69
|
How do I encode data as ICalendar format
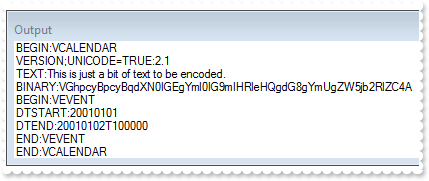
Dim oICalendar as P
Dim var_Component as P
Dim var_Component1 as P
Dim var_Component2 as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content
var_Component1 = var_Component.Components.Add("VCALENDAR")
var_Component1.Properties.Add("VERSION","2.1").Parameters.Add("UNICODE",.toICalendar(oICalendar.t.,2))
var_Component1.Properties.Add("TEXT","This is just a bit of text to be encoded.")
var_Component1.Properties.Add("BINARY",oICalendar.toICalendar("This is just a bit of text to be encoded.",1))
var_Component2 = var_Component1.Components.Add("VEVENT")
var_Component2.Properties.Add("DTSTART",{01/01/2001})
var_Component2.Properties.Add("DTEND",{01/02/2001 10:00:00})
? oICalendar.Save()
|
68
|
How do I get the occurrences between giving start/end margins
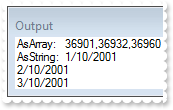
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? "AsArray: "
? oICalendar.RecurRange("DTSTART=19970310;FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3",{01/01/2001},{01/01/2002})
? "AsString: "
? oICalendar.RecurRangeAsString("DTSTART=19970310;FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3",{01/01/2001},{01/01/2002})
|
67
|
How do I get the value of specified part in the recurrence expression
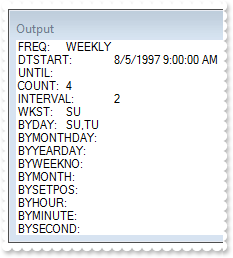
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? "FREQ: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",0)
? "DTSTART: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",1)
? "UNTIL: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",2)
? "COUNT: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",3)
? "INTERVAL: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",4)
? "WKST: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",14)
? "BYDAY: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",8)
? "BYMONTHDAY: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",9)
? "BYYEARDAY: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",10)
? "BYWEEKNO: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",11)
? "BYMONTH: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",12)
? "BYSETPOS: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",13)
? "BYHOUR: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",7)
? "BYMINUTE: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",6)
? "BYSECOND: "
? oICalendar.RecurPartValue("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",5)
|
66
|
How do I check if the recurrence expression is syntactically correct (method 2)
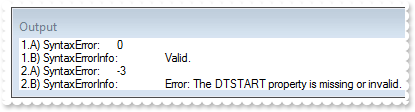
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? "1.A) SyntaxError: "
? oICalendar.RecurPartValue("DTSTART=20151205;FREQ=DAILY;BYDAY=MO",-1)
? "1.B) SyntaxErrorInfo: "
? oICalendar.RecurPartValue("DTSTART=20151205;FREQ=DAILY;BYDAY=MO",-2)
? "2.A) SyntaxError: "
? oICalendar.RecurPartValue("FREQ=DAILY;BYDAY=MO",-1)
? "2.B) SyntaxErrorInfo: "
? oICalendar.RecurPartValue("FREQ=DAILY;BYDAY=MO",-2)
|
65
|
How do I check if the recurrence expression is syntactically correct (method 1)
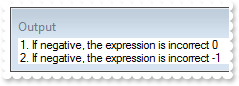
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? "1. If negative, the expression is incorrect"
? oICalendar.RecurCheck("DTSTART=20151205;FREQ=DAILY;BYDAY=MO",{12/05/2015})
? "2. If negative, the expression is incorrect"
? oICalendar.RecurCheck("junk",{01/01/2001})
|
64
|
How do I check if a specified date match the giving recurrence
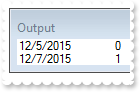
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? {12/05/2015}
? oICalendar.RecurCheck("DTSTART=20151205;FREQ=DAILY;BYDAY=MO",{12/05/2015})
? {12/07/2015}
? oICalendar.RecurCheck("DTSTART=20151205;FREQ=DAILY;BYDAY=MO",{12/07/2015})
|
63
|
Recur: The 2nd to last weekday of the month
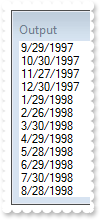
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970929;FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-2",12)
|
62
|
Recur: The 3rd instance into the month of one of Tuesday, Wednesday or Thursday, for the next 3 months

Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970904;FREQ=MONTHLY;COUNT=3;BYDAY=TU,WE,TH;BYSETPOS=3",12)
|
61
|
Recur: The last work day of the month
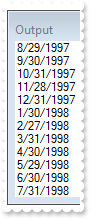
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970805;FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-1",12)
|
60
|
Recur: An example where the days generated makes a difference because of WKST (Sample 2)
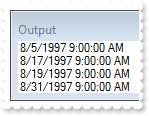
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",12)
|
59
|
Recur: An example where the days generated makes a difference because of WKST (Sample 1)
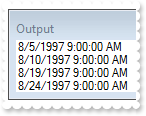
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=MO",12)
|
58
|
Recur: Every 20 minutes from 9:00 AM to 4:40 PM every day
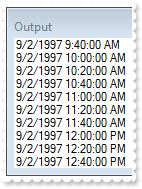
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=DAILY;BYHOUR=9,10,11,12,13,14,15,16;BYMINUTE=0,20,40",12)
|
57
|
Recur: Every hour and a half for 4 occurrences
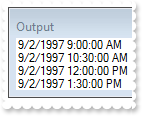
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=MINUTELY;INTERVAL=90;COUNT=4",12)
|
56
|
Recur: Every 15 minutes for 6 occurrences
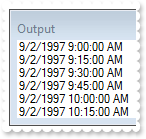
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=MINUTELY;INTERVAL=15;COUNT=6",12)
|
55
|
Recur: Every 3 hours from 9:00 AM to 5:00 PM on a specific day
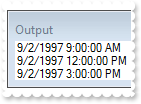
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=HOURLY;INTERVAL=3;UNTIL=19970902T170000Z",12)
|
54
|
Recur: Every four years, the first Tuesday after a Monday in November, forever (U.S. Presidential Election day)
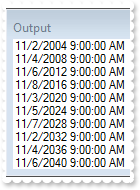
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19961105T090000;FREQ=YEARLY;INTERVAL=4;BYMONTH=11;BYDAY=TU;BYMONTHDAY=2,3,4,5,6,7,8",12)
|
53
|
Recur: The first Saturday that follows the first Sunday of the month, forever
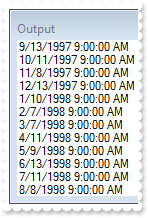
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970913T090000;FREQ=MONTHLY;BYDAY=SA;BYMONTHDAY=7,8,9,10,11,12,13",12)
|
52
|
Recur: Every Friday the 13th, forever
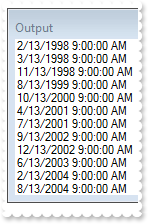
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=MONTHLY;BYDAY=FR;BYMONTHDAY=13",12)
|
51
|
Recur: Every Thursday, but only during June, July, and August, forever
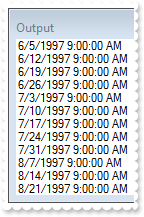
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970605T090000;FREQ=YEARLY;BYDAY=TH;BYMONTH=6,7,8",12)
|
50
|
Recur: Every Thursday in March, forever
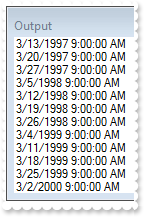
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970313T090000;FREQ=YEARLY;BYMONTH=3;BYDAY=TH",12)
|
49
|
Recur: Monday of week number 20 (where the default start of the week is Monday), forever
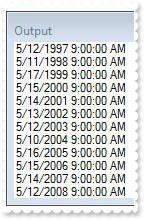
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970512T090000;FREQ=YEARLY;BYWEEKNO=20;BYDAY=MO",12)
|
48
|
Recur: Every 20th Monday of the year, forever
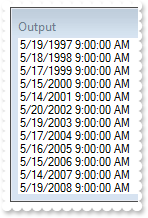
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970101T090000;FREQ=YEARLY;BYDAY=20MO",12)
|
47
|
Recur: Every 3rd year on the 1st, 100th and 200th day for 10 occurrences
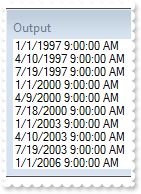
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970101T090000;FREQ=YEARLY;INTERVAL=3;COUNT=10;BYYEARDAY=1,100,200",12)
|
46
|
Recur: Every other year on January, February, and March for 10 occurrences
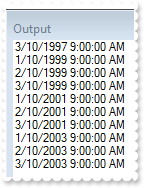
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970310T090000;FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3",12)
|
45
|
Recur: Yearly in June and July for 10 occurrences
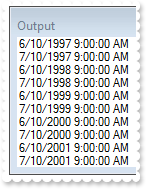
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970610T090000;FREQ=YEARLY;COUNT=10;BYMONTH=6,7",12)
|
44
|
Recur: Every Tuesday, every other month
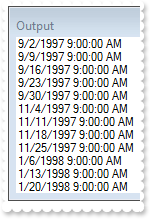
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=MONTHLY;INTERVAL=2;BYDAY=TU",12)
|
43
|
Recur: Every 18 months on the 10th thru 15th of the month for 10 occurrences
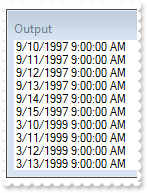
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970910T090000;FREQ=MONTHLY;INTERVAL=18;COUNT=10;BYMONTHDAY=10,11,12,13,14,15",12)
|
42
|
Recur: Monthly on the first and last day of the month for 10 occurrences
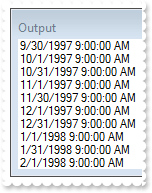
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970930T090000;FREQ=MONTHLY;COUNT=10;BYMONTHDAY=1,-1",12)
|
41
|
Recur: Monthly on the 2nd and 15th of the month for 10 occurrences
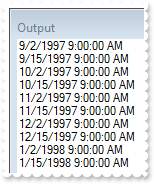
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=MONTHLY;COUNT=10;BYMONTHDAY=2,15",12)
|
40
|
Recur: Monthly on the third to the last day of the month, forever
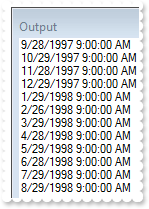
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970928T090000;FREQ=MONTHLY;BYMONTHDAY=-3",12)
|
39
|
Recur: Monthly on the second to last Monday of the month for 6 months
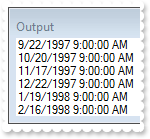
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970922T090000;FREQ=MONTHLY;COUNT=6;BYDAY=-2MO",12)
|
38
|
Recur: Every other month on the 1st and last Sunday of the month for 10 occurrences
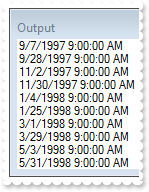
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970907T090000;FREQ=MONTHLY;INTERVAL=2;COUNT=10;BYDAY=1SU,-1SU",12)
|
37
|
Recur: Monthly on the 1st Friday until December 24, 1997
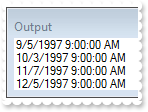
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970905T090000;FREQ=MONTHLY;UNTIL=19971224T000000Z;BYDAY=1FR",12)
|
36
|
Recur: Monthly on the 1st Friday for ten occurrences
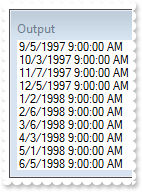
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970905T090000;FREQ=MONTHLY;COUNT=10;BYDAY=1FR",12)
|
35
|
Recur: Every other week on Tuesday and Thursday, for 8 occurrences
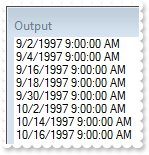
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=8;WKST=SU;BYDAY=TU,TH",12)
|
34
|
Recur: Every other week on Monday, Wednesday and Friday until December 24, 1997, but starting on Tuesday, September 2, 1997
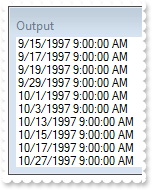
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=WEEKLY;INTERVAL=2;UNTIL=19971224T000000Z;WKST=SU;BYDAY=MO,WE,FR",12)
|
33
|
Recur: Weekly on Tuesday and Thursday for 5 weeks
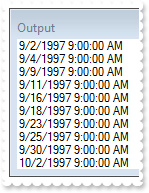
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=WEEKLY;UNTIL=19971007T000000Z;WKST=SU;BYDAY=TU,TH",12)
|
32
|
Recur: Weekly on Tuesday and Thursday for 5 weeks
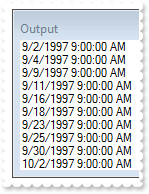
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=WEEKLY;COUNT=10;WKST=SU;BYDAY=TU,TH",12)
|
31
|
Recur: Every other day - forever
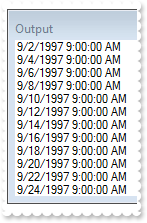
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=DAILY;INTERVAL=2",12)
|
30
|
Recur: Daily until December 24, 1997
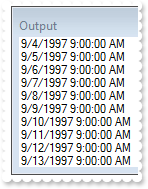
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=DAILY;UNTIL=19971224T000000Z",12)
|
29
|
Recur: Daily for 10 occurrences
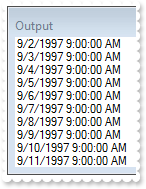
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? oICalendar.RecurAllAsString("DTSTART=19970902T090000;FREQ=DAILY;COUNT=10",12)
|
28
|
How can I add a property of UTC offset type
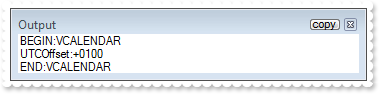
Dim oICalendar as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("UTCOffset","+0100")
? oICalendar.Save()
|
27
|
How can I add a property of URI type
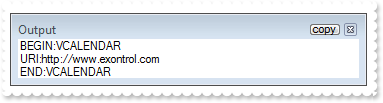
Dim oICalendar as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("URI","http://www.exontrol.com")
? oICalendar.Save()
|
26
|
How can I add a property of time type
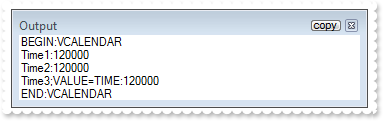
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Time1",{12/30/1899 12:00:00})
var_Component.Properties.Add("Time2",oICalendar.toICalendar(0.5,12))
var_Property = var_Component.Properties.Add("Time3")
var_Property.Value = 0.5
var_Property.Type = 12
? oICalendar.Save()
|
25
|
How can I add a property of text/string type
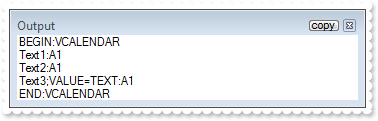
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Text1","A1")
var_Component.Properties.Add("Text2",oICalendar.toICalendar("A1",11))
var_Property = var_Component.Properties.Add("Text3")
var_Property.Value = "A1"
var_Property.Type = 11
? oICalendar.Save()
|
24
|
How can I find properties of recurence type
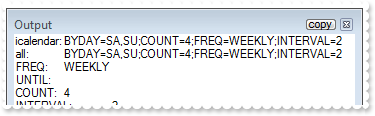
Dim i as
Dim oICalendar as P
Dim p as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Recur","FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=SA,SU")
p = oICalendar.Root.Properties.Item("Recur")
i = oICalendar.toICalendar(p.Value,p.GuessType)
? "icalendar:"
? i
? "all:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"")
? "FREQ:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"FREQ")
? "UNTIL:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"UNTIL")
? "COUNT:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"COUNT")
? "INTERVAL:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"INTERVAL")
? "BYSECOND:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYSECOND")
? "BYMINUTE:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYMINUTE")
? "BYHOUR:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYHOUR")
? "BYDAY:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYDAY")
? "BYMONTHDAY:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYMONTHDAY")
? "BYYEARDAY:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYYEARDAY")
? "BYWEEKNO:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYWEEKNO")
? "BYMONTH:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYMONTH")
? "BYSETPOS:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"BYSETPOS")
? "WKST:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"WKST")
|
23
|
How can I add a property of recurrence type
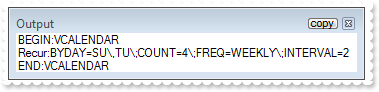
Dim oICalendar as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Recur","FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU")
? oICalendar.Save()
|
22
|
How can I find the duration in weeks, days, hours, minutes, seconds from a property of duration type
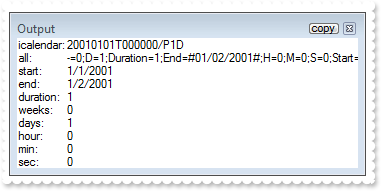
Dim i as
Dim oICalendar as P
Dim p as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Period",oICalendar.valuesToICalendar("Start=#1/1/2001#;Duration=1",9))
p = oICalendar.Root.Properties.Item("Period")
i = oICalendar.toICalendar(p.Value,p.GuessType)
? "icalendar:"
? i
? "all:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"")
? "start:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"Start")
? "end:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"End")
? "duration:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"Duration")
? "weeks:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"W")
? "days:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"D")
? "hour:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"H")
? "min:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"M")
? "sec:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"S")
|
21
|
How can I add a property of period type
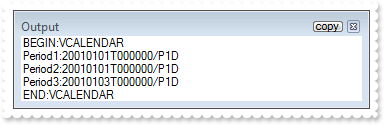
Dim oICalendar as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Period1",oICalendar.valuesToICalendar("Start=#1/1/2001#;Duration=1",9))
var_Component.Properties.Add("Period2",oICalendar.valuesToICalendar("Start=#1/1/2001#;End=#1/2/2001#",9))
var_Component.Properties.Add("Period3",oICalendar.valuesToICalendar("Duration=1;End=#1/2/2001#",9))
? oICalendar.Save()
|
20
|
How can I add a property of integer type
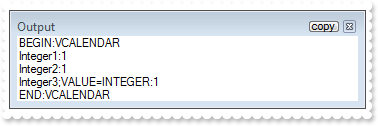
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Integer1",1)
var_Component.Properties.Add("Integer2",oICalendar.toICalendar(1,8))
var_Property = var_Component.Properties.Add("Integer3")
var_Property.Value = 1
var_Property.Type = 8
? oICalendar.Save()
|
19
|
How can I add a property of float type
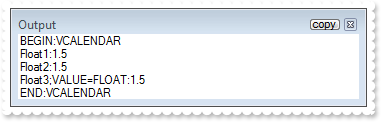
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Float1",1.5)
var_Component.Properties.Add("Float2",oICalendar.toICalendar(1.5,7))
var_Property = var_Component.Properties.Add("Float3")
var_Property.Value = 1.5
var_Property.Type = 7
? oICalendar.Save()
|
18
|
How do I get the type of the property
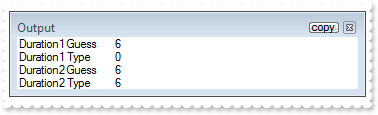
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
Dim var_Property1 as P
Dim var_Property2 as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Duration1",oICalendar.toICalendar(2.5,6))
var_Property = var_Component.Properties.Add("Duration2")
var_Property.Value = 2.5
var_Property.Type = 6
var_Property1 = oICalendar.Root.Properties.Item("Duration1")
? var_Property1.Name
? "Guess"
? var_Property1.GuessType
? var_Property1.Name
? "Type"
? var_Property1.Type
var_Property2 = oICalendar.Root.Properties.Item("Duration2")
? var_Property2.Name
? "Guess"
? var_Property2.GuessType
? var_Property2.Name
? "Type"
? var_Property2.Type
|
17
|
How can I get values of the duration iCalendar format
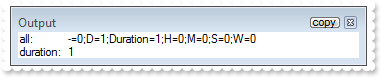
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
? "all:"
? oICalendar.valuesFromICalendar("P1D",6,"")
? "duration:"
? oICalendar.valuesFromICalendar("P1D",6,"Duration")
|
16
|
How can I find the duration in weeks, days, hours, minutes, seconds from a property of duration type
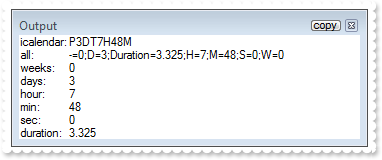
Dim i as
Dim oICalendar as P
Dim p as P
Dim var_Component as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Duration",oICalendar.toICalendar(3.325,6))
p = oICalendar.Root.Properties.Item("Duration")
i = oICalendar.toICalendar(p.Value,p.GuessType)
? "icalendar:"
? i
? "all:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"")
? "duration:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"Duration")
? "weeks:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"W")
? "days:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"D")
? "hour:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"H")
? "min:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"M")
? "sec:"
? oICalendar.valuesFromICalendar(i,p.GuessType,"S")
|
15
|
How can I add a property of duration type
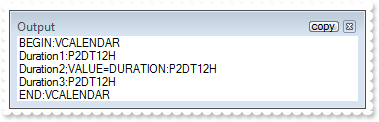
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Duration1",oICalendar.toICalendar(2.5,6))
var_Property = var_Component.Properties.Add("Duration2")
var_Property.Value = 2.5
var_Property.Type = 6
var_Component.Properties.Add("Duration3",oICalendar.valuesToICalendar("D=2;H=12",6))
? oICalendar.Save()
|
14
|
How can I add a property of date-time type
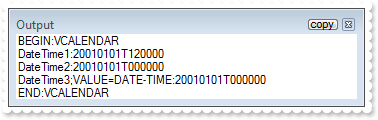
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("DateTime1",{01/01/2001 12:00:00})
var_Component.Properties.Add("DateTime2",oICalendar.toICalendar({01/01/2001},5))
var_Property = var_Component.Properties.Add("DateTime3")
var_Property.Value = {01/01/2001}
var_Property.Type = 5
? oICalendar.Save()
|
13
|
How can I add a property of date type
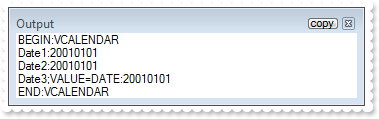
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Date1",{01/01/2001})
var_Component.Properties.Add("Date2",oICalendar.toICalendar({01/01/2001},4))
var_Property = var_Component.Properties.Add("Date3")
var_Property.Value = {01/01/2001}
var_Property.Type = 4
? oICalendar.Save()
|
12
|
How can I add a property of Calendar User Address type
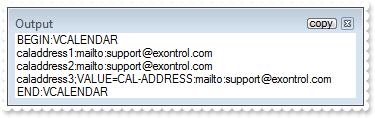
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("caladdress1","mailto:support@exontrol.com")
var_Component.Properties.Add("caladdress2",oICalendar.toICalendar("mailto:support@exontrol.com",3))
var_Property = var_Component.Properties.Add("caladdress3")
var_Property.Value = "mailto:support@exontrol.com"
var_Property.Type = 3
? oICalendar.Save()
|
11
|
How can I add a property of boolean type
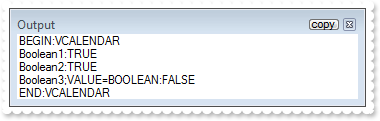
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Boolean1",.t.)
var_Component.Properties.Add("Boolean2",oICalendar.toICalendar("TRUE",2))
var_Property = var_Component.Properties.Add("Boolean3")
var_Property.Value = 0
var_Property.Type = 2
? oICalendar.Save()
|
10
|
How can I add a property of binary type
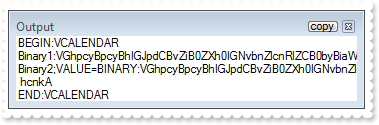
Dim oICalendar as P
Dim var_Component as P
Dim var_Property as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Component.Properties.Add("Binary1",oICalendar.toICalendar("This is a bit of text converted to binary",1))
var_Property = var_Component.Properties.Add("Binary2")
var_Property.Value = "This is a bit of text converted to binary"
var_Property.Type = 1
? oICalendar.Save()
|
9
|
How can I access the root element of the iCalendar format
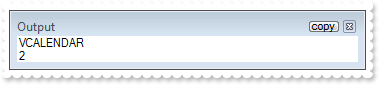
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
oICalendar.Load("BEGIN:VCALENDAR\r\nVERSION:2.0\r\nEND:VCALENDAR")
? oICalendar.Root.Name
? oICalendar.Root.Properties.Item("Version").Value
|
8
|
How can I get notified once the control loads a new component, property, when using Load or LoadFile methods
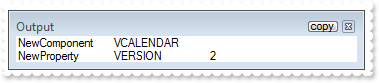
' Occurs when a new component is added.
function AddComponent as v (NewComponent as OLE::Exontrol.ICalendar.1::IComponent)
? NewComponent
end function
' Occurs when a new property is added.
function AddProperty as v (NewPropery as OLE::Exontrol.ICalendar.1::IProperty)
? NewPropery
end function
Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
oICalendar.FireEvents = .t.
oICalendar.Load("BEGIN:VCALENDAR\r\nVERSION:2.0\r\nEND:VCALENDAR")
|
7
|
How can I add a property with parameters
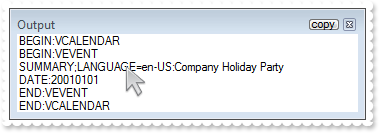
Dim oICalendar as P
Dim var_Component as P
Dim var_Properties as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Properties = var_Component.Components.Add("VEVENT").Properties
var_Properties.Add("SUMMARY","Company Holiday Party").Parameters.Add("LANGUAGE","en-US")
var_Properties.Add("DATE",{01/01/2001})
? oICalendar.Save()
|
6
|
How can I load iCalendar from a string

Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
oICalendar.Load("BEGIN:VCALENDAR\r\nVERSION:2.0\r\nEND:VCALENDAR")
? oICalendar.Content.Components.Item(0).Name
|
5
|
How can I add VEVENT objects
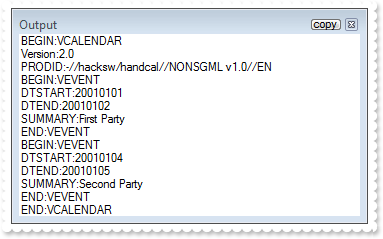
Dim oICalendar as P
Dim var_Component as P
Dim var_Properties as P
Dim var_Properties1 as P
Dim var_Properties2 as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Properties = var_Component.Properties
var_Properties.Add("Version","2.0")
var_Properties.Add("PRODID","-//hacksw/handcal//NONSGML v1.0//EN")
var_Properties1 = var_Component.Components.Add("VEVENT").Properties
var_Properties1.Add("DTSTART",{01/01/2001})
var_Properties1.Add("DTEND",{01/02/2001})
var_Properties1.Add("SUMMARY","First Party")
var_Properties2 = var_Component.Components.Add("VEVENT").Properties
var_Properties2.Add("DTSTART",{01/04/2001})
var_Properties2.Add("DTEND",{01/05/2001})
var_Properties2.Add("SUMMARY","Second Party")
? oICalendar.Save()
|
4
|
How can I save the control's content to iCalendar format, as a file
Dim oICalendar as P
Dim var_Component as P
Dim var_Properties as P
Dim var_Properties1 as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Properties = var_Component.Properties
var_Properties.Add("Version","2.0")
var_Properties.Add("PRODID","-//hacksw/handcal//NONSGML v1.0//EN")
var_Properties1 = var_Component.Components.Add("VEVENT").Properties
var_Properties1.Add("DTSTART",{01/01/2001})
var_Properties1.Add("DTEND",{01/02/2001})
var_Properties1.Add("SUMMARY","Bastille Day Party")
oICalendar.SaveFile("c:/temp/test.ical")
|
3
|
How can I load the iCalendar format from a file

Dim oICalendar as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
oICalendar.LoadFile("c:/temp/test.ical")
? oICalendar.Content.Components.Item("VCALENDAR").Properties.Item("PRODID").Value
|
2
|
How do I export the control's content to iCalendar format
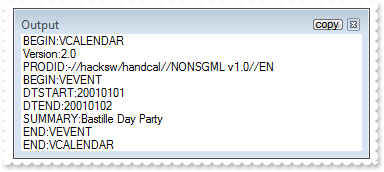
Dim oICalendar as P
Dim var_Component as P
Dim var_Properties as P
Dim var_Properties1 as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Component = oICalendar.Content.Components.Add("VCALENDAR")
var_Properties = var_Component.Properties
var_Properties.Add("Version","2.0")
var_Properties.Add("PRODID","-//hacksw/handcal//NONSGML v1.0//EN")
var_Properties1 = var_Component.Components.Add("VEVENT").Properties
var_Properties1.Add("DTSTART",{01/01/2001})
var_Properties1.Add("DTEND",{01/02/2001})
var_Properties1.Add("SUMMARY","Bastille Day Party")
? oICalendar.Save()
|
1
|
How can I generate a VCALENDAR object
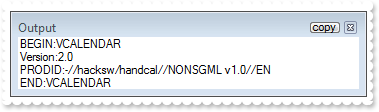
Dim oICalendar as P
Dim var_Properties as P
oICalendar = OLE.Create("Exontrol.ICalendar.1")
var_Properties = oICalendar.Content.Components.Add("VCALENDAR").Properties
var_Properties.Add("Version","2.0")
var_Properties.Add("PRODID","-//hacksw/handcal//NONSGML v1.0//EN")
? oICalendar.Save()
|