325
|
Clear Undo/Redo queue (method 2)
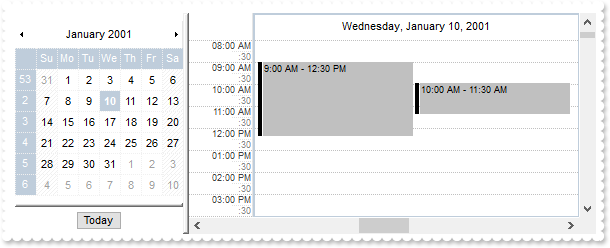
local c,oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
c = oSchedule.UndoRedoQueueLength
oSchedule.UndoRedoQueueLength = 0
oSchedule.UndoRedoQueueLength = c
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
324
|
Clear Undo/Redo queue (method 1)
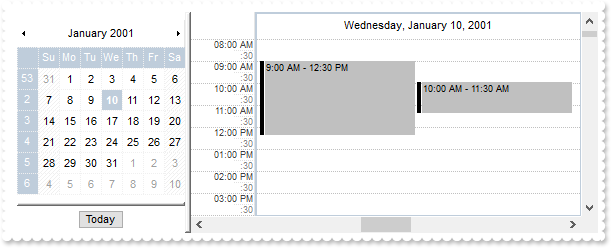
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.AllowUndoRedo = true
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
323
|
Removes Redo operations
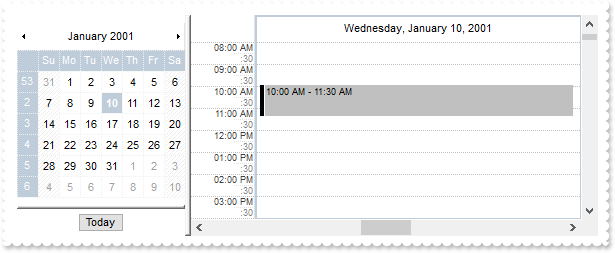
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.Undo()
oSchedule.RedoRemoveAction(13)
? oSchedule.RedoListAction()
oSchedule.EndUpdate()
|
322
|
Removes Undo operations
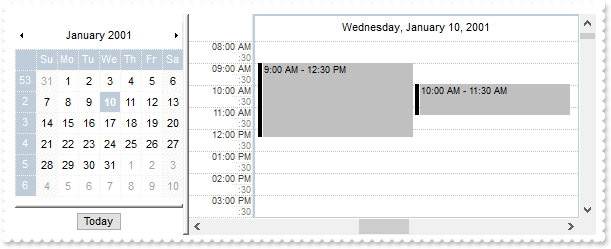
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.UndoRemoveAction(13)
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
321
|
Record the UI operations as a block of undo/redo operations
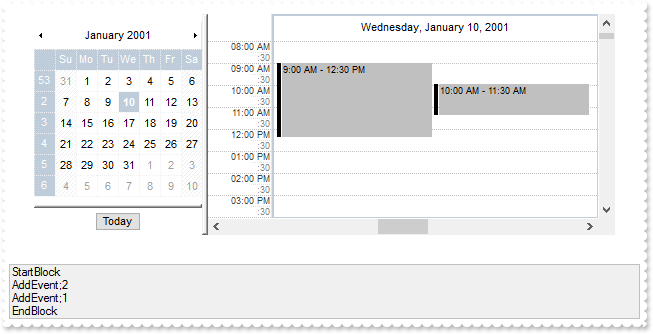
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.StartBlockUndoRedo()
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.EndBlockUndoRedo()
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
320
|
Groups the next to current Undo/Redo Actions in a single block
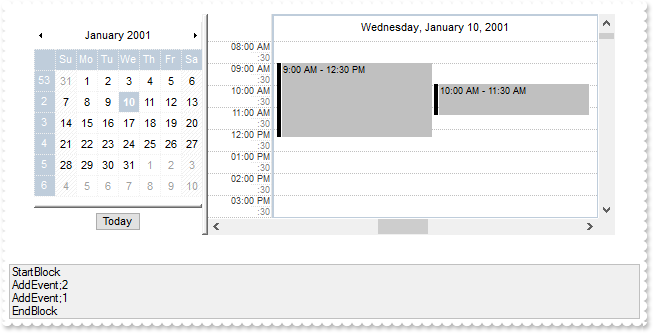
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.GroupUndoRedoActions(2)
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
319
|
Limits the number of entries within the Undo/Redo queue
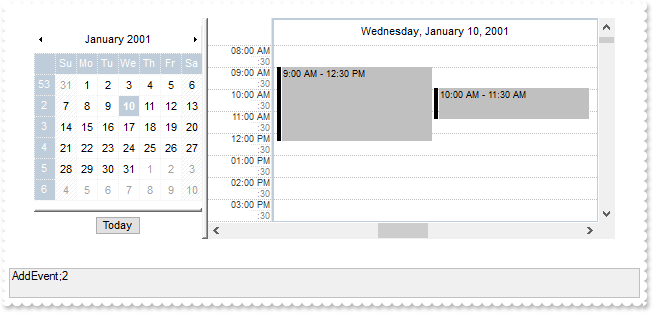
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.UndoRedoQueueLength = 1
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
318
|
Lists the Redo actions that can be performed on the control
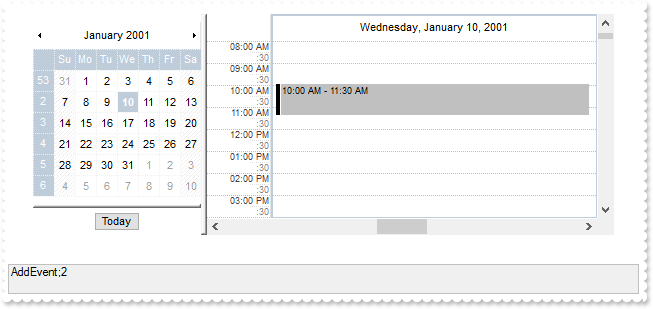
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.Undo()
? oSchedule.RedoListAction()
oSchedule.EndUpdate()
|
317
|
Lists the Undo actions that can be performed on the control
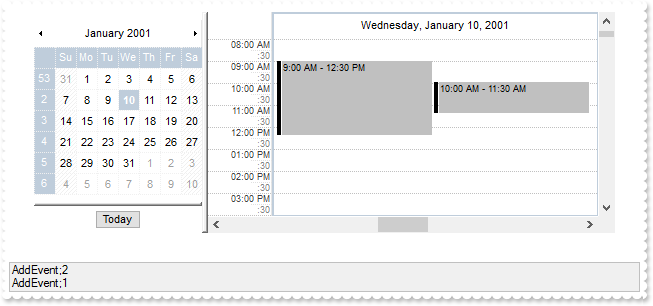
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
? oSchedule.UndoListAction()
oSchedule.EndUpdate()
|
316
|
Checks whether the Undo operation is possible
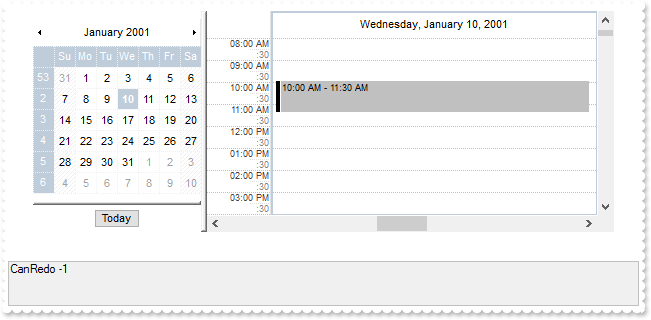
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.Undo()
? "CanRedo"
? Str(oSchedule.CanRedo)
oSchedule.EndUpdate()
|
315
|
Call Redo by code
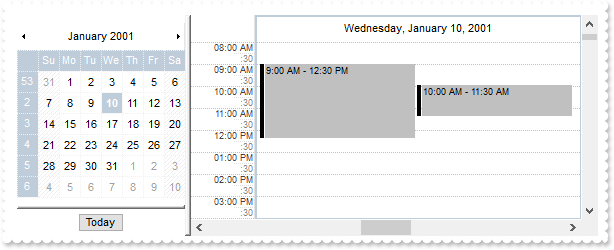
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.Undo()
oSchedule.Redo()
oSchedule.EndUpdate()
|
314
|
Checks whether the Undo operation is possible
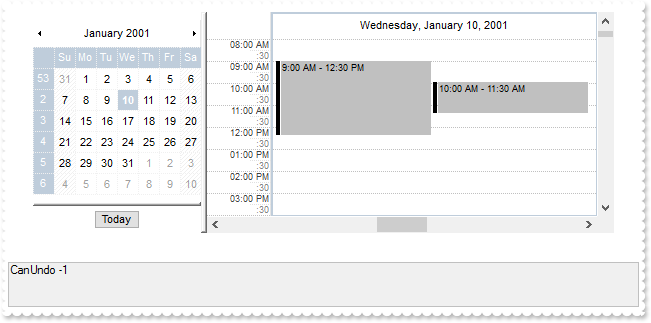
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
? "CanUndo"
? Str(oSchedule.CanUndo)
oSchedule.EndUpdate()
|
313
|
Call Undo by code
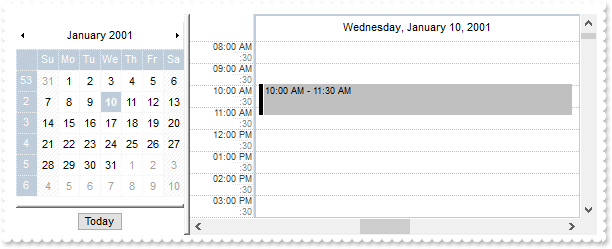
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.Undo()
oSchedule.EndUpdate()
|
312
|
Save the calendar-event's properties for Undo/Redo operations, by code
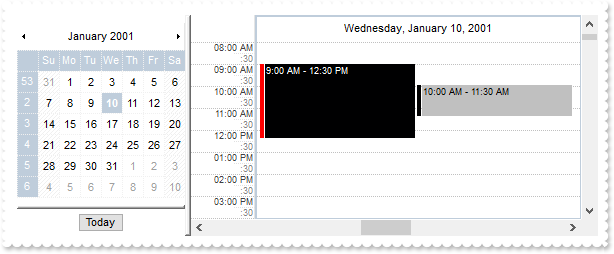
local h,oSchedule,var_Event
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
oSchedule.StartBlockUndoRedo()
var_Event = oSchedule.Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
h = var_Event.StartUpdateEvent
var_Event.BodyBackColor = 0x10000
var_Event.BodyForeColor = 0xffffff
var_Event.StatusColor = 0xff
var_Event.EndUpdateEvent(h)
oSchedule.EndBlockUndoRedo()
oSchedule.EndUpdate()
|
311
|
No color is restored for the calendar-event when Undo/Redo operation is performed
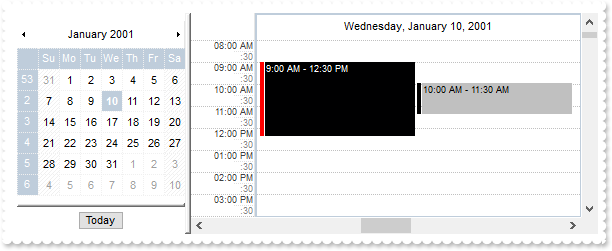
local h,oSchedule,var_Event
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.Events.Add("01/10/2001 10:00:00","01/10/2001 11:30:00")
oSchedule.StartBlockUndoRedo()
var_Event = oSchedule.Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
h = var_Event.StartUpdateEvent
var_Event.BodyBackColor = 0x10000
var_Event.BodyForeColor = 0xffffff
var_Event.StatusColor = 0xff
var_Event.EndUpdateEvent(h)
oSchedule.EndBlockUndoRedo()
oSchedule.EndUpdate()
|
310
|
How can I ensure that a specified calendar-event fits the control's visible area
local oSchedule,var_Events,var_Pattern
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Events = oSchedule.Events
var_Pattern = var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").BodyPattern
var_Pattern.Type = 6
var_Pattern.Color = 0xe0e0e0
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").EnsureVisible()
|
309
|
LayoutEndChanging(exUndo), LayoutEndChanging(exRedo) or LayoutEndChanging(exUndoRedoUpdate) notifiy your application once a Undo/Redo operation is executed (CTRL+Z, CTRL+Y) or updated
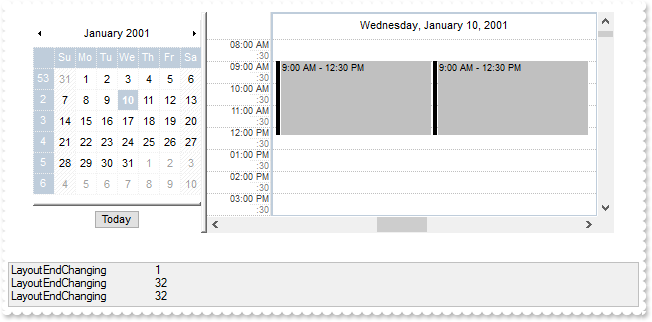
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? "LayoutEndChanging"
? Str(Operation)
return
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.EndUpdate()
|
308
|
Turn on the Undo/Redo feature
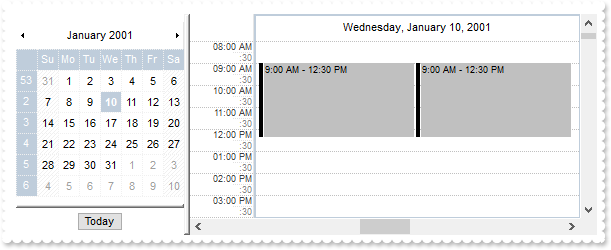
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowUndoRedo = true
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
oSchedule.EndUpdate()
|
307
|
How can I make the header (date/group) always visible, so it stays on the top while the user scrolls the chart
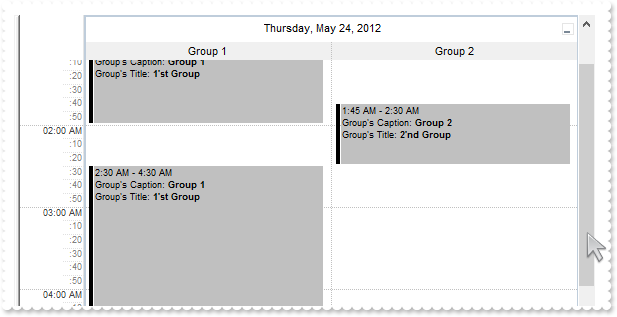
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? Str(Operation)
oSchedule.DayViewHeight = 2016
return
local oSchedule,var_Calendar,var_Event,var_Event1,var_Event2,var_Events,var_Group,var_Group1,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
// var_Calendar.SelectDate("05/20/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/20/2012#) = True]
endwith
var_Calendar.Select(3)
oSchedule.ScrollBars = 2
oSchedule.ShowViewCompact = 3
oSchedule.DayViewHeight = 2016
oSchedule.TimeScales.Item(0).MinorTimeRuler = "00:10"
oSchedule.DayStartTime = "00:00"
oSchedule.DayEndTime = "24:00"
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
oSchedule.ApplyGroupingColors = false
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"Group 1")
var_Group.Visible = true
var_Group.EventBackColor = 0x808080
var_Group.Title = "1'st Group"
var_Group1 = var_Groups.Add(2,"Group 2")
var_Group1.Visible = true
var_Group1.EventBackColor = 0xff
var_Group1.Title = "2'nd Group"
oSchedule.DefaultEventLongLabel = "<%=%256%><br>Group's Caption: <b><%=%262%></b><br>Group's Title: <b><%=%263%></b>"
oSchedule.DefaultEventShortLabel = oSchedule.DefaultEventLongLabel
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 01:00:00","05/24/2012 02:00:00").GroupID = 1
var_Event = var_Events.Add("05/24/2012 01:00:00","05/24/2012 02:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("05/24/2012 01:45:00","05/24/2012 02:30:00").GroupID = 2
var_Event1 = var_Events.Add("05/24/2012 01:45:00","05/24/2012 02:30:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
// var_Events.Add("05/24/2012 02:30:00","05/24/2012 04:30:00").GroupID = 1
var_Event2 = var_Events.Add("05/24/2012 02:30:00","05/24/2012 04:30:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.GroupID = 1]
endwith
oSchedule.EndUpdate()
|
306
|
It appears that Width property of the Group does not what. What am I doing wrong
local oSchedule,var_Event,var_Event1,var_Events,var_Group,var_Group1,var_Group2,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"Group 1")
var_Group.Title = "First"
var_Group.Visible = true
var_Group1 = var_Groups.Add(2,"Group 2")
var_Group1.Title = "Second"
var_Group1.Visible = true
var_Group2 = var_Groups.Add(3,"Group 3")
var_Group2.Title = "Third"
var_Group2.Visible = true
oSchedule.DayViewWidth = 144
oSchedule.Groups.Item(1).Width = 48
oSchedule.Groups.Item(2).Width = 48
var_Events = oSchedule.Events
// var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").GroupID = 1
var_Event = var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00").GroupID = 2
var_Event1 = var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
oSchedule.EndUpdate()
|
305
|
ImageSize property on 32 (specifies the size of control' icons/images/check-boxes/radio-buttons)
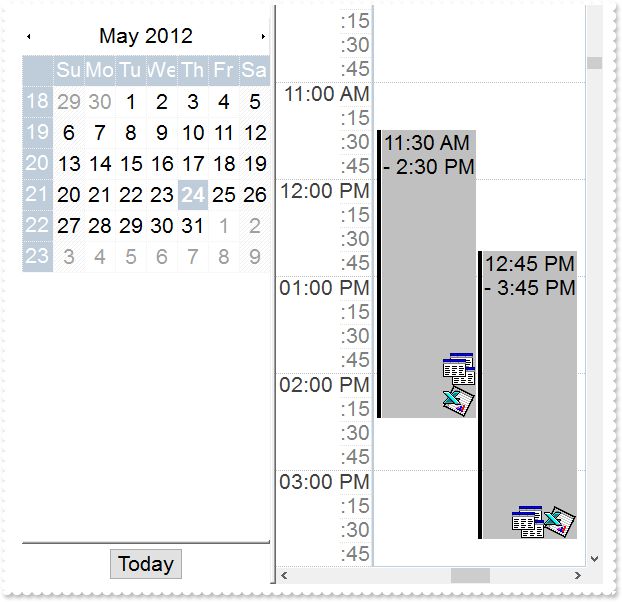
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.ImageSize = 32
oSchedule.Font.Size = 16
oSchedule.EventsFont.Size = 16
oSchedule.TimeScaleFont.Size = 16
oSchedule.TimeScales.Item(0).Width = 128
oSchedule.Images("gBJJgBAIDAAEg4AEEKAD/hz/EMNh8TIRNGwAjEZAEXjAojKAjMLjABhkaABAk0plUrlktl0vmExmUzmk1m03nE5nU7nk9miAoE+oVDolFo1HpFJpU5h8Sf9OqFNqUOqNUqdPq9VrFWrlbr1QpdhAFAkFis1ntFptVrtkrpszrNvmVxqk3uVtm1kmF3sdBvF/wGBmV+j9BYGHwWJulfxdax2NyFdx2JlV6l9Nw7AAGZymdz2Cy2GxErvWcz9ivlwyV21cuxugwktzGIzmvwtl0+53U5y0a0Wazmmyu/3dCyOMyXHx/J5nIr9q3uyqnBxFN3G46ma4vb7mD2Ng4nZze00fDkHC7t7us2rOX5tguetpHRlmz4HVqnXk1PjHO+CMPo9MBMC+j2vC8j7wS8cFNI4kBo05UIvfCT/NsnsApU+0Fqg/T+oy/kPxC0sEQfErKQK96+w28UWRI8UGvO8sTLS9r2PWmsMJTDTask3CsIbIEQRA3shOXEEAO/GclJ9FEKrrA8FRbKMXRIlb0JxCkjS1LMswhCcvuel0cv26cSMa8Ufx+2sQwhEUoSXOCjSbLcnxjKc7sdKUVyq28NtVI71P9P7JxtQEapjQ6fzfM8zPfNE2PhIsLL63E40slk5y7N89LcyU9SvMb3SdUc6VJLj5VLVLfO/PS9KzNFHUa/0XyBD0dxlS9cxhMlTRSoNXypPErWDPyfNS+MwprRNO0FD8wVVZ1AI08URwVRjtJ1WCn21QkkUrXVLVPQS/XIk" ;
+"FgTxT9iONZ9xVTdq+L1eKg3kkF6Upe68XtfV51/MtrVjBlwYFL1ev8y1/P6/lyzzYl02wntj0RVFmS1Qa+M5as93QxEUW9e993rfmQ2+vy65M/mL1lhl/2bj2ByVduMtNhCJT9hdz41nN14Ld12Z9UjfI/oUAaGseiw6+uFLLhcVabJOS5RqOE0BHlZ5VnEr5fOMs3st+aa/bbRzrJGV51Y0b0DbqaWXZD90hIsPbjWu52+6Wyadpe66hhO+P/XioW5rD8ZbrUZuVg6n1dsE/cXmewu1m9PVwnd35/nueXho/NaJzmjc61W76esuT77eG8pTquy9TwWH8LEzG8RDfFalx3Gcfvna9rvG/cptGLd9tuI6TZOP5Fiqi99vea+X4VRcBq/JZZtVQ9cwSs5lsXE372+a9z7PbfB3VVqHyvMctLto8uob6eV0m/cD6MN2v+T33t6sBut42vdv2bJ8a997x2maFJfK+qArbGJPEKE+1qTflMsIdW/GCJX17KcT6/czr/X+u1g29B7j/4BQfWkkx4zIHisjhPCmE0K4SwtXM+d4BvHRwNZOoBph9IJvPek9d40FoMJxf691jj2ywQQcHEWET4XJwkTszlVqm2GokewxtBT1DpQjRxDN0rUVDNKdC3lb6tzNOwh6upMSSYfv4YBCl/bsn9PxiFCEo7SI6Obc9HeOrnY8x4jtHtdpN4GRbaorhsbu18Pph5CiHymI0RpSXGJ/z2oUOxYxG858AyiI+bfJtuTcG5yelBJy" &
+"T8okhqFd4a5yxL0rvulYtKCsZiWxWkc1s1cRoxxwhA31DLE0mR9l9HqX8fJgTDmFMVH0MIsRzVYnwnMi1dyzmhLt2kS2pxIiU62Wj5ptQGlSYFakLonTUJNLKaM5WzlffEkuFkk5wTrhVO2eE7G6lJhxFFYUZ55zmn0WuBCD4pzhirFCKkbomsOoIYmZx5p90LoYWGPdD5g0QmJRKYxbZ6zYoVQ2jVGylSak7KSkFH6RSjpHKFuU+YMyNo5SulkC6I0vonTCitMXPoEpVS2H5FQfEqp2R1opIgAEkJISYARTCukOhmPNI5Ex/wzGHUsicMwA1LHgQ90Y/KpoQHAD+pB/R4NzIaMAB9Xaw1gqaAOsh/A/ptIkWUfhGK1kZH8RgH5GqvgArqRmt4AAPrTroRofBGADkqr6Rmu4D7CEaHARiwpJrEEZsXXwlVjyMWRsaRqwdkLGNBABZmytmyMnaINZqyVpLR2ftKAAAdd6h2osbaskdiq4EZtgSmyNcbVWRJNXe3AA7REar3b0stlAAXBtoRmvJGLjEYAHUWsFcwCD/rnaop9aEICMAPdK5hT6xpeuzdOtAgKuJeGfdq6ggEbkTvAP+p9UCHXrvKkcgIA==")
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 11:30:00","05/24/2012 14:30:00").Pictures = "1/2"
var_Event = var_Events.Add("05/24/2012 11:30:00","05/24/2012 14:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Pictures = "1/2"]
endwith
// var_Events.Add("05/24/2012 12:45:00","05/24/2012 15:45:00").Pictures = "1,2"
var_Event1 = var_Events.Add("05/24/2012 12:45:00","05/24/2012 15:45:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.Pictures = "1,2"]
endwith
oSchedule.EndUpdate()
|
304
|
ImageSize property on 16 (default) (specifies the size of control' icons)
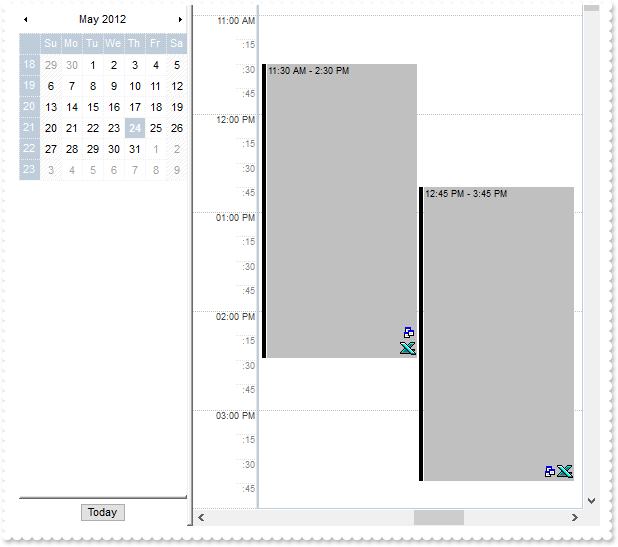
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.ImageSize = 16
oSchedule.Images("gBJJgBAIDAAEg4ACEKAD/hz/EMNh8TIRNGwAjEZAEXjAojJAjMLjABAAgjUYkUnlUrlktl0vmExmUzmk1m03nE5nU7nkrQCAntBoVDolFo1HoM/ADAplLptImdMYFOqdSqlXq1QrVbrlGpVWsFNrNdnNjsk7pQAtNroFnt0sh8Yr9iulTuNxs1Eu8OiT/vsnsNVutXlk/oGGtVKxGLxWNtsZtN8iUYuNvy0Zvd+xNYwdwvl4p870GCqc8vOeuVttmp1knyOayWVy+WzN/ze1wOElenm+12WUz/Bv2/3UyyWrzeutux2GSyGP2dQ33C1ur3GD3M4zUNzHdlWjq/E3nGzVpjWv4HA7fRy/Tv2IrN8rPW6nZ3ve7mUlfu20Z8acvQyb+vY9jasYoDwMm+LytVBDqKG3z8O3Cb8P+mkAuY9cCQ2uL4KaxDKvkp8RNLEjqugnrwQo/UWPzFyeQw5sNLZFENrI4kOqU66pw8uzmOKvTqNqjULJvGL1JO48GtTGsbLdEL3scxLlyiw8dQeoUVxdLTtyKmUjwGlslRPJsnK1HbAKbKCrsQo8uQk/CeP44iaR/ATnTNPLvyxPU+z9P9AUDQVBowiofJXQ6Oo+kKMpIkjztE4TKn4P6JowfgPnwD5/nAjB8AOeAPo0eAA1IAFH07UhAIMpYAVIYFHqBUhwVjV1S1EtQAHxW65V0AZwAeuQAnwB5gAPYViEDVhwAHTQBkCjB4gOhwDmCyhH0sACAg==")
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 11:30:00","05/24/2012 14:30:00").Pictures = "1/2"
var_Event = var_Events.Add("05/24/2012 11:30:00","05/24/2012 14:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Pictures = "1/2"]
endwith
// var_Events.Add("05/24/2012 12:45:00","05/24/2012 15:45:00").Pictures = "1,2"
var_Event1 = var_Events.Add("05/24/2012 12:45:00","05/24/2012 15:45:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.Pictures = "1,2"]
endwith
oSchedule.EndUpdate()
|
303
|
Is it possible to show a different background color for alternate days
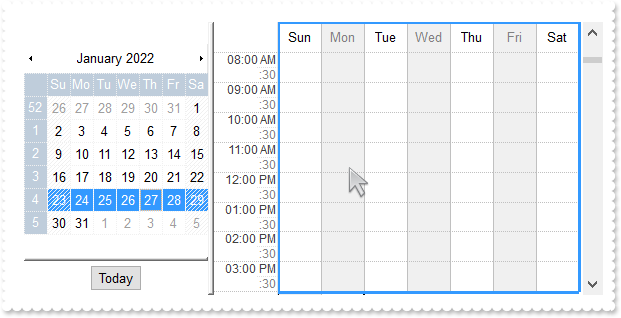
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.Template = [Background(41) = 0] // oSchedule.Background(41) = 0x0
oSchedule.Template = [Background(42) = 0] // oSchedule.Background(42) = 0x0
oSchedule.Template = [Background(159) = 15790320] // oSchedule.Background(159) = 0xf0f0f0
oSchedule.Template = [Background(160) = 8421504] // oSchedule.Background(160) = 0x808080
oSchedule.Calendar.Select(3)
|
302
|
How can I select all events
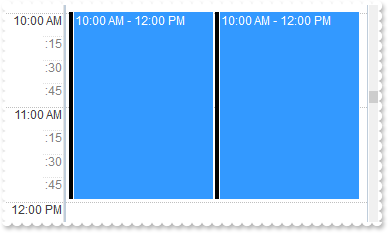
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
oSchedule.SelectAll()
oSchedule.EndUpdate()
|
301
|
How can I unselect all events
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
oSchedule.SelectAll()
oSchedule.Selection = ""
oSchedule.EndUpdate()
|
300
|
How do I immediately select a newly added event
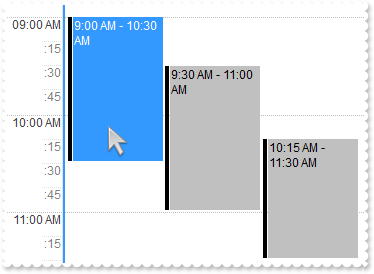
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
AddEvent = class::nativeObject_AddEvent
endwith
*/
// Notifies your application once the a new event is added.
function nativeObject_AddEvent(Ev)
/* Ev.Selected = True */
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
return
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
|
299
|
I would like to know if this allows me to setup a number of room(column). Let's say, i need to go up to 10 rooms is it possible. Also, the possibility to have a complete week of that 10 rooms.
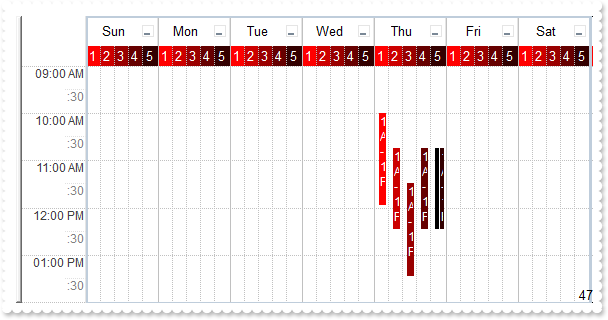
local oSchedule,var_Calendar,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Events,var_Group,var_Group1,var_Group2,var_Group3,var_Group4,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OnResizeControl = 2048
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
oSchedule.BodyEventForeColor = 0xffffff
oSchedule.DayStartTime = "09:00"
oSchedule.DayEndTime = "14:00"
var_Calendar = oSchedule.Calendar
// var_Calendar.SelectDate("05/24/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/24/2012#) = True]
endwith
var_Calendar.Select(3)
oSchedule.ScrollBars = 0
oSchedule.ShowViewCompact = 1
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"1")
var_Group.Visible = true
var_Group.EventBackColor = 0xff
var_Group.HeaderBackColor = var_Group.EventBackColor
var_Group.HeaderForeColor = 0xffffff
var_Group1 = var_Groups.Add(2,"2")
var_Group1.Visible = true
var_Group1.EventBackColor = 0xcc
var_Group1.HeaderBackColor = var_Group1.EventBackColor
var_Group1.HeaderForeColor = 0xffffff
var_Group2 = var_Groups.Add(3,"3")
var_Group2.Visible = true
var_Group2.EventBackColor = 0x99
var_Group2.HeaderBackColor = var_Group2.EventBackColor
var_Group2.HeaderForeColor = 0xffffff
var_Group3 = var_Groups.Add(4,"4")
var_Group3.Visible = true
var_Group3.EventBackColor = 0x66
var_Group3.HeaderBackColor = var_Group3.EventBackColor
var_Group3.HeaderForeColor = 0xffffff
var_Group4 = var_Groups.Add(5,"5")
var_Group4.Visible = true
var_Group4.EventBackColor = 0x33
var_Group4.HeaderBackColor = var_Group4.EventBackColor
var_Group4.HeaderForeColor = 0xffffff
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00").GroupID = 1
var_Event = var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 2
var_Event1 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
// var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00").GroupID = 3
var_Event2 = var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.GroupID = 3]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 4
var_Event3 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.GroupID = 4]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 5
var_Event4 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.GroupID = 5]
endwith
oSchedule.EndUpdate()
|
298
|
I would like to know if this allows me to setup a number of room(column). Let's say, i need to go up to 10 rooms is it possible
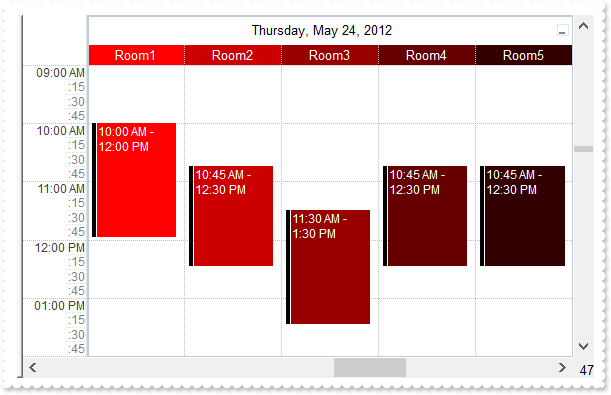
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Events,var_Group,var_Group1,var_Group2,var_Group3,var_Group4,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OnResizeControl = 2048
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
oSchedule.BodyEventForeColor = 0xffffff
oSchedule.DayStartTime = "09:00"
oSchedule.DayEndTime = "14:00"
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"Room1")
var_Group.Visible = true
var_Group.EventBackColor = 0xff
var_Group.HeaderBackColor = var_Group.EventBackColor
var_Group.HeaderForeColor = 0xffffff
var_Group1 = var_Groups.Add(2,"Room2")
var_Group1.Visible = true
var_Group1.EventBackColor = 0xcc
var_Group1.HeaderBackColor = var_Group1.EventBackColor
var_Group1.HeaderForeColor = 0xffffff
var_Group2 = var_Groups.Add(3,"Room3")
var_Group2.Visible = true
var_Group2.EventBackColor = 0x99
var_Group2.HeaderBackColor = var_Group2.EventBackColor
var_Group2.HeaderForeColor = 0xffffff
var_Group3 = var_Groups.Add(4,"Room4")
var_Group3.Visible = true
var_Group3.EventBackColor = 0x66
var_Group3.HeaderBackColor = var_Group3.EventBackColor
var_Group3.HeaderForeColor = 0xffffff
var_Group4 = var_Groups.Add(5,"Room5")
var_Group4.Visible = true
var_Group4.EventBackColor = 0x33
var_Group4.HeaderBackColor = var_Group4.EventBackColor
var_Group4.HeaderForeColor = 0xffffff
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00").GroupID = 1
var_Event = var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 2
var_Event1 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
// var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00").GroupID = 3
var_Event2 = var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.GroupID = 3]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 4
var_Event3 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.GroupID = 4]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 5
var_Event4 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.GroupID = 5]
endwith
oSchedule.EndUpdate()
|
297
|
Please could you let me know if it is possible to change the increment when the user scrolls the mouse wheel as its to slow by default
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.VerticalScrollWheel = 3
|
296
|
I am using the DefaultEventLongLabel property to specify the event's label. Is it possible to change the way the event's label is displayed when it is an all day event (sample 2)
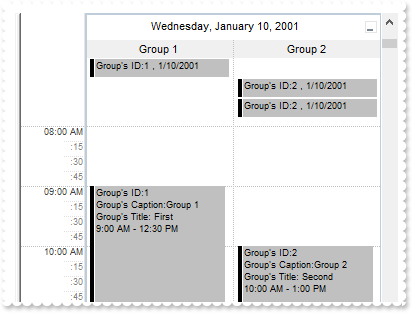
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Events,var_Group,var_Group1,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.SelectEventStyle = 48
oSchedule.AllowAllDayEventScroll = 4416 /*exAllDayEventWheelScroll | exAllDayEventMax4*/
oSchedule.DefaultEventLongLabel = "<%=%><%= ( %3 = 0 ? (`Group's ID:` + %4 + `<br>Group's Caption:` + %262 + `<br>Group's Title: ` + %263 + `<br>` + %256 ) : ( (`Group's ID:` + %4 + ` , ` + %256 ) replace `<br>` with `,` ) ) %>"
oSchedule.DefaultEventShortLabel = "<%=%><%= ( %3 = 0 ? (`Group's ID:` + %4 + `<br>Group's Caption:` + %262 + `<br>Group's Title: ` + %263 + `<br>` + %256 ) : ( (`Group's ID:` + %4 + ` , ` + %256 ) ) replace `<br>` with `\r\n` ) %>"
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
oSchedule.HeaderGroupHeight = 1
oSchedule.ShowAllDayHeader = true
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"Group 1")
var_Group.Title = "First"
var_Group.Visible = true
var_Group1 = var_Groups.Add(2,"Group 2")
var_Group1.Title = "Second"
var_Group1.Visible = true
var_Events = oSchedule.Events
// var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").GroupID = 1
var_Event = var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00").GroupID = 2
var_Event1 = var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
var_Event2 = var_Events.Add("01/10/2001","01/10/2001")
var_Event2.GroupID = 1
var_Event2.AllDayEvent = true
var_Event3 = var_Events.Add("01/10/2001","01/10/2001")
var_Event3.GroupID = 2
var_Event3.AllDayEvent = true
var_Event4 = var_Events.Add("01/10/2001","01/10/2001")
var_Event4.GroupID = 2
var_Event4.AllDayEvent = true
oSchedule.EndUpdate()
|
295
|
I am using the DefaultEventLongLabel property to specify the event's label. Is it possible to change the way the event's label is displayed when it is an all day event (sample 1)
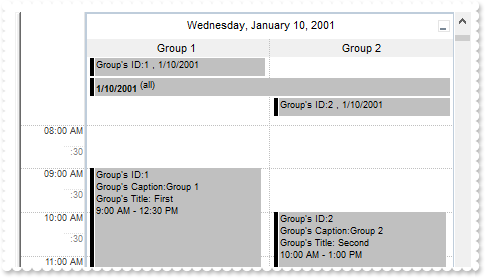
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Events,var_Group,var_Group1,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.SelectEventStyle = 48
oSchedule.DefaultEventLongLabel = "<%=%><%= %4 < 0 ? `<b>` + %256 + `</b> <off -4>(all)` : ( (`Group's ID:` + %4 + `<br>Group's Caption:` + %262 + `<br>Group's Title: ` + %263 + `<br>` + %256 ) replace ( %3 ? `<br>` : ``) with `,` ) %>"
oSchedule.DefaultEventShortLabel = "<%=%><%= %4 < 0 ? %256 : ( %3 = 0 ? (`Group's ID:` + %4 + `<br>Group's Caption:` + %262 + `<br>Group's Title: ` + %263 + `<br>` + %256 ) : ( (`Group's ID:` + %4 + ` , ` + %256 ) ) replace `<br>` with `\r\n` ) %>"
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
oSchedule.HeaderGroupHeight = 1
oSchedule.ShowAllDayHeader = true
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"Group 1")
var_Group.Title = "First"
var_Group.Visible = true
var_Group1 = var_Groups.Add(2,"Group 2")
var_Group1.Title = "Second"
var_Group1.Visible = true
var_Events = oSchedule.Events
// var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").GroupID = 1
var_Event = var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00").GroupID = 2
var_Event1 = var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
var_Event2 = var_Events.Add("01/10/2001","01/10/2001")
var_Event2.GroupID = 1
var_Event2.AllDayEvent = true
var_Event3 = var_Events.Add("01/10/2001","01/10/2001")
var_Event3.GroupID = -1
var_Event3.AllDayEvent = true
var_Event4 = var_Events.Add("01/10/2001","01/10/2001")
var_Event4.GroupID = 2
var_Event4.AllDayEvent = true
oSchedule.EndUpdate()
|
294
|
The Event.Caption does not support HTML, and so if using in DefaultEventLongLabel/DefaultEventShortLabel no HTML is applied, instead HTML tags are displayed as plain text. What can be done
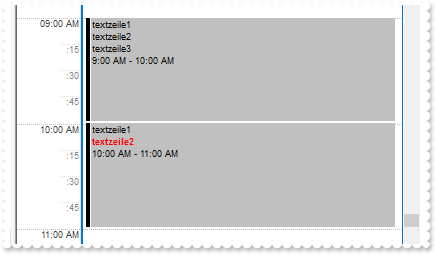
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.DefaultEventLongLabel = "<%=%><%=%5%><br><%=%256%>"
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 09:00:00","05/24/2012 10:00:00").Caption = "textzeile1<br>textzeile2<br>textzeile3"
var_Event = var_Events.Add("05/24/2012 09:00:00","05/24/2012 10:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Caption = "textzeile1<br>textzeile2<br>textzeile3"]
endwith
// var_Events.Add("05/24/2012 10:00:00","05/24/2012 11:00:00").Caption = "textzeile1<br><fgcolor-FF0000><b>textzeile2</b></fgcolor>"
var_Event1 = var_Events.Add("05/24/2012 10:00:00","05/24/2012 11:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.Caption = "textzeile1<br><fgcolor-FF0000><b>textzeile2</b></fgcolor>"]
endwith
oSchedule.EndUpdate()
|
293
|
Please could you let me know how I can remove/hide the time scale/marks from the scheduler. I am creating a month view that only requires a box for the day and no time markers required
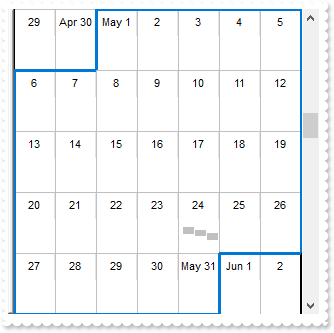
local oSchedule,var_Calendar,var_Event,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.TimeScales.Item(0).Visible = false
oSchedule.OnResizeControl = 2048
oSchedule.ShowViewCompact = -1
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "05/24/2012"
var_Calendar.Select(2)
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").ShowStatus = false
var_Event = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.ShowStatus = False]
endwith
var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
oSchedule.EndUpdate()
|
292
|
Is it possible to lock down the view to allow resizing of the days column but not to allow the scrolling outside of the dictated time zone
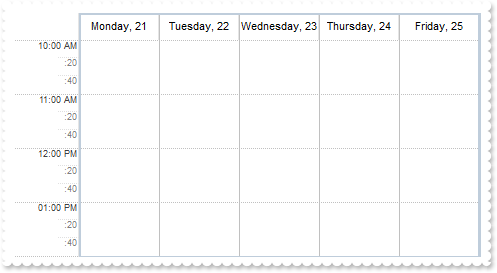
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "05/21/2012"
var_Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
oSchedule.ScrollBars = 2
oSchedule.ShowViewCompact = 1
oSchedule.AllowMoveSchedule = 0
oSchedule.AllowResizeSchedule = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.AllowExchangePanels = 0
oSchedule.AllowMoveTimeScale = 0
oSchedule.AllowResizeTimeScale = 0
oSchedule.AllowMultiDaysEvent = false
oSchedule.Template = [Background(36) = Background(37)] // oSchedule.Background(36) = oSchedule.Background(37)
oSchedule.TimeScales.Item(0).MinorTimeRuler = "00:10"
oSchedule.DayStartTime = "10:00"
oSchedule.DayEndTime = "14:00"
oSchedule.EndUpdate()
|
291
|
I would also like to control the column view to only show 5 days at a time with a side scroll, how would I achieve this please
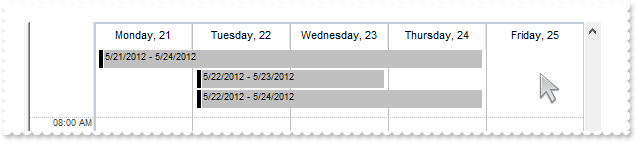
local oSchedule,var_Event,var_Event1,var_Event2,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.ScrollBars = 2
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
290
|
Can I force the schedule grid to only show a single day and then to step through each day using either a custom button click or using the built in schedule calendar
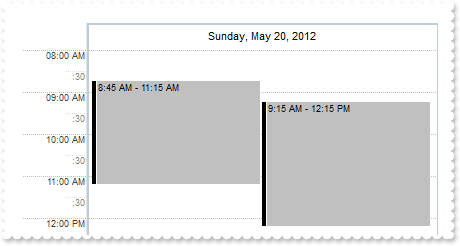
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
// var_Calendar.SelectDate("05/20/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/20/2012#) = True]
endwith
var_Calendar.Select(5)
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
oSchedule.ScrollBars = 0
oSchedule.ShowViewCompact = 1
oSchedule.AllowMoveSchedule = 0
oSchedule.AllowResizeSchedule = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.AllowExchangePanels = 0
oSchedule.AllowMoveTimeScale = 0
oSchedule.AllowResizeTimeScale = 0
oSchedule.AllowMultiDaysEvent = false
oSchedule.Template = [Background(36) = Background(37)] // oSchedule.Background(36) = oSchedule.Background(37)
oSchedule.EndUpdate()
|
289
|
Can I colour the background of the schedulers grid from a time point to another EG 9:00 to 12:00. This is to show users that they can only book appointments in this time zone
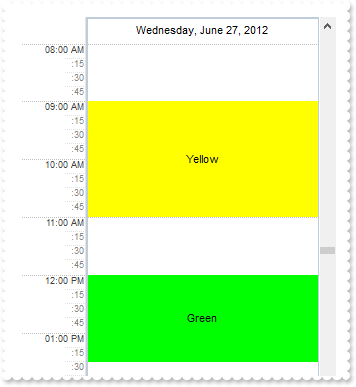
local oSchedule,var_MarkZone,var_MarkZone1
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
oSchedule.Calendar.Selection = "06/27/2012"
var_MarkZone = oSchedule.MarkZones.Add("zoneA","06/27/2012 09:00:00","06/27/2012 11:00:00")
var_MarkZone.Pattern.Type = 0
var_MarkZone.LongLabel = "Yellow"
var_MarkZone.BackColor = 0xffff
var_MarkZone1 = oSchedule.MarkZones.Add("zoneB","06/27/2012 12:00:00","06/27/2012 13:30:00")
var_MarkZone1.Pattern.Type = 0
var_MarkZone1.LongLabel = "Green"
var_MarkZone1.BackColor = 0xff00
oSchedule.EndUpdate()
|
288
|
My programming language has the following format for date 2012-05-24-13.04.06.810000 every other format returns a compiler error. Is there a possibility to use a string for the date
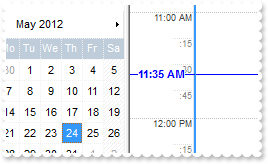
local oSchedule,var_MarkTime,var_MarkTimes
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = oSchedule.ExecuteTemplate("#5/24/2012#")
var_MarkTimes = oSchedule.MarkTimes
var_MarkTime = var_MarkTimes.Add("timer",oSchedule.ExecuteTemplate("#5/24/2012 11:35#"))
var_MarkTime.Movable = true
var_MarkTime.LineColor = 0xff0000
var_MarkTime.StatusEventBackColor = 0xff0000
var_MarkTime.TimeScaleLineColor = 0xff0000
var_MarkTime.TimeScaleLabel = "<fgcolor=0000FF><b><%hh%>:<%nn%> <%AM/PM%>"
oSchedule.EndUpdate()
|
287
|
How do I display a picture with transparency
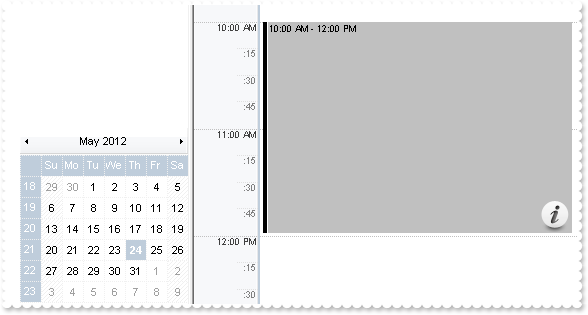
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
PictureClick = class::nativeObject_PictureClick
endwith
*/
// Occurs when the user clicks a picture within an event ( Event.Pictures/ExtraPictures ).
function nativeObject_PictureClick(Key)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? Str(Key)
return
local oSchedule,var_Event,var_Events,var_ExPictures
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.ShowSelectEvent = false
oSchedule.Calendar.Selection = "05/24/2012"
var_ExPictures = oSchedule.Pictures
var_ExPictures.Add("pic1","gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdPyyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QWk4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByEQGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2Cyd" ;
+"hGg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIieRIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpPhyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaGwnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBMGQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxTAmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+As" &
+"fwMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0HgfxniuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQXQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCyEkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJh4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6" &
+"HsXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2DeE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5AgjtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtip" &
+"G0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtAuBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKgFBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZBIAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnBdAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhv" &
+"BPlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfAbAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpBig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/hEhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9hIgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hz" &
+"B7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGgKBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAAChpAkgiAggqAsAEhigrAkgmASAygKB/BVhVB7hTBNg3BNA2hQBOg4BAAAgiBhB1BIA8BMgCAxBxAGAbhBAJgJgHBnAkhyhQBjBhgmAoBBgwgaAPBghJgLgShogYAxAIAZAZAghLBhhQAEgPAEAwgBAwhIgkBBBwhwAYBhhsBoBhhtAbAqArgrh8hehLBVAqA7grgXhPA+Bkglh+h6i4hfh7hehygvgMB9hPhEh9gXh/AKgihUhBh/A1hwA1gwAdh8ASg4AEB+ADh8AAB+AFh8AThHBxg7B4BmhFBZABAABjgbgwh2gBg4A8grArB/3UgDgQBfgzhpBzhpgUhOYogKBlBZB+ARgAA+NxgDhZgWBcgKg9BfgjBRhogngj4zBCg6Af" &
+"ApAzBwB+OiBtA2AbA/hHqYg6ADBZBMBNgfgfAfBfByh4g8BUAiAkgyBMBAg4AcA+BsgyAZBGgRgFA/gbAIh/h6h2AMh/huheg9hbB6h6g6hJhA1zAc5RhhgN5TBLASB3gIXagQAMAGBdAghJhUAvhahohBAiBshzBzBTB4A9AzhTgDh9AOAZBjBfAPAOAeA2ZlBKAJB61xhBE+gmhKATiWhnBvhlhrAcgMghhGg2hBAQA4B85xBWBQBQXiBIAchohkhih/gN36hyBqhiDRgABQgoB0AJAKBvAbAkAxhlBzAVgVAVBJA2gbANg5gdAOAWBrh2gzAW6HBIiagwh9hRhiBTBGAVgAgNB3g5giB/grARBlA6B/gsBhhjaTB2A0AaBQhahah6hWBVBVBlAUB+hNAEh2BVAtkNhSgFBxAAOjBOg2gxBhBTgnAzB9ADABAAh/BohRgjgzBPhrhVgaAaAagihWArSxA0gGhzB3gBhgAdhVPTgOhgA8gShjAsgcgjhUhcBdg+zcBshsgsgtgvAvAPA5hdguBWBUs1LxBbh7gsBahLhzBtjjBpg9AABDgjA6hVBtB0hygt7IAhAz7Jg3AkgkhEtvh5hYhRBhAhBzB6g9AsgwA3hng0AFgNB6hHB7hRBrgxAcBaAGVWhYgehXgjhLgcBvBththxhd7egugB7gBThoAzh57igHA5A3BYgsW8gshMgiAgBZgQh0AKghA3hLsfAGAwAWB0ARgagNBXhWBWAEB+7yBTga7zhpB6" &
+"BqBKBmh0g6AZAxBdhuhnAvWAB6huA0BGhIhGBzuRgcgThpAZAABigYAUhThRhbcEgEBmgpgHgWcHgsAUh+g6B8AsBoA+B6gGgGgxBTB6hVA2h2ACZYAxhSAAhIASB0gngQAphyg0hf3pAJgrAMBwg4BZAYgVAihihKgVhzgMBhg/h9AAAqBRh0BbgTsbgHhwAbhFATANhNBMhMhYgwhlhihRAThT6WBahYh6AzhZgLBAA7h6ALhJgLBZBnhzhthAhAhGB7h7htOfA0hzh4gxhJgBg6hThzBlg4hchyBFc/AsA0hIBihGEnB8BQgvAkgBggAhAmBvBrgngNBGhTgTAThWg1B5gsgAhABRBTgZB+AYAZBxBMBMhPBbJ9BohlhIB/Awh+g/gOAAAuBhhBhUB2gXBSgagKgXgXAXAtA5AXAdBvhCBJBKgrgqBEAHhjBjhO7dBthhBGh7B7BaAdgAzWgxBthXBTACAoAuAuAOBBBxgXgpF2AFhEhtgHgDACg1AfARAiVxAHA4Af9zhc0CB3BOAoATApBWhCp0gJA+hTA7gnAxhWgEhqgEgQB+BzBweFANBGBahVgZB6oehXBwBrAphiAkBCAhhbB0AEBLA3guBfg/AtArgvgXhVGUhfBPhwBlHFBYhcBlh0AxAYgb6phyA0A3g3hPgAAEA9ACgpgqBNAPAPAWBuB1B1BVBUgNBbgvBUhvgPhqgdheBoBxgUAFgiAsAPgN8VhvhxBWg4ANhRBSA+hNB8AwASAjh+B8" &
+"B8AcAMhMg3A0DThhgxhRgGBahxhggBApBEWFBmhS+/BBBhhwgIB4BgAYAJhJgOA2BDARgAhmF6A4gGB8Bo/Jgrg/Bfh/hXBnA8gOAEAWgZAUAIH8ApAshmA8Bjgcg5/Ugehh/WB3gxhNhugiAaA7AcBFgcgRADhSBWAThPBzgyBPALgSAABHABBRhCA72hBpBpApA/4BBrgBhKBFgnBfAZgYA2grgMhWBAgkAChgBBBWhzBiBkh0BghxeEZdALg9iNBvbw7whhhvBbgwgIhygrBfgrBTA6AhgvhjAYg+haBAF4vH4cze70OQyenwMnkQoiQrzCFQA9SUXGy9CMMAuywARTOoQYIFaLgw9RErSKcCginiCw7MHqeDoXjsuW69iWxWKDgAjGAaQQvTiMBuN1ugSmcgaaDsOSyDjA/Tm2FEN2E33AMEwTlUtAACAgAVAM2yhh0O0gVFuJCQHnogCO4QonwkVR4ShoaiOHmq/iCPTwiQmuBmgXGjgqjh2RA2HCUfHoiES4gi7EgcyU/xymE4UwSNCU3EMVA2YikFCiSycVCIBBGR2CdGQUBU9wqWVswAUgRIwGwIlkBFmImECyGcEUL3SESm1HIOFS9kgxRQsQIJCosyAmQg8HIslA+RYOEI6EwtS2cHyiiYJHAlAC0iiAUmEzEiksmHUrWUKBqAoih0OMyAIAAKSQFwnRTAEmS9Mwa36GQOhhCc5BcA8gAcFAfTJCEOSiDEsSaNEuC0EMrjRD8XB4LYziiOIJgN" &
+"ACNAAEkRROEQrhCGAgkBA")
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00").Pictures = "pic1"
var_Event = var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Pictures = "pic1"]
endwith
oSchedule.EndUpdate()
|
286
|
I need a border around each event/item on scheduler. Can you direct me to propery to use (to all)
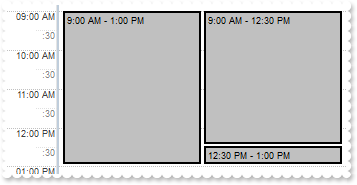
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
AddEvent = class::nativeObject_AddEvent
endwith
*/
// Notifies your application once the a new event is added.
function nativeObject_AddEvent(Ev)
/* Ev.BodyBackgroundExt = "[frame=RGB(0,0,0),framethick]" */
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
return
local oSchedule,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.SelectEventStyle = 48
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
oSchedule.StatusEventSize = 0
oSchedule.Template = [DefaultEventPadding(-1) = 4] // oSchedule.DefaultEventPadding(-1) = 4
var_Events = oSchedule.Events
var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
var_Events.Add("01/10/2001 09:00:00","01/10/2001 13:00:00")
var_Events.Add("01/10/2001 12:30:00","01/10/2001 13:00:00")
|
285
|
I need a border around each event/item on scheduler. Can you direct me to propery to use (distinct)
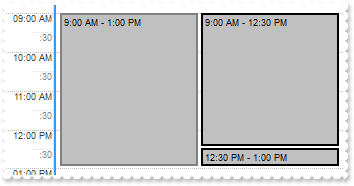
local oSchedule,var_Event,var_Event1,var_Event2,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.SelectEventStyle = 48
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
oSchedule.StatusEventSize = 0
oSchedule.Template = [DefaultEventPadding(-1) = 4] // oSchedule.DefaultEventPadding(-1) = 4
var_Events = oSchedule.Events
// var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").BodyBackgroundExt = "[frame=RGB(0,0,0),framethick]"
var_Event = var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.BodyBackgroundExt = "[frame=RGB(0,0,0),framethick]"]
endwith
// var_Events.Add("01/10/2001 09:00:00","01/10/2001 13:00:00").BodyBackgroundExt = "[frame=RGB(128,128,128),framethick]"
var_Event1 = var_Events.Add("01/10/2001 09:00:00","01/10/2001 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.BodyBackgroundExt = "[frame=RGB(128,128,128),framethick]"]
endwith
// var_Events.Add("01/10/2001 12:30:00","01/10/2001 13:00:00").BodyBackgroundExt = "[frame=RGB(0,0,0),framethick]"
var_Event2 = var_Events.Add("01/10/2001 12:30:00","01/10/2001 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.BodyBackgroundExt = "[frame=RGB(0,0,0),framethick]"]
endwith
|
284
|
How can I change/specify the caption of the groups, when the user clicks the drop down button
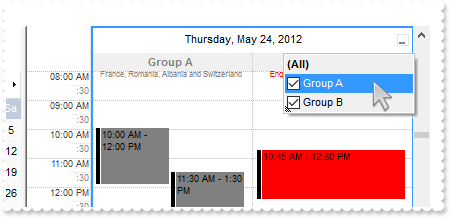
local oSchedule,var_Event,var_Event1,var_Event2,var_Events,var_Group,var_Group1,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"Group A")
var_Group.Caption = "<fgcolor=808080><c><b>Group A</b><c><br><font ;6>France, Romania, Albania and Switzerland"
var_Group.Visible = true
var_Group.EventBackColor = 0x808080
var_Group1 = var_Groups.Add(2,"Group B")
var_Group1.Caption = "<fgcolor=FF0000><c><b>Group B</b><c><br><font ;6>England, Russia, Wales and Slovakia"
var_Group1.Visible = true
var_Group1.EventBackColor = 0xff
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00").GroupID = 1
var_Event = var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 2
var_Event1 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
// var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00").GroupID = 1
var_Event2 = var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.GroupID = 1]
endwith
oSchedule.EndUpdate()
|
283
|
Is it possible to hide the group header, but still display the groups/captions
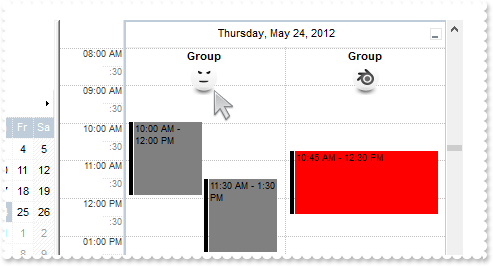
local oSchedule,var_Event,var_Event1,var_Event2,var_Events,var_Group,var_Group1,var_Groups
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Template = [HTMLPicture("pic1") = "gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdPyyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QWk4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByEQGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2CydhGg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIieRIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpPhyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaGwnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBMGQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxTAmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+AsfwMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0HgfxniuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQXQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCyEkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJh4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6HsXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2DeE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5AgjtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtipG0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtAuBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKgFBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZBIAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnBdAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhvBPlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfAbAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpBig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/hEhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9hIgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hzB7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGgKBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAACB5gkgiAggqAsAEhigrglg0ASAqgKBWgng7hSg9gdBPyphph0gQgeoOgyBNBMBIBMBnhjBsgEBJgMACAIgSBhAQAzAwg7BXA6A4BKgUBHB8Agg4BJgIgBA3ASAQAFAIhkhhAEA/ABRgBIhQAFgTgQhFARAEgsgkhngmAMAdhJh6A3gdBUhXBHhzhaB5hFB1HEgNgasJAOB1Bq3OB3Aeh3vth3A9gSgkh/g5hJ01A/hthbB9hQB/gCB4DPgCA9BAAeBYAGh2AAhvgBAvhkBZBOBiB5gsB2gsACBwB8AFBWBegGA6AOLkgvgiBvAABIhvh/AnAvhUhShIBmgzBRhLAyg+gDABB3gBgOhAA6AmAuBDBvA7hTA0hfAagfgfhXhrB5A4gyACAHAHgHhdh+A/B/gTh8AcAvBlhgOnhfBngyAZK2A4BMAlgqBohVAJAUAKAFALgaAmgthBA7AlhigLh/ghBAA0LxBchch8g6A7g7hbh3B+heBBBgB3B3A3ARhtBLgsBfgXBXh5A/g+B/hqBzh5heh0BagohIgBg4B/ApAatAg6BdBBBWBrhMA8AbgGAhhlByg5AvB+gzhFhihxBxghAjgeBlgEByB5h8g5gMA8BQgtBRAGh6B7gjgTZzANArArgrh+hquoAlhSgpB5B6B6A6BYBb57g858hcghAHhbAvhWhMhKAkBeKBguh/hRgNAjAjgjh6h0BshZg7AWALBZhD6KvyAtgtg7husuhJhJh7gYAzAihRhohJBnBtBGgNg9h3A4hogfgcAcA8ApBmBPBnhZAEAwALBngwBtp5gYAZgZhJg9h6BpBSgxA1g1hVga6XgsA2A3g3wIA9AR6pA9ARBIgYBnhjhxgTBRBIB2h3B0gYhuBxhRAHhPgnhciZh1gCAzAEgGBWBdADgPgoA/gXgIAEAuAKBbA+hRBBhRBohSgUAygAgIghgTh+hGAYBrghBQhoA2gNA7AEAQB5hxgmBSBpg0B4AJhChPg5ABAIBUBTgi4jAHBEBEgdAIhAB5hhA0gIggAhB5ACAWgahDgABFBjh3hThKABgJB5B2gzAagBBfhwB4AIh5BFAThbhohjBjBDT3BIgXgSgoBEhtgkAyAHARhkAtBoBrBrAzAqgMBcAXhgBbgkh7hghyAiBQBShSgShEgphFhzh7hIgzhgB2B2A2A4A0g0hUgXAMAGAlA6hNATB8k5gAgMgdBjBWB0BuBOBfA/AegBgBAjh8hTh3BZA9hoBUgBgKBRBvAvgChhhrhuAFBvA/AZBMgqB7B7h7hKh2h2g2gPg1A9hEgkANgOAjhhAwh9gxARAwg8B6heh3gQAdPFgiA/beAZABAd8lgQgKBEgPggBBAzhhBEgbhnAPBwg/hnA2hcBWB5AQBCA8gUBehjhIAfAABMAkB6BbhuhnBsgXgRggAAhhB5j+A/qYgEhbhrkOgqBuAIhVhuhrgyBuAuBLAdolAXBBggAGAyNFAABCgnhRALBUh9gdAFB6AOguhlAYB5hHhIByB3grB9hrAlhAAHBKBChCgCgNB8BdBf9XgngqhqhDgkAAAoAw7ShThwhsBrBoB7hRgvgBByg+AjhBBUgABkgJAuAjAMhEh+gggeAPA/gJg7BOhuh1ATBxBOgIvMAthpgv9wcYg1A1AFBnhmhmguh277ZQhiAGcchkgIgOhcBGhYBXgUBZbABVc5gTB1BEgAhJBUha+BB0h4+Chng4hjgyBygd7agAA1hCAQUPgRg8A7hNAQBQBwhaAFBQh0BCB4g4h1hoeRBhgp+Shnhxi8hhgygagABrAZAWBcBkgtgQA0Ahr4gkhLgvdwB/hzBtBagrgfBfA6h1hnAmguAiA8ANgLACA8AUgagOg3h0d5iBgBBCBjbIhWAGgYANAggsBthwBQAOzogx+yBHr+AGAuA0A0A2AGBPZoBnBAAwAIgnB5hcBmB3BWhWBWB+BdBcBcB8AZhsU6hxBuBgh7g7gwgVgBhuABiEh1giAmgBASAiABBWBkhAgZg0AEA1BRhQgNAwhyAgAMA0hsAxBNg7BsAbBmgQBxAUJZB1hQgMBZAVAPrchOBYgyg8gPg0hYA3gUAzAtBpB6gaB2Qvhhg/gEBoA8gshMg7AlAYgDBsBcAbACAKg6hWCthtqBAAhXgvhDgsA9hDAZAygthLADAVgSAkhhBsANBLAAhZgdBTArhAAQBDgTh6gEBnBNBHAVhrAPBAFkUnmLgChRECkYhG03G4rBi83imysh3uoBMaTqulIkxwIyAHSCmCcCGclnAegWGTOzSSUVurCrHwKymKmBA5EeSAivxCGV8rEopTmNwCOnA+mkIQ6sSeiEQlkKHTkp36CVC7BWPDcJ3SmgBYAASSKTiIriEYEFAQ="] // oSchedule.HTMLPicture("pic1") = "gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdPyyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QWk4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByEQGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2Cyd" ;
+"hGg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIieRIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpPhyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaGwnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBMGQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxTAmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+As" &
+"fwMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0HgfxniuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQXQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCyEkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJh4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6" &
+"HsXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2DeE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5AgjtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtip" &
+"G0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtAuBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKgFBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZBIAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnBdAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhv" &
+"BPlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfAbAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpBig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/hEhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9hIgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hz" &
+"B7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGgKBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAACB5gkgiAggqAsAEhigrglg0ASAqgKBWgng7hSg9gdBPyphph0gQgeoOgyBNBMBIBMBnhjBsgEBJgMACAIgSBhAQAzAwg7BXA6A4BKgUBHB8Agg4BJgIgBA3ASAQAFAIhkhhAEA/ABRgBIhQAFgTgQhFARAEgsgkhngmAMAdhJh6A3gdBUhXBHhzhaB5hFB1HEgNgasJAOB1Bq3OB3Aeh3vth3A9gSgkh/g5hJ01A/hthbB9hQB/gCB4DPgCA9BAAeBYAGh2AAhvgBAvhkBZBOBiB5gsB2gsACBwB8AFBWBegGA6AOLkgvgiBvAABIhvh/AnAvhUhShIBmgzBRhLAyg+gDABB3gBgOhAA6AmAuBDBvA7hTA0hfAagfgfhXhrB5A4" &
+"gyACAHAHgHhdh+A/B/gTh8AcAvBlhgOnhfBngyAZK2A4BMAlgqBohVAJAUAKAFALgaAmgthBA7AlhigLh/ghBAA0LxBchch8g6A7g7hbh3B+heBBBgB3B3A3ARhtBLgsBfgXBXh5A/g+B/hqBzh5heh0BagohIgBg4B/ApAatAg6BdBBBWBrhMA8AbgGAhhlByg5AvB+gzhFhihxBxghAjgeBlgEByB5h8g5gMA8BQgtBRAGh6B7gjgTZzANArArgrh+hquoAlhSgpB5B6B6A6BYBb57g858hcghAHhbAvhWhMhKAkBeKBguh/hRgNAjAjgjh6h0BshZg7AWALBZhD6KvyAtgtg7husuhJhJh7gYAzAihRhohJBnBtBGgNg9h3A4hogfgcAcA8ApBmBPBnhZAEAwALBngwBtp5gYAZgZhJg9h6BpBSgxA1g1hVga6XgsA2A3g3wIA9AR6pA9ARBIgYBnhjhxgTBRBIB2h3B0gYhuBxhRAHhPgnhciZh1gCAzAEgGBWBdADgPgoA/gXgIAEAuAKBbA+hRBBhRBohSgUAygAgIghgTh+hGAYBrghBQhoA2gNA7AEAQB5hxgmBSBpg0B4AJhChPg5ABAIBUBTgi4jAHBEBEgdAIhAB5hhA0gIggAhB5ACAWgahDgABFBjh3hThKABgJB5B2gzAagBBfhwB4AIh5BFAThbhohjBjBDT3BIgXgSgoBEhtgkAyAHARhkAtBoBrBrAzAqgMBcAXhgBbgkh7hghyAiBQ" &
+"BShSgShEgphFhzh7hIgzhgB2B2A2A4A0g0hUgXAMAGAlA6hNATB8k5gAgMgdBjBWB0BuBOBfA/AegBgBAjh8hTh3BZA9hoBUgBgKBRBvAvgChhhrhuAFBvA/AZBMgqB7B7h7hKh2h2g2gPg1A9hEgkANgOAjhhAwh9gxARAwg8B6heh3gQAdPFgiA/beAZABAd8lgQgKBEgPggBBAzhhBEgbhnAPBwg/hnA2hcBWB5AQBCA8gUBehjhIAfAABMAkB6BbhuhnBsgXgRggAAhhB5j+A/qYgEhbhrkOgqBuAIhVhuhrgyBuAuBLAdolAXBBggAGAyNFAABCgnhRALBUh9gdAFB6AOguhlAYB5hHhIByB3grB9hrAlhAAHBKBChCgCgNB8BdBf9XgngqhqhDgkAAAoAw7ShThwhsBrBoB7hRgvgBByg+AjhBBUgABkgJAuAjAMhEh+gggeAPA/gJg7BOhuh1ATBxBOgIvMAthpgv9wcYg1A1AFBnhmhmguh277ZQhiAGcchkgIgOhcBGhYBXgUBZbABVc5gTB1BEgAhJBUha+BB0h4+Chng4hjgyBygd7agAA1hCAQUPgRg8A7hNAQBQBwhaAFBQh0BCB4g4h1hoeRBhgp+Shnhxi8hhgygagABrAZAWBcBkgtgQA0Ahr4gkhLgvdwB/hzBtBagrgfBfA6h1hnAmguAiA8ANgLACA8AUgagOg3h0d5iBgBBCBjbIhWAGgYANAggsBthwBQAOzogx+yBHr+AGAuA0" &
+"A0A2AGBPZoBnBAAwAIgnB5hcBmB3BWhWBWB+BdBcBcB8AZhsU6hxBuBgh7g7gwgVgBhuABiEh1giAmgBASAiABBWBkhAgZg0AEA1BRhQgNAwhyAgAMA0hsAxBNg7BsAbBmgQBxAUJZB1hQgMBZAVAPrchOBYgyg8gPg0hYA3gUAzAtBpB6gaB2Qvhhg/gEBoA8gshMg7AlAYgDBsBcAbACAKg6hWCthtqBAAhXgvhDgsA9hDAZAygthLADAVgSAkhhBsANBLAAhZgdBTArhAAQBDgTh6gEBnBNBHAVhrAPBAFkUnmLgChRECkYhG03G4rBi83imysh3uoBMaTqulIkxwIyAHSCmCcCGclnAegWGTOzSSUVurCrHwKymKmBA5EeSAivxCGV8rEopTmNwCOnA+mkIQ6sSeiEQlkKHTkp36CVC7BWPDcJ3SmgBYAASSKTiIriEYEFAQ="
oSchedule.Template = [HTMLPicture("pic2") = "gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdPyyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QWk4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByEQGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2CydhGg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIieRIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpPhyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaGwnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBMGQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxTAmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+AsfwMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0HgfxniuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQXQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCyEkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJh4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6HsXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2DeE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5AgjtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtipG0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtAuBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKgFBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZBIAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnBdAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhvBPlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfAbAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpBig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/hEhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9hIgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hzB7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGgKBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAADBngkgiAggqAsAEhiiig2AqUgBPA5hnB3Bshug3A9g7BbhthqALPBglN0gCgUoQh4AQAIgAgVgTAhBEAIBYACBIhEB4hggoASBiBKBsBJARRDhwBkgMABBrAQADA+AUAlAmhGBJBaAVALghgSgaA2gpApgpgEgigEBehTBAhLB2g7Bdhdgzhnhih3gvg9AbA9gWhUAHgnB5BPhMgZh5hnh/g/h5hvgzAMB9hPgNh7gnh6h1vtAIA9hVAggVggAbAoWrgAB1ABhGhBg/AAg+hng4huAAB9BjhbATiLhihNgQB2gegoBkgxrEgxAQAQBGgABpAdgJgfgfhpgRBWh8h+tWgmhpgXAABgAzuAYRAhAtAEhfBVhVBVA/BsLNAWAyBpAZhRgChhBwg4BUjtB/LiAehMgCAeBwg+B9hiY9g7BThpgkgXAlA0AMgAgCBBhghshRAUBbBBBthOhYBxgvh/BgANAOgbg3hvAuAvgvAvAXBjhFgkB1hBADgHgPAkATBJh0gWAbBcgzgZA75YBbhUhWBaBqBegxhjgHAEg9hcBMg8BQg6V8h1gegPBXhOBjA0gAgkX2AFhggphNBmBOB+ZSgDBxBdAdAdhihWgagahXBdg7h3gzB6B6h6BvBmhIgkAyA6uihKAGhAggBQAoAIBCBJgwAZBigZhTANhz5+A1hs5/g9A3g3hXhEAgBygXAuhdAyAshaA0AohiX/A1h0h8BThzheAFgLAWAcA9h/B+A8hOh/ApgxAABAh7gIgHB6g7BeBABghggghfBWhWgmgCAYADBrgYAJg9AAhehgB5hGBj6KBbAbA1g/21gEgQBMBs40AHgOAeB0AV6oAphxAyhiBHhghbhuoEB/AgA5gUgAAhBQhoBPhMBHgCgPBpBEAqgqAqBcBIg9guArArALAZgEABBMBqlEAzAohGQ8gAgjACgASQq7hNAvBvhoBwBhhfAmAQBfKYg7gEAgBBAFhQBuBFhcg7g0s6gSMxhhAageBgB4BEAHgjBKBJhOhmh2A7V4jtBGAuAogcA1hLAlAQKehwBFhdBxgshXgrgUgUAUAkBzhHBhAhhDgHA+glAdAJAWAAASh1hQh4A5ghg8g5Bvg7h1hLB0h0BohRhJBVAUAeAshgApgqBSBeBbA4gxhQBSBSASAxB4A7A6A6AsBnBOAfBOgegbAbOoYxAb5uBGgkgHAjhqBvgDAHh64bAOAHAYQPACh4BegTgpgVA68JhMh7h3hvh3hABKg8BqADvRBLhThqh3BvB2hBgICDA5hHAPgdhzA2Bcg4BggHBZhlhdh7h2B4whAoBDgagIA2BbAGAZB5gEgkABAAB+AFh0hMAXgvhfZVhqBrglglASBhAQhkAMBVARg9hUhdA9AVARBlhuBkgJgTgOAe8zBLBwhQgQhp7dhXhtBfTJBJBFBFhFhKhyhlhLhDgUAfBkARB0gyBhBpBrAKAAAwAGATAZhb9FBNhQgeFVgNBGhDgjhchnhzBmBMgJh0h1h1gZgngmgmhigHAAgFhZBthMAhhshygEgCAygFnlB7AoBIAjgbg0BRACgKAihchLgGAwAvguguhHBGBJgzDtB9BzAWgtgBBwAwgiBJhAgAAyhChTAQglg0AHgfASAXhNBTAkArtGhLAAApgtATBlhSA/gAAMASB/AMA9B9HUhPAyBzBzgrhWBIYwhVByBhgjhHB8ArBXgsgJgRAqhEABhrA4hxhDAygXBBBXheBRARAGAwgmBBgoBZBgBVh1hrAmgtAWALBO8sBkh5BnBPgPgkhLAEgqgBhjBihKAoB2goBgklgfBdBfgxgyhcBYhYg4hJBgAMg2hkhJAShwg1hrhXAsgog+A2VGBHhZA6htgbB/hOgdg7hBARhhhhgTBqB9BEAVgbgrh+hTBchsg/AHhCgUgBAlhehcA1AqhkBsA1iOBqPEgJhpBIATA+gfAvgWgnApA3gYASB725he22BXgJh3gCAggiAQhVBWBWBKAog/ByhBBhAhA61gAvggBmAiBVgRLNgYAPhvAqgth1gnhLAAhFmbhcguBugaBPgBAwAyAyBiBHAOB/gGgQAFBJgbgNB/hR/fB+BjhDgHglghhETkgkgIgsgggyhNAuAgBXAgBbBYAygqgqhQhbg7B3BOBSB6h8AjBThDh8gvgbhzCIBoBhB8f0hNgIB7BEhghKBXBThdEpDkheg/B+hdB8Brh2htgzBVBAEADiS0kU52cjV4vDsbRgwy6gA0pl5EQke1MpQKMAa4iWgDwJU68n+MHuAScfxUfj+wi6EFuwHC5Wkhgkuwkc40G2qeE8elEOF8lHCNAeTwsMTwqWOyWeIGQjxIbyQB0AFHgLayJHARwI0iE+HuGQEAAlT0IR0KiDWW2I2EYcUoaE8/giYAEiGAEiijXAEGwpx4HmeAWQNXcBFGTwaQikDlE9Bo41iYTuOA4zjnB2qzk2NzeEy4YkGwlQIikAE8hGM805rEEAmAKmUtQEkHuO9yj2u1GofEONxMVR8SiSulw6EMjEESDOTgGgEQi2MjjU5mI5l4pSWl0s1e+vUCXC+iGQtjEIwOCXi+xqAGowVeoQU+jgeX63GgsHeq1CoTREEpAkL0hgNAA9AoFUQTRBYLzAJgIwAEQIhZAAZjNIEUAXMw+gUBQPCNHE0YFBsKgXC8Xg8DsRySGwhQFAWFQYIE0gAB4pjjFcrhcPk4ziMUiy/AMzQwAIZwUPsxwgGsAg0AUCiCOgkQyhEAhFAAzAgCs6h9BYjAqCkgjiEQDA4BEhhuF01SdLoqjaGARwjIY4APF44QEC4JBwNwhDOHEDwRAYDwVF49QxFk9hBA4KQnPMh4BDw9hIEkKDFFgoBGL4IQiEYGS+OQZBdKYLDAIsSwLMM9xkMo+y4OsRRICEkAEBgqShCkEArOMeBBEwTCiCcPgiDoNQpE48A9P4owtJ0CxoMEsACFkQjIM0zQVCk8hRH4LT4LcoT2FkZAmBMeCyAA8QYIASQJBEnSWIg/BtMw0SpOkUDEBsayZAAEADK0GTrAYICzIsuTMLgkBNH45gnOU8QAEgAT5JcFCSCIGgTBkFiNJkHRzLE9g3CA5QJAk0CGBF7gAywSRFE4RCuEIYCCQE="] // oSchedule.HTMLPicture("pic2") = "gCJKBOI4NBQaBQAhQNJJIIhShQAEEREAIA0ROZ6PT0hQKYZpIZDKBJkIgKByN5mNJsMsKPABVqXBI4KjrD7HL6GWKPJKiCIhMiySidKxbOzZZJWMLsGL2FqyLjZMonNa2CyiZDOUqsQqUEq0ZCNISFXDIFxzZ4hUrbdrefZ/fz3ZgzZ75Tz3XjvHZnZznPieb55AKgAqmRyOOzEhR7XirWaWQQMTa+QIhDbZOZAAoYUCPDAQG7FXI4JRrNCoIRdPyyFr0AYifDUKZ+PCufK4RReALLUbtdBHSrGTCCNKqT4MbRqUxxQx+CAAEQ2VCBbxqGaLYDZNgzFbCbLDarRCrqMYMM6cWqpHKUDqhZjnVijEoLcp0FCjVg2OYhTjN/QWk4bo4iseBsAcABIDoPA5g2HgADIkQfDCNxwkEQYnFmAIAB4OJHGcKAPioGRKFKdh2g6UB8iiZ5QkYQp3gKWhDlsWYmAARBcgCIAUniVpmiSA5AF3A4wG8P41nGWwDDAW4MAAIpSG+bRzBoGx3AeCJhh6C4ljCUJGnSRBUFKAIQA6EgIHMWBoHqYgAngHJDCALBmhCCAfHOARAScUBvAmc5zHYXxoguXQ8DEMIAH8dI8HmP4/AyQJAEAYAoHqRByEQGJiECBAzAkKIpBYNIcikAp8kcZhDn4EBChmUoMgqHIqhiWoIgaDImgyVQImaRw/F0EZGCcSw3DaM4Kn6GBBhwYYZDGZo3C+RgOAmNQnhYeYqgsTZenEVgSFYLo2Cyd" ;
+"hGg4OROF2HJjlydR7i+cJjDGFo8BgHgVl4Po+DufJRgcbQOlkCxyKuCJNAsdwIhSC4mgieYKkeHJWD0Ih8BQaYYkkMYppwTg0EsFhJC0SxEkgeodDSFpTheV5SDgLBIieRIigyVo5CeOpymoWhtEQfRACMR4zE2KxRnsV5dF2ehFCeC50G+GBkBiZgaCUGYnBySY+BsdIuEkJJJDSSRsjGeYqEWOhliYVYOHWDYbFuNhFmcS5siqbZrnGLYOh0DpPhyXo7D8d4ZHGXR1CcdRAnsMh7GELwIHiSx7CiXY0HYNZ1nOcoPg0SB+CWLwwGqUpbFAQJwEeEImlCVQwk4cJxAiFRIhMS4ulGYRRlmMQVDEHZxG8YxXhIaQSniLhIiaGwnDiJZGicZYnjeZw8D6OoSkWEIthwI4emudwtGwepNhuLQ3F8Zojm4bQrhALo0D0HZwCcJwoimeI0ASWR6CAJkJQORfAiFcLIXgahaiGCgMsKIpw8DPH8H4Pj2BhjrBMGQGYfxFjuEGIsB4rxbg+DSFsPAxBtChHoAQaYmRojVG0D0e6JALjVD2K0F4qxfjjGyPIRY/QXi1AOAILwFQGgOF8KYDwOgdBsHmCYcobRtjIHoGgZAmBgi7HgPcWoHxTAmCQCcVwTgDB+FYJgfQMAOj0F2PoZgkRMjeKQLkWATwdDzEkPMF4FxzAXDGJYfAlgPAuB+FkeIWxuizC0LkUwvQbD9ByHIDouxvBCBgCMCAvh4CXCMEgSA0BJDEH+As" &
+"fwMgfjhDeL0Ro/xkgvH4JMXA7RYjyAONgPAWhfjyCuBEcAFRSAWE4BIOwEAUgTCaIYfA4wSBUAcAsDowQOBFA4J0Hg9h2B4EmCQTYVBdB0FwIwU4rByjJGmHIRQ8gJAKB4IoZgShaDKAQOUIolQkjVBuGoSw6hugaFaJoeoWgajaDKDoO4dB5j0FcJ0Zw1Ang3CQDEdgNQnA6EmHgGw4QuCiCSAKFIXBgilEwGcLAZAtDmC0N0WgLhaApFiK0HgfxniuGKP4GIvhrhhGgHEZgaRtB5GSBUcIhg5BnHkOAeQFB5A6DiEEao2xoDHH0KIQ4bxYBfFEP8RogB5BfA8AQHwvwqAZBIBURgCgwgPAqAkKYCgfgTHCBwDIegcgjFUDQXQPQzA4DsCkDwnRABNAwE8OgTw5C6AkJEPgPRSg+DqCANoMRBjuHUKEJglQWDrHYOATg3BuDGDWEMa4CQbj3HMB0Z4Pw5jLFuCAWYsB/D2DgBEUQmB1iuDEMkfI0hUCyEkPIfwihKgqGsGobIGhNhfFGGoZY6gDDuGWDceANA1A2DyDUM4txaA/EwG0bo0wTDXEcH8Sg/BcD2GSHQC4pgtiuGOOkNIRg3hbG+MIGYjhzgaBeHwL4FgHAMAYFIfgJh4nJBQGkfAwRNiFAiO4KIlhoiKFiOoO4EwPiYGONUE4RATg6BOMcUwEApgZGmP4X4lxnjYGaLIZg7RNirH6FQG47xZCAC6OQLK5B1BYF8LgOQZAqh0FqGcBo/xMhpE6" &
+"HsXomQwBKCwIcfA6w/DxA+IURAIxwgmBSCMKoJgOhFD0JMeIkQdhREwFAEQKRFioAYKkJIqQlhpBYFEPYUQui0GCGgFI9BlCOAUDoS4nRhA7HOOkFYdguhgEgGYUgZB2DeE6IIYIMQEgyAiPYHgYgnBlFiNsPYghKiODqISfddhPgVEOCQE4hg5iWHWPEfwfB3BgFYPkAIWQPAOC8BIb1MwrD+QsNEQ43ACAMAKGUQgsBhBoHCGUSNrxBBoEqNgGo8QMAJF+MMOwRxGCOFUBwHYdhODvDwMEBILgk21AKKkOI6RrgyD6LIDoJxNjkDUOQF4yAXgoC4FMXgqhKCiE4KACQow9D5CoJgLQiA9CwEMLUYwOxmhAFaEAdoSwdBBF0CEPQEWWDrGOBoEgGhTAaDyBsPoNgXA4CmHIWw+guCDCSJsNIjxsgADcNQPg2hxC2FKLACo2hNDFEMMcKw/BeCcE6LYXoGRvDJGCN8GIxgUjYAyOUbg6BpDrB0OYWw5AgjtGSOoEofAgjRG2NgY4+RRCfBeKUN4qQvi7H+HAYAchwCOCWAcQQZBBFiG4EIUYww3CFFuEQSgRAlBdDMIwCQiRrCMGCMcIwxhuiKDeE0PAlxCC8GFZQS4YhIgaEkJYS43hLAxE8EkTodQUBbBWMcHoNxy0lHqOETYyAeDeD4I0AQlRhD0G2E0O4PgKCjHeEoSgmBKCGEsBUS4vxUA8C6M0K4ox7irD+O0VouhfD7FUA33I+gmiXE0IsLY1gtip" &
+"G0CQbQLQPjFDuDQHw2RtjFGsK0bw4x9j0CcO8N4/RtBnhSgvAcBehmAOh0ANgiAhAnhih8gwh1gbhugRhSBRhDhjh2Bvgyhfh3gPhThOBIBOA6B9gsAYAah+BdhlBWBtAuBoBThtB8gnhFheAlhcByh6BKhvAahNBnh5B1gJB1g+hCAsgAAbB1gOguAJhIAoAmhFBvqzACABh0BlgFggA6CaBvBQA7BDEHAaA0AABoAcgGBEACg5AAgYgZgLAIBKgFBBhWh9AggCAIBoBNgAANA9AJhwABBxBwAKAYAAALCJu9ADAYAFBLExBEAiBEgmBEgxBEANBENbhmgJh5gJBNgJgzgJBfgRAvAhpKhnAQg5AIpkARASA/ASKGAPBJhZBIAdBJAbARh7n4BIhshkAnAZDVgkBZAUg5AWh5AVB5AEgFAbBFA4BFACglA5hlAfAVAChVAtBVAig1AQh1ABBNB+gaAcgUA7AqAbAWgTg2gfB2gSB9AIBdA1BDh2BHAnBdAZg6Apgdh+h0g7lCBoAXh3BJBugahkBwBihkBkAsBYgtg/h7gNATBNgkhIgUhBg0gzI6BZgJRJglhvAvARgrAtBrALBbBFh2BxB2BZh9hFAcgOAcAdAcgCgcBzhcAVB7h9g5BlgxhohsArgDh5A8heA8BKh8hMB8gzB8APgPBmAdByAShQAVgUAWMMAaAThuATgpAWhNASgLARB3ASAwg+AsEwhiAoimBTBxhUAJhEAJhVhJBPhSBTBSBjgyhv" &
+"BPlWAbgUgfhRhYBUAkAoBTAoBQgrgygfyhgTBShXBSgwhUh0hWgKhTguhQBphRAdhWAjhoBvg1gQA0g0A1AKACAehLgegzgrgmhcAmBahmB+A4AihzAhhLA6ArAFBrAfAbAyhbAPh2hYB7BzB8AOBDgwhTg+hnALAXB8hXBph3AxAPA/BPA2gLg7A8hxg+AlAXANB9ARB+A6B+h5gBgEBAg9BLhFBBAUghAWAhANhhhsgRgBARBvgjAUgiALhjBpBig8hjhHgSA1ASBqgvA4gkhzAmgkglhRgnB7hlh8BKA0hNgxhMBtg7guh5gjAzhPAtB/BJgBBmhhBvAdhDAighg7g2glAzBlg+AVhUAVAphVAHhqURAGhvh7g0AgAahvA2FigMg2BhhaBrg6AMAegTA6AVi5B6BlgehNgMgoA9gigMAZA/hBgMgGg+hfgbAvAegSgbApgegXhZhqBagzBYgogfgwB/Cwg7hgh/hDA/gTg5B+gNh/gXh6B8hbh8B/hEhfASg2h/BHh6BfhZBbhuAjB/g9h7BbBth0h/gbh2Ayh/hAh+h/gnh/Ajh3AwgnA/gigPBzBPBVBegigfA1h8BPhshr1HgNhvAxB/hoBdgGgBhZh3sUhMh0gmh1hLg9hIgchQB7BthugQh5hbgugth6BTgLi/ALBkocoPgCA/BQBfhmh+hXhzA/hzh+g7B1hbgch/heh4gvhEg3hsBfBOgbA2B9ArhrANhPTSKXAMg7A+Bhh4AfgZh/AVhdg/hz" &
+"B7BTBsgfh5B+gdhZh/g7oQBogSh/BMrPhUhYBshvheBfA9AThph7ANhvhNgog/hZBNg/hdhvgIAxhjB2hHBhhOBtg1gPBCg3hZBDAmhDg0gfhNhAg8xMhgBiBvkig7BGgKBHBDBfBJhDBWhPg6BPxKAvBOBUhPB5hMhLhAh0mXBXAFhhB/A3hXBIhwB9AAgUBKg4AHA8AMgdgDhuB8hGghhcACgUAAgOA4AAA8AQABh2BQAegHA2BOB9BYhxhrBAA/hfg7hah4BCBrAxgABkAdAcK4BtgsAshdhZCSAVglgFgbASgeB3AQAPhNhIFJ2TBnhahchDBBh9gQBogABSAlhhBUgbBLANBvA+hbh+gAgWBzB5BXBWhFh/knAAADBngkgiAggqAsAEhiiig2AqUgBPA5hnB3Bshug3A9g7BbhthqALPBglN0gCgUoQh4AQAIgAgVgTAhBEAIBYACBIhEB4hggoASBiBKBsBJARRDhwBkgMABBrAQADA+AUAlAmhGBJBaAVALghgSgaA2gpApgpgEgigEBehTBAhLB2g7Bdhdgzhnhih3gvg9AbA9gWhUAHgnB5BPhMgZh5hnh/g/h5hvgzAMB9hPgNh7gnh6h1vtAIA9hVAggVggAbAoWrgAB1ABhGhBg/AAg+hng4huAAB9BjhbATiLhihNgQB2gegoBkgxrEgxAQAQBGgABpAdgJgfgfhpgRBWh8h+tWgmhpgXAABgAzuAYRAhAtAEhfBVhVBVA/BsLNAWAyBpAZ" &
+"hRgChhBwg4BUjtB/LiAehMgCAeBwg+B9hiY9g7BThpgkgXAlA0AMgAgCBBhghshRAUBbBBBthOhYBxgvh/BgANAOgbg3hvAuAvgvAvAXBjhFgkB1hBADgHgPAkATBJh0gWAbBcgzgZA75YBbhUhWBaBqBegxhjgHAEg9hcBMg8BQg6V8h1gegPBXhOBjA0gAgkX2AFhggphNBmBOB+ZSgDBxBdAdAdhihWgagahXBdg7h3gzB6B6h6BvBmhIgkAyA6uihKAGhAggBQAoAIBCBJgwAZBigZhTANhz5+A1hs5/g9A3g3hXhEAgBygXAuhdAyAshaA0AohiX/A1h0h8BThzheAFgLAWAcA9h/B+A8hOh/ApgxAABAh7gIgHB6g7BeBABghggghfBWhWgmgCAYADBrgYAJg9AAhehgB5hGBj6KBbAbA1g/21gEgQBMBs40AHgOAeB0AV6oAphxAyhiBHhghbhuoEB/AgA5gUgAAhBQhoBPhMBHgCgPBpBEAqgqAqBcBIg9guArArALAZgEABBMBqlEAzAohGQ8gAgjACgASQq7hNAvBvhoBwBhhfAmAQBfKYg7gEAgBBAFhQBuBFhcg7g0s6gSMxhhAageBgB4BEAHgjBKBJhOhmh2A7V4jtBGAuAogcA1hLAlAQKehwBFhdBxgshXgrgUgUAUAkBzhHBhAhhDgHA+glAdAJAWAAASh1hQh4A5ghg8g5Bvg7h1hLB0h0BohRhJBVAUAeAshgApgqBSBeBbA4gxhQ" &
+"BSBSASAxB4A7A6A6AsBnBOAfBOgegbAbOoYxAb5uBGgkgHAjhqBvgDAHh64bAOAHAYQPACh4BegTgpgVA68JhMh7h3hvh3hABKg8BqADvRBLhThqh3BvB2hBgICDA5hHAPgdhzA2Bcg4BggHBZhlhdh7h2B4whAoBDgagIA2BbAGAZB5gEgkABAAB+AFh0hMAXgvhfZVhqBrglglASBhAQhkAMBVARg9hUhdA9AVARBlhuBkgJgTgOAe8zBLBwhQgQhp7dhXhtBfTJBJBFBFhFhKhyhlhLhDgUAfBkARB0gyBhBpBrAKAAAwAGATAZhb9FBNhQgeFVgNBGhDgjhchnhzBmBMgJh0h1h1gZgngmgmhigHAAgFhZBthMAhhshygEgCAygFnlB7AoBIAjgbg0BRACgKAihchLgGAwAvguguhHBGBJgzDtB9BzAWgtgBBwAwgiBJhAgAAyhChTAQglg0AHgfASAXhNBTAkArtGhLAAApgtATBlhSA/gAAMASB/AMA9B9HUhPAyBzBzgrhWBIYwhVByBhgjhHB8ArBXgsgJgRAqhEABhrA4hxhDAygXBBBXheBRARAGAwgmBBgoBZBgBVh1hrAmgtAWALBO8sBkh5BnBPgPgkhLAEgqgBhjBihKAoB2goBgklgfBdBfgxgyhcBYhYg4hJBgAMg2hkhJAShwg1hrhXAsgog+A2VGBHhZA6htgbB/hOgdg7hBARhhhhgTBqB9BEAVgbgrh+hTBchsg/AHhCgUgBAlhe" &
+"hcA1AqhkBsA1iOBqPEgJhpBIATA+gfAvgWgnApA3gYASB725he22BXgJh3gCAggiAQhVBWBWBKAog/ByhBBhAhA61gAvggBmAiBVgRLNgYAPhvAqgth1gnhLAAhFmbhcguBugaBPgBAwAyAyBiBHAOB/gGgQAFBJgbgNB/hR/fB+BjhDgHglghhETkgkgIgsgggyhNAuAgBXAgBbBYAygqgqhQhbg7B3BOBSB6h8AjBThDh8gvgbhzCIBoBhB8f0hNgIB7BEhghKBXBThdEpDkheg/B+hdB8Brh2htgzBVBAEADiS0kU52cjV4vDsbRgwy6gA0pl5EQke1MpQKMAa4iWgDwJU68n+MHuAScfxUfj+wi6EFuwHC5Wkhgkuwkc40G2qeE8elEOF8lHCNAeTwsMTwqWOyWeIGQjxIbyQB0AFHgLayJHARwI0iE+HuGQEAAlT0IR0KiDWW2I2EYcUoaE8/giYAEiGAEiijXAEGwpx4HmeAWQNXcBFGTwaQikDlE9Bo41iYTuOA4zjnB2qzk2NzeEy4YkGwlQIikAE8hGM805rEEAmAKmUtQEkHuO9yj2u1GofEONxMVR8SiSulw6EMjEESDOTgGgEQi2MjjU5mI5l4pSWl0s1e+vUCXC+iGQtjEIwOCXi+xqAGowVeoQU+jgeX63GgsHeq1CoTREEpAkL0hgNAA9AoFUQTRBYLzAJgIwAEQIhZAAZjNIEUAXMw+gUBQPCNHE0YFBsKgXC8Xg8DsRySGwhQFAWFQY" &
+"IE0gAB4pjjFcrhcPk4ziMUiy/AMzQwAIZwUPsxwgGsAg0AUCiCOgkQyhEAhFAAzAgCs6h9BYjAqCkgjiEQDA4BEhhuF01SdLoqjaGARwjIY4APF44QEC4JBwNwhDOHEDwRAYDwVF49QxFk9hBA4KQnPMh4BDw9hIEkKDFFgoBGL4IQiEYGS+OQZBdKYLDAIsSwLMM9xkMo+y4OsRRICEkAEBgqShCkEArOMeBBEwTCiCcPgiDoNQpE48A9P4owtJ0CxoMEsACFkQjIM0zQVCk8hRH4LT4LcoT2FkZAmBMeCyAA8QYIASQJBEnSWIg/BtMw0SpOkUDEBsayZAAEADK0GTrAYICzIsuTMLgkBNH45gnOU8QAEgAT5JcFCSCIGgTBkFiNJkHRzLE9g3CA5QJAk0CGBF7gAywSRFE4RCuEIYCCQE="
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.DisplayGroupingButton = true
oSchedule.ShowGroupingEvents = true
var_Groups = oSchedule.Groups
var_Group = var_Groups.Add(1,"<c><b>Group</b><br><c><img>pic1</img>")
var_Group.Visible = true
var_Group.EventBackColor = 0x808080
var_Group1 = var_Groups.Add(2,"<c><b>Group</b><br><c><img>pic2</img>")
var_Group1.Visible = true
var_Group1.EventBackColor = 0xff
var_Events = oSchedule.Events
// var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00").GroupID = 1
var_Event = var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").GroupID = 2
var_Event1 = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.GroupID = 2]
endwith
// var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00").GroupID = 1
var_Event2 = var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.GroupID = 1]
endwith
oSchedule.HeaderGroupHeight = -1
oSchedule.EndUpdate()
|
282
|
When using the CopyTo method to print a range of dates the printed page is not showing the timescale, is there a way to make it appear (as is, without calendar, multiple-selection)
local oSchedule,s,var_Calendar,var_CopyTo,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "#1/1/2016#"
var_Calendar.Selection = "(int((yearday(value) -1- ((7-weekday(value - yearday(value) + 1)) mod 7) )/7) = int((yearday(#1/7/2016#))/7))"
oSchedule.BorderSelStyle = -1
oSchedule.ShowViewCompact = -1
var_Events = oSchedule.Events
var_Events.Add("01/10/2016 08:30:00","01/10/2016 14:30:00")
var_Events.Add("01/11/2016 09:30:00","01/11/2016 11:30:00")
var_Events.Add("01/15/2016 10:30:00","01/15/2016 11:30:00")
s = oSchedule.Calendar.Selection
oSchedule.Calendar.Selection = "0"
var_CopyTo = oSchedule.CopyTo("c:/temp/test.png")
? "Look for c:/temp/test.png file"
oSchedule.Calendar.Selection = s
|
281
|
When using the CopyTo method to print a range of dates the printed page is not showing the timescale, is there a way to make it appear (as is, without calendar)
local oSchedule,var_Calendar,var_CopyTo,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "01/01/2012"
// var_Calendar.SelectDate("01/01/2012") = false
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#1/1/2012#) = False]
endwith
var_Events = oSchedule.Events
var_Events.Add("01/01/2012 08:30:00","01/01/2012 09:30:00")
var_Events.Add("01/01/2012 08:35:00","01/01/2012 09:35:00")
var_Events.Add("01/01/2012 10:30:00","01/01/2012 12:30:00")
var_CopyTo = oSchedule.CopyTo("c:/temp/test.png")
? "Look for c:/temp/test.png file"
|
280
|
When using the CopyTo method to print a range of dates the printed page is not showing the timescale, is there a way to make it appear (as is, with calendar)
local oSchedule,var_Calendar,var_CopyTo,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "01/01/2012"
// var_Calendar.SelectDate("01/01/2012") = false
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#1/1/2012#) = False]
endwith
var_Events = oSchedule.Events
var_Events.Add("01/01/2012 08:30:00","01/01/2012 09:30:00")
var_Events.Add("01/01/2012 08:35:00","01/01/2012 09:35:00")
var_Events.Add("01/01/2012 10:30:00","01/01/2012 12:30:00")
var_CopyTo = oSchedule.CopyTo("c:/temp/test.png")
? "Look for c:/temp/test.png file"
|
279
|
I've seen that all-day header can be limited up to 4, 8 or 12 events. Can I change that limit, for instance, 3 events only
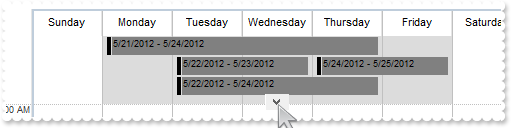
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value >= #5/20/2012# and value <= #5/26/2012#"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 4403 /*0x23 | exAllDayEventWheelScroll | exAllDayEventNoMax*/
oSchedule.BodyEventBackColor = 0x808080
oSchedule.Template = [Background(165) = 14474460] // oSchedule.Background(165) = 0xdcdcdc
var_Events = oSchedule.Events
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/25/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
278
|
Is it possible to highlight the newly created event ( runtime creation )
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.AllowSelectCreateEvent = 2
|
277
|
Is it possible to select the newly created event ( runtime creation )
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.AllowSelectCreateEvent = 1
|
276
|
I have noticed that the all-day header change its background, if has scrolling events. Is it possible to change that (ebn)
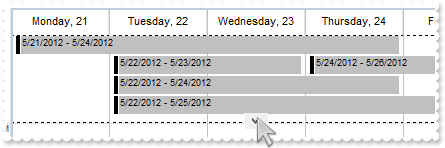
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.VisualAppearance.Add(1,"gBFLBCJwBAEHhEJAAChABRUIQAAYAQGKIaBoAKBQAGaAoDDQMQ3QwAAwjSLEEwsACEIrjKCRShyCYZRrGQBQTCIZBqEqSZLiEZRQCWIAxATGchwHIEQgND6cIDmMAHfj2PI+RZKMoRZJUExZFyERhASQZZoyN40UzOc6vfL9KRDEAEIRKAyTDLQdRyGSMMgEVBJBCbMiNBqhESIJo+GI4BhFYSUZalGSnO6gIBAgIA==")
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 4416 /*exAllDayEventWheelScroll | exAllDayEventMax4*/
oSchedule.Template = [Background(165) = 16777216] // oSchedule.Background(165) = 0x1000000
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/26/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/26/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
275
|
I have noticed that the all-day header change its background, if has scrolling events. Is it possible to change that (solid)
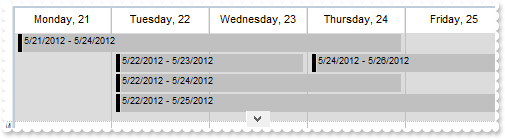
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 4416 /*exAllDayEventWheelScroll | exAllDayEventMax4*/
oSchedule.Template = [Background(165) = 14474460] // oSchedule.Background(165) = 0xdcdcdc
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/26/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/26/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
274
|
I have noticed that the all-day header change its background, if has scrolling events. Is it possible to remove that
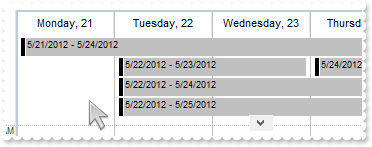
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 4416 /*exAllDayEventWheelScroll | exAllDayEventMax4*/
oSchedule.Template = [Background(165) = 0] // oSchedule.Background(165) = 0x0
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/26/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/26/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
273
|
The user scrolls through the all-day events, when mouse wheel is rotated. Can I disable that
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 320 /*exAllDayEventScroll | exAllDayEventMax4*/
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/26/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/26/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
272
|
Is it possible to specify the number of all-day events to be visible
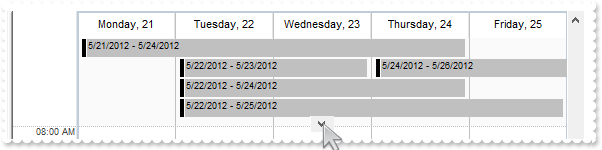
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 4416 /*exAllDayEventWheelScroll | exAllDayEventMax4*/
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/26/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/26/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
271
|
How do I disable scrolling the all-day header
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Event4,var_Event5,var_Event6,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.AllowSelectCreateEvent = 2
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
oSchedule.Calendar.Selection = "05/21/2012"
oSchedule.Calendar.Selection = "value in (#5/21/2012#,#5/22/2012#,#5/23/2012#,#5/24/2012#,#5/25/2012#)"
oSchedule.ShowViewCompact = -1
oSchedule.AllowAllDayEventScroll = 0
var_Events = oSchedule.Events
// var_Events.Add("05/22/2012","05/23/2012").AllDayEvent = true
var_Event = var_Events.Add("05/22/2012","05/23/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
// var_Events.Add("05/21/2012","05/24/2012").AllDayEvent = true
var_Event1 = var_Events.Add("05/21/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/24/2012").AllDayEvent = true
var_Event2 = var_Events.Add("05/22/2012","05/24/2012")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event3 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event3]
TemplateDef = var_Event3
Template = [var_Event3.AllDayEvent = True]
endwith
// var_Events.Add("05/22/2012","05/25/2012").AllDayEvent = true
var_Event4 = var_Events.Add("05/22/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event4]
TemplateDef = var_Event4
Template = [var_Event4.AllDayEvent = True]
endwith
// var_Events.Add("05/23/2012","05/25/2012").AllDayEvent = true
var_Event5 = var_Events.Add("05/23/2012","05/25/2012")
with (oSchedule)
TemplateDef = [dim var_Event5]
TemplateDef = var_Event5
Template = [var_Event5.AllDayEvent = True]
endwith
// var_Events.Add("05/24/2012","05/26/2012").AllDayEvent = true
var_Event6 = var_Events.Add("05/24/2012","05/26/2012")
with (oSchedule)
TemplateDef = [dim var_Event6]
TemplateDef = var_Event6
Template = [var_Event6.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
270
|
How can I display the current week only, when the user selects the date in the calendar panel
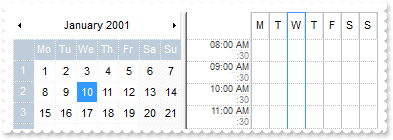
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OnResizeControl = 129 /*exDisableSplitter | exResizePanelRight*/
var_Calendar = oSchedule.Calendar
var_Calendar.OnSelectDate = 1
var_Calendar.Selection = "01/10/2001"
var_Calendar.SingleSel = true
oSchedule.Calendar.FirstWeekDay = 1
oSchedule.ScrollBars = 0
oSchedule.ShowViewCompact = -1
oSchedule.AllowResizeSchedule = 0
oSchedule.AllowMoveSchedule = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.Template = [Background(35) = Background(34)] // oSchedule.Background(35) = oSchedule.Background(34)
oSchedule.TimeScales.Item(0).AllowResize = false
oSchedule.DayViewWidth = 0
oSchedule.EndUpdate()
|
269
|
How do I capture the control and save it as PDF (Method 2)
local oSchedule,var_Calendar,var_CopyTo,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "06/01/2001"
var_Calendar.Selection = "value in (#6/11/2001#,#6/12/2001#)"
oSchedule.DayViewWidth = 512
oSchedule.DayViewHeight = 512
var_Events = oSchedule.Events
var_Events.Add("06/11/2001 10:00:00","06/11/2001 13:00:00")
var_Events.Add("06/12/2001 10:00:00","06/12/2001 13:00:00")
var_CopyTo = oSchedule.CopyTo("C:/Temp/test.pdf")
? "Look For: C:/Temp/test.pdf file"
|
268
|
How do I capture the control and save it as PDF (Method 1)
local oSchedule,var_Calendar,var_Events,var_Print
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "06/01/2001"
var_Calendar.Selection = "value in (#6/11/2001#,#6/12/2001#)"
oSchedule.DayViewWidth = 512
oSchedule.DayViewHeight = 512
var_Events = oSchedule.Events
var_Events.Add("06/11/2001 10:00:00","06/11/2001 13:00:00")
var_Events.Add("06/12/2001 10:00:00","06/12/2001 13:00:00")
var_Print = new OleAutoClient("Exontrol.Print")
var_Print.Options = "FitToPage=On"
var_Print.PrintExt = oSchedule
var_Print.CopyTo("C:/Temp/test.pdf")
? "Look For: C:/Temp/test.pdf file"
|
267
|
How do I capture the control and save it as an image (JPG or BMP) (Method 2)
local oSchedule,var_Calendar,var_CopyTo,var_CopyTo1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "06/01/2001"
var_Calendar.Selection = "value in (#6/11/2001#,#6/12/2001#)"
oSchedule.DayViewWidth = 512
oSchedule.DayViewHeight = 512
var_Events = oSchedule.Events
var_Events.Add("06/11/2001 10:00:00","06/11/2001 13:00:00")
var_Events.Add("06/12/2001 10:00:00","06/12/2001 13:00:00")
var_CopyTo = oSchedule.CopyTo("C:/Temp/test.bmp")
var_CopyTo1 = oSchedule.CopyTo("C:/Temp/test.jpg")
? "Look For: C:/Temp/test.* file"
|
266
|
How do I capture the control and save it as an image (JPG or BMP) (Method 1)
local oSchedule,var_Calendar,var_Events,var_Print
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "06/01/2001"
var_Calendar.Selection = "value in (#6/11/2001#,#6/12/2001#)"
oSchedule.DayViewWidth = 512
oSchedule.DayViewHeight = 512
var_Events = oSchedule.Events
var_Events.Add("06/11/2001 10:00:00","06/11/2001 13:00:00")
var_Events.Add("06/12/2001 10:00:00","06/12/2001 13:00:00")
var_Print = new OleAutoClient("Exontrol.Print")
var_Print.Options = "FitToPage=On"
var_Print.PrintExt = oSchedule
var_Print.CopyTo("C:/Temp/test.bmp")
var_Print.CopyTo("C:/Temp/test.jpg")
? "Look For: C:/Temp/test.* file"
|
265
|
Recurrence: The 2nd to last weekday of the month
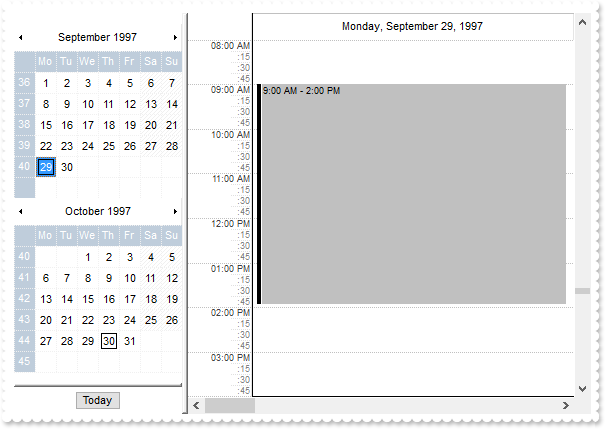
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/29/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/29/1997 09:00:00","09/29/1997 14:00:00").Repetitive = "FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-2"
var_Event = var_Events.Add("09/29/1997 09:00:00","09/29/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-2"]
endwith
oSchedule.EndUpdate()
|
264
|
Recurrence: The 3rd instance into the month of one of Tuesday, Wednesday or Thursday, for the next 3 months
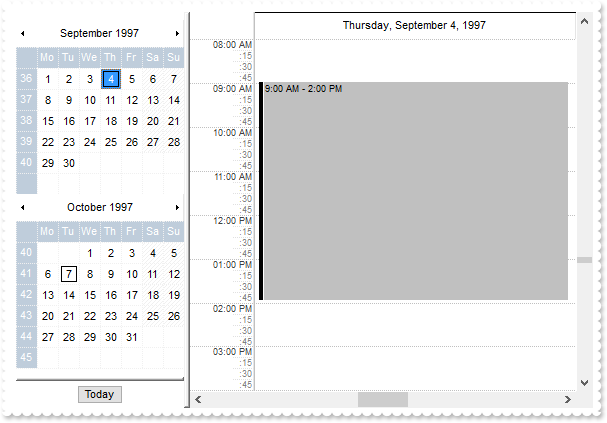
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/04/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/04/1997 09:00:00","09/04/1997 14:00:00").Repetitive = "FREQ=MONTHLY;COUNT=3;BYDAY=TU,WE,TH;BYSETPOS=3"
var_Event = var_Events.Add("09/04/1997 09:00:00","09/04/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;COUNT=3;BYDAY=TU,WE,TH;BYSETPOS=3"]
endwith
oSchedule.EndUpdate()
|
263
|
Recurrence: The last work day of the month
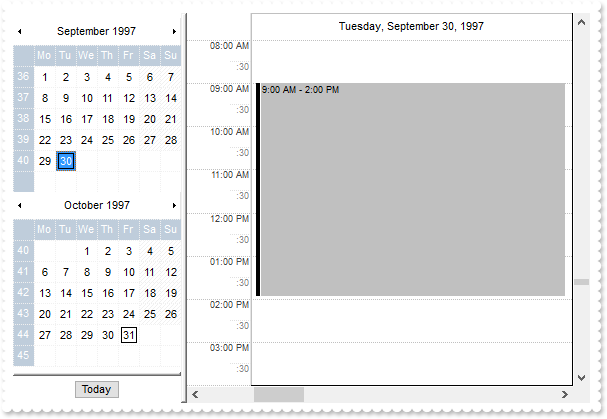
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/30/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("08/05/1997 09:00:00","08/05/1997 14:00:00").Repetitive = "FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-1"
var_Event = var_Events.Add("08/05/1997 09:00:00","08/05/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-1"]
endwith
oSchedule.EndUpdate()
|
262
|
Recurrence: An example where the days generated makes a difference because of WKST (Sample 2)
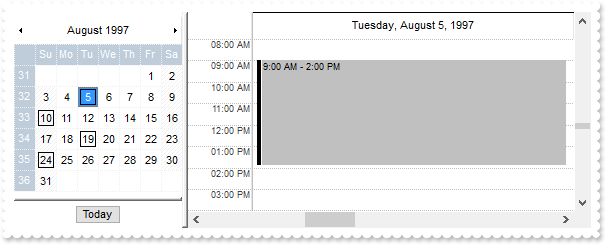
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "08/05/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 0
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("08/05/1997 09:00:00","08/05/1997 14:00:00").Repetitive = "FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU"
var_Event = var_Events.Add("08/05/1997 09:00:00","08/05/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU"]
endwith
oSchedule.EndUpdate()
|
261
|
Recurrence: An example where the days generated makes a difference because of WKST (Sample 1)
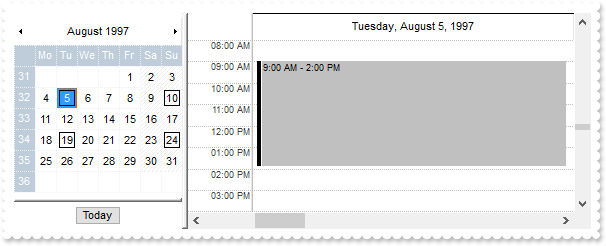
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "08/05/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("08/05/1997 09:00:00","08/05/1997 14:00:00").Repetitive = "FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU"
var_Event = var_Events.Add("08/05/1997 09:00:00","08/05/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU"]
endwith
oSchedule.EndUpdate()
|
260
|
Recurrence: Every four years, the first Tuesday after a Monday in November, forever (U.S. Presidential Election day)
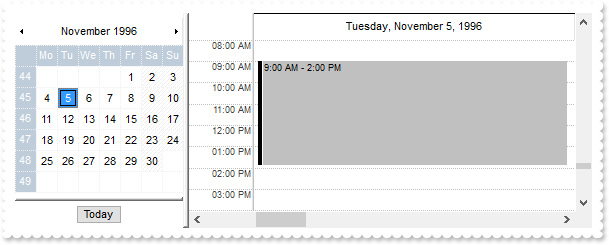
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "11/05/1996"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("11/05/1996 09:00:00","11/05/1996 14:00:00").Repetitive = "FREQ=YEARLY;INTERVAL=4;BYMONTH=11;BYDAY=TU;BYMONTHDAY=2,3,4,5,6,7,8"
var_Event = var_Events.Add("11/05/1996 09:00:00","11/05/1996 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;INTERVAL=4;BYMONTH=11;BYDAY=TU;BYMONTHDAY=2,3,4,5,6,7,8"]
endwith
oSchedule.EndUpdate()
|
259
|
Recurrence: The first Saturday that follows the first Sunday of the month, forever
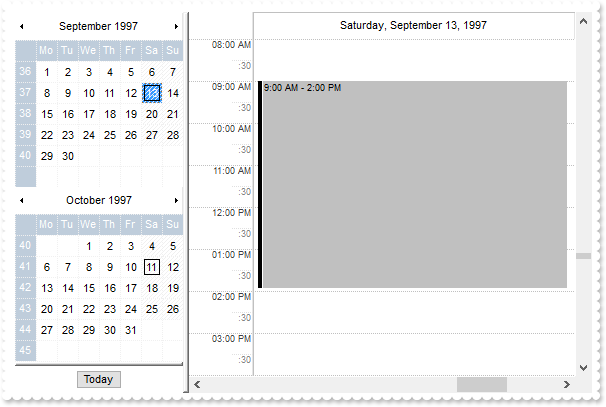
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/13/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/13/1997 09:00:00","09/13/1997 14:00:00").Repetitive = "FREQ=MONTHLY;BYDAY=SA;BYMONTHDAY=7,8,9,10,11,12,13"
var_Event = var_Events.Add("09/13/1997 09:00:00","09/13/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;BYDAY=SA;BYMONTHDAY=7,8,9,10,11,12,13"]
endwith
oSchedule.EndUpdate()
|
258
|
Recurrence: Every Friday the 13th, forever
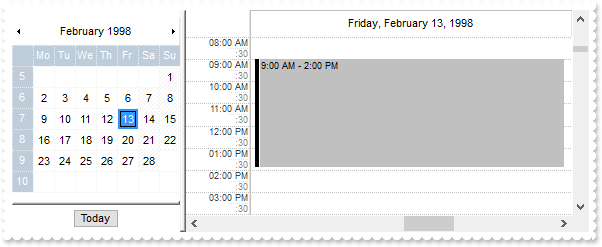
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "02/13/1998"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=MONTHLY;BYDAY=FR;BYMONTHDAY=13"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;BYDAY=FR;BYMONTHDAY=13"]
endwith
oSchedule.EndUpdate()
|
257
|
Recurrence: Every Thursday, but only during June, July, and August, forever
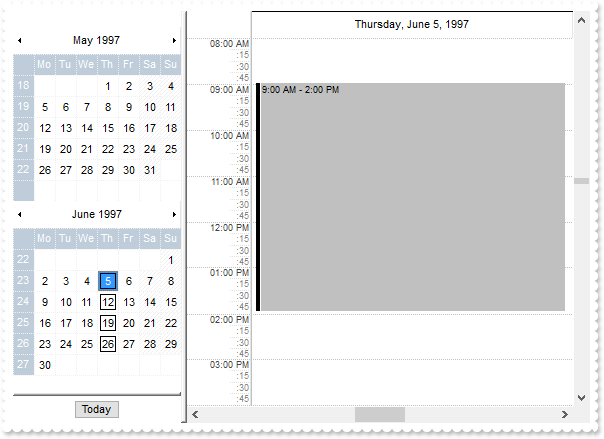
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "06/05/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("06/05/1997 09:00:00","06/05/1997 14:00:00").Repetitive = "FREQ=YEARLY;BYDAY=TH;BYMONTH=6,7,8"
var_Event = var_Events.Add("06/05/1997 09:00:00","06/05/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;BYDAY=TH;BYMONTH=6,7,8"]
endwith
oSchedule.EndUpdate()
|
256
|
Recurrence: Every Thursday in March, forever
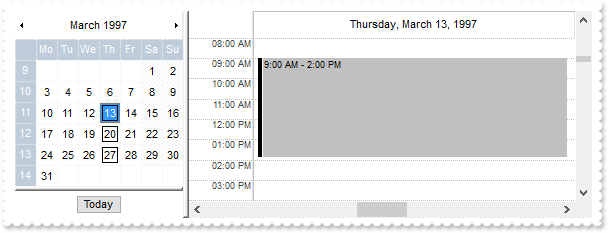
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "03/13/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("03/13/1997 09:00:00","03/13/1997 14:00:00").Repetitive = "FREQ=YEARLY;BYMONTH=3;BYDAY=TH"
var_Event = var_Events.Add("03/13/1997 09:00:00","03/13/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;BYMONTH=3;BYDAY=TH"]
endwith
oSchedule.EndUpdate()
|
255
|
Recurrence: Monday of week number 20 (where the default start of the week is Monday), forever
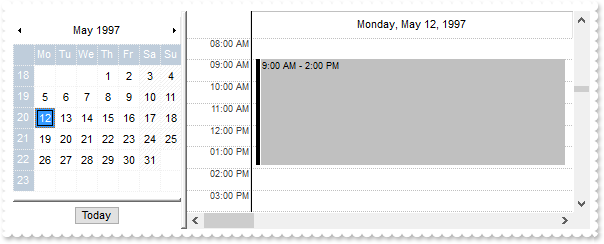
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "05/12/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("05/12/1997 09:00:00","05/12/1997 14:00:00").Repetitive = "FREQ=YEARLY;BYWEEKNO=20;BYDAY=MO"
var_Event = var_Events.Add("05/12/1997 09:00:00","05/12/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;BYWEEKNO=20;BYDAY=MO"]
endwith
oSchedule.EndUpdate()
|
254
|
Recurrence: Every 20th Monday of the year, forever
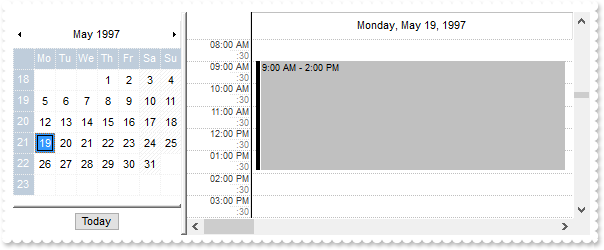
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "05/19/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("01/01/1997 09:00:00","01/01/1997 14:00:00").Repetitive = "FREQ=YEARLY;BYDAY=20MO"
var_Event = var_Events.Add("01/01/1997 09:00:00","01/01/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;BYDAY=20MO"]
endwith
oSchedule.EndUpdate()
|
253
|
Recurrence: Every 3rd year on the 1st, 100th and 200th day for 10 occurrences
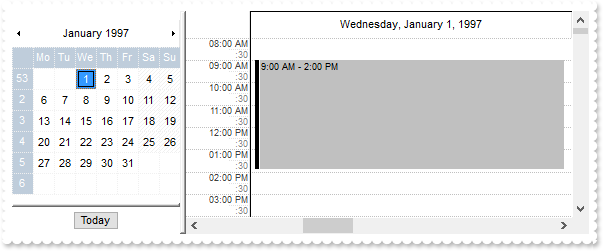
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "01/01/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("01/01/1997 09:00:00","01/01/1997 14:00:00").Repetitive = "FREQ=YEARLY;INTERVAL=3;COUNT=10;BYYEARDAY=1,100,200"
var_Event = var_Events.Add("01/01/1997 09:00:00","01/01/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;INTERVAL=3;COUNT=10;BYYEARDAY=1,100,200"]
endwith
oSchedule.EndUpdate()
|
252
|
Recurrence: Every other year on January, February, and March for 10 occurrences
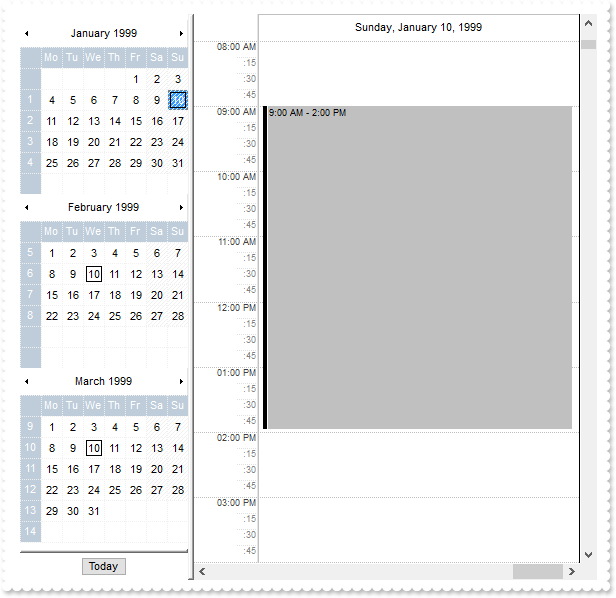
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "03/10/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("03/10/1997 09:00:00","03/10/1997 14:00:00").Repetitive = "FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3"
var_Event = var_Events.Add("03/10/1997 09:00:00","03/10/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3"]
endwith
oSchedule.EndUpdate()
|
251
|
Recurrence: Yearly in June and July for 10 occurrences
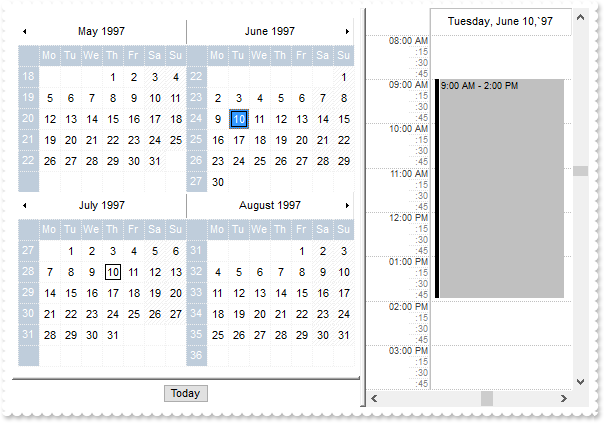
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "06/10/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthX = 2
var_Calendar.MaxMonthY = 2
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("06/10/1997 09:00:00","06/10/1997 14:00:00").Repetitive = "FREQ=YEARLY;COUNT=10;BYMONTH=6,7"
var_Event = var_Events.Add("06/10/1997 09:00:00","06/10/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=YEARLY;COUNT=10;BYMONTH=6,7"]
endwith
oSchedule.EndUpdate()
|
250
|
Recurrence: Every Tuesday, every other month
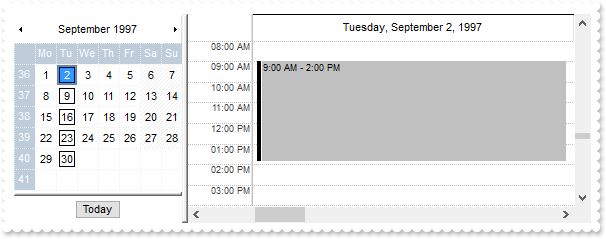
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/02/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=MONTHLY;INTERVAL=2;BYDAY=TU"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;INTERVAL=2;BYDAY=TU"]
endwith
oSchedule.EndUpdate()
|
249
|
Recurrence: Every 18 months on the 10th thru 15th of the month for 10 occurrences
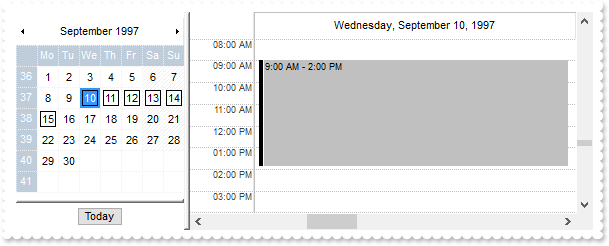
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/10/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/10/1997 09:00:00","09/10/1997 14:00:00").Repetitive = "FREQ=MONTHLY;INTERVAL=18;COUNT=10;BYMONTHDAY=10,11,12,13,14,15"
var_Event = var_Events.Add("09/10/1997 09:00:00","09/10/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;INTERVAL=18;COUNT=10;BYMONTHDAY=10,11,12,13,14,15"]
endwith
oSchedule.EndUpdate()
|
248
|
Recurrence: Monthly on the first and last day of the month for 10 occurrences
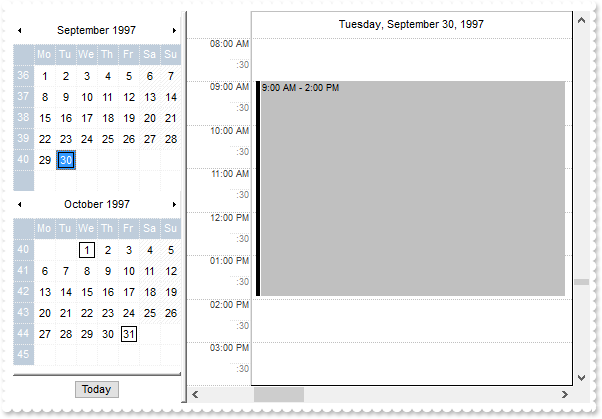
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/30/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/03/1997 09:00:00","09/03/1997 14:00:00").Repetitive = "FREQ=MONTHLY;COUNT=10;BYMONTHDAY=1,-1"
var_Event = var_Events.Add("09/03/1997 09:00:00","09/03/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;COUNT=10;BYMONTHDAY=1,-1"]
endwith
oSchedule.EndUpdate()
|
247
|
Recurrence: Monthly on the 2nd and 15th of the month for 10 occurrences
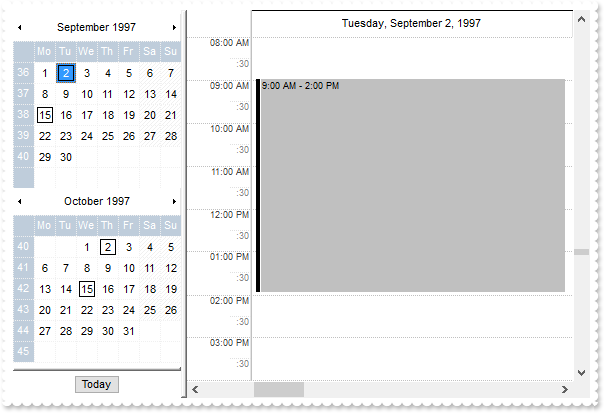
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/02/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=MONTHLY;COUNT=10;BYMONTHDAY=2,15"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;COUNT=10;BYMONTHDAY=2,15"]
endwith
oSchedule.EndUpdate()
|
246
|
Recurrence: Monthly on the third to the last day of the month, forever
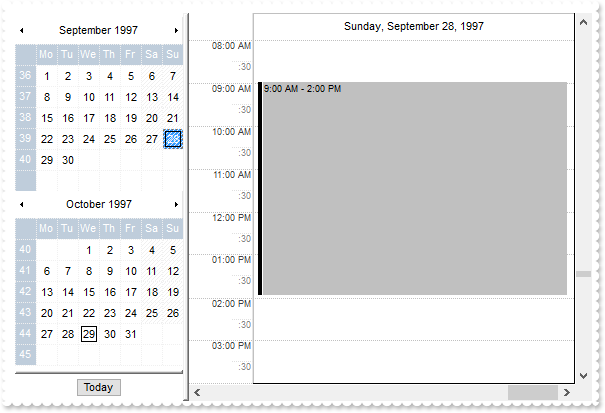
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/28/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/28/1997 09:00:00","09/28/1997 14:00:00").Repetitive = "FREQ=MONTHLY;BYMONTHDAY=-3"
var_Event = var_Events.Add("09/28/1997 09:00:00","09/28/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;BYMONTHDAY=-3"]
endwith
oSchedule.EndUpdate()
|
245
|
Recurrence: Monthly on the second to last Monday of the month for 6 months
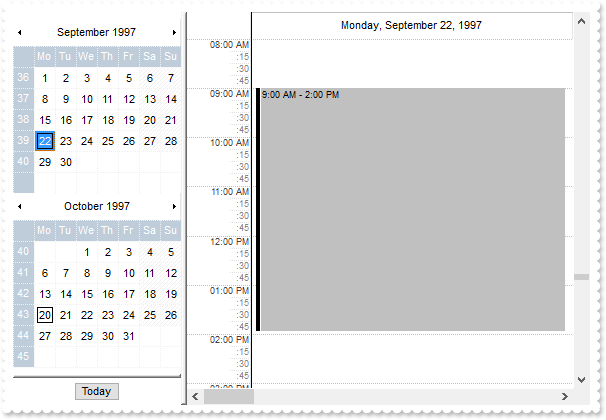
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/22/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/22/1997 09:00:00","09/22/1997 14:00:00").Repetitive = "FREQ=MONTHLY;COUNT=6;BYDAY=-2MO"
var_Event = var_Events.Add("09/22/1997 09:00:00","09/22/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;COUNT=6;BYDAY=-2MO"]
endwith
oSchedule.EndUpdate()
|
244
|
Recurrence: Every other month on the 1st and last Sunday of the month for 10 occurrences
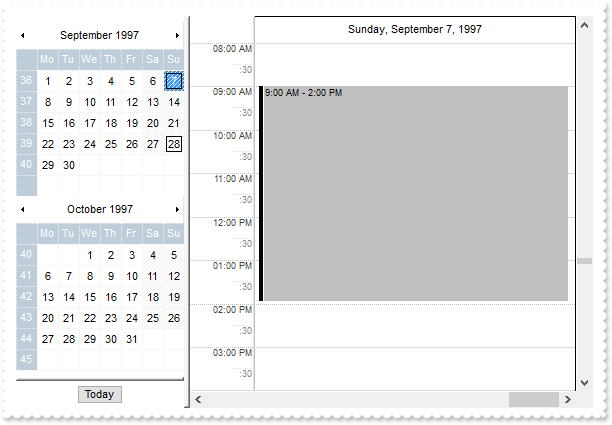
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/07/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/07/1997 09:00:00","09/07/1997 14:00:00").Repetitive = "FREQ=MONTHLY;INTERVAL=2;COUNT=10;BYDAY=1SU,-1SU"
var_Event = var_Events.Add("09/07/1997 09:00:00","09/07/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;INTERVAL=2;COUNT=10;BYDAY=1SU,-1SU"]
endwith
oSchedule.EndUpdate()
|
243
|
Recurrence: Monthly on the 1st Friday until December 24, 1997
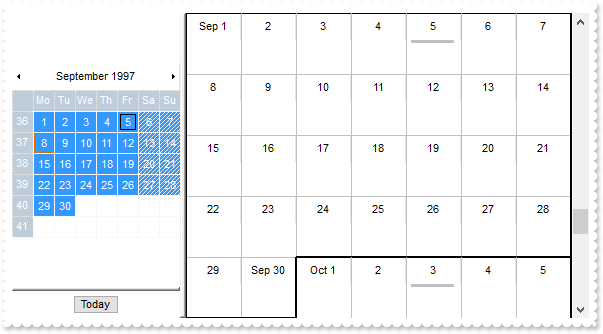
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/05/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/05/1997 09:00:00","09/05/1997 14:00:00").Repetitive = "FREQ=MONTHLY;UNTIL=19971224T000000Z;BYDAY=1FR"
var_Event = var_Events.Add("09/05/1997 09:00:00","09/05/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;UNTIL=19971224T000000Z;BYDAY=1FR"]
endwith
oSchedule.EndUpdate()
|
242
|
Recurrence: Monthly on the 1st Friday for ten occurrences
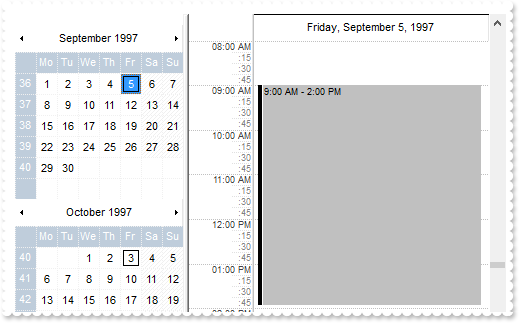
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/05/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/05/1997 09:00:00","09/05/1997 14:00:00").Repetitive = "FREQ=MONTHLY;COUNT=10;BYDAY=1FR"
var_Event = var_Events.Add("09/05/1997 09:00:00","09/05/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=MONTHLY;COUNT=10;BYDAY=1FR"]
endwith
oSchedule.EndUpdate()
|
241
|
Recurrence: Every other week on Tuesday and Thursday, for 8 occurrences
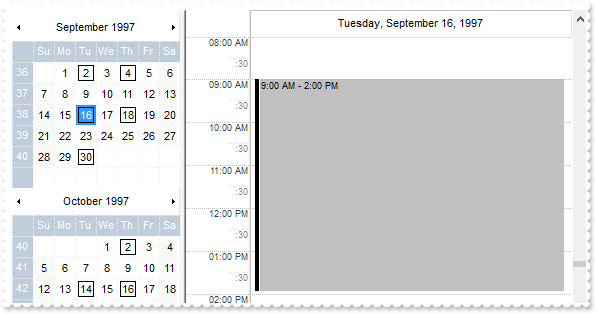
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/16/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 0
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=WEEKLY;INTERVAL=2;COUNT=8;WKST=SU;BYDAY=TU,TH"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=WEEKLY;INTERVAL=2;COUNT=8;WKST=SU;BYDAY=TU,TH"]
endwith
oSchedule.EndUpdate()
|
240
|
Recurrence: Every other week on Monday, Wednesday and Friday until December 24, 1997, but starting on Tuesday, September 2, 1997
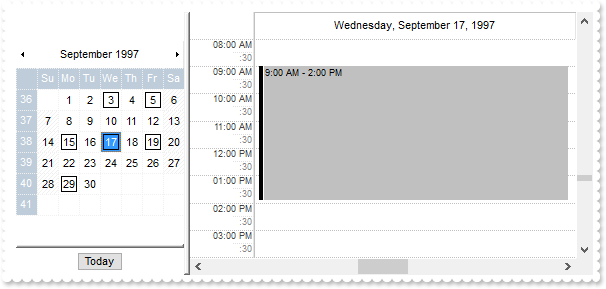
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/17/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 0
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=WEEKLY;INTERVAL=2;UNTIL=19971224T000000Z;WKST=SU;BYDAY=MO,WE,FR"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=WEEKLY;INTERVAL=2;UNTIL=19971224T000000Z;WKST=SU;BYDAY=MO,WE,FR"]
endwith
oSchedule.EndUpdate()
|
239
|
Recurrence: Weekly on Tuesday and Thursday for 5 weeks
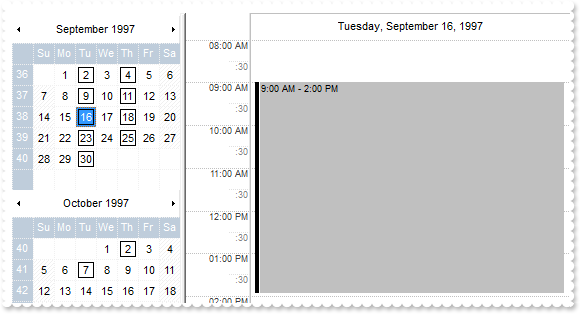
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/16/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 0
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=WEEKLY;UNTIL=19971007T000000Z;WKST=SU;BYDAY=TU,TH"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=WEEKLY;UNTIL=19971007T000000Z;WKST=SU;BYDAY=TU,TH"]
endwith
oSchedule.EndUpdate()
|
238
|
Recurrence: Weekly on Tuesday and Thursday for 5 weeks
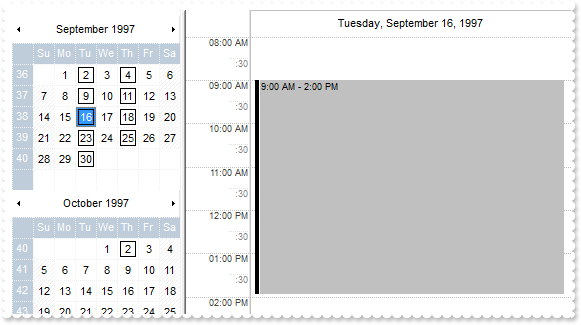
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/16/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 0
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=WEEKLY;COUNT=10;WKST=SU;BYDAY=TU,TH"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=WEEKLY;COUNT=10;WKST=SU;BYDAY=TU,TH"]
endwith
oSchedule.EndUpdate()
|
237
|
Recurrence: Every other day - forever
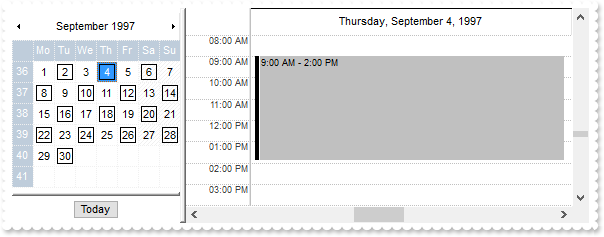
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/04/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=DAILY;INTERVAL=2"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=DAILY;INTERVAL=2"]
endwith
oSchedule.EndUpdate()
|
236
|
Recurrence: Daily until December 24, 1997
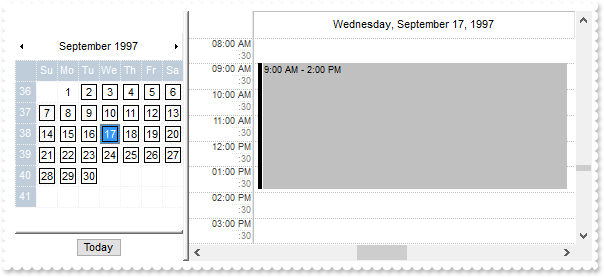
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/17/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Calendar.FirstWeekDay = 1
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=DAILY;UNTIL=19971224T000000Z"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=DAILY;UNTIL=19971224T000000Z"]
endwith
oSchedule.EndUpdate()
|
235
|
Recurrence: Daily for 10 occurrences
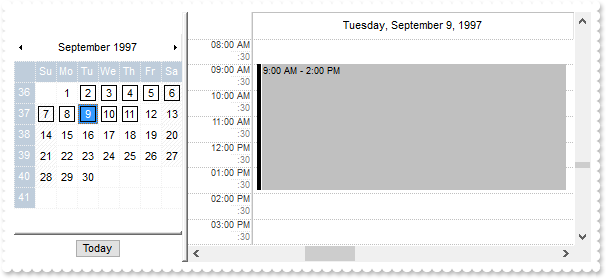
local oSchedule,var_Calendar,var_Event,var_Events,var_Highlight
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "09/09/1997"
var_Calendar.ShowNonMonthDays = false
var_Calendar.MaxMonthY = 4
var_Highlight = var_Calendar.HighlightEvent
var_Highlight.Pattern.Type = 256
var_Highlight.Bold = false
var_Calendar.FirstWeekDay = 1
oSchedule.ShowViewCompact = -1
oSchedule.BorderSelStyle = -1
oSchedule.Template = [Background(81) = 15790320] // oSchedule.Background(81) = 0xf0f0f0
var_Events = oSchedule.Events
// var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00").Repetitive = "FREQ=DAILY;COUNT=10"
var_Event = var_Events.Add("09/02/1997 09:00:00","09/02/1997 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.Repetitive = "FREQ=DAILY;COUNT=10"]
endwith
oSchedule.EndUpdate()
|
234
|
How do I display the week number according, so the January 1st is in the first week
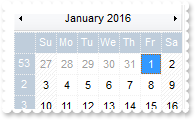
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "01/01/2016"
var_Calendar.FirstWeekDay = 0
var_Calendar.DisplayWeekNumberAs = 1
oSchedule.EndUpdate()
|
233
|
How do I display the week number according to ISO8601 standard
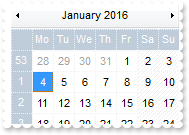
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "01/04/2016"
var_Calendar.FirstWeekDay = 1
var_Calendar.DisplayWeekNumberAs = 0
oSchedule.EndUpdate()
|
232
|
How do I hide the week number
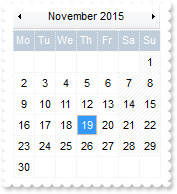
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.FirstWeekDay = 1
var_Calendar.ShowWeeks = false
var_Calendar.ShowNonMonthDays = false
oSchedule.EndUpdate()
|
231
|
How can I change the background color of the all day header
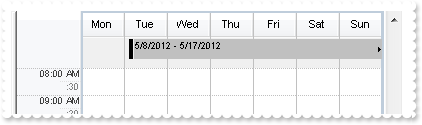
local oSchedule,var_Calendar,var_Event
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OnResizeControl = 2048
oSchedule.ShowAllDayHeader = true
var_Calendar = oSchedule.Calendar
var_Calendar.FirstWeekDay = 1
// var_Calendar.SelectDate("05/08/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/8/2012#) = True]
endwith
var_Calendar.Select(3)
// var_Calendar.SelectDate("05/15/2012") = false
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/15/2012#) = False]
endwith
var_Calendar.Select(19 /*exSelectToggle | exSelectWeek*/)
oSchedule.Template = [Background(87) = 15790320] // oSchedule.Background(87) = 0xf0f0f0
oSchedule.HeaderAllDayEventHeight = -20
// oSchedule.Events.Add("05/08/2012","05/17/2012").AllDayEvent = true
var_Event = oSchedule.Events.Add("05/08/2012","05/17/2012")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.AllDayEvent = True]
endwith
oSchedule.EndUpdate()
|
230
|
How can I display a single text on a specified date
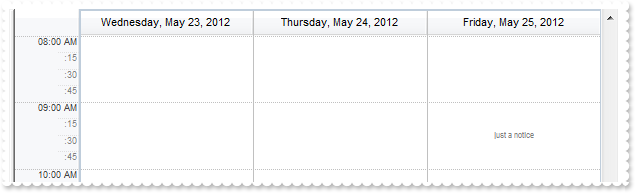
local oSchedule,var_NonworkingPattern
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/26/2012"
oSchedule.Calendar.Selection = "value in (#05/23/2012#,#05/24/2012#,#05/25/2012#)"
// oSchedule.NonworkingPatterns.Add(1234,0).BackgroundExt = "[text=`<font ;6><fgcolor 808080>just a notice`,align=0x11]"
var_NonworkingPattern = oSchedule.NonworkingPatterns.Add(1234,0)
with (oSchedule)
TemplateDef = [dim var_NonworkingPattern]
TemplateDef = var_NonworkingPattern
Template = [var_NonworkingPattern.BackgroundExt = "[text=`<font ;6><fgcolor 808080>just a notice`,align=0x11]"]
endwith
oSchedule.NonworkingTimes.Add("value = #05/25/2012#","09:00","10:00",1234)
oSchedule.EndUpdate()
|
229
|
I am using the control's DataSource property, the question is how can I get the event's identifier Datafield(exEventID)
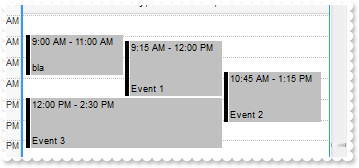
local oSchedule,rs
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
rs = new OleAutoClient("ADOR.Recordset")
rs.Open("Events","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Program Files\Exontrol\ExSchedule\Sample\Access2007\datasource.accdb",3,3)
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "11/11/2013"
oSchedule.Template = [DataField(1) = "Start"] // oSchedule.DataField(1) = "Start"
oSchedule.Template = [DataField(2) = "End"] // oSchedule.DataField(2) = "End"
oSchedule.Template = [DataField(11) = "Extra"] // oSchedule.DataField(11) = "Extra"
oSchedule.DataSource = rs
? Str(oSchedule.Events.Item(1).KnownProperty(12))
oSchedule.EndUpdate()
|
228
|
Is it possible to display some text/image on the schedule's view background
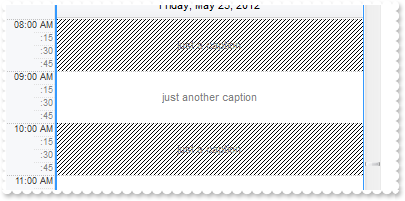
local oSchedule,var_NonworkingPattern,var_NonworkingPattern1
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/25/2012"
// oSchedule.NonworkingPatterns.Add(1234,6).BackgroundExt = "[text=`<fgcolor 808080>just a caption`,align=0x11]"
var_NonworkingPattern = oSchedule.NonworkingPatterns.Add(1234,6)
with (oSchedule)
TemplateDef = [dim var_NonworkingPattern]
TemplateDef = var_NonworkingPattern
Template = [var_NonworkingPattern.BackgroundExt = "[text=`<fgcolor 808080>just a caption`,align=0x11]"]
endwith
// oSchedule.NonworkingPatterns.Add(1235,0).BackgroundExt = "[text=`<fgcolor 808080>just another caption`,align=0x11]"
var_NonworkingPattern1 = oSchedule.NonworkingPatterns.Add(1235,0)
with (oSchedule)
TemplateDef = [dim var_NonworkingPattern1]
TemplateDef = var_NonworkingPattern1
Template = [var_NonworkingPattern1.BackgroundExt = "[text=`<fgcolor 808080>just another caption`,align=0x11]"]
endwith
oSchedule.NonworkingTimes.Add("weekday(value) = 5","08:00","08:59:59",1234)
oSchedule.NonworkingTimes.Add("weekday(value) = 5","09:00","09:59:59",1235)
oSchedule.NonworkingTimes.Add("weekday(value) = 5","10:00","10:59:59",1234)
oSchedule.EndUpdate()
|
227
|
How can I display a text on the schedule's view background
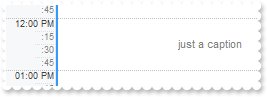
local oSchedule,var_NonworkingPattern
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/25/2012"
// oSchedule.NonworkingPatterns.Add(1234,0).BackgroundExt = "[text=`<fgcolor 808080>just a caption`,align=0x11]"
var_NonworkingPattern = oSchedule.NonworkingPatterns.Add(1234,0)
with (oSchedule)
TemplateDef = [dim var_NonworkingPattern]
TemplateDef = var_NonworkingPattern
Template = [var_NonworkingPattern.BackgroundExt = "[text=`<fgcolor 808080>just a caption`,align=0x11]"]
endwith
oSchedule.NonworkingTimes.Add("weekday(value) = 5","12:00","13:00",1234)
oSchedule.EndUpdate()
|
226
|
Is it possible to change the visual appearance of the edit's context menu
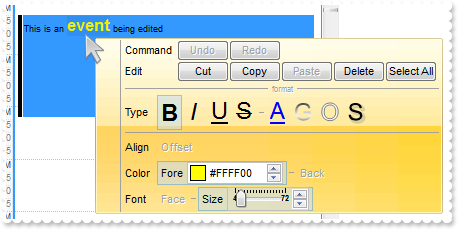
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.VisualAppearance.Add(1,"c:\exontrol\images\normal.ebn")
oSchedule.Template = [Background(99) = 16777216] // oSchedule.Background(99) = 0x1000000
|
225
|
How do I customize the edit's context menu
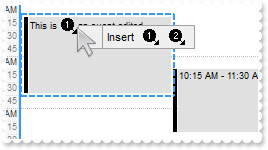
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.Images("gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTqlVq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yNAOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=")
oSchedule.EditContextMenuItems = "Insert[group=3](<img>1</img>[id=57763],<img>2</img>[id=57763])"
|
224
|
How can I lock/fix the date header, so it stays on the top while the user scrolls the chart
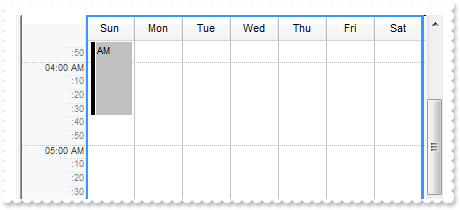
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
LayoutEndChanging = class::nativeObject_LayoutEndChanging
endwith
*/
// Notifies your application once the control's layout has been changed.
function nativeObject_LayoutEndChanging(Operation)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? Str(Operation)
oSchedule.DayViewHeight = 2016
return
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
// var_Calendar.SelectDate("05/20/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/20/2012#) = True]
endwith
var_Calendar.Select(3)
oSchedule.ScrollBars = 2
oSchedule.ShowViewCompact = 3
oSchedule.DayViewHeight = 2016
oSchedule.AllowMoveSchedule = 0
oSchedule.AllowResizeSchedule = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.AllowExchangePanels = 0
oSchedule.AllowMoveTimeScale = 0
oSchedule.AllowResizeTimeScale = 0
oSchedule.AllowMultiDaysEvent = false
oSchedule.TimeScales.Item(0).MinorTimeRuler = "00:10"
oSchedule.DayStartTime = "00:00"
oSchedule.DayEndTime = "24:00"
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
oSchedule.EndUpdate()
|
223
|
Is there any way to control the vertical size of each hour other than by the font and/or the minor time scale being changed
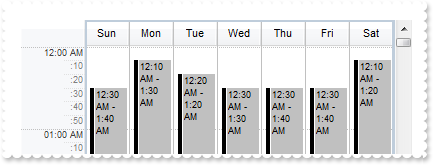
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
// var_Calendar.SelectDate("05/20/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/20/2012#) = True]
endwith
var_Calendar.Select(3)
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
oSchedule.ScrollBars = 2
oSchedule.ShowViewCompact = 1
oSchedule.DayViewHeight = 2016
oSchedule.AllowMoveSchedule = 0
oSchedule.AllowResizeSchedule = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.AllowExchangePanels = 0
oSchedule.AllowMoveTimeScale = 0
oSchedule.AllowResizeTimeScale = 0
oSchedule.AllowMultiDaysEvent = false
oSchedule.Template = [Background(36) = Background(37)] // oSchedule.Background(36) = oSchedule.Background(37)
oSchedule.TimeScales.Item(0).MinorTimeRuler = "00:10"
oSchedule.DayStartTime = "00:00"
oSchedule.DayEndTime = "24:00"
oSchedule.EndUpdate()
|
222
|
How can I show a single week, no calendar
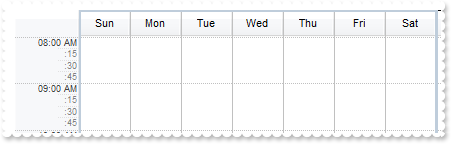
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
// var_Calendar.SelectDate("05/20/2012") = true
with (oSchedule)
TemplateDef = [dim var_Calendar]
TemplateDef = var_Calendar
Template = [var_Calendar.SelectDate(#5/20/2012#) = True]
endwith
var_Calendar.Select(3)
oSchedule.OnResizeControl = 768 /*exChangePanels | exHideSplitter*/
oSchedule.ScrollBars = 0
oSchedule.ShowViewCompact = 1
oSchedule.AllowMoveSchedule = 0
oSchedule.AllowResizeSchedule = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.AllowExchangePanels = 0
oSchedule.AllowMoveTimeScale = 0
oSchedule.AllowResizeTimeScale = 0
oSchedule.AllowMultiDaysEvent = false
oSchedule.Template = [Background(36) = Background(37)] // oSchedule.Background(36) = oSchedule.Background(37)
oSchedule.EndUpdate()
|
221
|
How do I select the dates within a giving interval only
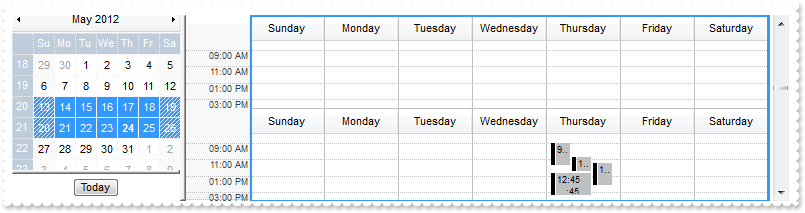
local oSchedule,var_Calendar,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "05/01/2012"
var_Calendar.Selection = "(value >= #5/13/2012#) and (value <= #5/26/2012#)"
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 09:00:00","05/24/2012 12:00:00")
var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:45:00")
var_Events.Add("05/24/2012 11:30:00","05/24/2012 14:30:00")
var_Events.Add("05/24/2012 12:45:00","05/24/2012 15:45:00")
oSchedule.EndUpdate()
|
220
|
How do I display the dates within a giving interval only
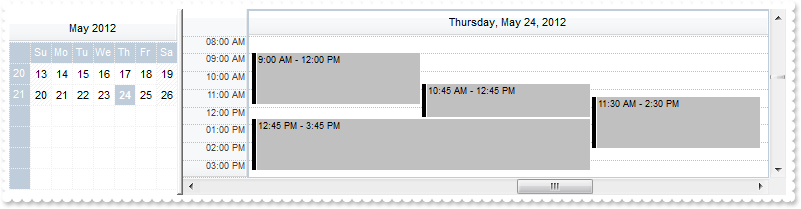
local oSchedule,var_Calendar,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
var_Calendar = oSchedule.Calendar
var_Calendar.Selection = "05/24/2012"
var_Calendar.MinDate = "05/13/2012"
var_Calendar.MaxDate = "05/26/2012"
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 09:00:00","05/24/2012 12:00:00")
var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:45:00")
var_Events.Add("05/24/2012 11:30:00","05/24/2012 14:30:00")
var_Events.Add("05/24/2012 12:45:00","05/24/2012 15:45:00")
oSchedule.EndUpdate()
|
219
|
Is it possible that the time is displayed starting from 00:00 to 24:00 00 instead of 08:00 AM to 04:00 PM

local oSchedule,var_TimeScale
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.DayStartTime = "00:00"
oSchedule.DayEndTime = "24:00"
var_TimeScale = oSchedule.TimeScales.Item(0)
var_TimeScale.MajorTimeLabel = "<%hh%>:<%nn%>"
var_TimeScale.Width = 32
|
218
|
How do I show a double frame
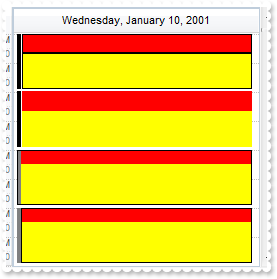
local oSchedule,var_Event,var_Event1,var_Event2,var_Event3,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.SelectEventStyle = 48
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.DefaultEventLongLabel = ""
oSchedule.DefaultEventShortLabel = ""
var_Events = oSchedule.Events
var_Event = var_Events.Add("01/10/2001 08:00:00","01/10/2001 10:00:00")
var_Event.BodyBackgroundExt = "top[25%,back=RGB(255,0,0),align=0x21,pattern=0x000,frame=RGB(0,0,0)],client[back=RGB(255,255,0),align=0x21,pattern=0x000,frame=RGB(0,0,0)]"
// var_Event.BodyBackgroundExtValue(1,1) = 255
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.BodyBackgroundExtValue(1,1) = 255]
endwith
// var_Event.BodyBackgroundExtValue(1,2) = "35%"
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.BodyBackgroundExtValue(1,2) = "35%"]
endwith
var_Event1 = var_Events.Add("01/10/2001 10:00:00","01/10/2001 12:00:00")
var_Event1.BodyBackgroundExt = "top[25%,back=RGB(255,0,0),align=0x22],client[back=RGB(255,255,0),align=0x22]"
// var_Event1.BodyBackgroundExtValue(1,2) = "35%"
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.BodyBackgroundExtValue(1,2) = "35%"]
endwith
// var_Event1.BodyBackgroundExtValue(2,1) = 65535
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.BodyBackgroundExtValue(2,1) = 65535]
endwith
var_Event2 = var_Events.Add("01/10/2001 12:00:00","01/10/2001 14:00:00")
var_Event2.ShowStatus = false
var_Event2.BodyBackgroundExt = "left[4,back=RGB(128,128,128)],top[25%,back=RGB(255,0,0)],client[back=RGB(255,255,0)],none[(0%,0%,100%,100%),pattern=0x000,frame=RGB(0,0,0)]"
var_Event3 = var_Events.Add("01/10/2001 14:00:00","01/10/2001 16:00:00")
var_Event3.ShowStatus = false
var_Event3.BodyBackgroundExt = "left[4,back=RGB(128,128,128)],top[25%,back=RGB(255,0,0)],client[back=RGB(255,255,0)],none[(4,0%,100%-4,100%),pattern=0x000,frame=RGB(0,0,0)]"
|
217
|
How can I add more colors on the event
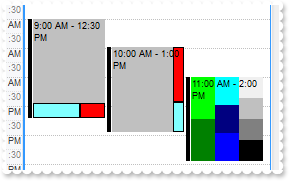
local oSchedule,var_Event,var_Event1,var_Event2,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.SelectEventStyle = 48
oSchedule.Calendar.Selection = "01/10/2001"
var_Events = oSchedule.Events
// var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").BodyBackgroundExt = "bottom[15%](left[65%,frame,back=RGB(128,255,255)],client[back=RGB(255,0,0),frame])"
var_Event = var_Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.BodyBackgroundExt = "bottom[15%](left[65%,frame,back=RGB(128,255,255)],client[back=RGB(255,0,0),frame])"]
endwith
// var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00").BodyBackgroundExt = "right[15%](bottom[35%,frame,back=RGB(128,255,255)],client[back=RGB(255,0,0),frame])"
var_Event1 = var_Events.Add("01/10/2001 10:00:00","01/10/2001 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.BodyBackgroundExt = "right[15%](bottom[35%,frame,back=RGB(128,255,255)],client[back=RGB(255,0,0),frame])"]
endwith
// var_Events.Add("01/10/2001 11:00:00","01/10/2001 14:00:00").BodyBackgroundExt = "left[33%,back=RGB(0,128,0)](top[50%,back=RGB(0,255,0)]),left[33%](top[33%,back=RGB(0,255,255)],top[33%,back=RGB(0,0,128)],client[back=RGB(0,0,255)]),client(top[25%,back=RGB(240,240,240)],top[25%,back=RGB(192,192,192)],top[25%,back=RGB(128,128,128)],client[back=RGB(0,0,0)])"
var_Event2 = var_Events.Add("01/10/2001 11:00:00","01/10/2001 14:00:00")
with (oSchedule)
TemplateDef = [dim var_Event2]
TemplateDef = var_Event2
Template = [var_Event2.BodyBackgroundExt = "left[33%,back=RGB(0,128,0)](top[50%,back=RGB(0,255,0)]),left[33%](top[33%,back=RGB(0,255,255)],top[33%,back=RGB(0,0,128)],client[back=RGB(0,0,255)]),client(top[25%,back=RGB(240,240,240)],top[25%,back=RGB(192,192,192)],top[25%,back=RGB(128,128,128)],client[back=RGB(0,0,0)])"]
endwith
|
216
|
When I have 3 month visible in the calendar section, it seems I can only move back and forth through the months 3 months at a time. Is it possible to move back and forth through the months 1 month at a time
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.AlignDate = false
var_Calendar.MinMonthY = 2
var_Calendar.MaxMonthY = 2
|
215
|
How can I bound the control to a data source
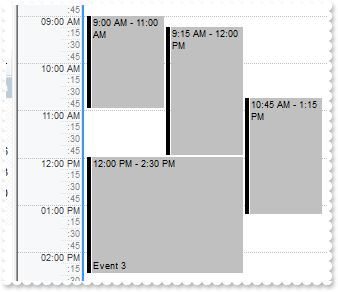
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
Error = class::nativeObject_Error
endwith
*/
// Fired when an internal error occurs.
function nativeObject_Error(Error,Description)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? Str(Description)
return
local oSchedule,rs
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
rs = new OleAutoClient("ADOR.Recordset")
rs.Open("Events","Provider=Microsoft.ACE.OLEDB.12.0;Data Source=C:\Program Files\Exontrol\ExSchedule\Sample\Access2007\datasource.accdb",3,3)
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "11/11/2013"
oSchedule.Template = [DataField(1) = "Start"] // oSchedule.DataField(1) = "Start"
oSchedule.Template = [DataField(2) = "End"] // oSchedule.DataField(2) = "End"
oSchedule.Template = [DataField(11) = "Extra"] // oSchedule.DataField(11) = "Extra"
oSchedule.DataSource = rs
oSchedule.EndUpdate()
|
214
|
How can I start drag and drop an event
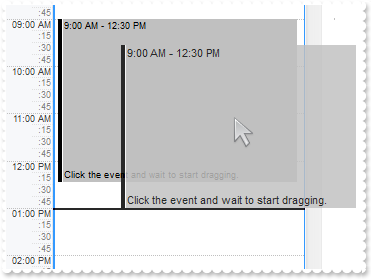
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
OLEStartDrag = class::nativeObject_OLEStartDrag
endwith
*/
// Occurs when the OLEDrag method is called.
function nativeObject_OLEStartDrag(Data,AllowedEffects)
/* Data.SetData( "your data to be dragged" ) */
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
AllowedEffects = 1
return
local oSchedule,var_Event
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.OLEDropMode = 1
oSchedule.SelectEventStyle = 48
oSchedule.DefaultEventLongLabel = "<%=%256%><br><%=%5%>"
oSchedule.DefaultEventShortLabel = oSchedule.DefaultEventLongLabel
oSchedule.Calendar.Selection = "01/10/2001"
oSchedule.OnResizeControl = 3073 /*exCalendarAutoHide | exCalendarFit | exResizePanelRight*/
// oSchedule.Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00").ExtraLabel = "Click the event and wait to start dragging."
var_Event = oSchedule.Events.Add("01/10/2001 09:00:00","01/10/2001 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.ExtraLabel = "Click the event and wait to start dragging."]
endwith
oSchedule.EndUpdate()
|
213
|
I use the HighlightDate property to mark a date, instead the selection is not visible if I select the same date. What can be done
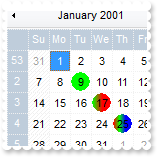
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.ShowHighlightDate = 4353 /*exHighlightDateCalendarEllipticClip | exHighlightDateCalendarGradient | exShowHighlightDateCalendar*/
oSchedule.Calendar.Selection = "01/01/2001"
oSchedule.Template = [HighlightDate(#1/9/2001#) = 65280] // oSchedule.HighlightDate("01/09/2001") = 65280
oSchedule.Template = [HighlightDate(#1/17/2001#) = "65280,255"] // oSchedule.HighlightDate("01/17/2001") = "65280,255"
oSchedule.Template = [HighlightDate(#1/25/2001#) = "255,65280,16711680"] // oSchedule.HighlightDate("01/25/2001") = "255,65280,16711680"
|
212
|
How can I arrange the colors to highlight the date vertically
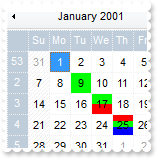
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.ShowHighlightDate = 49 /*exHighlightDateVertical | exShowHighlightDateCalendar*/
oSchedule.Calendar.Selection = "01/01/2001"
oSchedule.Template = [HighlightDate(#1/9/2001#) = 65280] // oSchedule.HighlightDate("01/09/2001") = 65280
oSchedule.Template = [HighlightDate(#1/17/2001#) = "65280,255"] // oSchedule.HighlightDate("01/17/2001") = "65280,255"
oSchedule.Template = [HighlightDate(#1/25/2001#) = "255,65280,16711680"] // oSchedule.HighlightDate("01/25/2001") = "255,65280,16711680"
|
211
|
Is it possible to highlight a date in gradient
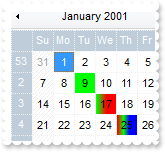
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.ShowHighlightDate = 257 /*exHighlightDateCalendarGradient | exShowHighlightDateCalendar*/
oSchedule.Calendar.Selection = "01/01/2001"
oSchedule.Template = [HighlightDate(#1/9/2001#) = 65280] // oSchedule.HighlightDate("01/09/2001") = 65280
oSchedule.Template = [HighlightDate(#1/17/2001#) = "65280,255"] // oSchedule.HighlightDate("01/17/2001") = "65280,255"
oSchedule.Template = [HighlightDate(#1/25/2001#) = "255,65280,16711680"] // oSchedule.HighlightDate("01/25/2001") = "255,65280,16711680"
|
210
|
Is it possible to highlight a date in the calendar panel only
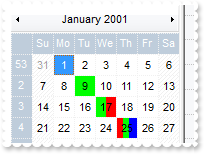
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.ShowHighlightDate = 1
oSchedule.Calendar.Selection = "01/01/2001"
oSchedule.Template = [HighlightDate(#1/9/2001#) = 65280] // oSchedule.HighlightDate("01/09/2001") = 65280
oSchedule.Template = [HighlightDate(#1/17/2001#) = "65280,255"] // oSchedule.HighlightDate("01/17/2001") = "65280,255"
oSchedule.Template = [HighlightDate(#1/25/2001#) = "255,65280,16711680"] // oSchedule.HighlightDate("01/25/2001") = "255,65280,16711680"
|
209
|
Is it possible to highlight a date in the control
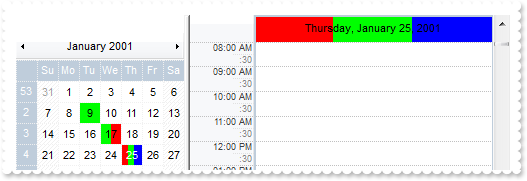
local oSchedule
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.ShowHighlightDate = 3
oSchedule.Calendar.Selection = "01/25/2001"
oSchedule.Template = [HighlightDate(#1/9/2001#) = 65280] // oSchedule.HighlightDate("01/09/2001") = 65280
oSchedule.Template = [HighlightDate(#1/17/2001#) = "65280,255"] // oSchedule.HighlightDate("01/17/2001") = "65280,255"
oSchedule.Template = [HighlightDate(#1/25/2001#) = "255,65280,16711680"] // oSchedule.HighlightDate("01/25/2001") = "255,65280,16711680"
|
208
|
How we need to put "nonworking time" to each group, because staff A is working different times then staff B. Is this possible
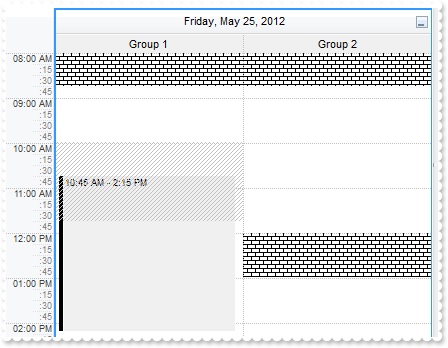
local oSchedule,var_Event,var_Group,var_Group1,var_NonworkingTime,var_NonworkingTime1,var_NonworkingTimes
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.BodyEventBackColor = 0xf0f0f0
oSchedule.ShowGroupingEvents = true
oSchedule.DisplayGroupingButton = true
// oSchedule.Groups.Add(1,"Group 1").Visible = true
var_Group = oSchedule.Groups.Add(1,"Group 1")
with (oSchedule)
TemplateDef = [dim var_Group]
TemplateDef = var_Group
Template = [var_Group.Visible = True]
endwith
// oSchedule.Groups.Add(2,"Group 2").Visible = true
var_Group1 = oSchedule.Groups.Add(2,"Group 2")
with (oSchedule)
TemplateDef = [dim var_Group1]
TemplateDef = var_Group1
Template = [var_Group1.Visible = True]
endwith
oSchedule.Calendar.Selection = "05/25/2012"
oSchedule.NonworkingPatterns.Add(1234,11)
var_NonworkingTimes = oSchedule.NonworkingTimes
var_NonworkingTimes.Add("1","00:00","08:45",1234)
// var_NonworkingTimes.Add("weekday(value) = 5","10:00","11:45",1).GroupID = 1
var_NonworkingTime = var_NonworkingTimes.Add("weekday(value) = 5","10:00","11:45",1)
with (oSchedule)
TemplateDef = [dim var_NonworkingTime]
TemplateDef = var_NonworkingTime
Template = [var_NonworkingTime.GroupID = 1]
endwith
// var_NonworkingTimes.Add("weekday(value) = 5","12:00","13:00",1234).GroupID = 2
var_NonworkingTime1 = var_NonworkingTimes.Add("weekday(value) = 5","12:00","13:00",1234)
with (oSchedule)
TemplateDef = [dim var_NonworkingTime1]
TemplateDef = var_NonworkingTime1
Template = [var_NonworkingTime1.GroupID = 2]
endwith
// oSchedule.Events.Add("05/25/2012 09:30:00","05/25/2012 13:00:00").GroupID = 1
var_Event = oSchedule.Events.Add("05/25/2012 09:30:00","05/25/2012 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.GroupID = 1]
endwith
oSchedule.EndUpdate()
|
207
|
I have a double click event set to launch a window so the user can supply input. The problem is that double click changes the view. How do I change this behavior
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
DblClick = class::nativeObject_DblClick
endwith
*/
// Occurs when the user dblclk the left mouse button over an object.
function nativeObject_DblClick(Shift,X,Y)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? "DblClick "
? Str(X)
? Str(Y)
return
local oSchedule,var_Event,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
// var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00").StatusColor = 0xff
var_Event = var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.StatusColor = 255]
endwith
var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
oSchedule.AllowEditEvent = 0
oSchedule.AllowToggleSchedule = 0
oSchedule.EndUpdate()
|
206
|
I have two-time scales. How do I change the label while updating the events to show the date-time on the second time-scale
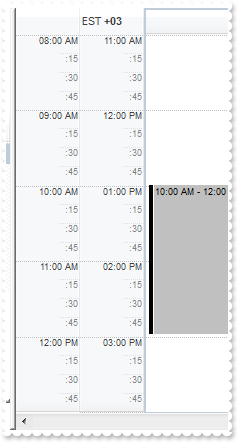
local oSchedule,var_Events,var_TimeScale
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.DayEndTime = "13:00"
var_TimeScale = oSchedule.TimeScales.Add("+3:00")
var_TimeScale.AlignLeft = true
var_TimeScale.Caption = "EST <b>+03"
oSchedule.BeginUpdate()
oSchedule.Calendar.Selection = "05/24/2012"
oSchedule.UpdateEventsLabel = "Start: <%=date(%1+3/24)%><br>End: <%=date(%2+3/24)%>"
var_Events = oSchedule.Events
var_Events.Add("05/24/2012 10:00:00","05/24/2012 12:00:00")
var_Events.Add("05/24/2012 10:45:00","05/24/2012 12:30:00")
var_Events.Add("05/24/2012 11:30:00","05/24/2012 13:30:00")
oSchedule.EndUpdate()
|
205
|
Is there a way to add a hyperlink to the event that would run a report with parameters
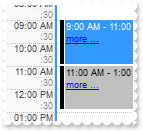
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
AnchorClick = class::nativeObject_AnchorClick
endwith
*/
// Occurs when an anchor element is clicked.
function nativeObject_AnchorClick(AnchorID,Options)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? Str(AnchorID)
? Str(Options)
return
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.Calendar.Selection = "06/20/2012"
var_Events = oSchedule.Events
// var_Events.Add("06/20/2012 09:00:00","06/20/2012 11:00:00").LongLabel = "<%=%256%><br><a 1234;option 1>more ...</a>"
var_Event = var_Events.Add("06/20/2012 09:00:00","06/20/2012 11:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.LongLabel = "<%=%256%><br><a 1234;option 1>more ...</a>"]
endwith
// var_Events.Add("06/20/2012 11:00:00","06/20/2012 13:00:00").LongLabel = "<%=%256%><br><a 1235;option 2>more ...</a>"
var_Event1 = var_Events.Add("06/20/2012 11:00:00","06/20/2012 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.LongLabel = "<%=%256%><br><a 1235;option 2>more ...</a>"]
endwith
|
204
|
Can I have multiple months in the calendar section
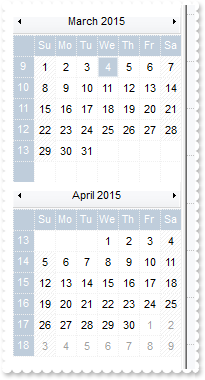
local oSchedule,var_Calendar
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
var_Calendar = oSchedule.Calendar
var_Calendar.MinMonthY = 2
var_Calendar.MaxMonthY = 2
|
203
|
I need to make sure that at least the order number stays visible when the event is resized. Is there a way during event modification (another event be added at the same time that makes the event size shrink) to adjust the caption location
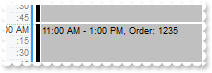
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.Calendar.Selection = "06/20/2012"
var_Events = oSchedule.Events
var_Event = var_Events.Add("06/20/2012 09:00:00","06/20/2012 11:00:00")
var_Event.UserData = 1234
var_Event.ShortLabel = "<%=%256%><br>Order: <%=%6%>"
var_Event.LongLabel = var_Event.ShortLabel
var_Event1 = var_Events.Add("06/20/2012 11:00:00","06/20/2012 13:00:00")
var_Event1.UserData = 1235
var_Event1.ShortLabel = "<%=%256%>, Order: <%=%6%>"
var_Event1.LongLabel = var_Event1.ShortLabel
|
202
|
I need to make sure that at least the order number stays visible when the event is resized. Is there a way during event modification (another event be added at the same time that makes the event size shrink) to adjust the caption location
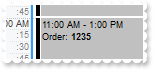
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.Calendar.Selection = "06/20/2012"
oSchedule.DefaultEventShortLabel = "<%=%256%><br>Order: <b><%=%6%>"
oSchedule.DefaultEventLongLabel = oSchedule.DefaultEventShortLabel
var_Events = oSchedule.Events
// var_Events.Add("06/20/2012 09:00:00","06/20/2012 11:00:00").UserData = 1234
var_Event = var_Events.Add("06/20/2012 09:00:00","06/20/2012 11:00:00")
with (oSchedule)
TemplateDef = [dim var_Event]
TemplateDef = var_Event
Template = [var_Event.UserData = 1234]
endwith
// var_Events.Add("06/20/2012 11:00:00","06/20/2012 13:00:00").UserData = 1235
var_Event1 = var_Events.Add("06/20/2012 11:00:00","06/20/2012 13:00:00")
with (oSchedule)
TemplateDef = [dim var_Event1]
TemplateDef = var_Event1
Template = [var_Event1.UserData = 1235]
endwith
|
201
|
Can you add text with links in the event
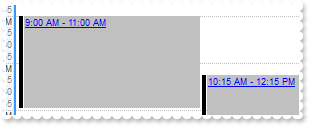
/*
with (this.EXSCHEDULEACTIVEXCONTROL1.nativeObject)
AnchorClick = class::nativeObject_AnchorClick
endwith
*/
// Occurs when an anchor element is clicked.
function nativeObject_AnchorClick(AnchorID,Options)
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
? Str(AnchorID)
? Str(Options)
return
local oSchedule,var_Event,var_Event1,var_Events
oSchedule = form.EXSCHEDULEACTIVEXCONTROL1.nativeObject
oSchedule.Calendar.Selection = "06/20/2012"
var_Events = oSchedule.Events
var_Event = var_Events.Add("06/20/2012 09:00:00","06/20/2012 11:00:00")
var_Event.ShortLabel = "<a 1><%=%256%></a>"
var_Event.LongLabel = var_Event.ShortLabel
var_Event1 = var_Events.Add("06/20/2012 11:00:00","06/20/2012 13:00:00")
var_Event1.ShortLabel = "<a 2><%=%256%></a>"
var_Event1.LongLabel = var_Event1.ShortLabel
|