36CVC7BWPDcJ3SmgBYAASSKTiIriEYEFAQ=');
set_HTMLPicture('pic2','gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa' +
'2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdP' +
'yyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QW' +
'k4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW' +
'4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByE' +
'QGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2Cydh' +
'Gg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIie' +
'RIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpP' +
'hyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaG' +
'wnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBM' +
'GQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxT' +
'AmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+Asf' +
'wMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB' +
'4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0Hgfx' +
'niuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQ' +
'XQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCy' +
'EkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJ' +
'h4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6H' +
'sXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2D' +
'eE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo' +
'8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0' +
'CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5Ag' +
'jtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS' +
'43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtipG' +
'0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtA' +
'uBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKg' +
'FBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZB' +
'IAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnB' +
'dAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB' +
'7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhvB' +
'PlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfA' +
'bAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpB' +
'ig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA' +
'2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/h' +
'EhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9h' +
'IgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hzB' +
'7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGg' +
'KBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA' +
'/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAADBng' +
'kgiAggqAsAEhiiig2AqUgBPA5hnB3Bshug3A9g7BbhthqALPBglN0gCgUoQh4AQAIgAgVgTAhBEAIBYACBIhEB4hggoASBiBKBsBJARRDhwBkgMABBrAQADA+AUAlAmh' +
'GBJBaAVALghgSgaA2gpApgpgEgigEBehTBAhLB2g7Bdhdgzhnhih3gvg9AbA9gWhUAHgnB5BPhMgZh5hnh/g/h5hvgzAMB9hPgNh7gnh6h1vtAIA9hVAggVggAbAoWrg' +
'AB1ABhGhBg/AAg+hng4huAAB9BjhbATiLhihNgQB2gegoBkgxrEgxAQAQBGgABpAdgJgfgfhpgRBWh8h+tWgmhpgXAABgAzuAYRAhAtAEhfBVhVBVA/BsLNAWAyBpAZh' +
'RgChhBwg4BUjtB/LiAehMgCAeBwg+B9hiY9g7BThpgkgXAlA0AMgAgCBBhghshRAUBbBBBthOhYBxgvh/BgANAOgbg3hvAuAvgvAvAXBjhFgkB1hBADgHgPAkATBJh0g' +
'WAbBcgzgZA75YBbhUhWBaBqBegxhjgHAEg9hcBMg8BQg6V8h1gegPBXhOBjA0gAgkX2AFhggphNBmBOB+ZSgDBxBdAdAdhihWgagahXBdg7h3gzB6B6h6BvBmhIgkAyA' +
'6uihKAGhAggBQAoAIBCBJgwAZBigZhTANhz5+A1hs5/g9A3g3hXhEAgBygXAuhdAyAshaA0AohiX/A1h0h8BThzheAFgLAWAcA9h/B+A8hOh/ApgxAABAh7gIgHB6g7B' +
'eBABghggghfBWhWgmgCAYADBrgYAJg9AAhehgB5hGBj6KBbAbA1g/21gEgQBMBs40AHgOAeB0AV6oAphxAyhiBHhghbhuoEB/AgA5gUgAAhBQhoBPhMBHgCgPBpBEAqg' +
'qAqBcBIg9guArArALAZgEABBMBqlEAzAohGQ8gAgjACgASQq7hNAvBvhoBwBhhfAmAQBfKYg7gEAgBBAFhQBuBFhcg7g0s6gSMxhhAageBgB4BEAHgjBKBJhOhmh2A7V' +
'4jtBGAuAogcA1hLAlAQKehwBFhdBxgshXgrgUgUAUAkBzhHBhAhhDgHA+glAdAJAWAAASh1hQh4A5ghg8g5Bvg7h1hLB0h0BohRhJBVAUAeAshgApgqBSBeBbA4gxhQB' +
'SBSASAxB4A7A6A6AsBnBOAfBOgegbAbOoYxAb5uBGgkgHAjhqBvgDAHh64bAOAHAYQPACh4BegTgpgVA68JhMh7h3hvh3hABKg8BqADvRBLhThqh3BvB2hBgICDA5hHA' +
'PgdhzA2Bcg4BggHBZhlhdh7h2B4whAoBDgagIA2BbAGAZB5gEgkABAAB+AFh0hMAXgvhfZVhqBrglglASBhAQhkAMBVARg9hUhdA9AVARBlhuBkgJgTgOAe8zBLBwhQg' +
'Qhp7dhXhtBfTJBJBFBFhFhKhyhlhLhDgUAfBkARB0gyBhBpBrAKAAAwAGATAZhb9FBNhQgeFVgNBGhDgjhchnhzBmBMgJh0h1h1gZgngmgmhigHAAgFhZBthMAhhshyg' +
'EgCAygFnlB7AoBIAjgbg0BRACgKAihchLgGAwAvguguhHBGBJgzDtB9BzAWgtgBBwAwgiBJhAgAAyhChTAQglg0AHgfASAXhNBTAkArtGhLAAApgtATBlhSA/gAAMASB' +
'/AMA9B9HUhPAyBzBzgrhWBIYwhVByBhgjhHB8ArBXgsgJgRAqhEABhrA4hxhDAygXBBBXheBRARAGAwgmBBgoBZBgBVh1hrAmgtAWALBO8sBkh5BnBPgPgkhLAEgqgBh' +
'jBihKAoB2goBgklgfBdBfgxgyhcBYhYg4hJBgAMg2hkhJAShwg1hrhXAsgog+A2VGBHhZA6htgbB/hOgdg7hBARhhhhgTBqB9BEAVgbgrh+hTBchsg/AHhCgUgBAlheh' +
'cA1AqhkBsA1iOBqPEgJhpBIATA+gfAvgWgnApA3gYASB725he22BXgJh3gCAggiAQhVBWBWBKAog/ByhBBhAhA61gAvggBmAiBVgRLNgYAPhvAqgth1gnhLAAhFmbhcg' +
'uBugaBPgBAwAyAyBiBHAOB/gGgQAFBJgbgNB/hR/fB+BjhDgHglghhETkgkgIgsgggyhNAuAgBXAgBbBYAygqgqhQhbg7B3BOBSB6h8AjBThDh8gvgbhzCIBoBhB8f0h' +
'NgIB7BEhghKBXBThdEpDkheg/B+hdB8Brh2htgzBVBAEADiS0kU52cjV4vDsbRgwy6gA0pl5EQke1MpQKMAa4iWgDwJU68n+MHuAScfxUfj+wi6EFuwHC5Wkhgkuwkc4' +
'0G2qeE8elEOF8lHCNAeTwsMTwqWOyWeIGQjxIbyQB0AFHgLayJHARwI0iE+HuGQEAAlT0IR0KiDWW2I2EYcUoaE8/giYAEiGAEiijXAEGwpx4HmeAWQNXcBFGTwaQikD' +
'lE9Bo41iYTuOA4zjnB2qzk2NzeEy4YkGwlQIikAE8hGM805rEEAmAKmUtQEkHuO9yj2u1GofEONxMVR8SiSulw6EMjEESDOTgGgEQi2MjjU5mI5l4pSWl0s1e+vUCXC+' +
'iGQtjEIwOCXi+xqAGowVeoQU+jgeX63GgsHeq1CoTREEpAkL0hgNAA9AoFUQTRBYLzAJgIwAEQIhZAAZjNIEUAXMw+gUBQPCNHE0YFBsKgXC8Xg8DsRySGwhQFAWFQYI' +
'E0gAB4pjjFcrhcPk4ziMUiy/AMzQwAIZwUPsxwgGsAg0AUCiCOgkQyhEAhFAAzAgCs6h9BYjAqCkgjiEQDA4BEhhuF01SdLoqjaGARwjIY4APF44QEC4JBwNwhDOHEDw' +
'RAYDwVF49QxFk9hBA4KQnPMh4BDw9hIEkKDFFgoBGL4IQiEYGS+OQZBdKYLDAIsSwLMM9xkMo+y4OsRRICEkAEBgqShCkEArOMeBBEwTCiCcPgiDoNQpE48A9P4owtJ0' +
'CxoMEsACFkQjIM0zQVCk8hRH4LT4LcoT2FkZAmBMeCyAA8QYIASQJBEnSWIg/BtMw0SpOkUDEBsayZAAEADK0GTrAYICzIsuTMLgkBNH45gnOU8QAEgAT5JcFCSCIGgT' +
'BkFiNJkHRzLE9g3CA5QJAk0CGBF7gAywSRFE4RCuEIYCCQE=');
Calendar.Selection := '5/24/2012';
DisplayGroupingButton := True;
ShowGroupingEvents := True;
with Groups do
begin
with Add(1,'<c><b>Group</b><br><c><img>pic1</img>') do
begin
Visible := True;
EventBackColor := $808080;
end;
with Add(2,'<c><b>Group</b><br><c><img>pic2</img>') do
begin
Visible := True;
EventBackColor := $ff;
end;
end;
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM').GroupID := 1;
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').GroupID := 2;
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM').GroupID := 1;
end;
HeaderGroupHeight := -1;
EndUpdate();
end
|
282
|
When using the CopyTo method to print a range of dates the printed page is not showing the timescale, is there a way to make it appear (as is, without calendar, multiple-selection)
with AxSchedule1 do
begin
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
with Calendar do
begin
Selection := '#1/1/2016#';
Selection := '(int((yearday(value) -1- ((7-weekday(value - yearday(value) + 1)) mod 7) )/7) = int((yearday(#1/7/2016#))/7))';
end;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
with Events do
begin
Add('1/10/2016 8:30:00 AM','1/10/2016 2:30:00 PM');
Add('1/11/2016 9:30:00 AM','1/11/2016 11:30:00 AM');
Add('1/15/2016 10:30:00 AM','1/15/2016 11:30:00 AM');
end;
s := Calendar.Selection;
Calendar.Selection := '0';
var_CopyTo := get_CopyTo('c:/temp/test.png');
OutputDebugString( 'Look for c:/temp/test.png file' );
Calendar.Selection := TObject(s);
end
|
281
|
When using the CopyTo method to print a range of dates the printed page is not showing the timescale, is there a way to make it appear (as is, without calendar)
with AxSchedule1 do
begin
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
with Calendar do
begin
Selection := '1/1/2012';
SelectDate['1/1/2012'] := False;
end;
with Events do
begin
Add('1/1/2012 8:30:00 AM','1/1/2012 9:30:00 AM');
Add('1/1/2012 8:35:00 AM','1/1/2012 9:35:00 AM');
Add('1/1/2012 10:30:00 AM','1/1/2012 12:30:00 PM');
end;
var_CopyTo := get_CopyTo('c:/temp/test.png');
OutputDebugString( 'Look for c:/temp/test.png file' );
end
|
280
|
When using the CopyTo method to print a range of dates the printed page is not showing the timescale, is there a way to make it appear (as is, with calendar)
with AxSchedule1 do
begin
with Calendar do
begin
Selection := '1/1/2012';
SelectDate['1/1/2012'] := False;
end;
with Events do
begin
Add('1/1/2012 8:30:00 AM','1/1/2012 9:30:00 AM');
Add('1/1/2012 8:35:00 AM','1/1/2012 9:35:00 AM');
Add('1/1/2012 10:30:00 AM','1/1/2012 12:30:00 PM');
end;
var_CopyTo := get_CopyTo('c:/temp/test.png');
OutputDebugString( 'Look for c:/temp/test.png file' );
end
|
279
|
I've seen that all-day header can be limited up to 4, 8 or 12 events. Can I change that limit, for instance, 3 events only
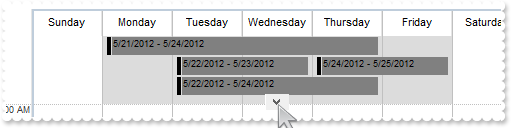
with AxSchedule1 do
begin
BeginUpdate();
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value >= #5/20/2012# and value <= #5/26/2012#';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := EXSCHEDULELib.AllDayEventScrollEnum($23 Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventWheelScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventNoMax));
BodyEventBackColor := Color.FromArgb(128,128,128);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleAllDayEventScrollBackColor,$dcdcdc);
with Events do
begin
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/25/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
278
|
Is it possible to highlight the newly created event ( runtime creation )
with AxSchedule1 do
begin
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
end
|
277
|
Is it possible to select the newly created event ( runtime creation )
with AxSchedule1 do
begin
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exSelectCreateEvent;
end
|
276
|
I have noticed that the all-day header change its background, if has scrolling events. Is it possible to change that (ebn)
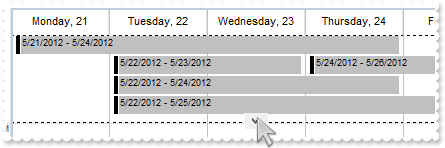
with AxSchedule1 do
begin
BeginUpdate();
VisualAppearance.Add(1,'gBFLBCJwBAEHhEJAAChABRUIQAAYAQGKIaBoAKBQAGaAoDDQMQ3QwAAwjSLEEwsACEIrjKCRShyCYZRrGQBQTCIZBqEqSZLiEZRQCWIAxATGchwHIEQgND6cIDmMAHfj' +
'2PI+RZKMoRZJUExZFyERhASQZZoyN40UzOc6vfL9KRDEAEIRKAyTDLQdRyGSMMgEVBJBCbMiNBqhESIJo+GI4BhFYSUZalGSnO6gIBAgIA==');
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventWheelScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventMax4);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleAllDayEventScrollBackColor,$1000000);
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/26/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
275
|
I have noticed that the all-day header change its background, if has scrolling events. Is it possible to change that (solid)
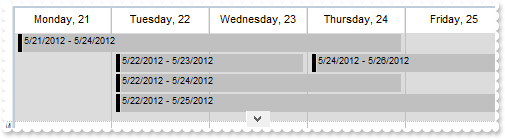
with AxSchedule1 do
begin
BeginUpdate();
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventWheelScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventMax4);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleAllDayEventScrollBackColor,$dcdcdc);
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/26/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
274
|
I have noticed that the all-day header change its background, if has scrolling events. Is it possible to remove that
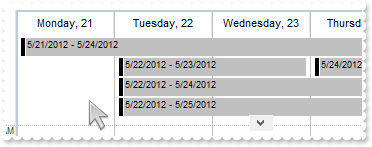
with AxSchedule1 do
begin
BeginUpdate();
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventWheelScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventMax4);
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleAllDayEventScrollBackColor,$0);
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/26/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
273
|
The user scrolls through the all-day events, when mouse wheel is rotated. Can I disable that
with AxSchedule1 do
begin
BeginUpdate();
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventMax4);
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/26/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
272
|
Is it possible to specify the number of all-day events to be visible
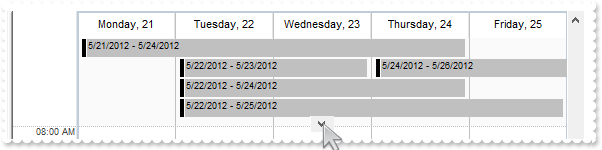
with AxSchedule1 do
begin
BeginUpdate();
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventWheelScroll) Or Integer(EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventMax4);
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/26/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
271
|
How do I disable scrolling the all-day header
with AxSchedule1 do
begin
BeginUpdate();
AllowSelectCreateEvent := EXSCHEDULELib.SelectCreateEventEnum.exHighlightCreateEvent;
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
Calendar.Selection := '5/21/2012';
Calendar.Selection := 'value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)';
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowAllDayEventScroll := EXSCHEDULELib.AllDayEventScrollEnum.exAllDayEventNoMin;
with Events do
begin
Add('5/22/2012','5/23/2012').AllDayEvent := True;
Add('5/21/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/24/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/22/2012','5/25/2012').AllDayEvent := True;
Add('5/23/2012','5/25/2012').AllDayEvent := True;
Add('5/24/2012','5/26/2012').AllDayEvent := True;
end;
EndUpdate();
end
|
270
|
How can I display the current week only, when the user selects the date in the calendar panel
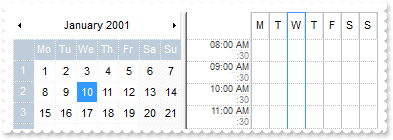
with AxSchedule1 do
begin
BeginUpdate();
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exDisableSplitter) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
with Calendar do
begin
OnSelectDate := EXSCHEDULELib.OnSelectDateEnum.exEnsureVisibleDate;
Selection := '1/10/2001';
SingleSel := True;
end;
Calendar.FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exNoScroll;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
AllowResizeSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMoveSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderMonthColor,get_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderDateColor));
TimeScales.Item[TObject(0)].AllowResize := False;
DayViewWidth := 0;
EndUpdate();
end
|
269
|
How do I capture the control and save it as PDF (Method 2)
with AxSchedule1 do
begin
with Calendar do
begin
Selection := '6/1/2001';
Selection := 'value in (#6/11/2001#,#6/12/2001#)';
end;
DayViewWidth := 512;
DayViewHeight := 512;
with Events do
begin
Add('6/11/2001 10:00:00 AM','6/11/2001 1:00:00 PM');
Add('6/12/2001 10:00:00 AM','6/12/2001 1:00:00 PM');
end;
var_CopyTo := get_CopyTo('C:/Temp/test.pdf');
OutputDebugString( 'Look For: C:/Temp/test.pdf file' );
end
|
268
|
How do I capture the control and save it as PDF (Method 1)
with AxSchedule1 do
begin
with Calendar do
begin
Selection := '6/1/2001';
Selection := 'value in (#6/11/2001#,#6/12/2001#)';
end;
DayViewWidth := 512;
DayViewHeight := 512;
with Events do
begin
Add('6/11/2001 10:00:00 AM','6/11/2001 1:00:00 PM');
Add('6/12/2001 10:00:00 AM','6/12/2001 1:00:00 PM');
end;
with (ComObj.CreateComObject(ComObj.ProgIDToClassID('Exontrol.Print')) as EXPRINTLib.Print) do
begin
Options := 'FitToPage=On';
PrintExt := (AxSchedule1.GetOcx() as EXSCHEDULELib.Schedule).DefaultDispatch;
CopyTo('C:/Temp/test.pdf');
OutputDebugString( 'Look For: C:/Temp/test.pdf file' );
end;
end
|
267
|
How do I capture the control and save it as an image (JPG or BMP) (Method 2)
with AxSchedule1 do
begin
with Calendar do
begin
Selection := '6/1/2001';
Selection := 'value in (#6/11/2001#,#6/12/2001#)';
end;
DayViewWidth := 512;
DayViewHeight := 512;
with Events do
begin
Add('6/11/2001 10:00:00 AM','6/11/2001 1:00:00 PM');
Add('6/12/2001 10:00:00 AM','6/12/2001 1:00:00 PM');
end;
var_CopyTo := get_CopyTo('C:/Temp/test.bmp');
var_CopyTo1 := get_CopyTo('C:/Temp/test.jpg');
OutputDebugString( 'Look For: C:/Temp/test.* file' );
end
|
266
|
How do I capture the control and save it as an image (JPG or BMP) (Method 1)
with AxSchedule1 do
begin
with Calendar do
begin
Selection := '6/1/2001';
Selection := 'value in (#6/11/2001#,#6/12/2001#)';
end;
DayViewWidth := 512;
DayViewHeight := 512;
with Events do
begin
Add('6/11/2001 10:00:00 AM','6/11/2001 1:00:00 PM');
Add('6/12/2001 10:00:00 AM','6/12/2001 1:00:00 PM');
end;
with (ComObj.CreateComObject(ComObj.ProgIDToClassID('Exontrol.Print')) as EXPRINTLib.Print) do
begin
Options := 'FitToPage=On';
PrintExt := (AxSchedule1.GetOcx() as EXSCHEDULELib.Schedule).DefaultDispatch;
CopyTo('C:/Temp/test.bmp');
CopyTo('C:/Temp/test.jpg');
OutputDebugString( 'Look For: C:/Temp/test.* file' );
end;
end
|
265
|
Recurrence: The 2nd to last weekday of the month
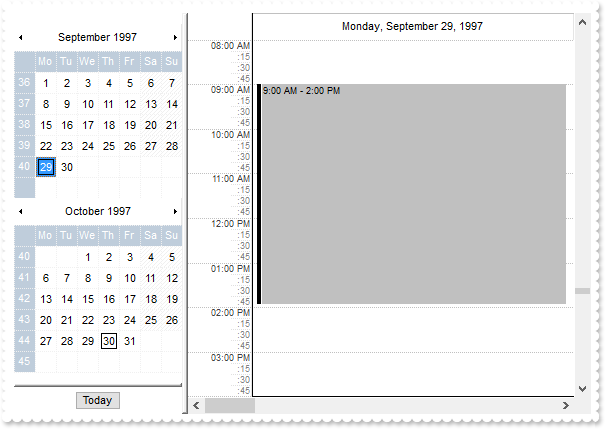
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/29/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/29/1997 9:00:00 AM','9/29/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-2';
end;
EndUpdate();
end
|
264
|
Recurrence: The 3rd instance into the month of one of Tuesday, Wednesday or Thursday, for the next 3 months
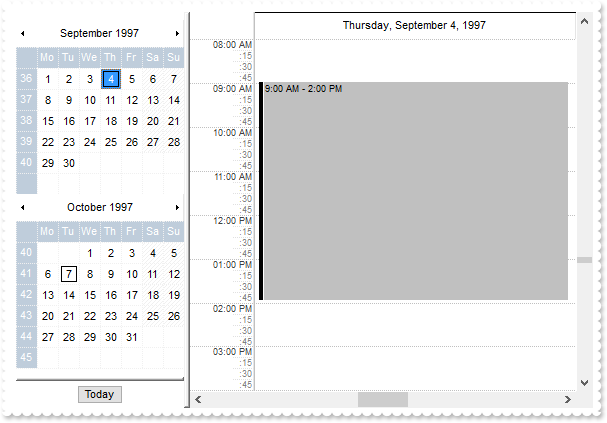
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/4/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/4/1997 9:00:00 AM','9/4/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;COUNT=3;BYDAY=TU,WE,TH;BYSETPOS=3';
end;
EndUpdate();
end
|
263
|
Recurrence: The last work day of the month
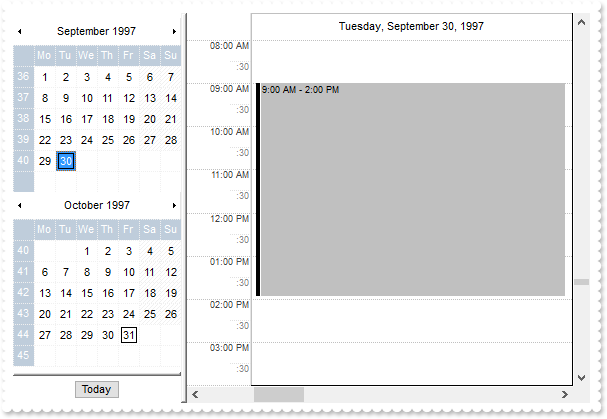
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/30/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('8/5/1997 9:00:00 AM','8/5/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-1';
end;
EndUpdate();
end
|
262
|
Recurrence: An example where the days generated makes a difference because of WKST (Sample 2)
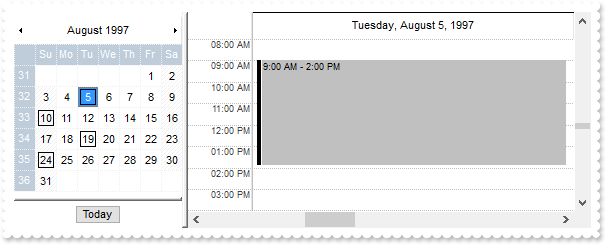
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '8/5/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exSunday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('8/5/1997 9:00:00 AM','8/5/1997 2:00:00 PM').Repetitive := 'FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU';
end;
EndUpdate();
end
|
261
|
Recurrence: An example where the days generated makes a difference because of WKST (Sample 1)
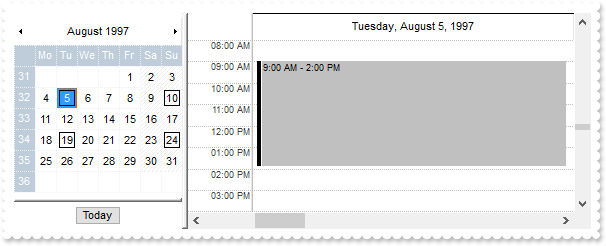
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '8/5/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('8/5/1997 9:00:00 AM','8/5/1997 2:00:00 PM').Repetitive := 'FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU';
end;
EndUpdate();
end
|
260
|
Recurrence: Every four years, the first Tuesday after a Monday in November, forever (U.S. Presidential Election day)
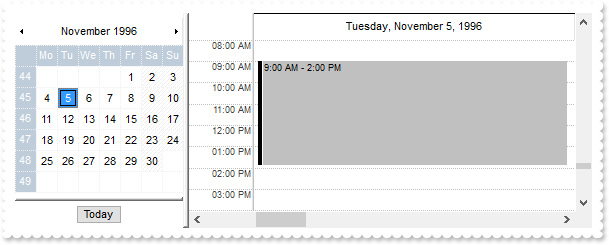
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '11/5/1996';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('11/5/1996 9:00:00 AM','11/5/1996 2:00:00 PM').Repetitive := 'FREQ=YEARLY;INTERVAL=4;BYMONTH=11;BYDAY=TU;BYMONTHDAY=2,3,4,5,6,7,8';
end;
EndUpdate();
end
|
259
|
Recurrence: The first Saturday that follows the first Sunday of the month, forever
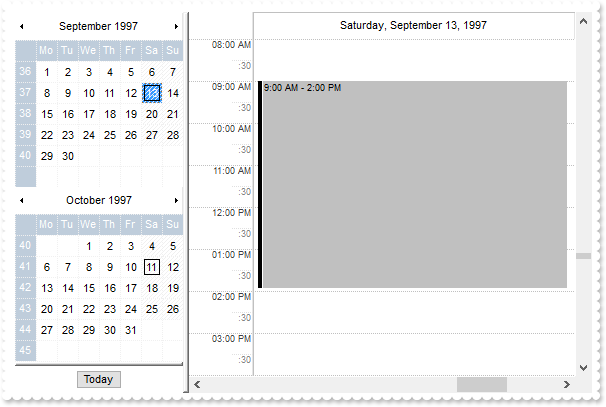
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/13/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/13/1997 9:00:00 AM','9/13/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;BYDAY=SA;BYMONTHDAY=7,8,9,10,11,12,13';
end;
EndUpdate();
end
|
258
|
Recurrence: Every Friday the 13th, forever
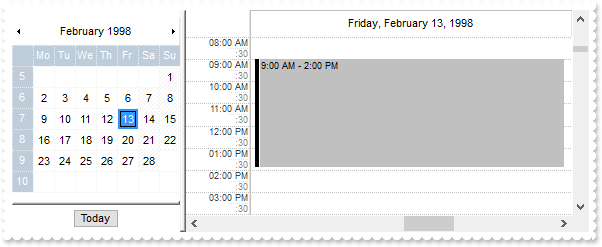
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '2/13/1998';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;BYDAY=FR;BYMONTHDAY=13';
end;
EndUpdate();
end
|
257
|
Recurrence: Every Thursday, but only during June, July, and August, forever
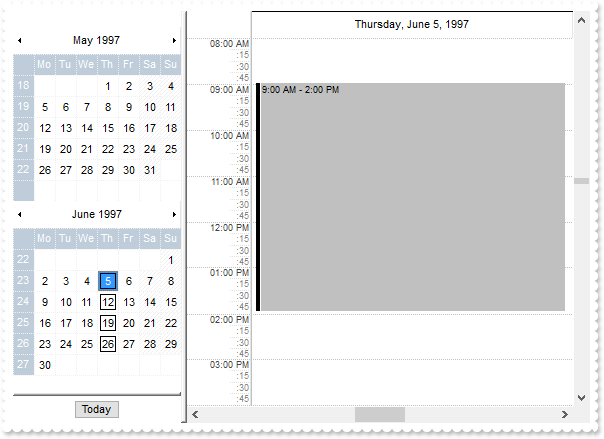
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '6/5/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('6/5/1997 9:00:00 AM','6/5/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;BYDAY=TH;BYMONTH=6,7,8';
end;
EndUpdate();
end
|
256
|
Recurrence: Every Thursday in March, forever
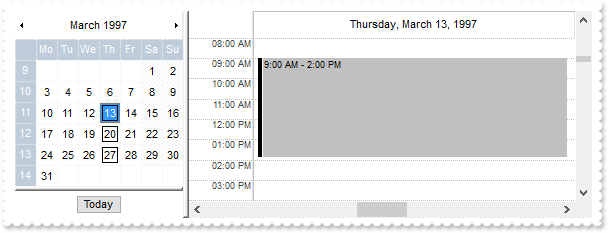
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '3/13/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('3/13/1997 9:00:00 AM','3/13/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;BYMONTH=3;BYDAY=TH';
end;
EndUpdate();
end
|
255
|
Recurrence: Monday of week number 20 (where the default start of the week is Monday), forever
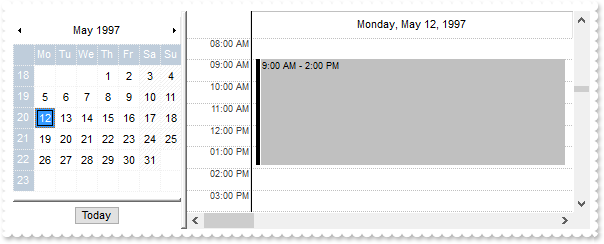
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '5/12/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('5/12/1997 9:00:00 AM','5/12/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;BYWEEKNO=20;BYDAY=MO';
end;
EndUpdate();
end
|
254
|
Recurrence: Every 20th Monday of the year, forever
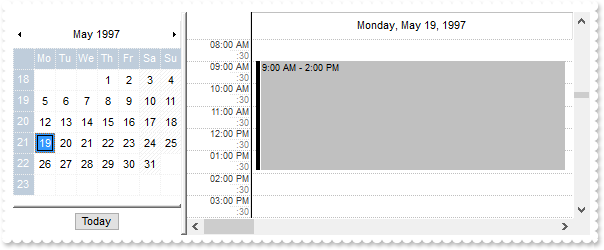
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '5/19/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('1/1/1997 9:00:00 AM','1/1/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;BYDAY=20MO';
end;
EndUpdate();
end
|
253
|
Recurrence: Every 3rd year on the 1st, 100th and 200th day for 10 occurrences
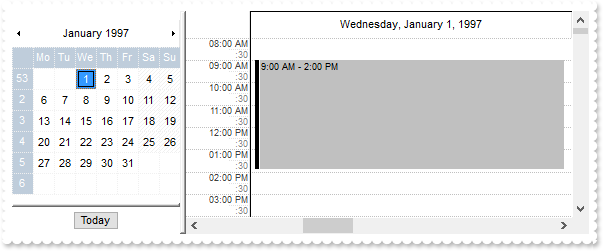
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '1/1/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('1/1/1997 9:00:00 AM','1/1/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;INTERVAL=3;COUNT=10;BYYEARDAY=1,100,200';
end;
EndUpdate();
end
|
252
|
Recurrence: Every other year on January, February, and March for 10 occurrences
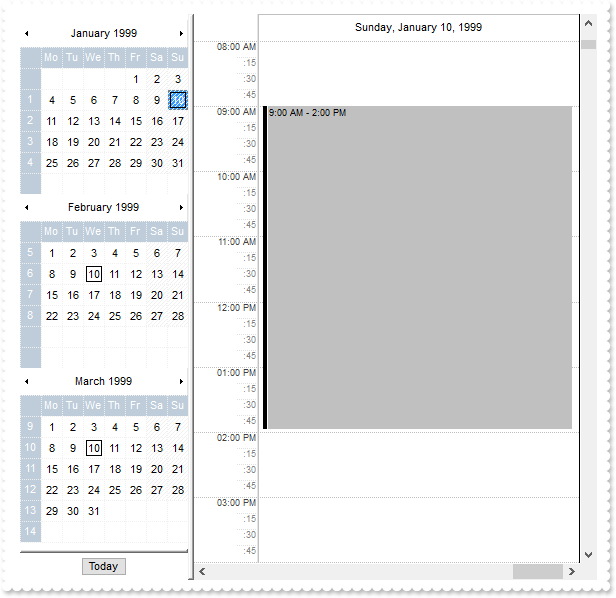
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '3/10/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('3/10/1997 9:00:00 AM','3/10/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3';
end;
EndUpdate();
end
|
251
|
Recurrence: Yearly in June and July for 10 occurrences
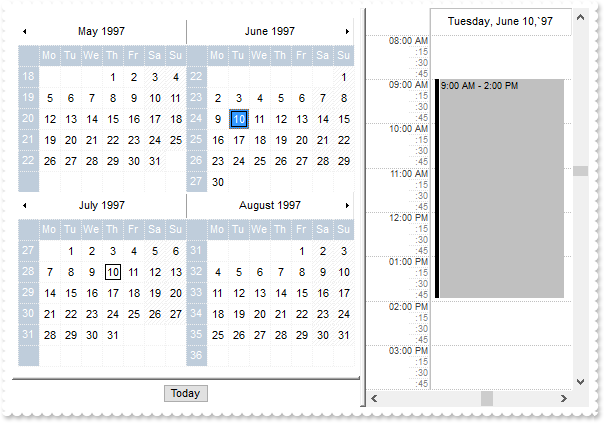
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '6/10/1997';
ShowNonMonthDays := False;
MaxMonthX := 2;
MaxMonthY := 2;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('6/10/1997 9:00:00 AM','6/10/1997 2:00:00 PM').Repetitive := 'FREQ=YEARLY;COUNT=10;BYMONTH=6,7';
end;
EndUpdate();
end
|
250
|
Recurrence: Every Tuesday, every other month
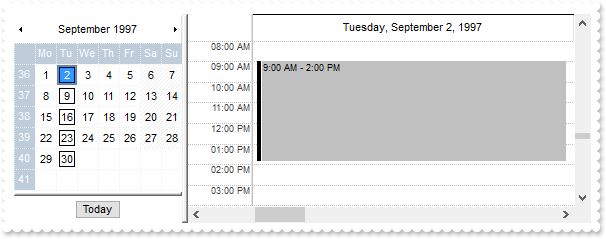
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/2/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;INTERVAL=2;BYDAY=TU';
end;
EndUpdate();
end
|
249
|
Recurrence: Every 18 months on the 10th thru 15th of the month for 10 occurrences
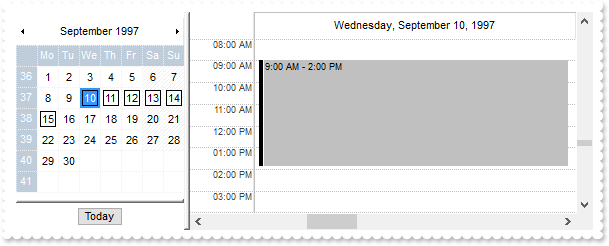
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/10/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/10/1997 9:00:00 AM','9/10/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;INTERVAL=18;COUNT=10;BYMONTHDAY=10,11,12,13,14,15';
end;
EndUpdate();
end
|
248
|
Recurrence: Monthly on the first and last day of the month for 10 occurrences
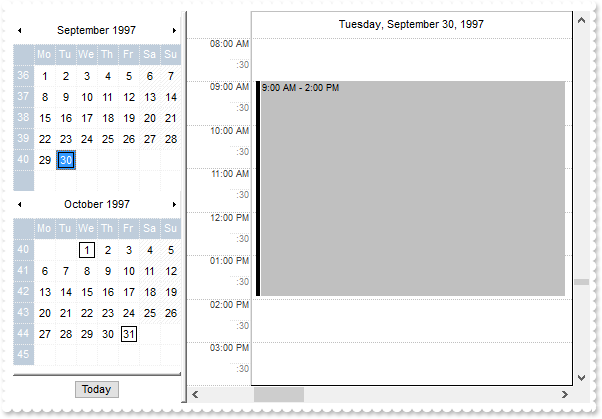
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/30/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/3/1997 9:00:00 AM','9/3/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;COUNT=10;BYMONTHDAY=1,-1';
end;
EndUpdate();
end
|
247
|
Recurrence: Monthly on the 2nd and 15th of the month for 10 occurrences
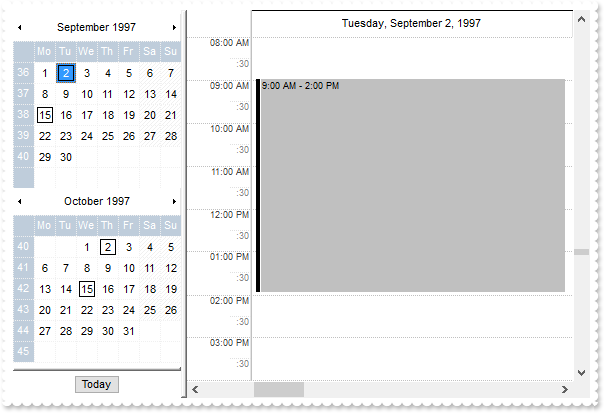
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/2/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;COUNT=10;BYMONTHDAY=2,15';
end;
EndUpdate();
end
|
246
|
Recurrence: Monthly on the third to the last day of the month, forever
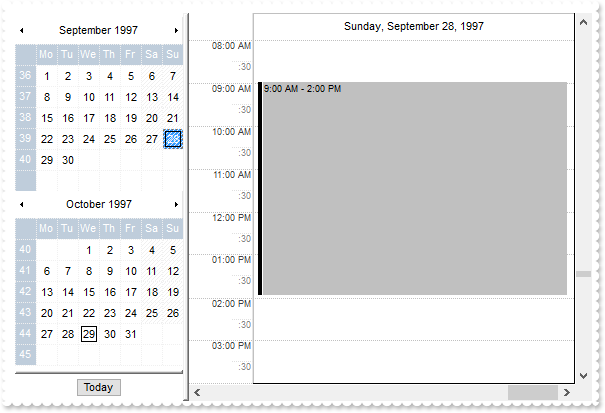
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/28/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/28/1997 9:00:00 AM','9/28/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;BYMONTHDAY=-3';
end;
EndUpdate();
end
|
245
|
Recurrence: Monthly on the second to last Monday of the month for 6 months
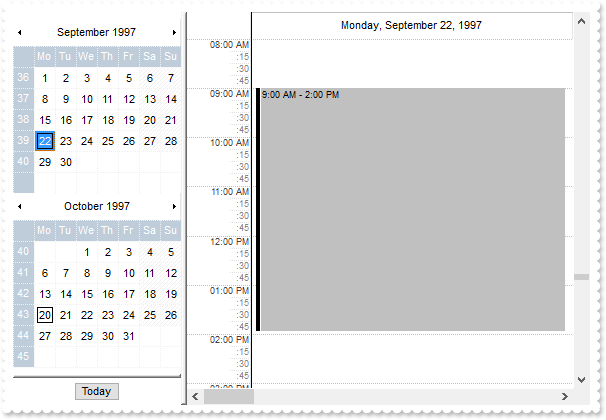
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/22/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/22/1997 9:00:00 AM','9/22/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;COUNT=6;BYDAY=-2MO';
end;
EndUpdate();
end
|
244
|
Recurrence: Every other month on the 1st and last Sunday of the month for 10 occurrences
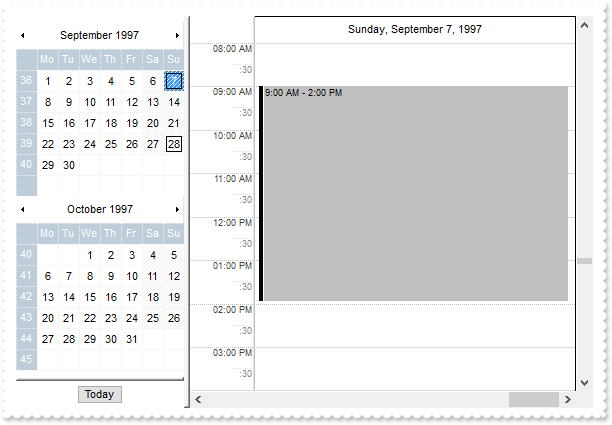
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/7/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/7/1997 9:00:00 AM','9/7/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;INTERVAL=2;COUNT=10;BYDAY=1SU,-1SU';
end;
EndUpdate();
end
|
243
|
Recurrence: Monthly on the 1st Friday until December 24, 1997
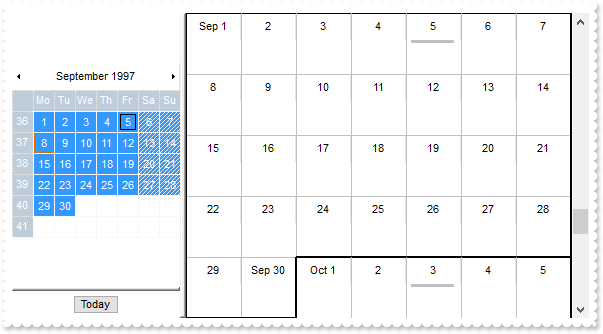
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/5/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/5/1997 9:00:00 AM','9/5/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;UNTIL=19971224T000000Z;BYDAY=1FR';
end;
EndUpdate();
end
|
242
|
Recurrence: Monthly on the 1st Friday for ten occurrences
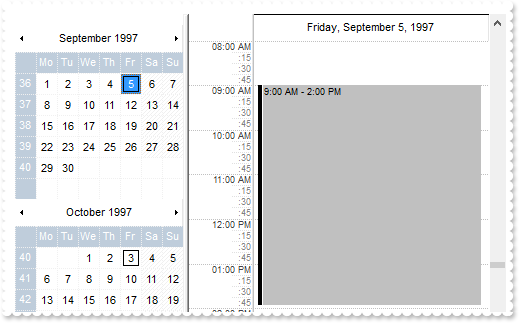
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/5/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/5/1997 9:00:00 AM','9/5/1997 2:00:00 PM').Repetitive := 'FREQ=MONTHLY;COUNT=10;BYDAY=1FR';
end;
EndUpdate();
end
|
241
|
Recurrence: Every other week on Tuesday and Thursday, for 8 occurrences
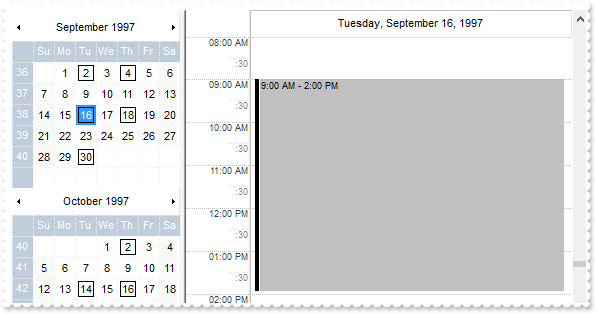
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/16/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exSunday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=WEEKLY;INTERVAL=2;COUNT=8;WKST=SU;BYDAY=TU,TH';
end;
EndUpdate();
end
|
240
|
Recurrence: Every other week on Monday, Wednesday and Friday until December 24, 1997, but starting on Tuesday, September 2, 1997
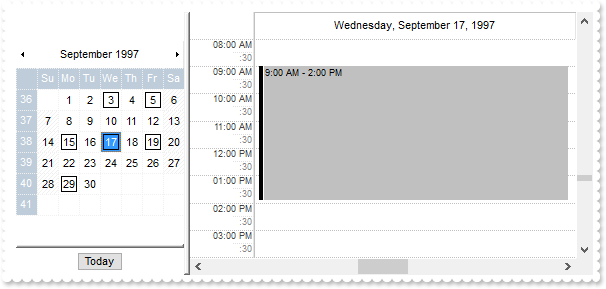
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/17/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exSunday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=WEEKLY;INTERVAL=2;UNTIL=19971224T000000Z;WKST=SU;BYDAY=MO,WE,FR';
end;
EndUpdate();
end
|
239
|
Recurrence: Weekly on Tuesday and Thursday for 5 weeks
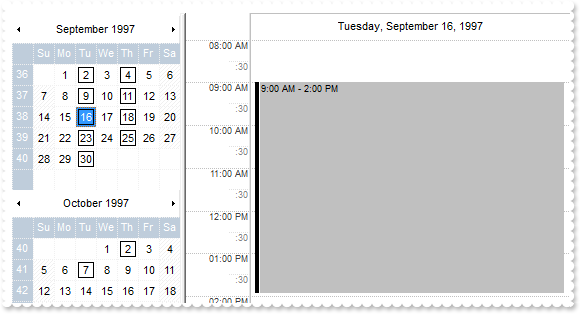
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/16/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exSunday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=WEEKLY;UNTIL=19971007T000000Z;WKST=SU;BYDAY=TU,TH';
end;
EndUpdate();
end
|
238
|
Recurrence: Weekly on Tuesday and Thursday for 5 weeks
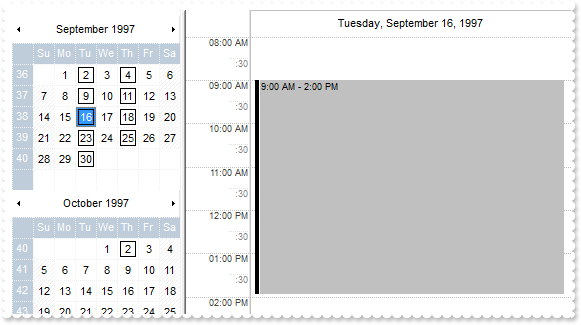
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/16/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exSunday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=WEEKLY;COUNT=10;WKST=SU;BYDAY=TU,TH';
end;
EndUpdate();
end
|
237
|
Recurrence: Every other day - forever
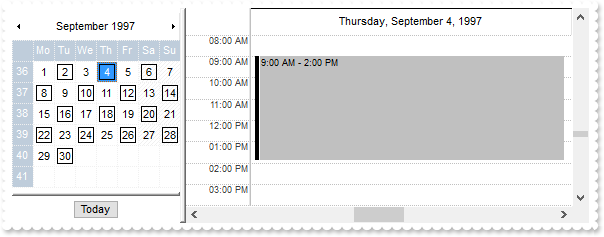
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/4/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=DAILY;INTERVAL=2';
end;
EndUpdate();
end
|
236
|
Recurrence: Daily until December 24, 1997
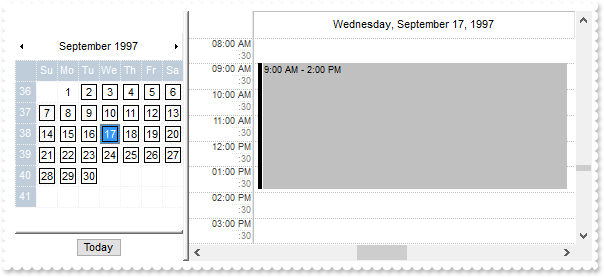
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/17/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=DAILY;UNTIL=19971224T000000Z';
end;
EndUpdate();
end
|
235
|
Recurrence: Daily for 10 occurrences
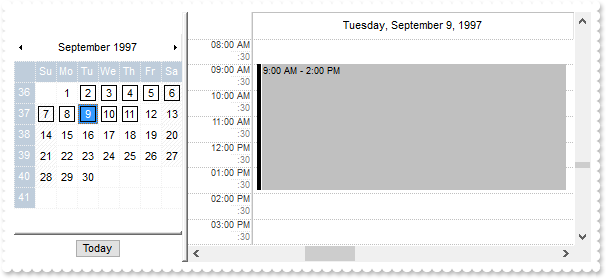
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '9/9/1997';
ShowNonMonthDays := False;
MaxMonthY := 4;
with HighlightEvent do
begin
Pattern.Type := EXSCHEDULELib.PatternEnum.exPatternFrame;
Bold := False;
end;
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
end;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewCalendarCompact;
BorderSelStyle := EXSCHEDULELib.LinesStyleEnum.exNoLines;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleMarkTodayBackColor,$f0f0f0);
with Events do
begin
Add('9/2/1997 9:00:00 AM','9/2/1997 2:00:00 PM').Repetitive := 'FREQ=DAILY;COUNT=10';
end;
EndUpdate();
end
|
234
|
How do I display the week number according, so the January 1st is in the first week
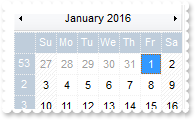
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '1/1/2016';
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exSunday;
DisplayWeekNumberAs := EXSCHEDULELib.WeekNumberAsEnum.exSimpleWeekNumber;
end;
EndUpdate();
end
|
233
|
How do I display the week number according to ISO8601 standard
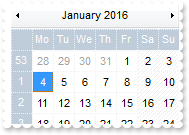
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '1/4/2016';
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
DisplayWeekNumberAs := EXSCHEDULELib.WeekNumberAsEnum.exISO8601WeekNumber;
end;
EndUpdate();
end
|
232
|
How do I hide the week number
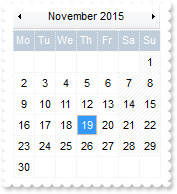
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
ShowWeeks := False;
ShowNonMonthDays := False;
end;
EndUpdate();
end
|
231
|
How can I change the background color of the all day header
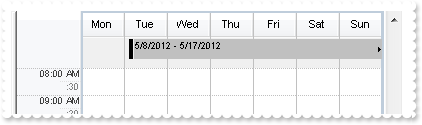
with AxSchedule1 do
begin
BeginUpdate();
OnResizeControl := EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide;
ShowAllDayHeader := True;
with Calendar do
begin
FirstWeekDay := EXSCHEDULELib.WeekDayEnum.exMonday;
SelectDate['5/8/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
SelectDate['5/15/2012'] := False;
Select(Integer(EXSCHEDULELib.SelectCalendarDateEnum.exSelectToggle) Or Integer(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek));
end;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleAllDayHeaderBackColor,$f0f0f0);
HeaderAllDayEventHeight := -20;
Events.Add('5/8/2012','5/17/2012').AllDayEvent := True;
EndUpdate();
end
|
230
|
How can I display a single text on a specified date
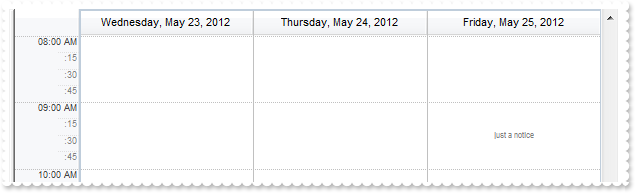
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/26/2012';
Calendar.Selection := 'value in (#05/23/2012#,#05/24/2012#,#05/25/2012#)';
NonworkingPatterns.Add(1234,EXSCHEDULELib.PatternEnum.exPatternEmpty).BackgroundExt := '[text=`<font ;6><fgcolor 808080>just a notice`,align=0x11]';
NonworkingTimes.Add('value = #05/25/2012#','09:00','10:00',1234);
EndUpdate();
end
|
229
|
I am using the control's DataSource property, the question is how can I get the event's identifier Datafield(exEventID)
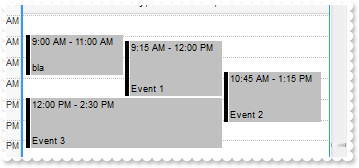
with AxSchedule1 do
begin
rs := (ComObj.CreateComObject(ComObj.ProgIDToClassID('ADOR.Recordset')) as ADODB.Recordset);
with rs do
begin
Open('Events','Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Program Files\Exontrol\ExSchedule\Sample\Access2007\datasource.accdb',3,3,Nil);
end;
BeginUpdate();
Calendar.Selection := '11/11/2013';
set_DataField(EXSCHEDULELib.EventKnownPropertyEnum.exEventStartDateTime,'Start');
set_DataField(EXSCHEDULELib.EventKnownPropertyEnum.exEventEndDateTime,'End');
set_DataField(EXSCHEDULELib.EventKnownPropertyEnum.exEventExtraLabel,'Extra');
DataSource := (rs as ADODB.Recordset);
OutputDebugString( Events.Item[TObject(1)].KnownProperty[EXSCHEDULELib.EventKnownPropertyEnum.exEventID] );
EndUpdate();
end
|
228
|
Is it possible to display some text/image on the schedule's view background
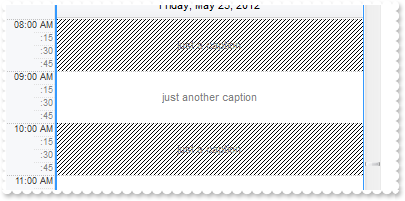
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/25/2012';
NonworkingPatterns.Add(1234,EXSCHEDULELib.PatternEnum.exPatternBDiagonal).BackgroundExt := '[text=`<fgcolor 808080>just a caption`,align=0x11]';
NonworkingPatterns.Add(1235,EXSCHEDULELib.PatternEnum.exPatternEmpty).BackgroundExt := '[text=`<fgcolor 808080>just another caption`,align=0x11]';
NonworkingTimes.Add('weekday(value) = 5','08:00','08:59:59',1234);
NonworkingTimes.Add('weekday(value) = 5','09:00','09:59:59',1235);
NonworkingTimes.Add('weekday(value) = 5','10:00','10:59:59',1234);
EndUpdate();
end
|
227
|
How can I display a text on the schedule's view background
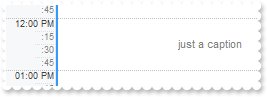
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/25/2012';
NonworkingPatterns.Add(1234,EXSCHEDULELib.PatternEnum.exPatternEmpty).BackgroundExt := '[text=`<fgcolor 808080>just a caption`,align=0x11]';
NonworkingTimes.Add('weekday(value) = 5','12:00','13:00',1234);
EndUpdate();
end
|
226
|
Is it possible to change the visual appearance of the edit's context menu
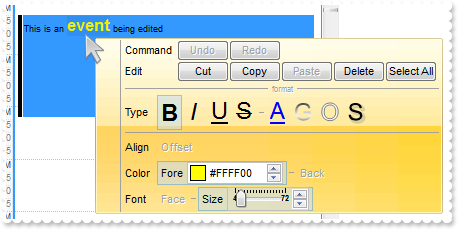
with AxSchedule1 do
begin
VisualAppearance.Add(1,'c:\exontrol\images\normal.ebn');
set_Background(EXSCHEDULELib.BackgroundPartEnum.exContextMenuAppearance,$1000000);
end
|
225
|
How do I customize the edit's context menu
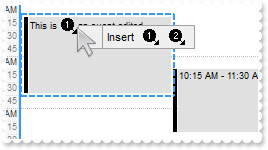
with AxSchedule1 do
begin
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
EditContextMenuItems := 'Insert[group=3](<img>1</img>[id=57763],<img>2</img>[id=57763])';
end
|
224
|
How can I lock/fix the date header, so it stays on the top while the user scrolls the chart
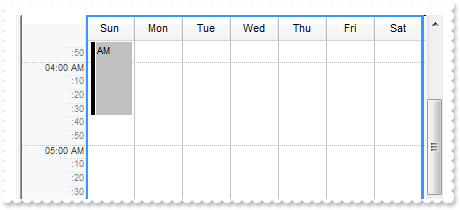
// LayoutEndChanging event - Notifies your application once the control's layout has been changed.
procedure TWinForm1.AxSchedule1_LayoutEndChanging(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_LayoutEndChangingEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( e.operation );
DayViewHeight := 2016;
end
end;
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
SelectDate['5/20/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
end;
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exVertical;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRowLockHeader;
DayViewHeight := 2016;
AllowMoveSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowExchangePanels := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMoveTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMultiDaysEvent := False;
TimeScales.Item[TObject(0)].MinorTimeRuler := '00:10';
DayStartTime := '00:00';
DayEndTime := '24:00';
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
EndUpdate();
end
|
223
|
Is there any way to control the vertical size of each hour other than by the font and/or the minor time scale being changed
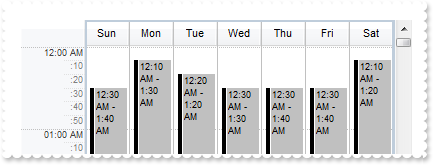
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
SelectDate['5/20/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
end;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exVertical;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRow;
DayViewHeight := 2016;
AllowMoveSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowExchangePanels := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMoveTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMultiDaysEvent := False;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColor,get_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColorUnFocus));
TimeScales.Item[TObject(0)].MinorTimeRuler := '00:10';
DayStartTime := '00:00';
DayEndTime := '24:00';
EndUpdate();
end
|
222
|
How can I show a single week, no calendar
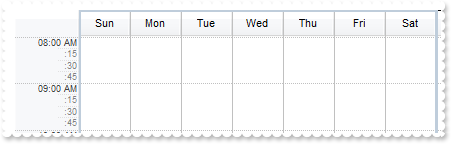
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
SelectDate['5/20/2012'] := True;
Select(EXSCHEDULELib.SelectCalendarDateEnum.exSelectWeek);
end;
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exChangePanels) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exHideSplitter);
ScrollBars := EXSCHEDULELib.ScrollBarsEnum.exNoScroll;
ShowViewCompact := EXSCHEDULELib.ShowViewCompactEnum.exViewSingleRow;
AllowMoveSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowExchangePanels := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMoveTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowResizeTimeScale := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowMultiDaysEvent := False;
set_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColor,get_Background(EXSCHEDULELib.BackgroundPartEnum.exScheduleBorderSelColorUnFocus));
EndUpdate();
end
|
221
|
How do I select the dates within a giving interval only
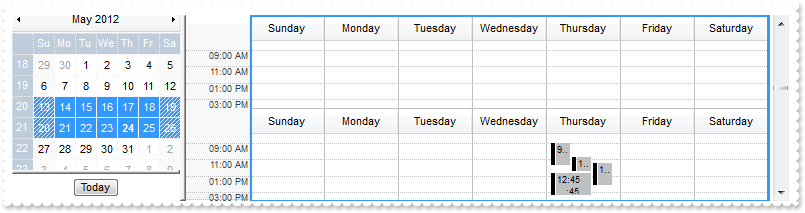
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '5/1/2012';
Selection := '(value >= #5/13/2012#) and (value <= #5/26/2012#)';
end;
with Events do
begin
Add('5/24/2012 9:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:45:00 AM','5/24/2012 12:45:00 PM');
Add('5/24/2012 11:30:00 AM','5/24/2012 2:30:00 PM');
Add('5/24/2012 12:45:00 PM','5/24/2012 3:45:00 PM');
end;
EndUpdate();
end
|
220
|
How do I display the dates within a giving interval only
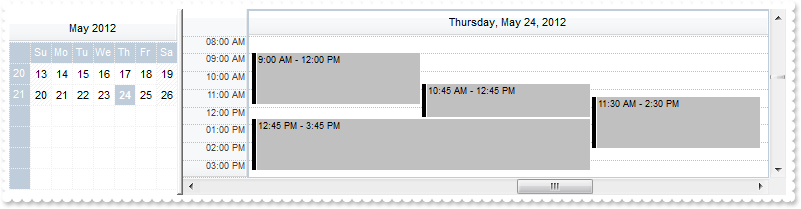
with AxSchedule1 do
begin
BeginUpdate();
with Calendar do
begin
Selection := '5/24/2012';
MinDate := '5/13/2012';
MaxDate := '5/26/2012';
end;
with Events do
begin
Add('5/24/2012 9:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:45:00 AM','5/24/2012 12:45:00 PM');
Add('5/24/2012 11:30:00 AM','5/24/2012 2:30:00 PM');
Add('5/24/2012 12:45:00 PM','5/24/2012 3:45:00 PM');
end;
EndUpdate();
end
|
219
|
Is it possible that the time is displayed starting from 00:00 to 24:00 00 instead of 08:00 AM to 04:00 PM

with AxSchedule1 do
begin
DayStartTime := '00:00';
DayEndTime := '24:00';
with TimeScales.Item[TObject(0)] do
begin
MajorTimeLabel := '<%hh%>:<%nn%>';
Width := 32;
end;
end
|
218
|
How do I show a double frame
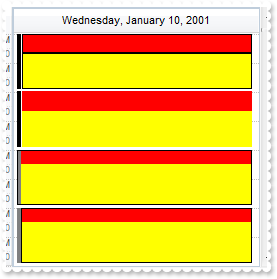
with AxSchedule1 do
begin
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
Calendar.Selection := '1/10/2001';
DefaultEventLongLabel := '';
DefaultEventShortLabel := '';
with Events do
begin
with Add('1/10/2001 8:00:00 AM','1/10/2001 10:00:00 AM') do
begin
BodyBackgroundExt := 'top[25%,back=RGB(255,0,0),align=0x21,pattern=0x000,frame=RGB(0,0,0)],client[back=RGB(255,255,0),align=0x21,pattern=0x000,frame=R' +
'GB(0,0,0)]';
BodyBackgroundExtValue[EXSCHEDULELib.IndexExtEnum.exIndexExt1,EXSCHEDULELib.BackgroundExtPropertyEnum.exBackColorExt] := TObject(255);
BodyBackgroundExtValue[EXSCHEDULELib.IndexExtEnum.exIndexExt1,EXSCHEDULELib.BackgroundExtPropertyEnum.exClientExt] := '35%';
end;
with Add('1/10/2001 10:00:00 AM','1/10/2001 12:00:00 PM') do
begin
BodyBackgroundExt := 'top[25%,back=RGB(255,0,0),align=0x22],client[back=RGB(255,255,0),align=0x22]';
BodyBackgroundExtValue[EXSCHEDULELib.IndexExtEnum.exIndexExt1,EXSCHEDULELib.BackgroundExtPropertyEnum.exClientExt] := '35%';
BodyBackgroundExtValue[EXSCHEDULELib.IndexExtEnum.exIndexExt2,EXSCHEDULELib.BackgroundExtPropertyEnum.exBackColorExt] := TObject(65535);
end;
with Add('1/10/2001 12:00:00 PM','1/10/2001 2:00:00 PM') do
begin
ShowStatus := False;
BodyBackgroundExt := 'left[4,back=RGB(128,128,128)],top[25%,back=RGB(255,0,0)],client[back=RGB(255,255,0)],none[(0%,0%,100%,100%),pattern=0x000,frame=' +
'RGB(0,0,0)]';
end;
with Add('1/10/2001 2:00:00 PM','1/10/2001 4:00:00 PM') do
begin
ShowStatus := False;
BodyBackgroundExt := 'left[4,back=RGB(128,128,128)],top[25%,back=RGB(255,0,0)],client[back=RGB(255,255,0)],none[(4,0%,100%-4,100%),pattern=0x000,frame' +
'=RGB(0,0,0)]';
end;
end;
end
|
217
|
How can I add more colors on the event
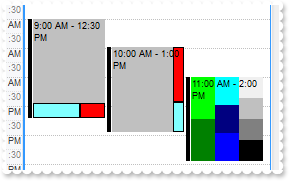
with AxSchedule1 do
begin
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
Calendar.Selection := '1/10/2001';
with Events do
begin
Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').BodyBackgroundExt := 'bottom[15%](left[65%,frame,back=RGB(128,255,255)],client[back=RGB(255,0,0),frame])';
Add('1/10/2001 10:00:00 AM','1/10/2001 1:00:00 PM').BodyBackgroundExt := 'right[15%](bottom[35%,frame,back=RGB(128,255,255)],client[back=RGB(255,0,0),frame])';
Add('1/10/2001 11:00:00 AM','1/10/2001 2:00:00 PM').BodyBackgroundExt := 'left[33%,back=RGB(0,128,0)](top[50%,back=RGB(0,255,0)]),left[33%](top[33%,back=RGB(0,255,255)],top[33%,back=RGB(0,0,128)],client' +
'[back=RGB(0,0,255)]),client(top[25%,back=RGB(240,240,240)],top[25%,back=RGB(192,192,192)],top[25%,back=RGB(128,128,128)],client[' +
'back=RGB(0,0,0)])';
end;
end
|
216
|
When I have 3 month visible in the calendar section, it seems I can only move back and forth through the months 3 months at a time. Is it possible to move back and forth through the months 1 month at a time
with AxSchedule1 do
begin
with Calendar do
begin
AlignDate := False;
MinMonthY := 2;
MaxMonthY := 2;
end;
end
|
215
|
How can I bound the control to a data source
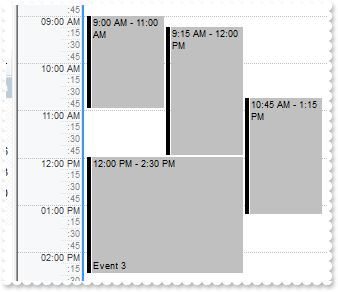
// Error event - Fired when an internal error occurs.
procedure TWinForm1.AxSchedule1_Error(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_ErrorEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( e.description );
end
end;
with AxSchedule1 do
begin
rs := (ComObj.CreateComObject(ComObj.ProgIDToClassID('ADOR.Recordset')) as ADODB.Recordset);
with rs do
begin
Open('Events','Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Program Files\Exontrol\ExSchedule\Sample\Access2007\datasource.accdb',3,3,Nil);
end;
BeginUpdate();
Calendar.Selection := '11/11/2013';
set_DataField(EXSCHEDULELib.EventKnownPropertyEnum.exEventStartDateTime,'Start');
set_DataField(EXSCHEDULELib.EventKnownPropertyEnum.exEventEndDateTime,'End');
set_DataField(EXSCHEDULELib.EventKnownPropertyEnum.exEventExtraLabel,'Extra');
DataSource := (rs as ADODB.Recordset);
EndUpdate();
end
|
214
|
How can I start drag and drop an event
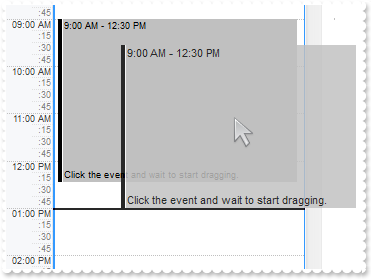
// OLEStartDrag event - Occurs when the OLEDrag method is called.
procedure TWinForm1.AxSchedule1_OLEStartDrag(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_OLEStartDragEvent);
begin
// Data.SetData( "your data to be dragged" )
with AxSchedule1 do
begin
e.allowedEffects := 1;
end
end;
with AxSchedule1 do
begin
BeginUpdate();
OLEDropMode := EXSCHEDULELib.exOLEDropModeEnum.exOLEDropManual;
SelectEventStyle := EXSCHEDULELib.LinesStyleEnum.exLinesSolid;
DefaultEventLongLabel := '<%=%256%><br><%=%5%>';
DefaultEventShortLabel := DefaultEventLongLabel;
Calendar.Selection := '1/10/2001';
OnResizeControl := Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarAutoHide) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exCalendarFit) Or Integer(EXSCHEDULELib.OnResizeControlEnum.exResizePanelRight);
Events.Add('1/10/2001 9:00:00 AM','1/10/2001 12:30:00 PM').ExtraLabel := 'Click the event and wait to start dragging.';
EndUpdate();
end
|
213
|
I use the HighlightDate property to mark a date, instead the selection is not visible if I select the same date. What can be done
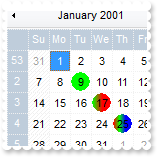
with AxSchedule1 do
begin
ShowHighlightDate := Integer(EXSCHEDULELib.ShowHighlightDateEnum.exHighlightDateCalendarEllipticClip) Or Integer(EXSCHEDULELib.ShowHighlightDateEnum.exHighlightDateCalendarGradient) Or Integer(EXSCHEDULELib.ShowHighlightDateEnum.exShowHighlightDateCalendar);
Calendar.Selection := '1/1/2001';
set_HighlightDate('1/9/2001',TObject(65280));
set_HighlightDate('1/17/2001','65280,255');
set_HighlightDate('1/25/2001','255,65280,16711680');
end
|
212
|
How can I arrange the colors to highlight the date vertically
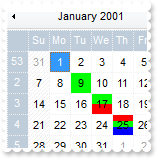
with AxSchedule1 do
begin
ShowHighlightDate := Integer(EXSCHEDULELib.ShowHighlightDateEnum.exHighlightDateVertical) Or Integer(EXSCHEDULELib.ShowHighlightDateEnum.exShowHighlightDateCalendar);
Calendar.Selection := '1/1/2001';
set_HighlightDate('1/9/2001',TObject(65280));
set_HighlightDate('1/17/2001','65280,255');
set_HighlightDate('1/25/2001','255,65280,16711680');
end
|
211
|
Is it possible to highlight a date in gradient
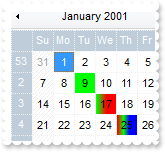
with AxSchedule1 do
begin
ShowHighlightDate := Integer(EXSCHEDULELib.ShowHighlightDateEnum.exHighlightDateCalendarGradient) Or Integer(EXSCHEDULELib.ShowHighlightDateEnum.exShowHighlightDateCalendar);
Calendar.Selection := '1/1/2001';
set_HighlightDate('1/9/2001',TObject(65280));
set_HighlightDate('1/17/2001','65280,255');
set_HighlightDate('1/25/2001','255,65280,16711680');
end
|
210
|
Is it possible to highlight a date in the calendar panel only
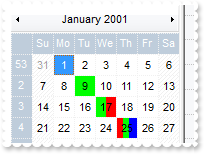
with AxSchedule1 do
begin
ShowHighlightDate := EXSCHEDULELib.ShowHighlightDateEnum.exShowHighlightDateCalendar;
Calendar.Selection := '1/1/2001';
set_HighlightDate('1/9/2001',TObject(65280));
set_HighlightDate('1/17/2001','65280,255');
set_HighlightDate('1/25/2001','255,65280,16711680');
end
|
209
|
Is it possible to highlight a date in the control
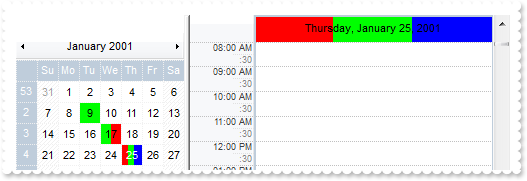
with AxSchedule1 do
begin
ShowHighlightDate := EXSCHEDULELib.ShowHighlightDateEnum.exShowHighlightDate;
Calendar.Selection := '1/25/2001';
set_HighlightDate('1/9/2001',TObject(65280));
set_HighlightDate('1/17/2001','65280,255');
set_HighlightDate('1/25/2001','255,65280,16711680');
end
|
208
|
How we need to put "nonworking time" to each group, because staff A is working different times then staff B. Is this possible
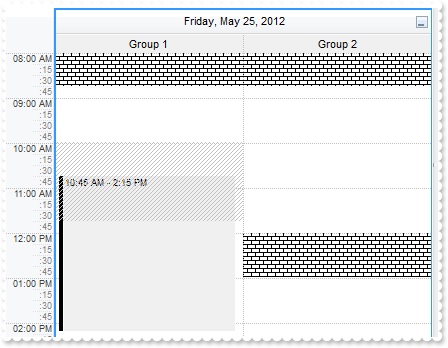
with AxSchedule1 do
begin
BeginUpdate();
BodyEventBackColor := Color.FromArgb(240,240,240);
ShowGroupingEvents := True;
DisplayGroupingButton := True;
Groups.Add(1,'Group 1').Visible := True;
Groups.Add(2,'Group 2').Visible := True;
Calendar.Selection := '5/25/2012';
NonworkingPatterns.Add(1234,EXSCHEDULELib.PatternEnum.exPatternBrick);
with NonworkingTimes do
begin
Add('1','00:00','08:45',1234);
Add('weekday(value) = 5','10:00','11:45',1).GroupID := TObject(1);
Add('weekday(value) = 5','12:00','13:00',1234).GroupID := TObject(2);
end;
Events.Add('5/25/2012 9:30:00 AM','5/25/2012 1:00:00 PM').GroupID := 1;
EndUpdate();
end
|
207
|
I have a double click event set to launch a window so the user can supply input. The problem is that double click changes the view. How do I change this behavior
// DblClick event - Occurs when the user dblclk the left mouse button over an object.
procedure TWinForm1.AxSchedule1_DblClick(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_DblClickEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( 'DblClick ' );
OutputDebugString( e.x );
OutputDebugString( e.y );
end
end;
with AxSchedule1 do
begin
BeginUpdate();
Calendar.Selection := '5/24/2012';
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM').StatusColor := $ff;
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM');
end;
AllowEditEvent := EXSCHEDULELib.AllowKeysEnum.exDisallow;
AllowToggleSchedule := EXSCHEDULELib.AllowKeysEnum.exDisallow;
EndUpdate();
end
|
206
|
I have two-time scales. How do I change the label while updating the events to show the date-time on the second time-scale
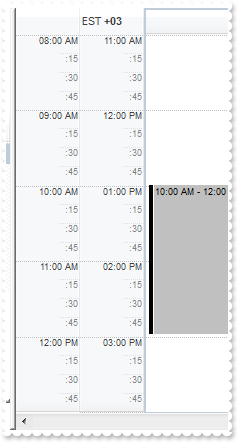
with AxSchedule1 do
begin
DayEndTime := '13:00';
with TimeScales.Add('+3:00') do
begin
AlignLeft := True;
Caption := 'EST <b>+03';
end;
BeginUpdate();
Calendar.Selection := '5/24/2012';
UpdateEventsLabel := 'Start: <%=date(%1+3/24)%><br>End: <%=date(%2+3/24)%>';
with Events do
begin
Add('5/24/2012 10:00:00 AM','5/24/2012 12:00:00 PM');
Add('5/24/2012 10:45:00 AM','5/24/2012 12:30:00 PM');
Add('5/24/2012 11:30:00 AM','5/24/2012 1:30:00 PM');
end;
EndUpdate();
end
|
205
|
Is there a way to add a hyperlink to the event that would run a report with parameters
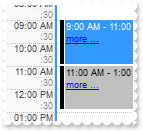
// AnchorClick event - Occurs when an anchor element is clicked.
procedure TWinForm1.AxSchedule1_AnchorClick(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_AnchorClickEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( e.anchorID );
OutputDebugString( e.options );
end
end;
with AxSchedule1 do
begin
Calendar.Selection := '6/20/2012';
with Events do
begin
Add('6/20/2012 9:00:00 AM','6/20/2012 11:00:00 AM').LongLabel := '<%=%256%><br><a 1234;option 1>more ...</a>';
Add('6/20/2012 11:00:00 AM','6/20/2012 1:00:00 PM').LongLabel := '<%=%256%><br><a 1235;option 2>more ...</a>';
end;
end
|
204
|
Can I have multiple months in the calendar section
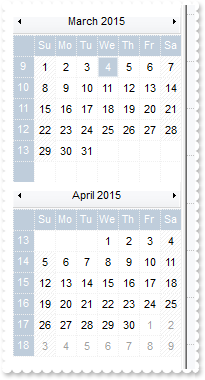
with AxSchedule1 do
begin
with Calendar do
begin
MinMonthY := 2;
MaxMonthY := 2;
end;
end
|
203
|
I need to make sure that at least the order number stays visible when the event is resized. Is there a way during event modification (another event be added at the same time that makes the event size shrink) to adjust the caption location
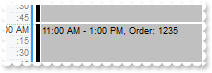
with AxSchedule1 do
begin
Calendar.Selection := '6/20/2012';
with Events do
begin
with Add('6/20/2012 9:00:00 AM','6/20/2012 11:00:00 AM') do
begin
UserData := TObject(1234);
ShortLabel := '<%=%256%><br>Order: <%=%6%>';
LongLabel := ShortLabel;
end;
with Add('6/20/2012 11:00:00 AM','6/20/2012 1:00:00 PM') do
begin
UserData := TObject(1235);
ShortLabel := '<%=%256%>, Order: <%=%6%>';
LongLabel := ShortLabel;
end;
end;
end
|
202
|
I need to make sure that at least the order number stays visible when the event is resized. Is there a way during event modification (another event be added at the same time that makes the event size shrink) to adjust the caption location
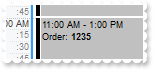
with AxSchedule1 do
begin
Calendar.Selection := '6/20/2012';
DefaultEventShortLabel := '<%=%256%><br>Order: <b><%=%6%>';
DefaultEventLongLabel := DefaultEventShortLabel;
with Events do
begin
Add('6/20/2012 9:00:00 AM','6/20/2012 11:00:00 AM').UserData := TObject(1234);
Add('6/20/2012 11:00:00 AM','6/20/2012 1:00:00 PM').UserData := TObject(1235);
end;
end
|
201
|
Can you add text with links in the event
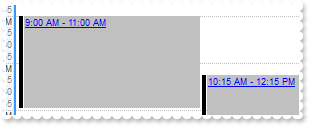
// AnchorClick event - Occurs when an anchor element is clicked.
procedure TWinForm1.AxSchedule1_AnchorClick(sender: System.Object; e: AxEXSCHEDULELib._IScheduleEvents_AnchorClickEvent);
begin
with AxSchedule1 do
begin
OutputDebugString( e.anchorID );
OutputDebugString( e.options );
end
end;
with AxSchedule1 do
begin
Calendar.Selection := '6/20/2012';
with Events do
begin
with Add('6/20/2012 9:00:00 AM','6/20/2012 11:00:00 AM') do
begin
ShortLabel := '<a 1><%=%256%></a>';
LongLabel := ShortLabel;
end;
with Add('6/20/2012 11:00:00 AM','6/20/2012 1:00:00 PM') do
begin
ShortLabel := '<a 2><%=%256%></a>';
LongLabel := ShortLabel;
end;
end;
end
|