205
|
Is it possible to show just expressions
public void init()
{
;
super();
exedit1.AddWild("<fgcolor=00FF00>(");
exedit1.AddWild("<fgcolor=00FF00>)");
exedit1.AddExpression("<fgcolor=FF0000><b>(*","<fgcolor=FF0000> ","<fgcolor=FF0000><b>*)");
exedit1.InsertText("some text ( another text ) other text\\r\\n",COMVariant::createFromInt(1));
exedit1.InsertText("some text (* another text *) other text\\r\\n",COMVariant::createFromInt(1));
exedit1.Show("expression");
}
|
204
|
How can I stop any highlight
public void init()
{
;
super();
exedit1.AddWild("<fgcolor=00FF00>(");
exedit1.AddWild("<fgcolor=00FF00>)");
exedit1.AddExpression("<fgcolor=FF0000><b>(*","<fgcolor=FF0000> ","<fgcolor=FF0000><b>*)");
exedit1.InsertText("some text ( another text ) other text\\r\\n",COMVariant::createFromInt(1));
exedit1.InsertText("some text (* another text *) other text\\r\\n",COMVariant::createFromInt(1));
exedit1.Show("");
}
|
203
|
How can I highlight the start of the line until a specified character is found
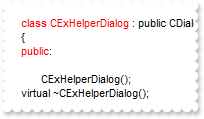
public void init()
{
;
super();
exedit1.AddExpression("^","<fgcolor=FF0000> ",":");
exedit1.Refresh();
}
|
202
|
Can I use code completion without any UI
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
exedit1.CodeCompletion(1/*exCodeCompletionEnableNoUI*/);
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("class");
}
|
201
|
How can I hide the control's horizontal scroll bar
public void init()
{
;
super();
exedit1.ScrollBars(2/*exVertical*/);
}
|
200
|
Is it possible to change the line's height
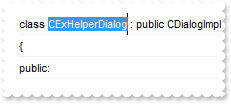
public void init()
{
;
super();
exedit1.LineHeight("value + 8 * dpi");
exedit1.DrawGridLines(true);
}
|
199
|
How to bold everything between two * (asterisk) characters

public void init()
{
COM com_StdFont;
anytype var_StdFont;
;
super();
var_StdFont = exedit1.Font(); com_StdFont = var_StdFont;
com_StdFont.Name("Consolas");
com_StdFont.Size(12);
exedit1.AddExpression("<fgcolor=FF0000><b>*","<fgcolor=FF0000> ","<fgcolor=FF0000><b>*");
exedit1.InsertText("some text * another text * other text\\r\\n",COMVariant::createFromInt(1));
exedit1.Refresh();
}
|
198
|
How to bold everything that starts with * (asterisk), to the end of the line

public void init()
{
COM com_StdFont;
anytype var_StdFont;
;
super();
var_StdFont = exedit1.Font(); com_StdFont = var_StdFont;
com_StdFont.Name("Consolas");
com_StdFont.Size(12);
exedit1.AddWild("<fgcolor=FF0000><b>\\**");
exedit1.InsertText("some text * another text * other text\\r\\n",COMVariant::createFromInt(1));
exedit1.Refresh();
}
|
197
|
How to make a * (asterisk) bold, not the entire / rest line

public void init()
{
COM com_StdFont;
anytype var_StdFont;
;
super();
var_StdFont = exedit1.Font(); com_StdFont = var_StdFont;
com_StdFont.Name("Consolas");
com_StdFont.Size(12);
exedit1.AddWild("<fgcolor=FF0000><b>\\*");
exedit1.InsertText("some text * another text * other text\\r\\n",COMVariant::createFromInt(1));
exedit1.Refresh();
}
|
196
|
How can I change the control's font (template)
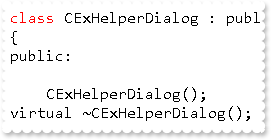
public void init()
{
;
super();
exedit1.Template("Font { Name = `Consolas`; Size = 12 }");
exedit1.AddKeyword("<fgcolor=FF0000>class</fgcolor>");
exedit1.Refresh();
}
|
195
|
How can I change the control's font (runtime)
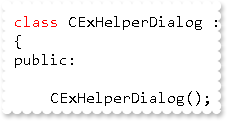
public void init()
{
COM com_StdFont;
anytype var_StdFont;
;
super();
var_StdFont = exedit1.Font(); com_StdFont = var_StdFont;
com_StdFont.Name("Consolas");
com_StdFont.Size(12);
exedit1.AddKeyword("<fgcolor=FF0000>class</fgcolor>");
exedit1.Refresh();
}
|
194
|
When I click and drag to try and select some text, sometimes my cursor turns into a hand and drags the whole text in the window around. I would like to disable this feature, could you tell me what it is called so I can disable it please
public void init()
{
;
super();
exedit1.OLEDropMode(-1/*exOLEDropAutomatic*/);
}
|
193
|
How can I display information about events the control fires
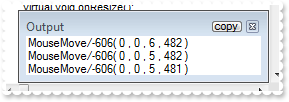
// Event event - Notifies the application once the control fires an event.
void onEvent_Event(int _EventID)
{
;
print( exedit1.EventParam(-2) );
}
public void init()
{
str var_s;
;
super();
exedit1.AddWild("<fgcolor=808080>(?*)</fgcolor>");
var_s = "a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality";
exedit1.AddKeyword("<b>class</b>",COMVariant::createFromStr(var_s));
exedit1.Refresh();
}
|
192
|
How do I highlights words based on wild characters
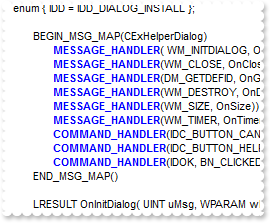
public void init()
{
;
super();
exedit1.AddWild("<fgcolor=0000FF><b>[MC]*_HANDLER*</b></fgcolor>(*)");
exedit1.Refresh();
}
|
191
|
How do I highlights words based on wild characters
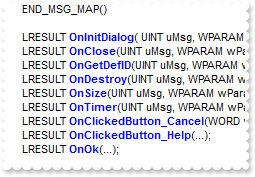
public void init()
{
;
super();
exedit1.AddWild("<fgcolor=0000FF><b> *</b></fgcolor>(*)*;");
exedit1.Refresh();
}
|
190
|
How can I provide different tooltip for the same keyword
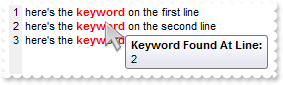
// QueryContext event - Queries for the context at the specified location, to provide different tooltips for the same keyword on QueryContextToolTip event.
void onEvent_QueryContext(int _XCursor,int _YCursor,COMVariant /*variant*/ _QContext)
{
;
_QContext = _YCursor;
}
// QueryContextToolTip event - Asks for the tooltip/title of the keyword on the context retrieved by the QueryContext event.
void onEvent_QueryContextToolTip(str _QContext,str _Keyword,COMVariant /*variant*/ _QToolTip,COMVariant /*variant*/ _QToolTipTitle)
{
;
_QToolTip = _QContext;
_QToolTipTitle = "Keyword Found At Line:";
}
public void init()
{
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.AddKeyword("<fgcolor=FF0000><b>keyword</b></fgcolor>");
exedit1.Text("");
exedit1.InsertText("here's the keyword on the first line");
exedit1.InsertText("\\r\\nhere's the keyword on the second line");
exedit1.InsertText("\\r\\nhere's the keyword on the third line");
}
|
189
|
Is it possible to left, right or center align the inline tooltip
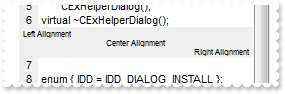
public void init()
{
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.CaretLine(6);
exedit1.Background(160/*exTempInlineToolTipBackColor*/,WinApi::RGB2int(240,240,240));
exedit1.TempInlineToolTip("<font ;6>Left Alignment<br><c>Center Alignment<br><r>Right Alignment");
exedit1.Refresh();
}
|
188
|
Is it possible to display the inline tooltip with a different appearance than temporarily inline tooltip
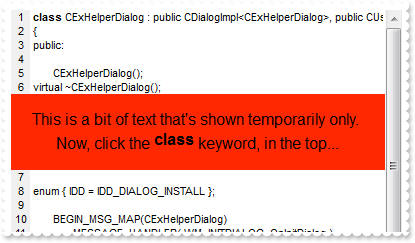
public void init()
{
str var_s,var_s1;
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
var_s = "<r>a set or category of things having some property or attribute in common and differentiated from others by kind, type, or qual";
var_s = var_s + "ity.";
exedit1.AddKeyword("<b>class</b>",COMVariant::createFromStr(var_s));
exedit1.AllowInlineToolTip(513/*exInlineToolTipWordWrap | exInlineToolTip*/);
exedit1.Background(159/*exInlineToolTipForeColor*/,WinApi::RGB2int(128,128,128));
exedit1.Background(158/*exInlineToolTipBackColor*/,WinApi::RGB2int(240,240,240));
exedit1.CaretLine(6);
exedit1.Background(160/*exTempInlineToolTipBackColor*/,WinApi::RGB2int(255,40,0));
exedit1.Background(161/*exTempInlineToolTipForeColor*/,WinApi::RGB2int(0,0,1));
var_s1 = "<br><c><font ;12>This is a bit of text that's shown temporarily only. <br><c>Now, click the <off -4><b>class</b></off> keyword, ";
var_s1 = var_s1 + "in the top...<br>";
exedit1.TempInlineToolTip(var_s1);
exedit1.Refresh();
}
|
187
|
How can I display the inline tooltip over the lines, instead pushing the lines
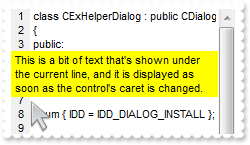
public void init()
{
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.Background(160/*exTempInlineToolTipBackColor*/,WinApi::RGB2int(255,255,0));
exedit1.CaretLine(3);
exedit1.TempInlineToolTip("This is a bit of text that's shown under the current line, and it is displayed as soon as the control's caret is changed.");
exedit1.AllowInlineToolTip(768/*exInlineToolTipWordWrap | exInlineToolTipOver*/);
exedit1.Refresh();
}
|
186
|
Is it possible to display the inline tooltip all the time
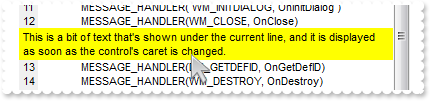
// SelChange event - Occurs when the user selects text in the control.
void onEvent_SelChange()
{
;
exedit1.TempInlineToolTip("This is a bit of text that's shown under the current line, and it is displayed as soon as the control's caret is changed.");
}
public void init()
{
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.Background(160/*exTempInlineToolTipBackColor*/,WinApi::RGB2int(255,255,0));
exedit1.CaretLine(12);
exedit1.AllowInlineToolTip(512/*exInlineToolTipWordWrap*/);
exedit1.Refresh();
}
|
185
|
Is it possible to display images in the inline tooltip
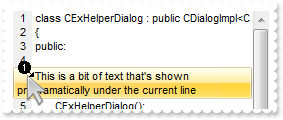
public void init()
{
str var_s;
;
super();
exedit1.VisualAppearance().Add(1,"c:\\exontrol\\images\\normal.ebn");
var_s = "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql";
var_s = var_s + "Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0";
var_s = var_s + "ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN";
var_s = var_s + "AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=";
exedit1.Images(COMVariant::createFromStr(var_s));
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.CaretLine(4);
exedit1.Background(160/*exTempInlineToolTipBackColor*/,0x1000000);
exedit1.AllowInlineToolTip(512/*exInlineToolTipWordWrap*/);
exedit1.TempInlineToolTip("<img>1</img>This is a bit of text that's shown programatically under the current line");
}
|
184
|
How can I change the visual appearance of the temporarily inline tooltip
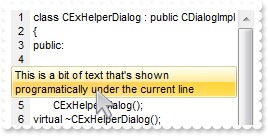
public void init()
{
;
super();
exedit1.VisualAppearance().Add(1,"c:\\exontrol\\images\\normal.ebn");
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.CaretLine(4);
exedit1.Background(160/*exTempInlineToolTipBackColor*/,0x1000000);
exedit1.AllowInlineToolTip(512/*exInlineToolTipWordWrap*/);
exedit1.TempInlineToolTip("This is a bit of text that's shown programatically under the current line");
}
|
183
|
How can I display programmatically the inline tooltip, but using word-wrapping
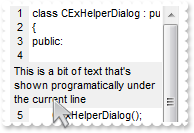
public void init()
{
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.CaretLine(4);
exedit1.Background(160/*exTempInlineToolTipBackColor*/,WinApi::RGB2int(240,240,240));
exedit1.AllowInlineToolTip(512/*exInlineToolTipWordWrap*/);
exedit1.TempInlineToolTip("This is a bit of text that's shown programatically under the current line");
}
|
182
|
How can I display programmatically the inline tooltip
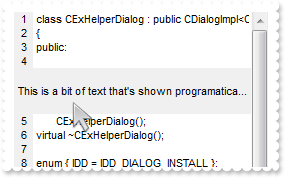
public void init()
{
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.CaretLine(4);
exedit1.Background(160/*exTempInlineToolTipBackColor*/,WinApi::RGB2int(240,240,240));
exedit1.TempInlineToolTip("<br><c>This is a bit of text that's shown programatically under the current line<br>");
}
|
181
|
How can I show the inline tooltip with a different appearance
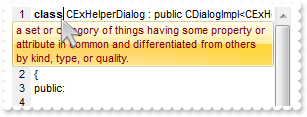
public void init()
{
str var_s;
;
super();
exedit1.VisualAppearance().Add(1,"c:\\exontrol\\images\\normal.ebn");
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
var_s = "a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality";
var_s = var_s + ".";
exedit1.AddKeyword("<b>class</b>",COMVariant::createFromStr(var_s));
exedit1.AllowInlineToolTip(513/*exInlineToolTipWordWrap | exInlineToolTip*/);
exedit1.Background(158/*exInlineToolTipBackColor*/,0x1000000);
exedit1.Background(159/*exInlineToolTipForeColor*/,WinApi::RGB2int(128,0,0));
exedit1.Refresh();
}
|
180
|
Is it possible to prevent moving the lines after the inline tooltip
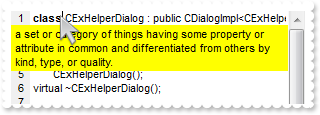
public void init()
{
str var_s;
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
var_s = "a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality";
var_s = var_s + ".";
exedit1.AddKeyword("<b>class</b>",COMVariant::createFromStr(var_s));
exedit1.AllowInlineToolTip(769/*exInlineToolTipWordWrap | exInlineToolTipOver | exInlineToolTip*/);
exedit1.Background(158/*exInlineToolTipBackColor*/,WinApi::RGB2int(255,255,0));
exedit1.Refresh();
}
|
179
|
How can I display the inline tooltip, when typing only
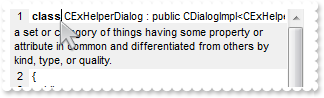
public void init()
{
str var_s;
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
exedit1.ToolTipOnTyping(false);
var_s = "a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality";
var_s = var_s + ".";
exedit1.AddKeyword("<b>class</b>",COMVariant::createFromStr(var_s));
exedit1.AllowInlineToolTip(514/*exInlineToolTipWordWrap | exInlineToolTipOnChange*/);
exedit1.Background(158/*exInlineToolTipBackColor*/,WinApi::RGB2int(240,240,240));
exedit1.Refresh();
}
|
178
|
How do I enable the inline tooltip support
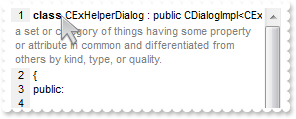
public void init()
{
str var_s;
;
super();
exedit1.LineNumberWidth(-1);
exedit1.LineNumberBackColor(WinApi::RGB2int(240,240,240));
var_s = "a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality";
var_s = var_s + ".";
exedit1.AddKeyword("<b>class</b>",COMVariant::createFromStr(var_s));
exedit1.AllowInlineToolTip(513/*exInlineToolTipWordWrap | exInlineToolTip*/);
exedit1.Background(159/*exInlineToolTipForeColor*/,WinApi::RGB2int(128,128,128));
exedit1.Refresh();
}
|
177
|
How do I display a tooltip for a non-keyword
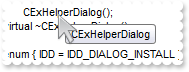
// MouseMove event - Occurs when the user moves the mouse.
void onEvent_MouseMove(int _Button,int _Shift,int _X,int _Y)
{
;
exedit1.ShowToolTip(exedit1.WordFromPoint(-1,-1),,,"+8","+8");
}
public void init()
{
;
super();
}
|
176
|
How do I get the text from the cursor
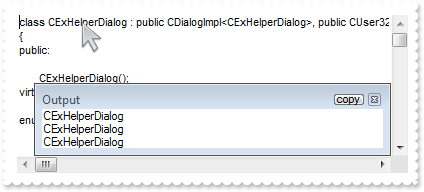
// MouseMove event - Occurs when the user moves the mouse.
void onEvent_MouseMove(int _Button,int _Shift,int _X,int _Y)
{
;
print( exedit1.WordFromPoint(-1,-1) );
}
public void init()
{
;
super();
}
|
175
|
I've noticed that while I type, the control's sensitive context selects the item that contains the typing word, so the question is how can I disable it
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("exText");
com_Context.Add("exHTML");
com_Context.Options(7/*exContextDisableIncrementalSearchContains*/,COMVariant::createFromBoolean(true));
exedit1.Text("");
exedit1.InsertText("Press CTRL+SPACE, and type h, so the exHTML is not selected.");
}
|
174
|
I have a context that inserts some comments, it is possible to set the cursor before comment begins, when user selects a value from the control's sensitive context
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
exedit1.AddExpression("<fgcolor=008000>'</fgcolor>","<fgcolor=008000> </fgcolor>","");
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("exText (0)","0 ' specifies the exText flag");
com_Context.Add("exHTML (-1)","-1 ' specifies the exHTML flag");
com_Context.Options(6/*exContextInsertCaretPos*/,"(0:=value lfind `'`) < 0 ? -1 : ( =:0 - (len(1:=(value left =:0)) - len(ltrim(reverse(=:1)))))");
exedit1.Text("");
exedit1.InsertText("Press CTRL + SPACE, and select any item, a number is inserted");
}
|
173
|
How can I show a different sensitive context when user press a key/character
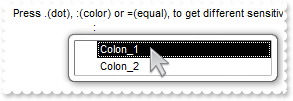
// Change event - Indicates that the control's text have changed.
void onEvent_Change()
{
;
exedit1.ShowContext(exedit1.ChangeOnKey());
exedit1.ActiveContextItems("");
}
public void init()
{
COM com_Context,com_Context1,com_Context2;
anytype var_Context,var_Context1,var_Context2;
;
super();
exedit1.Text("");
exedit1.InsertText("Press .(dot), :(color) or =(equal), to get different sensitive context");
exedit1.ActiveContextItems("");
var_Context = exedit1.Context("61"); com_Context = var_Context;
com_Context.Add("Equal_1");
com_Context.Add("Equal_2");
var_Context1 = exedit1.Context("46"); com_Context1 = var_Context1;
com_Context1.Add("Dot_1");
com_Context1.Add("Dot_2");
var_Context2 = exedit1.Context("58"); com_Context2 = var_Context2;
com_Context2.Add("Colon_1");
com_Context2.Add("Colon_2");
}
|
172
|
How can I allow spaces when control's sentitive context is shown/opened
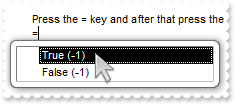
// Change event - Indicates that the control's text have changed.
void onEvent_Change()
{
;
exedit1.ShowContext(exedit1.ChangeOnKey());
exedit1.ActiveContextItems("");
}
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
var_Context = exedit1.Context("61"); com_Context = var_Context;
com_Context.Add("True (-1)","True");
com_Context.Add("False (-1)","False");
com_Context.Options(5/*exContextAllowSpaceOnFront*/,COMVariant::createFromBoolean(true));
exedit1.Text("");
exedit1.InsertText("Press the = key and after that press the space keys");
exedit1.InsertText("");
}
|
171
|
How can I display more pages on the control's senitive context
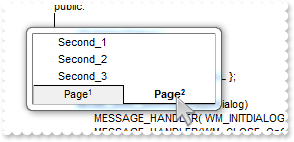
public void init()
{
COM com_Context,com_Context1;
anytype var_Context,var_Context1;
;
super();
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("First_1");
com_Context.Add("First_2");
var_Context1 = exedit1.Context("Second"); com_Context1 = var_Context1;
com_Context1.Add("Second_1");
com_Context1.Add("Second_2");
com_Context1.Add("Second_3");
exedit1.ActiveContextItems("Second");
exedit1.PagesContextItems(":Page<font ;6><off -4>1</off></font>,Second:Page<font ;6><off -4>2</off></font>");
}
|
170
|
Is it possible to disable showing tooltip for items in the control's senitive context
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("Column");
com_Context.Options(3/*exContextItemToolTip*/,"This is bit of text that shown when user selects the <b>Column</b> item.");
com_Context.Add("Item");
com_Context.Options(3/*exContextItemToolTip*/,"This is bit of text that shown when user selects the <b>Item</b> item.");
com_Context.Options(2/*exContextAllowToolTip*/,COMVariant::createFromBoolean(false));
}
|
169
|
How can I assign tooltips for items in the control's senitive context
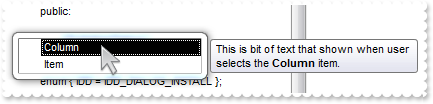
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("Column");
com_Context.Options(3/*exContextItemToolTip*/,"This is bit of text that shown when user selects the <b>Column</b> item.");
com_Context.Add("Item");
com_Context.Options(3/*exContextItemToolTip*/,"This is bit of text that shown when user selects the <b>Item</b> item.");
}
|
168
|
By default, the control shows the Context(""). How can I display other items
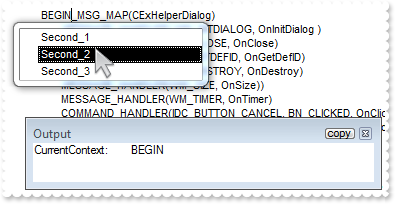
// OnContext event - Occurs when the user invokes the control's context window.
void onEvent_OnContext(int _Start,str _Context)
{
;
print( "CurrentContext:" );
print( _Context );
exedit1.ActiveContextItems("Second");
}
public void init()
{
COM com_Context,com_Context1;
anytype var_Context,var_Context1;
;
super();
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("First_1");
com_Context.Add("First_2");
var_Context1 = exedit1.Context("Second"); com_Context1 = var_Context1;
com_Context1.Add("Second_1");
com_Context1.Add("Second_2");
com_Context1.Add("Second_3");
}
|
167
|
How can I show the control's sensitive context
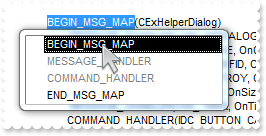
// DblClick event - Occurs when the user double clicks the left mouse button over an object.
void onEvent_DblClick(int _Shift,int _X,int _Y)
{
;
exedit1.ShowContext("DB");
}
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
var_Context = exedit1.Context("DB"); com_Context = var_Context;
com_Context.Add("BEGIN_MSG_MAP");
com_Context.Add("<fgcolor=808080>MESSAGE_HANDLER");
com_Context.Add("<fgcolor=808080>COMMAND_HANDLER");
com_Context.Add("END_MSG_MAP");
}
|
166
|
How can I provide different sensitive context
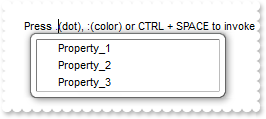
// Change event - Indicates that the control's text have changed.
void onEvent_Change()
{
;
exedit1.ShowContext(exedit1.ChangeOnKey());
}
public void init()
{
COM com_Context,com_Context1,com_Context2;
anytype var_Context,var_Context1,var_Context2;
;
super();
exedit1.Text("");
exedit1.InsertText("Press .(dot), :(colon) or CTRL + SPACE to invoke the control's context");
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("General_1");
com_Context.Add("General_2");
var_Context1 = exedit1.Context("46"); com_Context1 = var_Context1;
com_Context1.Add("Property_1");
com_Context1.Add("Property_2");
com_Context1.Add("Property_3");
var_Context2 = exedit1.Context("58"); com_Context2 = var_Context2;
com_Context2.Add("Method_1");
com_Context2.Add("Method_2");
com_Context2.Add("Method_3");
}
|
165
|
How can I change the control's background/foreground colors while the control is locked/read-only
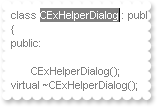
public void init()
{
;
super();
exedit1.Locked(true);
exedit1.SelBackColor(WinApi::RGB2int(128,128,128));
exedit1.ForeColorLockedLine(WinApi::RGB2int(128,128,128));
exedit1.BackColorLockedLine(WinApi::RGB2int(255,255,255));
}
|
164
|
How can change the color for selected text, when the control has no focus
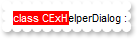
public void init()
{
;
super();
exedit1.HideSelection(false);
exedit1.SelLength(10);
exedit1.SelBackColorHide(WinApi::RGB2int(255,0,0));
}
|
163
|
How do I change the "Incremental Search" caption
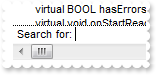
public void init()
{
;
super();
exedit1.Cursor(3/*exIncrementalSearchArea*/,"exHelp");
exedit1.Caption(3/*exIncrementalSearchField*/,0/*exCaption*/,"Search for: %s");
exedit1.IncrementalSearchError(WinApi::RGB2int(255,0,0));
}
|
162
|
How do I enable the scrollbar-extension, as thumb to be shown outside of the control's client area
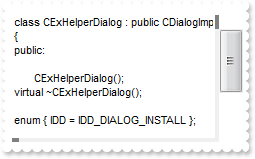
public void init()
{
;
super();
exedit1.ScrollBars(3/*exBoth*/);
exedit1.ScrollPartVisible(0/*exVScroll*/,65536/*exExtentThumbPart*/,true);
exedit1.ScrollPartVisible(1/*exHScroll*/,65536/*exExtentThumbPart*/,true);
exedit1.ScrollPartVisible(2,65536/*exExtentThumbPart*/,true);
exedit1.ScrollWidth(4);
exedit1.Background(276/*exVSBack*/,WinApi::RGB2int(240,240,240));
exedit1.Background(260/*exVSThumb*/,WinApi::RGB2int(128,128,128));
exedit1.ScrollHeight(4);
exedit1.Background(404/*exHSBack*/,exedit1.Background(276/*exVSBack*/));
exedit1.Background(388/*exHSThumb*/,exedit1.Background(260/*exVSThumb*/));
exedit1.Background(3/*exSizeGrip*/,exedit1.Background(276/*exVSBack*/));
}
|
161
|
How can I get ride of control's horizontal scroll bar
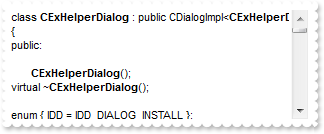
public void init()
{
;
super();
exedit1.AddKeyword("<b>CExHelperDialog</b>");
exedit1.Refresh();
exedit1.ScrollBars(2/*exVertical*/);
}
|
160
|
How do I specify the characters to close the sensitive context
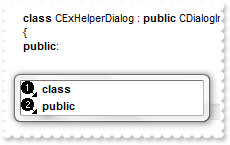
public void init()
{
COM com_Context;
anytype var_Context;
str var_s;
;
super();
var_s = "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql";
var_s = var_s + "Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0";
var_s = var_s + "ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN";
var_s = var_s + "AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=";
exedit1.Images(COMVariant::createFromStr(var_s));
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.AddKeyword("<b>public</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = exedit1.Context(); com_Context = var_Context;
com_Context.Add("<b>class</b>","",COMVariant::createFromInt(1));
com_Context.Add("<b>public</b>","",COMVariant::createFromInt(2));
com_Context.Options(1/*exContextAllowChars*/,"_ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz");
}
|
159
|
How do I sort items in the sensitive context
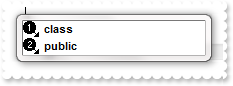
public void init()
{
COM com_Context;
anytype var_Context;
str var_s;
;
super();
var_s = "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql";
var_s = var_s + "Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0";
var_s = var_s + "ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN";
var_s = var_s + "AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=";
exedit1.Images(COMVariant::createFromStr(var_s));
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.AddKeyword("<b>public</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("<b>public</b>","",COMVariant::createFromInt(2));
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("<b>class</b>","",COMVariant::createFromInt(1));
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Sort(true);
}
|
158
|
Can I add icons to the sensitive context
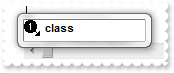
public void init()
{
COM com_Context;
anytype var_Context;
str var_s;
;
super();
var_s = "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql";
var_s = var_s + "Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0";
var_s = var_s + "ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN";
var_s = var_s + "AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=";
exedit1.Images(COMVariant::createFromStr(var_s));
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("<b>class</b>","",COMVariant::createFromInt(1));
}
|
157
|
How can I change the keys combination that invokes the sensitive context
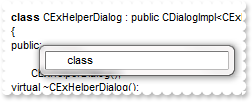
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
exedit1.ContextKey(544);
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("class");
}
|
156
|
How do I enable or disable the sensitive context menu
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
exedit1.CodeCompletion(0/*exCodeCompletionDisable*/);
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("class");
}
|
155
|
How can I add a sensitive context menu
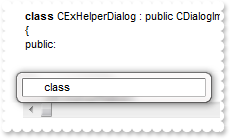
public void init()
{
COM com_Context;
anytype var_Context;
;
super();
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
var_Context = COM::createFromObject(exedit1.Context()); com_Context = var_Context;
com_Context.Add("class");
}
|
154
|
Can I use wild characters to define keys in your control
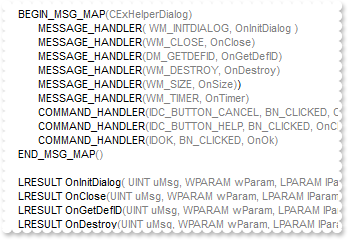
public void init()
{
;
super();
exedit1.AddWild("<fgcolor=808080>(*)</fgcolor>");
exedit1.Refresh();
}
|
153
|
Can I use wild characters to define keys in your control
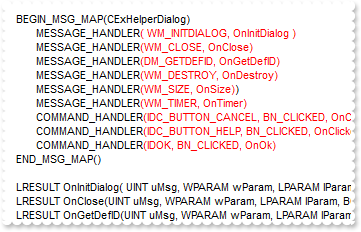
public void init()
{
;
super();
exedit1.AddWild("_HANDLER<fgcolor=FF0000>(*)</fgcolor>");
exedit1.Refresh();
}
|
152
|
How can I remove or delete all expressions
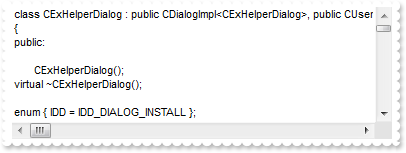
public void init()
{
;
super();
exedit1.AddExpression("(","<b><fgcolor=FF0000> </fgcolor></b>",")",COMVariant::createFromBoolean(false));
exedit1.ClearExpressions();
exedit1.Refresh();
}
|
151
|
How can I remove or delete an expression
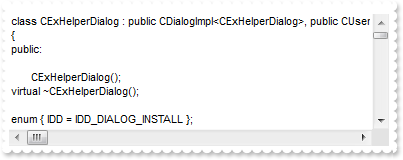
public void init()
{
;
super();
exedit1.AddExpression("(","<b><fgcolor=FF0000> </fgcolor></b>",")",COMVariant::createFromBoolean(false));
exedit1.DeleteExpression("(");
exedit1.Refresh();
}
|
150
|
How can I add an expression
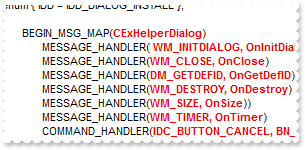
public void init()
{
;
super();
exedit1.AddExpression("(","<b><fgcolor=FF0000> </fgcolor></b>",")",COMVariant::createFromBoolean(false));
exedit1.Refresh();
}
|
149
|
How can I add an expression on multiple lines
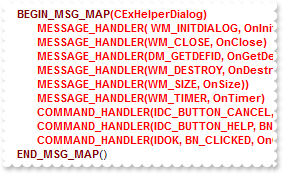
public void init()
{
;
super();
exedit1.AddExpression("<fgcolor=800000><b>BEGIN_MSG_MAP</b></fgcolor>","<b><fgcolor=FF0000> </fgcolor></b>","<fgcolor=800000><b>END_MSG_MAP</b></fgcolor>",COMVariant::createFromBoolean(true));
exedit1.Refresh();
}
|
148
|
How can I remove or delete all keywords
public void init()
{
;
super();
exedit1.AddKeyword("<b><fgcolor=FF0000>class</fgcolor></b>");
exedit1.ClearKeywords();
exedit1.Refresh();
}
|
147
|
How can I remove or delete keyword
public void init()
{
;
super();
exedit1.AddKeyword("<b><fgcolor=FF0000>class</fgcolor></b>");
exedit1.DeleteKeyword("class");
exedit1.Refresh();
}
|
146
|
How do I add a keyword that's not case sensitive
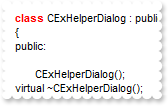
public void init()
{
;
super();
exedit1.AddKeyword("<b><fgcolor=FF0000>class</fgcolor></b>","","",COMVariant::createFromInt(2));
exedit1.Refresh();
exedit1.InsertText("ClasS\\r\\n",COMVariant::createFromInt(1));
exedit1.InsertText("CLASS\\r\\n",COMVariant::createFromInt(1));
}
|
145
|
How do I add a keyword that's not case sensitive
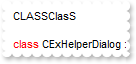
public void init()
{
;
super();
exedit1.AddKeyword("<fgcolor=FF0000>class</fgcolor>","","",COMVariant::createFromInt(1));
exedit1.Refresh();
exedit1.InsertText("ClasS\\r\\n",COMVariant::createFromInt(1));
exedit1.InsertText("CLASS\\r\\n",COMVariant::createFromInt(1));
}
|
144
|
How can I assign a tooltip to a keyword
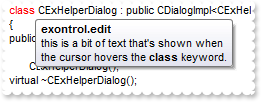
public void init()
{
;
super();
exedit1.AddKeyword("<fgcolor=FF0000>class</fgcolor>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
}
|
143
|
How do I add a keyword

public void init()
{
;
super();
exedit1.AddKeyword("<fgcolor=FF0000>class</fgcolor>");
exedit1.Refresh();
}
|
142
|
How do I add a keyword
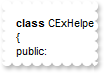
public void init()
{
;
super();
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
}
|
141
|
How can I display a tooltip as soon as the user types a keyword
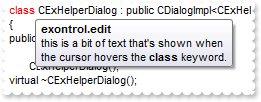
public void init()
{
;
super();
exedit1.ToolTipDelay(1);
exedit1.ToolTipOnTyping(true);
exedit1.AddKeyword("<b>class</b>","this is a bit of text that's shown when the cursor hovers the <b>class</b> keyword.","exontrol.edit");
exedit1.Refresh();
}
|
140
|
How do I change the color for a locked or a read only line
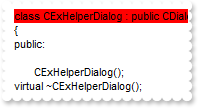
public void init()
{
;
super();
exedit1.ForeColorLockedLine(WinApi::RGB2int(0,0,0));
exedit1.BackColorLockedLine(WinApi::RGB2int(255,0,0));
exedit1.LockedLine(1,true);
}
|
139
|
How do I lock or make read only a line
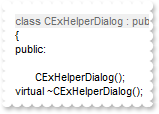
public void init()
{
;
super();
exedit1.LockedLine(1,true);
}
|
138
|
How do I start overtyping
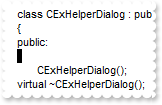
public void init()
{
;
super();
exedit1.Overtype(true);
}
|
137
|
How do I get the selection
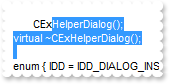
public void init()
{
;
super();
exedit1.GetSelection(sy,sx,ey,ex);
print( sy );
print( sx );
print( ey );
print( ex );
}
|
136
|
How do I select multiple lines
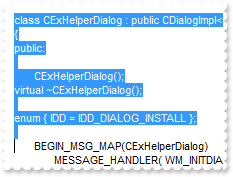
public void init()
{
;
super();
exedit1.SetSelection(COMVariant::createFromInt(0),COMVariant::createFromInt(0),COMVariant::createFromInt(10),COMVariant::createFromInt(0));
exedit1.HideSelection(false);
}
|
135
|
How can I change the shape of the cursor when it hovers the selected text
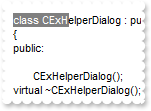
public void init()
{
;
super();
exedit1.Cursor(4/*exSelectedText*/,"exHelp");
exedit1.SelLength(10);
exedit1.HideSelection(false);
}
|
134
|
How can I change the shape of the cursor when it hovers the incremental search area
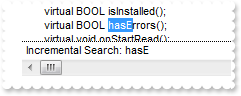
public void init()
{
;
super();
exedit1.Cursor(3/*exIncrementalSearchArea*/,"exHelp");
}
|
133
|
How can I change the shape of the cursor when it hovers the line numbers area
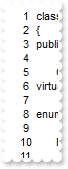
public void init()
{
;
super();
exedit1.Cursor(2/*exLineNumberArea*/,"exHelp");
exedit1.LineNumberWidth(16);
}
|
132
|
How can I change the shape of the cursor when it hovers the bookmark area
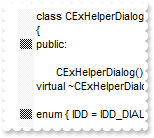
public void init()
{
;
super();
exedit1.Cursor(1/*exBookmarkArea*/,"exHelp");
exedit1.BookmarkWidth(16);
}
|
131
|
How can I change the shape of the cursor when it hovers the edit
public void init()
{
;
super();
exedit1.Cursor(0/*exEditArea*/,"exHelp");
}
|
130
|
How can I enable or disable OLE drag and drop operations
public void init()
{
;
super();
exedit1.OLEDropMode(-1/*exOLEDropAutomatic*/);
}
|
129
|
How can I change the descriptions for items in the control's context menu
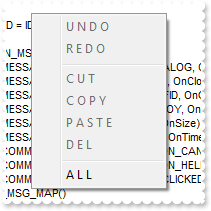
public void init()
{
;
super();
exedit1.Caption(2/*exContextMenu*/,16384/*exContextUndo*/,"U N D O");
exedit1.Caption(2/*exContextMenu*/,16385/*exContextRedo*/,"R E D O");
exedit1.Caption(2/*exContextMenu*/,16387/*exContextCut*/,"C U T");
exedit1.Caption(2/*exContextMenu*/,16388/*exContextCopy*/,"C O P Y");
exedit1.Caption(2/*exContextMenu*/,16389/*exContextPaste*/,"P A S T E");
exedit1.Caption(2/*exContextMenu*/,16390/*exContextDelete*/,"D E L");
exedit1.Caption(2/*exContextMenu*/,16392/*exContextSelectAll*/,"A L L ");
}
|
128
|
How can I change the descriptions for fields in the Replace dialog
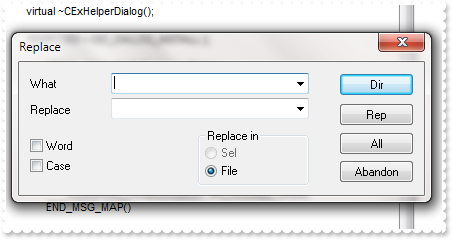
public void init()
{
;
super();
exedit1.Caption(1/*exReplaceDialog*/,202/*exFieldFindWhat*/,"What");
exedit1.Caption(1/*exReplaceDialog*/,204/*exFieldReplaceWith*/,"Replace");
exedit1.Caption(1/*exReplaceDialog*/,104/*exFieldWordOnly*/,"Word");
exedit1.Caption(1/*exReplaceDialog*/,105/*exFieldMatchCase*/,"Case");
exedit1.Caption(1/*exReplaceDialog*/,103/*exFieldFindNext*/,"Dir");
exedit1.Caption(1/*exReplaceDialog*/,113/*exFieldSelection*/,"Sel");
exedit1.Caption(1/*exReplaceDialog*/,114/*exFieldWholeFile*/,"File");
exedit1.Caption(1/*exReplaceDialog*/,21199/*exFieldReplace*/,"Rep");
exedit1.Caption(1/*exReplaceDialog*/,21200/*exFieldReplaceAll*/,"All");
exedit1.Caption(1/*exReplaceDialog*/,2/*exFieldCancel*/,"Abandon");
exedit1.Caption(1/*exReplaceDialog*/,32000/*exErrorTitle*/,"Title");
exedit1.Caption(1/*exReplaceDialog*/,32001/*exErrorFindNext*/,"Failed!");
exedit1.Caption(1/*exReplaceDialog*/,32001/*exErrorFindNext*/,"Done");
}
|
127
|
How can I change the descriptions for fields in the Find dialog
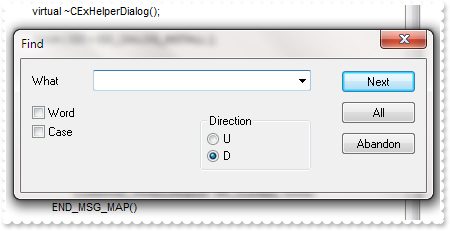
public void init()
{
;
super();
exedit1.Caption(0/*exFindDialog*/,202/*exFieldFindWhat*/,"What");
exedit1.Caption(0/*exFindDialog*/,104/*exFieldWordOnly*/,"Word");
exedit1.Caption(0/*exFindDialog*/,105/*exFieldMatchCase*/,"Case");
exedit1.Caption(0/*exFindDialog*/,103/*exFieldFindNext*/,"Dir");
exedit1.Caption(0/*exFindDialog*/,113/*exFieldSelection*/,"U");
exedit1.Caption(0/*exFindDialog*/,114/*exFieldWholeFile*/,"D");
exedit1.Caption(0/*exFindDialog*/,103/*exFieldFindNext*/,"Next");
exedit1.Caption(0/*exFindDialog*/,21199/*exFieldReplace*/,"All");
exedit1.Caption(0/*exFindDialog*/,2/*exFieldCancel*/,"Abandon");
exedit1.Caption(0/*exFindDialog*/,32001/*exErrorFindNext*/,"Failed!");
}
|
126
|
How can I change the caption for the Replace dialog
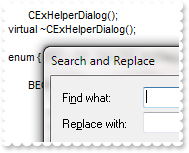
public void init()
{
;
super();
exedit1.Caption(1/*exReplaceDialog*/,0/*exCaption*/,"Search and Replace");
}
|
125
|
How can I change the caption for the Find dialog
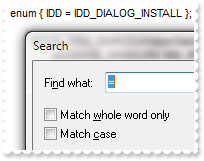
public void init()
{
;
super();
exedit1.Caption(0/*exFindDialog*/,0/*exCaption*/,"Search");
}
|
124
|
How can I move the cursor when user invokes the control's context menu
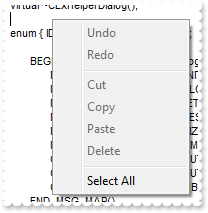
public void init()
{
;
super();
exedit1.RClick(true);
}
|
123
|
How can I disable indenting the selected text when the user presses the TAB key
public void init()
{
;
super();
exedit1.IndentOnTab(false);
}
|
122
|
How can I indent a line
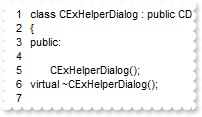
public void init()
{
;
super();
exedit1.LineNumberWidth(18);
exedit1.HideSelection(false);
exedit1.SelectLine(3);
exedit1.IndentSel(true);
}
|
121
|
How can I show or hide the control's splitter
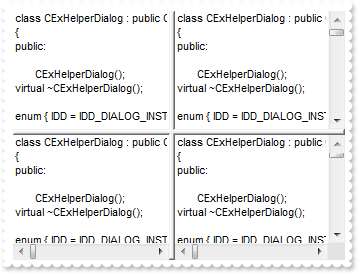
public void init()
{
;
super();
exedit1.AllowSplitter(3/*exBothSplitter*/);
exedit1.SplitPaneHeight(128);
exedit1.SplitPaneWidth(128);
}
|
120
|
How can I select a line
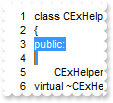
public void init()
{
;
super();
exedit1.LineNumberWidth(18);
exedit1.HideSelection(false);
exedit1.SelectLine(3);
}
|
119
|
How do I change the font to display the line numbers
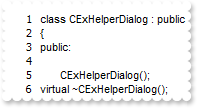
public void init()
{
;
super();
exedit1.LineNumberFont().Name("Tahoma");
exedit1.LineNumberWidth(18);
}
|
118
|
How can I change the height of the line
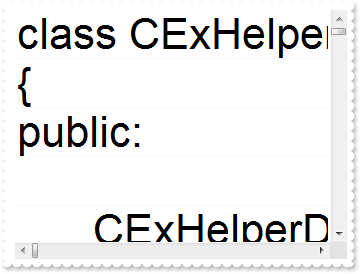
public void init()
{
;
super();
exedit1.Font().Size(32);
exedit1.DrawGridLines(true);
exedit1.Refresh();
}
|
117
|
How can I show or hide the grid lines
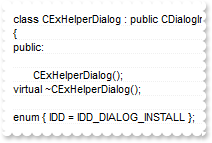
public void init()
{
;
super();
exedit1.DrawGridLines(true);
}
|
116
|
How do I highlight the position of multiple lines expression on the vertical scroll bar
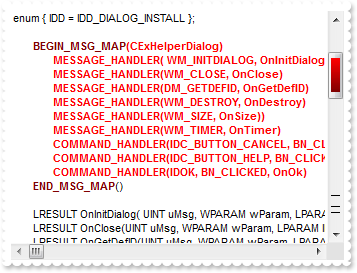
public void init()
{
;
super();
exedit1.AllowMark(true);
exedit1.MarkContinueBlocks(true);
exedit1.AddKeyword("<b>CAxWnd");
exedit1.AddExpression("<fgcolor=800000><b>BEGIN_MSG_MAP</b></fgcolor>","<b><fgcolor=FF0000> </fgcolor></b>","<fgcolor=800000><b>END_MSG_MAP</b></fgcolor>",COMVariant::createFromBoolean(true));
exedit1.MarkColor("BEGIN_MSG_MAP",WinApi::RGB2int(255,0,0));
exedit1.MarkColor("END_MSG_MAP",WinApi::RGB2int(128,0,0));
exedit1.MarkColor("CAxWnd",WinApi::RGB2int(0,0,0));
exedit1.Refresh();
}
|
115
|
How do I ignore \" in a string
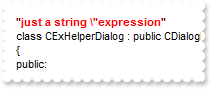
public void init()
{
;
super();
exedit1.InsertText("\"just a string \\\"expression\"\\r\\n",COMVariant::createFromInt(1));
exedit1.AddExpression("<fgcolor=800000><b>\"</b></fgcolor>","<b><fgcolor=FF0000> </fgcolor></b>","<fgcolor=800000><b>\"</b></fgcolor>",COMVariant::createFromBoolean(true));
exedit1.IgnorePrefixInExpression("\"","\\");
exedit1.Refresh();
}
|
114
|
How can I change the color for the line number's border
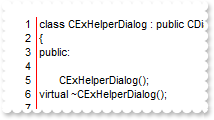
public void init()
{
;
super();
exedit1.LineNumberBorderColor(WinApi::RGB2int(255,0,0));
exedit1.LineNumberWidth(18);
}
|
113
|
How can I change the color for the bookmark's border
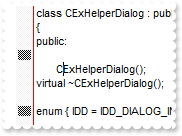
public void init()
{
;
super();
exedit1.BookmarkBorderColor(WinApi::RGB2int(255,0,0));
exedit1.BookmarkWidth(18);
}
|
112
|
Can I display a custom icon or picture for bookmarks
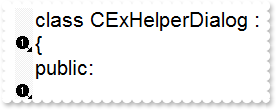
public void init()
{
str var_s;
;
super();
var_s = "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql";
var_s = var_s + "Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0";
var_s = var_s + "ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN";
var_s = var_s + "AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=";
exedit1.Images(COMVariant::createFromStr(var_s));
exedit1.BookmarkImage(1);
exedit1.Bookmark(2,true);
exedit1.Bookmark(4,true);
exedit1.BookmarkWidth(18);
}
|
111
|
Can I display a custom icon or picture in the bookmark area
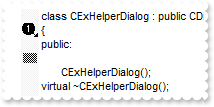
public void init()
{
str var_s;
;
super();
var_s = "gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql";
var_s = var_s + "Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0";
var_s = var_s + "ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN";
var_s = var_s + "AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=";
exedit1.Images(COMVariant::createFromStr(var_s));
exedit1.BookmarkImageLine(2,1);
exedit1.Bookmark(4,true);
exedit1.BookmarkWidth(18);
}
|
110
|
How do I remove the line's background color
public void init()
{
;
super();
exedit1.BackColorLine(1,WinApi::RGB2int(255,0,0));
exedit1.ClearBackColorLine(1);
}
|
109
|
How do I change the foreground color for a line
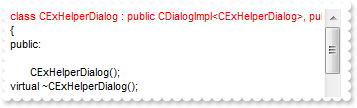
public void init()
{
;
super();
exedit1.ForeColorLine(1,WinApi::RGB2int(255,0,0));
}
|
108
|
How do I change the background color for a line
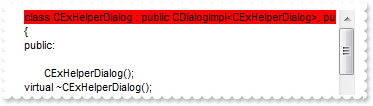
public void init()
{
;
super();
exedit1.BackColorLine(1,WinApi::RGB2int(255,0,0));
}
|
107
|
How can I add my own items in the control's context menu
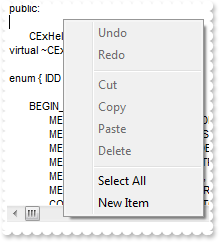
public void init()
{
;
super();
exedit1.ContextMenuItems("New Item");
}
|
106
|
How do I ensure that a specified line is visible
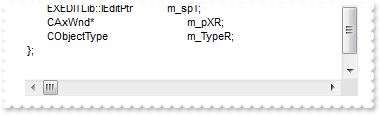
public void init()
{
;
super();
exedit1.EnsureVisibleLine(exedit1.Count());
}
|
105
|
How can I programmatically perform a REDO operation
public void init()
{
;
super();
exedit1.Redo();
}
|
104
|
How can I programmatically perform an UNDO operation
public void init()
{
;
super();
exedit1.Undo();
}
|
103
|
How do I get the bookmarks as a list
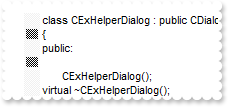
public void init()
{
COMVariant var_BookmarksList;
;
super();
exedit1.Bookmark(2,true);
exedit1.Bookmark(4,true);
exedit1.BookmarkWidth(16);
var_BookmarksList = exedit1.BookmarksList();
}
|
102
|
How can I move to the previous bookmark
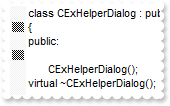
public void init()
{
;
super();
exedit1.Bookmark(2,true);
exedit1.Bookmark(4,true);
exedit1.BookmarkWidth(16);
exedit1.PrevBookmark();
}
|
101
|
How can I move to the next bookmark
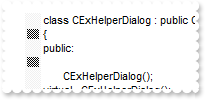
public void init()
{
;
super();
exedit1.Bookmark(2,true);
exedit1.Bookmark(4,true);
exedit1.BookmarkWidth(16);
exedit1.NextBookmark();
}
|