22
|
How can I play animated GIF using the control
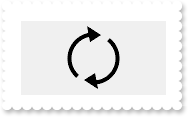
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->Create(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll",L"System.Windows.Forms.Label");
spNETHost1->GetHost()->PutTemplate(L"Image = LoadPicture(`C:\\Program Files (x86)\\Exontrol\\ExNETHost\\Sample\\wait.gif`)");
|
21
|
How can I use the Template property
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TreeView");
spNETHost1->GetHost()->PutTemplate(L"Nodes { Add(`Root 1`).Nodes { Add(`Child 1`); Add(`Child 2`) } }");
|
20
|
How can I use the TemplateResult property (method 2)
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TreeView");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"Nodes.Add(`Root 1`){ BackColor = RGB(255,0,0);ForeColor = RGB(255,255,255) }");
var_NETHostObject->GetTemplateResult()->PutTemplate(L"Nodes{ Add(`Child 1`); Add(`Child 2`) }; Expand() }");
|
19
|
How can I use the TemplateResult property (method 1)
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TreeView");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"Nodes.Add(`Root 1`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject1 = var_NETHostObject->GetTemplateResult();
var_NETHostObject1->PutTemplate(L"Nodes.Add(`Child 1`)");
var_NETHostObject1->PutTemplate(L"Nodes.Add(`Child 2`)");
var_NETHostObject1->PutTemplate(L"Expand()");
|
18
|
How can I find the information about the hosting control, like name, version, ...
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyQualifiedName(L"System.Windows.Forms.ListBox, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
OutputDebugStringW( _bstr_t(var_NETHostObject->GetItem(L"ProductName")->GetValue()) );
OutputDebugStringW( _bstr_t(var_NETHostObject->GetItem(L"ProductVersion")->GetValue()) );
OutputDebugStringW( _bstr_t(var_NETHostObject->GetItem(L"CompanyName")->GetValue()) );
|
17
|
How do I get the hwnd/handle of the hosting control
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
OutputDebugStringW( _bstr_t(spNETHost1->GetHost()->GetItem(L"Handle")->GetAsInt()) );
|
16
|
How do I get the number of arguments that an event has
// HostEvent event - The hosting control fires an event.
void OnHostEventNETHost1(LPDISPATCH Ev)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
OutputDebugStringW( L"Ev.Arguments.Item(\"GetType().GetProperties().Length\").AsString" );
OutputDebugStringW( L"Ev" );
}
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
|
15
|
How can I change the control's background color, as BackgroundColor seems to have no effect
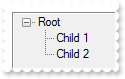
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TreeView");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"BackColor = RGB(240,240,240)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject1 = var_NETHostObject->GetItem(L"Nodes.Add(`Root`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject = var_NETHostObject1->GetItem(L"Nodes.Add(`Child 1`)");
var_NETHostObject1 = var_NETHostObject1->GetItem(L"Nodes.Add(`Child 2`)");
ObjectPtr var_Object = ((ObjectPtr)(var_NETHostObject1->GetItem(L"Expand()")));
|
14
|
How can I add a TreeView
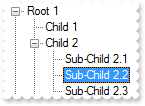
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TreeView");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject1 = var_NETHostObject->GetItem(L"Nodes.Add(`Root 1`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject = var_NETHostObject1->GetItem(L"Nodes.Add(`Child 1`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject2 = var_NETHostObject1->GetItem(L"Nodes.Add(`Child 2`)");
var_NETHostObject1 = var_NETHostObject2->GetItem(L"Nodes.Add(`Sub-Child 2.1`)");
var_NETHostObject2 = var_NETHostObject2->GetItem(L"Nodes.Add(`Sub-Child 2.2`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject3 = var_NETHostObject2->GetItem(L"Nodes.Add(`Sub-Child 2.3`)");
ObjectPtr var_Object = ((ObjectPtr)(var_NETHostObject2->GetItem(L"Expand()")));
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject4 = var_NETHostObject1->GetItem(L"Nodes.Add(`Child 3`)");
ObjectPtr var_Object1 = ((ObjectPtr)(var_NETHostObject1->GetItem(L"Expand()")));
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject5 = var_NETHostObject->GetItem(L"Nodes.Add(`Root 2`)");
var_NETHostObject5 = var_NETHostObject5->GetItem(L"Nodes.Add(`Child 1`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject6 = var_NETHostObject5->GetItem(L"Nodes.Add(`Child 2`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject7 = var_NETHostObject5->GetItem(L"Nodes.Add(`Child 3`)");
|
13
|
How can I add a ProgressBar
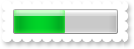
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.ProgressBar");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->SetTemplateDef("Dim percent");
var_NETHostObject->SetTemplateDef(long(50));
var_NETHostObject->PutTemplate(L"Value = percent");
|
12
|
How can I add a DateTimePicker
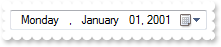
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.DateTimePicker");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->SetTemplateDef("Dim date");
var_NETHostObject->SetTemplateDef(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_NETHostObject->PutTemplate(L"Value = date");
|
11
|
How can I add CheckedListBox

/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.CheckedListBox");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"FormattingEnabled = True");
var_NETHostObject->PutTemplate(L"Items.Add(`Check 1`)");
var_NETHostObject->PutTemplate(L"Items.Add(`Check 2`,True)");
|
10
|
How can I specify the list of events to be handled
// HostEvent event - The hosting control fires an event.
void OnHostEventNETHost1(LPDISPATCH Ev)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
OutputDebugStringW( L"Ev" );
OutputDebugStringW( L"Button " );
OutputDebugStringW( L"Ev" );
}
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutBackgroundColor(16777215);
spNETHost1->PutHostEvents(L"MouseUp MouseDown");
spNETHost1->Create(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll",L"System.Windows.Forms.MonthCalendar");
spNETHost1->GetHost()->PutTemplate(L"MaxSelectionCount = 1");
|
9
|
Is it possible to handle only a specific event
// HostEvent event - The hosting control fires an event.
void OnHostEventNETHost1(LPDISPATCH Ev)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
OutputDebugStringW( L"Ev" );
}
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutBackgroundColor(16777215);
spNETHost1->Create(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll",L"System.Windows.Forms.MonthCalendar");
spNETHost1->PutHostEvents(L"DateSelected");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"MaxSelectionCount = 1");
|
8
|
How can I handle events withing the control
// HostEvent event - The hosting control fires an event.
void OnHostEventNETHost1(LPDISPATCH Ev)
{
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
OutputDebugStringW( L"Ev" );
}
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutBackgroundColor(16777215);
spNETHost1->Create(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll",L"System.Windows.Forms.MonthCalendar");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"MaxSelectionCount = 1");
|
7
|
How can I add the Tab page, and pages inside (method 2)
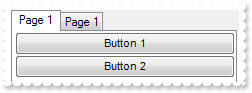
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TabControl");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject1 = var_NETHostObject->GetItem(_bstr_t("Dim page; page = CreateObject(`System.Windows.Forms.TabPage, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyT") +
"oken=b77a5c561934e089`){Text = `new`};Controls.Add(page);page");
var_NETHostObject1->PutTemplate(L"Text = `Page 1`;UseVisualStyleBackColor = True");
var_NETHostObject1->PutTemplate(_bstr_t("Dim c; c = CreateObject(`System.Windows.Forms.Button, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b7") +
"7a5c561934e089`){Text=`Button 2`;Dock=1}; Controls.Add(c)");
var_NETHostObject1->PutTemplate(_bstr_t("Dim c; c = CreateObject(`System.Windows.Forms.Button, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b7") +
"7a5c561934e089`){Text=`Button 1`;Dock=1}; Controls.Add(c)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject2 = var_NETHostObject->GetItem(_bstr_t("Dim page; page = CreateObject(`System.Windows.Forms.TabPage, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyT") +
"oken=b77a5c561934e089`){Text = `new`};Controls.Add(page);page");
var_NETHostObject2->PutTemplate(L"Text = `Page 1`;UseVisualStyleBackColor = True");
var_NETHostObject2->PutTemplate(_bstr_t("Dim c; c = CreateObject(`System.Windows.Forms.TextBox, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b") +
"77a5c561934e089`){Text=`Edit 2`;Dock=1}; Controls.Add(c)");
var_NETHostObject2->PutTemplate(_bstr_t("Dim c; c = CreateObject(`System.Windows.Forms.TextBox, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b") +
"77a5c561934e089`){Text=`Edit 1`;Dock=1}; Controls.Add(c)");
|
6
|
How can I add the Tab page, and pages inside (method 1)
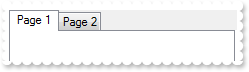
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.TabControl");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject1 = var_NETHostObject->GetItem(_bstr_t("CreateObject(`System.Windows.Forms.TabPage, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934") +
"e089`)");
var_NETHostObject1->PutTemplate(L"Text = `Page 1`;UseVisualStyleBackColor = True");
var_NETHostObject->SetTemplateDef("Dim page");
var_NETHostObject->SetTemplateDef(var_NETHostObject->GetTemplateResult()->GetValue());
var_NETHostObject->PutTemplate(L"Controls.Add(page)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject2 = var_NETHostObject->GetItem(_bstr_t("CreateObject(`System.Windows.Forms.TabPage, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934") +
"e089`)");
var_NETHostObject2->PutTemplate(L"Text = `Page 2`;UseVisualStyleBackColor = True");
var_NETHostObject->SetTemplateDef("Dim page");
var_NETHostObject->SetTemplateDef(var_NETHostObject->GetTemplateResult()->GetValue());
var_NETHostObject->PutTemplate(L"Controls.Add(page)");
|
5
|
Just wondering if I can host your /NET control
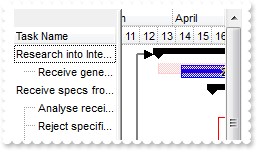
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Program Files\\Exontrol\\ExG2antt.NET\\Sample\\exontrol.exg2antt.dll");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"LoadXML(`http://www.exontrol.net/testing.xml`)");
|
4
|
How can I insert the MonthCalendar of /NET framework
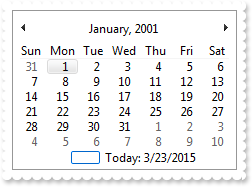
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutBackgroundColor(16777215);
spNETHost1->Create(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll",L"System.Windows.Forms.MonthCalendar");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->SetTemplateDef("Dim x");
var_NETHostObject->SetTemplateDef(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_NETHostObject->PutTemplate(L"MaxSelectionCount = 1;SelectionStart = x");
|
3
|
How can I use the AssemblyQualifiedName property
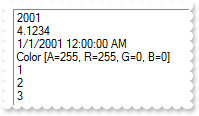
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyQualifiedName(L"System.Windows.Forms.ListBox, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject = spNETHost1->GetHost()->GetItem(L"Items");
var_NETHostObject->PutTemplate(L"Add(2001)");
var_NETHostObject->PutTemplate(L"Add(4.1234)");
var_NETHostObject->PutTemplate(L"Add(#1/1/2001#)");
var_NETHostObject->PutTemplate(L"Add(RGB(255,0,0))");
var_NETHostObject->PutTemplate(L"Add(1);Add(2);Add(3)");
|
2
|
How can I use the AssemblyLocation property
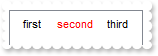
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyLocation(L"C:\\Windows\\assembly\\GAC_MSIL\\System.Windows.Forms\\2.0.0.0__b77a5c561934e089\\System.Windows.Forms.dll");
spNETHost1->PutAssemblyName(L"System.Windows.Forms.ListView");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"Items.Add(`first`)");
exontrol_NETHost::INETObjectTemplatePtr var_NETHostObject1 = var_NETHostObject->GetItem(_bstr_t("CreateObject(`System.Windows.Forms.ListViewItem, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c5") +
"61934e089`)");
var_NETHostObject1->PutTemplate(L"Text = `second`;ForeColor = RGB(255,0,0)");
var_NETHostObject->SetTemplateDef("Dim n");
var_NETHostObject->SetTemplateDef(var_NETHostObject->GetTemplateResult()->GetValue());
var_NETHostObject->PutTemplate(L"Items.Add(n)");
var_NETHostObject->PutTemplate(L"Items.Add(`third`)");
|
1
|
How can I insert the PropertyGrid of /NET framework
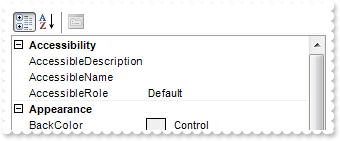
/*
Copy and paste the following directives to your header file as
it defines the namespace 'exontrol_NETHost' for the library: 'Exontrol NETHost ActiveX Component'
#import <exontrol.NETHost.tlb>
*/
exontrol_NETHost::INETHostCtrlPtr spNETHost1 = GetDlgItem(IDC_NETHOST1)->GetControlUnknown();
spNETHost1->PutAssemblyQualifiedName(L"System.Windows.Forms.PropertyGrid, System.Windows.Forms, Version=2.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089");
exontrol_NETHost::INETHostObjectPtr var_NETHostObject = spNETHost1->GetHost();
var_NETHostObject->PutTemplate(L"BackColor = RGB(255,255,255);ViewBackColor = RGB(255,255,255);LineColor = ViewBackColor");
var_NETHostObject->SetTemplateDef("dim object");
var_NETHostObject->SetTemplateDef(spNETHost1);
var_NETHostObject->PutTemplate(L"SelectedObject = object");
|