69
|
How do I encode data as ICalendar format
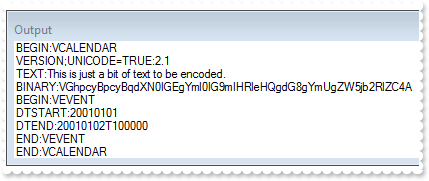
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent();
EXICALENDARLib::IComponentPtr var_Component1 = var_Component->GetComponents()->Add(L"VCALENDAR");
var_Component1->GetProperties()->Add(L"VERSION","2.1")->GetParameters()->Add(L"UNICODE",spICalendar1->GettoICalendar(VARIANT_TRUE,EXICALENDARLib::exPropertyTypeBoolean));
var_Component1->GetProperties()->Add(L"TEXT","This is just a bit of text to be encoded.");
var_Component1->GetProperties()->Add(L"BINARY",spICalendar1->GettoICalendar("This is just a bit of text to be encoded.",EXICALENDARLib::exPropertyTypeBinary));
EXICALENDARLib::IComponentPtr var_Component2 = var_Component1->GetComponents()->Add(L"VEVENT");
var_Component2->GetProperties()->Add(L"DTSTART",COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Component2->GetProperties()->Add(L"DTEND",COleDateTime(2001,1,2,10,00,00).operator DATE());
OutputDebugStringW( spICalendar1->Save() );
|
68
|
How do I get the occurrences between giving start/end margins
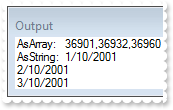
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( L"AsArray: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurRange(L"DTSTART=19970310;FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3",COleDateTime(2001,1,1,0,00,00).operator DATE(),COleDateTime(2002,1,1,0,00,00).operator DATE())) );
OutputDebugStringW( L"AsString: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurRangeAsString(L"DTSTART=19970310;FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3",COleDateTime(2001,1,1,0,00,00).operator DATE(),COleDateTime(2002,1,1,0,00,00).operator DATE())) );
|
67
|
How do I get the value of specified part in the recurrence expression
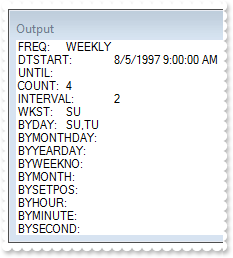
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( L"FREQ: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurFREQ)) );
OutputDebugStringW( L"DTSTART: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurDTSTART)) );
OutputDebugStringW( L"UNTIL: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurUNTIL)) );
OutputDebugStringW( L"COUNT: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurCOUNT)) );
OutputDebugStringW( L"INTERVAL: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurINTERVAL)) );
OutputDebugStringW( L"WKST: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurWKST)) );
OutputDebugStringW( L"BYDAY: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYDAY)) );
OutputDebugStringW( L"BYMONTHDAY: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYMONTHDAY)) );
OutputDebugStringW( L"BYYEARDAY: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYYEARDAY)) );
OutputDebugStringW( L"BYWEEKNO: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYWEEKNO)) );
OutputDebugStringW( L"BYMONTH: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYMONTH)) );
OutputDebugStringW( L"BYSETPOS: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYSETPOS)) );
OutputDebugStringW( L"BYHOUR: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYHOUR)) );
OutputDebugStringW( L"BYMINUTE: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYMINUTE)) );
OutputDebugStringW( L"BYSECOND: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",EXICALENDARLib::exRecurBYSECOND)) );
|
66
|
How do I check if the recurrence expression is syntactically correct (method 2)
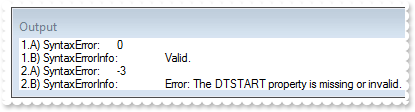
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( L"1.A) SyntaxError: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=20151205;FREQ=DAILY;BYDAY=MO",EXICALENDARLib::exRecurSyntaxError)) );
OutputDebugStringW( L"1.B) SyntaxErrorInfo: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"DTSTART=20151205;FREQ=DAILY;BYDAY=MO",EXICALENDARLib::exRecurSyntaxErrorInfo)) );
OutputDebugStringW( L"2.A) SyntaxError: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"FREQ=DAILY;BYDAY=MO",EXICALENDARLib::exRecurSyntaxError)) );
OutputDebugStringW( L"2.B) SyntaxErrorInfo: " );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurPartValue(L"FREQ=DAILY;BYDAY=MO",EXICALENDARLib::exRecurSyntaxErrorInfo)) );
|
65
|
How do I check if the recurrence expression is syntactically correct (method 1)
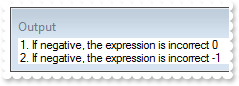
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( L"1. If negative, the expression is incorrect" );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurCheck(L"DTSTART=20151205;FREQ=DAILY;BYDAY=MO",COleDateTime(2015,12,5,0,00,00).operator DATE())) );
OutputDebugStringW( L"2. If negative, the expression is incorrect" );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurCheck(L"junk",COleDateTime(2001,1,1,0,00,00).operator DATE())) );
|
64
|
How do I check if a specified date match the giving recurrence
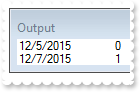
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( L"COleDateTime(2015,12,5,0,00,00).operator DATE()" );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurCheck(L"DTSTART=20151205;FREQ=DAILY;BYDAY=MO",COleDateTime(2015,12,5,0,00,00).operator DATE())) );
OutputDebugStringW( L"COleDateTime(2015,12,7,0,00,00).operator DATE()" );
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurCheck(L"DTSTART=20151205;FREQ=DAILY;BYDAY=MO",COleDateTime(2015,12,7,0,00,00).operator DATE())) );
|
63
|
Recur: The 2nd to last weekday of the month
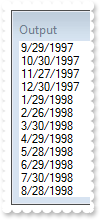
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970929;FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-2",long(12))) );
|
62
|
Recur: The 3rd instance into the month of one of Tuesday, Wednesday or Thursday, for the next 3 months

/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970904;FREQ=MONTHLY;COUNT=3;BYDAY=TU,WE,TH;BYSETPOS=3",long(12))) );
|
61
|
Recur: The last work day of the month
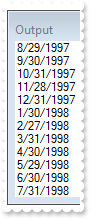
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970805;FREQ=MONTHLY;BYDAY=MO,TU,WE,TH,FR;BYSETPOS=-1",long(12))) );
|
60
|
Recur: An example where the days generated makes a difference because of WKST (Sample 2)
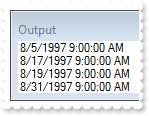
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=SU",long(12))) );
|
59
|
Recur: An example where the days generated makes a difference because of WKST (Sample 1)
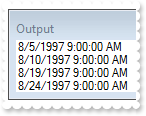
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970805T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU;WKST=MO",long(12))) );
|
58
|
Recur: Every 20 minutes from 9:00 AM to 4:40 PM every day
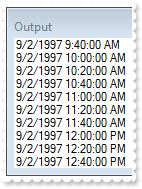
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=DAILY;BYHOUR=9,10,11,12,13,14,15,16;BYMINUTE=0,20,40",long(12))) );
|
57
|
Recur: Every hour and a half for 4 occurrences
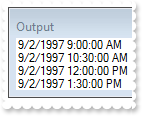
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=MINUTELY;INTERVAL=90;COUNT=4",long(12))) );
|
56
|
Recur: Every 15 minutes for 6 occurrences
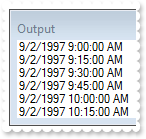
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=MINUTELY;INTERVAL=15;COUNT=6",long(12))) );
|
55
|
Recur: Every 3 hours from 9:00 AM to 5:00 PM on a specific day
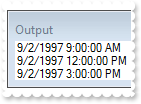
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=HOURLY;INTERVAL=3;UNTIL=19970902T170000Z",long(12))) );
|
54
|
Recur: Every four years, the first Tuesday after a Monday in November, forever (U.S. Presidential Election day)
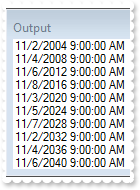
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19961105T090000;FREQ=YEARLY;INTERVAL=4;BYMONTH=11;BYDAY=TU;BYMONTHDAY=2,3,4,5,6,7,8",long(12))) );
|
53
|
Recur: The first Saturday that follows the first Sunday of the month, forever
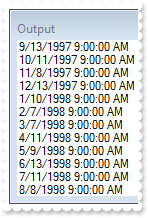
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970913T090000;FREQ=MONTHLY;BYDAY=SA;BYMONTHDAY=7,8,9,10,11,12,13",long(12))) );
|
52
|
Recur: Every Friday the 13th, forever
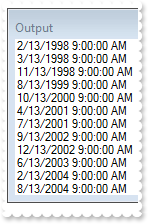
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=MONTHLY;BYDAY=FR;BYMONTHDAY=13",long(12))) );
|
51
|
Recur: Every Thursday, but only during June, July, and August, forever
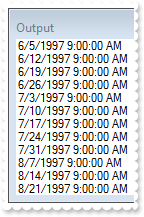
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970605T090000;FREQ=YEARLY;BYDAY=TH;BYMONTH=6,7,8",long(12))) );
|
50
|
Recur: Every Thursday in March, forever
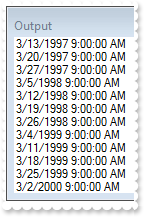
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970313T090000;FREQ=YEARLY;BYMONTH=3;BYDAY=TH",long(12))) );
|
49
|
Recur: Monday of week number 20 (where the default start of the week is Monday), forever
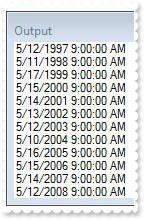
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970512T090000;FREQ=YEARLY;BYWEEKNO=20;BYDAY=MO",long(12))) );
|
48
|
Recur: Every 20th Monday of the year, forever
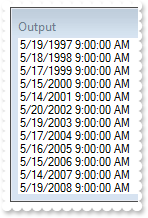
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970101T090000;FREQ=YEARLY;BYDAY=20MO",long(12))) );
|
47
|
Recur: Every 3rd year on the 1st, 100th and 200th day for 10 occurrences
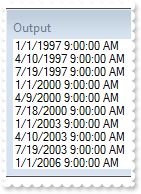
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970101T090000;FREQ=YEARLY;INTERVAL=3;COUNT=10;BYYEARDAY=1,100,200",long(12))) );
|
46
|
Recur: Every other year on January, February, and March for 10 occurrences
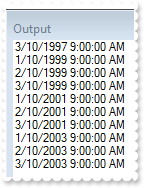
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970310T090000;FREQ=YEARLY;INTERVAL=2;COUNT=10;BYMONTH=1,2,3",long(12))) );
|
45
|
Recur: Yearly in June and July for 10 occurrences
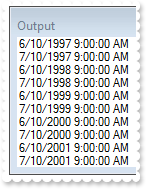
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970610T090000;FREQ=YEARLY;COUNT=10;BYMONTH=6,7",long(12))) );
|
44
|
Recur: Every Tuesday, every other month
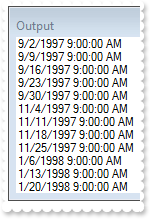
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=MONTHLY;INTERVAL=2;BYDAY=TU",long(12))) );
|
43
|
Recur: Every 18 months on the 10th thru 15th of the month for 10 occurrences
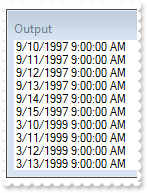
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970910T090000;FREQ=MONTHLY;INTERVAL=18;COUNT=10;BYMONTHDAY=10,11,12,13,14,15",long(12))) );
|
42
|
Recur: Monthly on the first and last day of the month for 10 occurrences
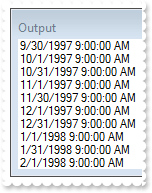
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970930T090000;FREQ=MONTHLY;COUNT=10;BYMONTHDAY=1,-1",long(12))) );
|
41
|
Recur: Monthly on the 2nd and 15th of the month for 10 occurrences
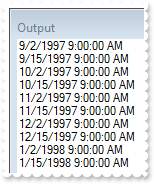
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=MONTHLY;COUNT=10;BYMONTHDAY=2,15",long(12))) );
|
40
|
Recur: Monthly on the third to the last day of the month, forever
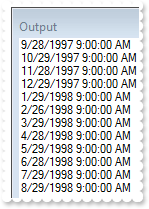
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970928T090000;FREQ=MONTHLY;BYMONTHDAY=-3",long(12))) );
|
39
|
Recur: Monthly on the second to last Monday of the month for 6 months
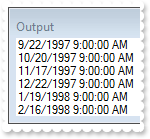
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970922T090000;FREQ=MONTHLY;COUNT=6;BYDAY=-2MO",long(12))) );
|
38
|
Recur: Every other month on the 1st and last Sunday of the month for 10 occurrences
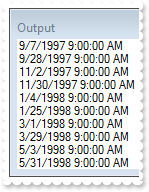
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970907T090000;FREQ=MONTHLY;INTERVAL=2;COUNT=10;BYDAY=1SU,-1SU",long(12))) );
|
37
|
Recur: Monthly on the 1st Friday until December 24, 1997
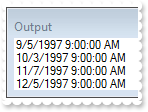
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970905T090000;FREQ=MONTHLY;UNTIL=19971224T000000Z;BYDAY=1FR",long(12))) );
|
36
|
Recur: Monthly on the 1st Friday for ten occurrences
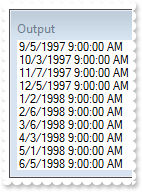
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970905T090000;FREQ=MONTHLY;COUNT=10;BYDAY=1FR",long(12))) );
|
35
|
Recur: Every other week on Tuesday and Thursday, for 8 occurrences
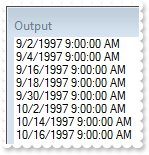
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=WEEKLY;INTERVAL=2;COUNT=8;WKST=SU;BYDAY=TU,TH",long(12))) );
|
34
|
Recur: Every other week on Monday, Wednesday and Friday until December 24, 1997, but starting on Tuesday, September 2, 1997
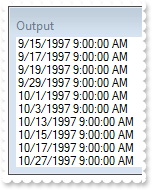
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=WEEKLY;INTERVAL=2;UNTIL=19971224T000000Z;WKST=SU;BYDAY=MO,WE,FR",long(12))) );
|
33
|
Recur: Weekly on Tuesday and Thursday for 5 weeks
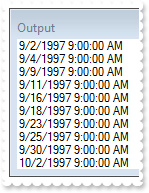
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=WEEKLY;UNTIL=19971007T000000Z;WKST=SU;BYDAY=TU,TH",long(12))) );
|
32
|
Recur: Weekly on Tuesday and Thursday for 5 weeks
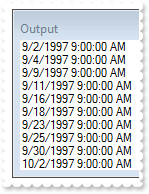
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=WEEKLY;COUNT=10;WKST=SU;BYDAY=TU,TH",long(12))) );
|
31
|
Recur: Every other day - forever
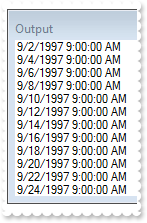
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=DAILY;INTERVAL=2",long(12))) );
|
30
|
Recur: Daily until December 24, 1997
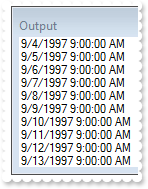
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=DAILY;UNTIL=19971224T000000Z",long(12))) );
|
29
|
Recur: Daily for 10 occurrences
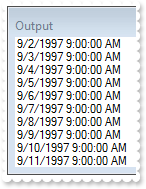
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( _bstr_t(spICalendar1->GetRecurAllAsString(L"DTSTART=19970902T090000;FREQ=DAILY;COUNT=10",long(12))) );
|
28
|
How can I add a property of UTC offset type
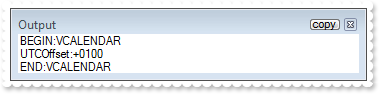
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"UTCOffset","+0100");
OutputDebugStringW( spICalendar1->Save() );
|
27
|
How can I add a property of URI type
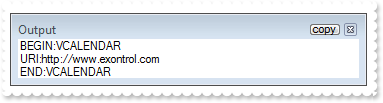
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"URI","http://www.exontrol.com");
OutputDebugStringW( spICalendar1->Save() );
|
26
|
How can I add a property of time type
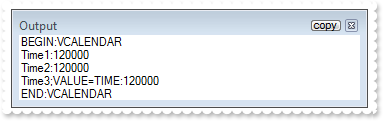
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Time1",COleDateTime(1899,12,30,12,00,00).operator DATE());
var_Component->GetProperties()->Add(L"Time2",spICalendar1->GettoICalendar(double(0.5),EXICALENDARLib::exPropertyTypeTime));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Time3",vtMissing);
var_Property->PutValue(double(0.5));
var_Property->PutType(EXICALENDARLib::exPropertyTypeTime);
OutputDebugStringW( spICalendar1->Save() );
|
25
|
How can I add a property of text/string type
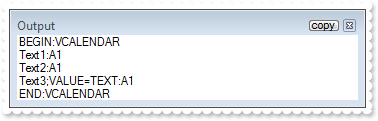
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Text1","A1");
var_Component->GetProperties()->Add(L"Text2",spICalendar1->GettoICalendar("A1",EXICALENDARLib::exPropertyTypeText));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Text3",vtMissing);
var_Property->PutValue("A1");
var_Property->PutType(EXICALENDARLib::exPropertyTypeText);
OutputDebugStringW( spICalendar1->Save() );
|
24
|
How can I find properties of recurence type
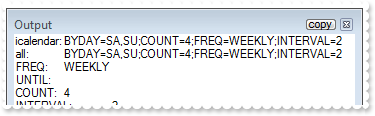
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Recur","FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=SA,SU");
EXICALENDARLib::IPropertyPtr p = spICalendar1->GetRoot()->GetProperties()->GetItem("Recur");
_bstr_t i = spICalendar1->GettoICalendar(p->GetValue(),p->GetGuessType());
OutputDebugStringW( L"icalendar:" );
OutputDebugStringW( L"i" );
OutputDebugStringW( L"all:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"")) );
OutputDebugStringW( L"FREQ:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"FREQ")) );
OutputDebugStringW( L"UNTIL:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"UNTIL")) );
OutputDebugStringW( L"COUNT:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"COUNT")) );
OutputDebugStringW( L"INTERVAL:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"INTERVAL")) );
OutputDebugStringW( L"BYSECOND:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYSECOND")) );
OutputDebugStringW( L"BYMINUTE:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYMINUTE")) );
OutputDebugStringW( L"BYHOUR:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYHOUR")) );
OutputDebugStringW( L"BYDAY:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYDAY")) );
OutputDebugStringW( L"BYMONTHDAY:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYMONTHDAY")) );
OutputDebugStringW( L"BYYEARDAY:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYYEARDAY")) );
OutputDebugStringW( L"BYWEEKNO:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYWEEKNO")) );
OutputDebugStringW( L"BYMONTH:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYMONTH")) );
OutputDebugStringW( L"BYSETPOS:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"BYSETPOS")) );
OutputDebugStringW( L"WKST:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"WKST")) );
|
23
|
How can I add a property of recurrence type
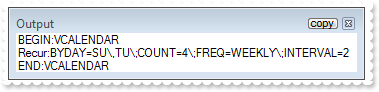
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Recur","FREQ=WEEKLY;INTERVAL=2;COUNT=4;BYDAY=TU,SU");
OutputDebugStringW( spICalendar1->Save() );
|
22
|
How can I find the duration in weeks, days, hours, minutes, seconds from a property of duration type
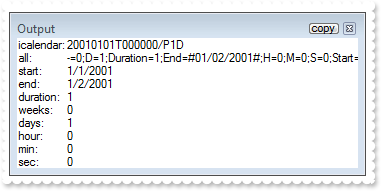
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Period",spICalendar1->GetvaluesToICalendar(L"Start=#1/1/2001#;Duration=1",EXICALENDARLib::exPropertyTypePeriod));
EXICALENDARLib::IPropertyPtr p = spICalendar1->GetRoot()->GetProperties()->GetItem("Period");
_bstr_t i = spICalendar1->GettoICalendar(p->GetValue(),p->GetGuessType());
OutputDebugStringW( L"icalendar:" );
OutputDebugStringW( L"i" );
OutputDebugStringW( L"all:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"")) );
OutputDebugStringW( L"start:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"Start")) );
OutputDebugStringW( L"end:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"End")) );
OutputDebugStringW( L"duration:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"Duration")) );
OutputDebugStringW( L"weeks:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"W")) );
OutputDebugStringW( L"days:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"D")) );
OutputDebugStringW( L"hour:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"H")) );
OutputDebugStringW( L"min:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"M")) );
OutputDebugStringW( L"sec:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"S")) );
|
21
|
How can I add a property of period type
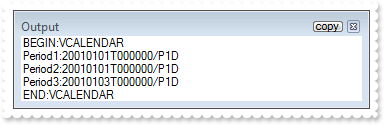
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Period1",spICalendar1->GetvaluesToICalendar(L"Start=#1/1/2001#;Duration=1",EXICALENDARLib::exPropertyTypePeriod));
var_Component->GetProperties()->Add(L"Period2",spICalendar1->GetvaluesToICalendar(L"Start=#1/1/2001#;End=#1/2/2001#",EXICALENDARLib::exPropertyTypePeriod));
var_Component->GetProperties()->Add(L"Period3",spICalendar1->GetvaluesToICalendar(L"Duration=1;End=#1/2/2001#",EXICALENDARLib::exPropertyTypePeriod));
OutputDebugStringW( spICalendar1->Save() );
|
20
|
How can I add a property of integer type
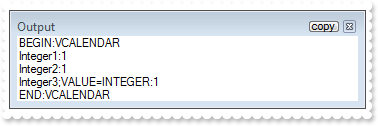
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Integer1",long(1));
var_Component->GetProperties()->Add(L"Integer2",spICalendar1->GettoICalendar(long(1),EXICALENDARLib::exPropertyTypeInteger));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Integer3",vtMissing);
var_Property->PutValue(long(1));
var_Property->PutType(EXICALENDARLib::exPropertyTypeInteger);
OutputDebugStringW( spICalendar1->Save() );
|
19
|
How can I add a property of float type
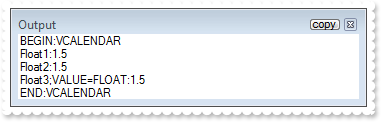
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Float1",double(1.5));
var_Component->GetProperties()->Add(L"Float2",spICalendar1->GettoICalendar(double(1.5),EXICALENDARLib::exPropertyTypeFloat));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Float3",vtMissing);
var_Property->PutValue(double(1.5));
var_Property->PutType(EXICALENDARLib::exPropertyTypeFloat);
OutputDebugStringW( spICalendar1->Save() );
|
18
|
How do I get the type of the property
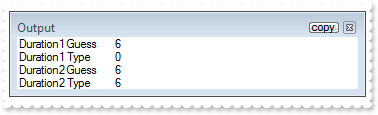
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Duration1",spICalendar1->GettoICalendar(double(2.5),EXICALENDARLib::exPropertyTypeDuration));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Duration2",vtMissing);
var_Property->PutValue(double(2.5));
var_Property->PutType(EXICALENDARLib::exPropertyTypeDuration);
EXICALENDARLib::IPropertyPtr var_Property1 = spICalendar1->GetRoot()->GetProperties()->GetItem("Duration1");
OutputDebugStringW( var_Property1->GetName() );
OutputDebugStringW( L"Guess" );
OutputDebugStringW( _bstr_t(var_Property1->GetGuessType()) );
OutputDebugStringW( var_Property1->GetName() );
OutputDebugStringW( L"Type" );
OutputDebugStringW( _bstr_t(var_Property1->GetType()) );
EXICALENDARLib::IPropertyPtr var_Property2 = spICalendar1->GetRoot()->GetProperties()->GetItem("Duration2");
OutputDebugStringW( var_Property2->GetName() );
OutputDebugStringW( L"Guess" );
OutputDebugStringW( _bstr_t(var_Property2->GetGuessType()) );
OutputDebugStringW( var_Property2->GetName() );
OutputDebugStringW( L"Type" );
OutputDebugStringW( _bstr_t(var_Property2->GetType()) );
|
17
|
How can I get values of the duration iCalendar format
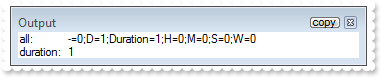
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
OutputDebugStringW( L"all:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"P1D",EXICALENDARLib::exPropertyTypeDuration,L"")) );
OutputDebugStringW( L"duration:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"P1D",EXICALENDARLib::exPropertyTypeDuration,L"Duration")) );
|
16
|
How can I find the duration in weeks, days, hours, minutes, seconds from a property of duration type
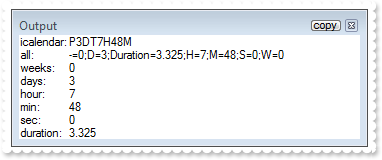
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Duration",spICalendar1->GettoICalendar(double(3.325),EXICALENDARLib::exPropertyTypeDuration));
EXICALENDARLib::IPropertyPtr p = spICalendar1->GetRoot()->GetProperties()->GetItem("Duration");
_bstr_t i = spICalendar1->GettoICalendar(p->GetValue(),p->GetGuessType());
OutputDebugStringW( L"icalendar:" );
OutputDebugStringW( L"i" );
OutputDebugStringW( L"all:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"")) );
OutputDebugStringW( L"duration:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"Duration")) );
OutputDebugStringW( L"weeks:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"W")) );
OutputDebugStringW( L"days:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"D")) );
OutputDebugStringW( L"hour:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"H")) );
OutputDebugStringW( L"min:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"M")) );
OutputDebugStringW( L"sec:" );
OutputDebugStringW( _bstr_t(spICalendar1->GetvaluesFromICalendar(L"i",p->GetGuessType(),L"S")) );
|
15
|
How can I add a property of duration type
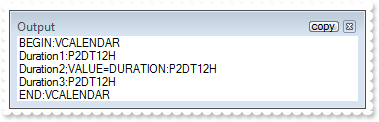
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Duration1",spICalendar1->GettoICalendar(double(2.5),EXICALENDARLib::exPropertyTypeDuration));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Duration2",vtMissing);
var_Property->PutValue(double(2.5));
var_Property->PutType(EXICALENDARLib::exPropertyTypeDuration);
var_Component->GetProperties()->Add(L"Duration3",spICalendar1->GetvaluesToICalendar(L"D=2;H=12",EXICALENDARLib::exPropertyTypeDuration));
OutputDebugStringW( spICalendar1->Save() );
|
14
|
How can I add a property of date-time type
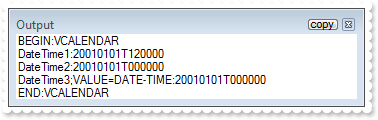
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"DateTime1",COleDateTime(2001,1,1,12,00,00).operator DATE());
var_Component->GetProperties()->Add(L"DateTime2",spICalendar1->GettoICalendar(COleDateTime(2001,1,1,0,00,00).operator DATE(),EXICALENDARLib::exPropertyTypeDateTime));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"DateTime3",vtMissing);
var_Property->PutValue(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Property->PutType(EXICALENDARLib::exPropertyTypeDateTime);
OutputDebugStringW( spICalendar1->Save() );
|
13
|
How can I add a property of date type
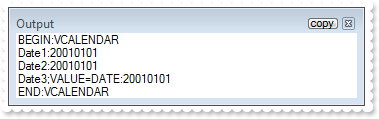
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Date1",COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Component->GetProperties()->Add(L"Date2",spICalendar1->GettoICalendar(COleDateTime(2001,1,1,0,00,00).operator DATE(),EXICALENDARLib::exPropertyTypeDate));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Date3",vtMissing);
var_Property->PutValue(COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Property->PutType(EXICALENDARLib::exPropertyTypeDate);
OutputDebugStringW( spICalendar1->Save() );
|
12
|
How can I add a property of Calendar User Address type
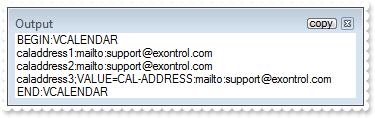
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"caladdress1","mailto:support@exontrol.com");
var_Component->GetProperties()->Add(L"caladdress2",spICalendar1->GettoICalendar("mailto:support@exontrol.com",EXICALENDARLib::exPropertyTypeCalAddress));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"caladdress3",vtMissing);
var_Property->PutValue("mailto:support@exontrol.com");
var_Property->PutType(EXICALENDARLib::exPropertyTypeCalAddress);
OutputDebugStringW( spICalendar1->Save() );
|
11
|
How can I add a property of boolean type
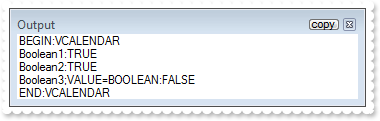
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Boolean1",VARIANT_TRUE);
var_Component->GetProperties()->Add(L"Boolean2",spICalendar1->GettoICalendar("TRUE",EXICALENDARLib::exPropertyTypeBoolean));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Boolean3",vtMissing);
var_Property->PutValue(long(0));
var_Property->PutType(EXICALENDARLib::exPropertyTypeBoolean);
OutputDebugStringW( spICalendar1->Save() );
|
10
|
How can I add a property of binary type
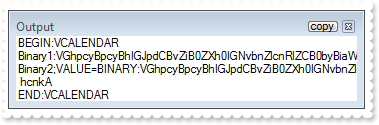
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
var_Component->GetProperties()->Add(L"Binary1",spICalendar1->GettoICalendar("This is a bit of text converted to binary",EXICALENDARLib::exPropertyTypeBinary));
EXICALENDARLib::IPropertyPtr var_Property = var_Component->GetProperties()->Add(L"Binary2",vtMissing);
var_Property->PutValue("This is a bit of text converted to binary");
var_Property->PutType(EXICALENDARLib::exPropertyTypeBinary);
OutputDebugStringW( spICalendar1->Save() );
|
9
|
How can I access the root element of the iCalendar format
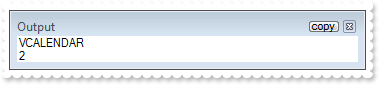
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
spICalendar1->Load(L"BEGIN:VCALENDAR\\r\\nVERSION:2.0\\r\\nEND:VCALENDAR");
OutputDebugStringW( spICalendar1->GetRoot()->GetName() );
OutputDebugStringW( _bstr_t(spICalendar1->GetRoot()->GetProperties()->GetItem("Version")->GetValue()) );
|
8
|
How can I get notified once the control loads a new component, property, when using Load or LoadFile methods
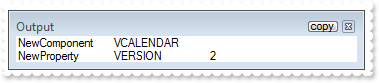
// AddComponent event - Occurs when a new component is added.
void OnAddComponentICalendar1(LPDISPATCH NewComponent)
{
OutputDebugStringW( L"NewComponent" );
}
// AddProperty event - Occurs when a new property is added.
void OnAddPropertyICalendar1(LPDISPATCH NewPropery)
{
OutputDebugStringW( L"NewPropery" );
}
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
spICalendar1->PutFireEvents(VARIANT_TRUE);
spICalendar1->Load(L"BEGIN:VCALENDAR\\r\\nVERSION:2.0\\r\\nEND:VCALENDAR");
|
7
|
How can I add a property with parameters
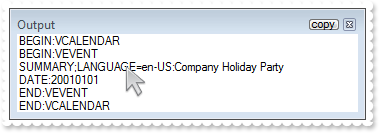
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
EXICALENDARLib::IPropertiesPtr var_Properties = var_Component->GetComponents()->Add(L"VEVENT")->GetProperties();
var_Properties->Add(L"SUMMARY","Company Holiday Party")->GetParameters()->Add(L"LANGUAGE","en-US");
var_Properties->Add(L"DATE",COleDateTime(2001,1,1,0,00,00).operator DATE());
OutputDebugStringW( spICalendar1->Save() );
|
6
|
How can I load iCalendar from a string

/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
spICalendar1->Load(L"BEGIN:VCALENDAR\\r\\nVERSION:2.0\\r\\nEND:VCALENDAR");
OutputDebugStringW( spICalendar1->GetContent()->GetComponents()->GetItem(long(0))->GetName() );
|
5
|
How can I add VEVENT objects
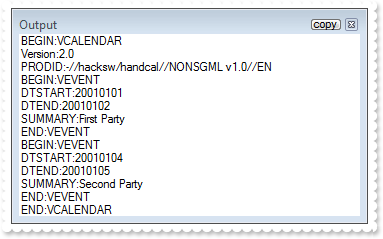
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
EXICALENDARLib::IPropertiesPtr var_Properties = var_Component->GetProperties();
var_Properties->Add(L"Version","2.0");
var_Properties->Add(L"PRODID","-//hacksw/handcal//NONSGML v1.0//EN");
EXICALENDARLib::IPropertiesPtr var_Properties1 = var_Component->GetComponents()->Add(L"VEVENT")->GetProperties();
var_Properties1->Add(L"DTSTART",COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Properties1->Add(L"DTEND",COleDateTime(2001,1,2,0,00,00).operator DATE());
var_Properties1->Add(L"SUMMARY","First Party");
EXICALENDARLib::IPropertiesPtr var_Properties2 = var_Component->GetComponents()->Add(L"VEVENT")->GetProperties();
var_Properties2->Add(L"DTSTART",COleDateTime(2001,1,4,0,00,00).operator DATE());
var_Properties2->Add(L"DTEND",COleDateTime(2001,1,5,0,00,00).operator DATE());
var_Properties2->Add(L"SUMMARY","Second Party");
OutputDebugStringW( spICalendar1->Save() );
|
4
|
How can I save the control's content to iCalendar format, as a file
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
EXICALENDARLib::IPropertiesPtr var_Properties = var_Component->GetProperties();
var_Properties->Add(L"Version","2.0");
var_Properties->Add(L"PRODID","-//hacksw/handcal//NONSGML v1.0//EN");
EXICALENDARLib::IPropertiesPtr var_Properties1 = var_Component->GetComponents()->Add(L"VEVENT")->GetProperties();
var_Properties1->Add(L"DTSTART",COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Properties1->Add(L"DTEND",COleDateTime(2001,1,2,0,00,00).operator DATE());
var_Properties1->Add(L"SUMMARY","Bastille Day Party");
spICalendar1->SaveFile(L"c:/temp/test.ical");
|
3
|
How can I load the iCalendar format from a file

/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
spICalendar1->LoadFile(L"c:/temp/test.ical");
OutputDebugStringW( _bstr_t(spICalendar1->GetContent()->GetComponents()->GetItem("VCALENDAR")->GetProperties()->GetItem("PRODID")->GetValue()) );
|
2
|
How do I export the control's content to iCalendar format
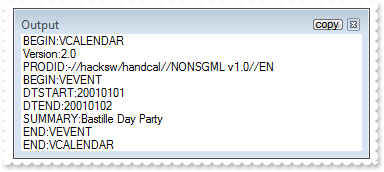
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IComponentPtr var_Component = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR");
EXICALENDARLib::IPropertiesPtr var_Properties = var_Component->GetProperties();
var_Properties->Add(L"Version","2.0");
var_Properties->Add(L"PRODID","-//hacksw/handcal//NONSGML v1.0//EN");
EXICALENDARLib::IPropertiesPtr var_Properties1 = var_Component->GetComponents()->Add(L"VEVENT")->GetProperties();
var_Properties1->Add(L"DTSTART",COleDateTime(2001,1,1,0,00,00).operator DATE());
var_Properties1->Add(L"DTEND",COleDateTime(2001,1,2,0,00,00).operator DATE());
var_Properties1->Add(L"SUMMARY","Bastille Day Party");
OutputDebugStringW( spICalendar1->Save() );
|
1
|
How can I generate a VCALENDAR object
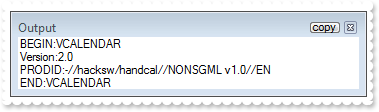
/*
Includes the definition for CreateObject function like follows:
#include <comdef.h>
IUnknownPtr CreateObject( BSTR Object )
{
IUnknownPtr spResult;
spResult.CreateInstance( Object );
return spResult;
};
*/
/*
Copy and paste the following directives to your header file as
it defines the namespace 'EXICALENDARLib' for the library: 'ICalendar 1.0 Type Library'
#import <ExICalendar.dll>
using namespace EXICALENDARLib;
*/
EXICALENDARLib::IICalendarPtr spICalendar1 = ::CreateObject(L"Exontrol.ICalendar.1");
EXICALENDARLib::IPropertiesPtr var_Properties = spICalendar1->GetContent()->GetComponents()->Add(L"VCALENDAR")->GetProperties();
var_Properties->Add(L"Version","2.0");
var_Properties->Add(L"PRODID","-//hacksw/handcal//NONSGML v1.0//EN");
OutputDebugStringW( spICalendar1->Save() );
|