205
|
Is it possible to show just expressions
with Edit1 do
begin
AddWild('<fgcolor=00FF00>(');
AddWild('<fgcolor=00FF00>)');
AddExpression('<fgcolor=FF0000><b>(*','<fgcolor=FF0000> ','<fgcolor=FF0000><b>*)',Null,Null);
InsertText('some text ( another text ) other text\r\n',OleVariant(1));
InsertText('some text (* another text *) other text\r\n',OleVariant(1));
Show := 'expression';
end
|
204
|
How can I stop any highlight
with Edit1 do
begin
AddWild('<fgcolor=00FF00>(');
AddWild('<fgcolor=00FF00>)');
AddExpression('<fgcolor=FF0000><b>(*','<fgcolor=FF0000> ','<fgcolor=FF0000><b>*)',Null,Null);
InsertText('some text ( another text ) other text\r\n',OleVariant(1));
InsertText('some text (* another text *) other text\r\n',OleVariant(1));
Show := '';
end
|
203
|
How can I highlight the start of the line until a specified character is found
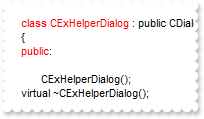
with Edit1 do
begin
AddExpression('^','<fgcolor=FF0000> ',':',Null,Null);
Refresh();
end
|
202
|
Can I use code completion without any UI
with Edit1 do
begin
CodeCompletion := EXEDITLib_TLB.exCodeCompletionEnableNoUI;
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
Context[Null].Add('class',Null,Null,Null);
end
|
201
|
How can I hide the control's horizontal scroll bar
with Edit1 do
begin
ScrollBars := EXEDITLib_TLB.exVertical;
end
|
200
|
Is it possible to change the line's height
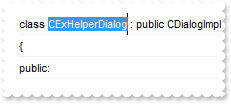
with Edit1 do
begin
LineHeight := 'value + 8 * dpi';
DrawGridLines := True;
end
|
199
|
How to bold everything between two * (asterisk) characters

with Edit1 do
begin
with Font do
begin
Name := 'Consolas';
Size := 12;
end;
AddExpression('<fgcolor=FF0000><b>*','<fgcolor=FF0000> ','<fgcolor=FF0000><b>*',Null,Null);
InsertText('some text * another text * other text\r\n',OleVariant(1));
Refresh();
end
|
198
|
How to bold everything that starts with * (asterisk), to the end of the line

with Edit1 do
begin
with Font do
begin
Name := 'Consolas';
Size := 12;
end;
AddWild('<fgcolor=FF0000><b>\**');
InsertText('some text * another text * other text\r\n',OleVariant(1));
Refresh();
end
|
197
|
How to make a * (asterisk) bold, not the entire / rest line

with Edit1 do
begin
with Font do
begin
Name := 'Consolas';
Size := 12;
end;
AddWild('<fgcolor=FF0000><b>\*');
InsertText('some text * another text * other text\r\n',OleVariant(1));
Refresh();
end
|
196
|
How can I change the control's font (template)
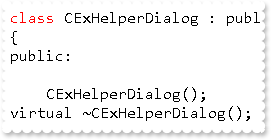
with Edit1 do
begin
Template := 'Font { Name = `Consolas`; Size = 12 }';
AddKeyword('<fgcolor=FF0000>class</fgcolor>',Null,Null,Null);
Refresh();
end
|
195
|
How can I change the control's font (runtime)
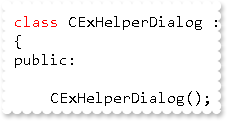
with Edit1 do
begin
with Font do
begin
Name := 'Consolas';
Size := 12;
end;
AddKeyword('<fgcolor=FF0000>class</fgcolor>',Null,Null,Null);
Refresh();
end
|
194
|
When I click and drag to try and select some text, sometimes my cursor turns into a hand and drags the whole text in the window around. I would like to disable this feature, could you tell me what it is called so I can disable it please
with Edit1 do
begin
OLEDropMode := EXEDITLib_TLB.exOLEDropAutomatic;
end
|
193
|
How can I display information about events the control fires
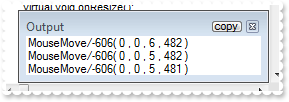
// Event event - Notifies the application once the control fires an event.
procedure TForm1.Edit1Event(ASender: TObject; EventID : Integer);
begin
with Edit1 do
begin
OutputDebugString( EventParam[-2] );
end
end;
with Edit1 do
begin
AddWild('<fgcolor=808080>(?*)</fgcolor>');
AddKeyword('<b>class</b>','a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality' +
'',Null,Null);
Refresh();
end
|
192
|
How do I highlights words based on wild characters
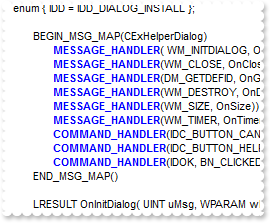
with Edit1 do
begin
AddWild('<fgcolor=0000FF><b>[MC]*_HANDLER*</b></fgcolor>(*)');
Refresh();
end
|
191
|
How do I highlights words based on wild characters
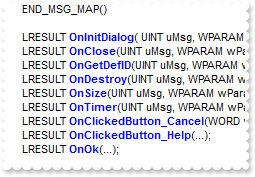
with Edit1 do
begin
AddWild('<fgcolor=0000FF><b> *</b></fgcolor>(*)*;');
Refresh();
end
|
190
|
How can I provide different tooltip for the same keyword
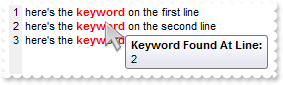
// QueryContext event - Queries for the context at the specified location, to provide different tooltips for the same keyword on QueryContextToolTip event.
procedure TForm1.Edit1QueryContext(ASender: TObject; XCursor : Integer;YCursor : Integer;var QContext : OleVariant);
begin
with Edit1 do
begin
QContext := YCursor;
end
end;
// QueryContextToolTip event - Asks for the tooltip/title of the keyword on the context retrieved by the QueryContext event.
procedure TForm1.Edit1QueryContextToolTip(ASender: TObject; QContext : WideString;Keyword : WideString;var QToolTip : OleVariant;var QToolTipTitle : OleVariant);
begin
with Edit1 do
begin
QToolTip := QContext;
QToolTipTitle := 'Keyword Found At Line:';
end
end;
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
AddKeyword('<fgcolor=FF0000><b>keyword</b></fgcolor>',Null,Null,Null);
Text := '';
InsertText('here''s the keyword on the first line',Null);
InsertText('\r\nhere''s the keyword on the second line',Null);
InsertText('\r\nhere''s the keyword on the third line',Null);
end
|
189
|
Is it possible to left, right or center align the inline tooltip
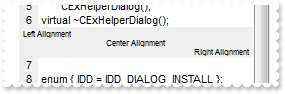
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
CaretLine := 6;
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $f0f0f0;
TempInlineToolTip := '<font ;6>Left Alignment<br><c>Center Alignment<br><r>Right Alignment';
Refresh();
end
|
188
|
Is it possible to display the inline tooltip with a different appearance than temporarily inline tooltip
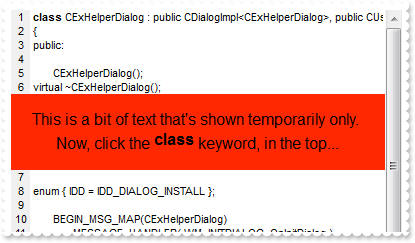
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
AddKeyword('<b>class</b>','<r>a set or category of things having some property or attribute in common and differentiated from others by kind, type, or qual' +
'ity.',Null,Null);
AllowInlineToolTip := Integer(EXEDITLib_TLB.exInlineToolTipWordWrap) Or Integer(EXEDITLib_TLB.exInlineToolTip);
Background[EXEDITLib_TLB.exInlineToolTipForeColor] := $808080;
Background[EXEDITLib_TLB.exInlineToolTipBackColor] := $f0f0f0;
CaretLine := 6;
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $28ff;
Background[EXEDITLib_TLB.exTempInlineToolTipForeColor] := $10000;
TempInlineToolTip := '<br><c><font ;12>This is a bit of text that''s shown temporarily only. <br><c>Now, click the <off -4><b>class</b></off> keyword, ' +
'in the top...<br>';
Refresh();
end
|
187
|
How can I display the inline tooltip over the lines, instead pushing the lines
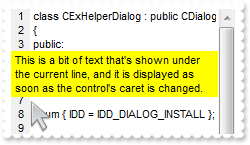
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $ffff;
CaretLine := 3;
TempInlineToolTip := 'This is a bit of text that''s shown under the current line, and it is displayed as soon as the control''s caret is changed.';
AllowInlineToolTip := Integer(EXEDITLib_TLB.exInlineToolTipWordWrap) Or Integer(EXEDITLib_TLB.exInlineToolTipOver);
Refresh();
end
|
186
|
Is it possible to display the inline tooltip all the time
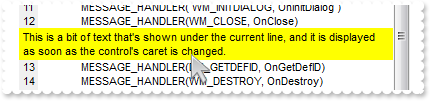
// SelChange event - Occurs when the user selects text in the control.
procedure TForm1.Edit1SelChange(ASender: TObject; );
begin
with Edit1 do
begin
TempInlineToolTip := 'This is a bit of text that''s shown under the current line, and it is displayed as soon as the control''s caret is changed.';
end
end;
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $ffff;
CaretLine := 12;
AllowInlineToolTip := EXEDITLib_TLB.exInlineToolTipWordWrap;
Refresh();
end
|
185
|
Is it possible to display images in the inline tooltip
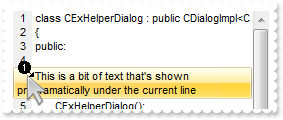
with Edit1 do
begin
VisualAppearance.Add(1,'c:\exontrol\images\normal.ebn');
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
CaretLine := 4;
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $1000000;
AllowInlineToolTip := EXEDITLib_TLB.exInlineToolTipWordWrap;
TempInlineToolTip := '<img>1</img>This is a bit of text that''s shown programatically under the current line';
end
|
184
|
How can I change the visual appearance of the temporarily inline tooltip
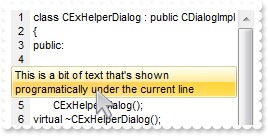
with Edit1 do
begin
VisualAppearance.Add(1,'c:\exontrol\images\normal.ebn');
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
CaretLine := 4;
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $1000000;
AllowInlineToolTip := EXEDITLib_TLB.exInlineToolTipWordWrap;
TempInlineToolTip := 'This is a bit of text that''s shown programatically under the current line';
end
|
183
|
How can I display programmatically the inline tooltip, but using word-wrapping
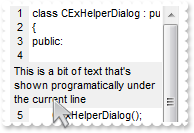
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
CaretLine := 4;
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $f0f0f0;
AllowInlineToolTip := EXEDITLib_TLB.exInlineToolTipWordWrap;
TempInlineToolTip := 'This is a bit of text that''s shown programatically under the current line';
end
|
182
|
How can I display programmatically the inline tooltip
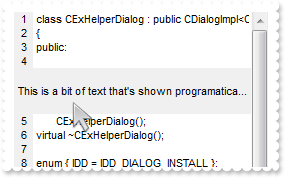
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
CaretLine := 4;
Background[EXEDITLib_TLB.exTempInlineToolTipBackColor] := $f0f0f0;
TempInlineToolTip := '<br><c>This is a bit of text that''s shown programatically under the current line<br>';
end
|
181
|
How can I show the inline tooltip with a different appearance
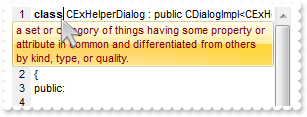
with Edit1 do
begin
VisualAppearance.Add(1,'c:\exontrol\images\normal.ebn');
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
AddKeyword('<b>class</b>','a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality' +
'.',Null,Null);
AllowInlineToolTip := Integer(EXEDITLib_TLB.exInlineToolTipWordWrap) Or Integer(EXEDITLib_TLB.exInlineToolTip);
Background[EXEDITLib_TLB.exInlineToolTipBackColor] := $1000000;
Background[EXEDITLib_TLB.exInlineToolTipForeColor] := $80;
Refresh();
end
|
180
|
Is it possible to prevent moving the lines after the inline tooltip
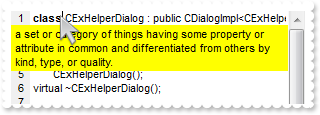
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
AddKeyword('<b>class</b>','a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality' +
'.',Null,Null);
AllowInlineToolTip := Integer(EXEDITLib_TLB.exInlineToolTipWordWrap) Or Integer(EXEDITLib_TLB.exInlineToolTipOver) Or Integer(EXEDITLib_TLB.exInlineToolTip);
Background[EXEDITLib_TLB.exInlineToolTipBackColor] := $ffff;
Refresh();
end
|
179
|
How can I display the inline tooltip, when typing only
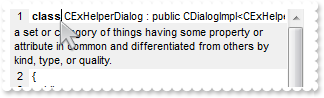
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
ToolTipOnTyping := False;
AddKeyword('<b>class</b>','a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality' +
'.',Null,Null);
AllowInlineToolTip := Integer(EXEDITLib_TLB.exInlineToolTipWordWrap) Or Integer(EXEDITLib_TLB.exInlineToolTipOnChange);
Background[EXEDITLib_TLB.exInlineToolTipBackColor] := $f0f0f0;
Refresh();
end
|
178
|
How do I enable the inline tooltip support
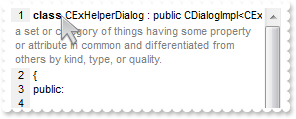
with Edit1 do
begin
LineNumberWidth := -1;
LineNumberBackColor := RGB(240,240,240);
AddKeyword('<b>class</b>','a set or category of things having some property or attribute in common and differentiated from others by kind, type, or quality' +
'.',Null,Null);
AllowInlineToolTip := Integer(EXEDITLib_TLB.exInlineToolTipWordWrap) Or Integer(EXEDITLib_TLB.exInlineToolTip);
Background[EXEDITLib_TLB.exInlineToolTipForeColor] := $808080;
Refresh();
end
|
177
|
How do I display a tooltip for a non-keyword
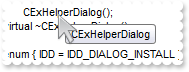
// MouseMove event - Occurs when the user moves the mouse.
procedure TForm1.Edit1MouseMove(ASender: TObject; Button : Smallint;Shift : Smallint;X : Integer;Y : Integer);
begin
with Edit1 do
begin
ShowToolTip(WordFromPoint[-1,-1,Null],Null,Null,'+8','+8');
end
end;
|
176
|
How do I get the text from the cursor
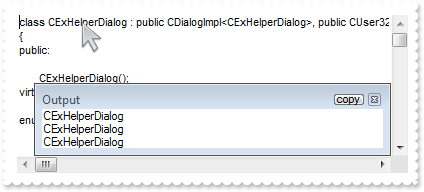
// MouseMove event - Occurs when the user moves the mouse.
procedure TForm1.Edit1MouseMove(ASender: TObject; Button : Smallint;Shift : Smallint;X : Integer;Y : Integer);
begin
with Edit1 do
begin
OutputDebugString( WordFromPoint[-1,-1,Null] );
end
end;
|
175
|
I've noticed that while I type, the control's sensitive context selects the item that contains the typing word, so the question is how can I disable it
with Edit1 do
begin
with Context[Null] do
begin
Add('exText',Null,Null,Null);
Add('exHTML',Null,Null,Null);
Options[EXEDITLib_TLB.exContextDisableIncrementalSearchContains] := OleVariant(True);
end;
Text := '';
InsertText('Press CTRL+SPACE, and type h, so the exHTML is not selected.',Null);
end
|
174
|
I have a context that inserts some comments, it is possible to set the cursor before comment begins, when user selects a value from the control's sensitive context
with Edit1 do
begin
AddExpression('<fgcolor=008000>''</fgcolor>','<fgcolor=008000> </fgcolor>','',Null,Null);
with Context[Null] do
begin
Add('exText (0)','0 '' specifies the exText flag',Null,Null);
Add('exHTML (-1)','-1 '' specifies the exHTML flag',Null,Null);
Options[EXEDITLib_TLB.exContextInsertCaretPos] := '(0:=value lfind `''`) < 0 ? -1 : ( =:0 - (len(1:=(value left =:0)) - len(ltrim(reverse(=:1)))))';
end;
Text := '';
InsertText('Press CTRL + SPACE, and select any item, a number is inserted',Null);
end
|
173
|
How can I show a different sensitive context when user press a key/character
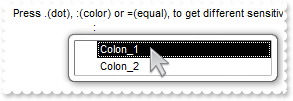
// Change event - Indicates that the control's text have changed.
procedure TForm1.Edit1Change(ASender: TObject; );
begin
with Edit1 do
begin
ShowContext(OleVariant(ChangeOnKey));
ActiveContextItems := '';
end
end;
with Edit1 do
begin
Text := '';
InsertText('Press .(dot), :(color) or =(equal), to get different sensitive context',Null);
ActiveContextItems := '';
with Context['61'] do
begin
Add('Equal_1',Null,Null,Null);
Add('Equal_2',Null,Null,Null);
end;
with Context['46'] do
begin
Add('Dot_1',Null,Null,Null);
Add('Dot_2',Null,Null,Null);
end;
with Context['58'] do
begin
Add('Colon_1',Null,Null,Null);
Add('Colon_2',Null,Null,Null);
end;
end
|
172
|
How can I allow spaces when control's sentitive context is shown/opened
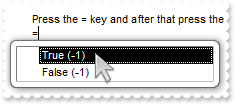
// Change event - Indicates that the control's text have changed.
procedure TForm1.Edit1Change(ASender: TObject; );
begin
with Edit1 do
begin
ShowContext(OleVariant(ChangeOnKey));
ActiveContextItems := '';
end
end;
with Edit1 do
begin
with Context['61'] do
begin
Add('True (-1)','True',Null,Null);
Add('False (-1)','False',Null,Null);
Options[EXEDITLib_TLB.exContextAllowSpaceOnFront] := OleVariant(True);
end;
Text := '';
InsertText('Press the = key and after that press the space keys',Null);
InsertText('',Null);
end
|
171
|
How can I display more pages on the control's senitive context
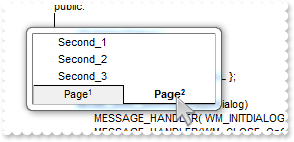
with Edit1 do
begin
with Context[Null] do
begin
Add('First_1',Null,Null,Null);
Add('First_2',Null,Null,Null);
end;
with Context['Second'] do
begin
Add('Second_1',Null,Null,Null);
Add('Second_2',Null,Null,Null);
Add('Second_3',Null,Null,Null);
end;
ActiveContextItems := 'Second';
PagesContextItems := ':Page<font ;6><off -4>1</off></font>,Second:Page<font ;6><off -4>2</off></font>';
end
|
170
|
Is it possible to disable showing tooltip for items in the control's senitive context
with Edit1 do
begin
with Context[Null] do
begin
Add('Column',Null,Null,Null);
Options[EXEDITLib_TLB.exContextItemToolTip] := 'This is bit of text that shown when user selects the <b>Column</b> item.';
Add('Item',Null,Null,Null);
Options[EXEDITLib_TLB.exContextItemToolTip] := 'This is bit of text that shown when user selects the <b>Item</b> item.';
Options[EXEDITLib_TLB.exContextAllowToolTip] := OleVariant(False);
end;
end
|
169
|
How can I assign tooltips for items in the control's senitive context
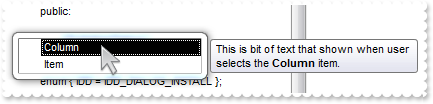
with Edit1 do
begin
with Context[Null] do
begin
Add('Column',Null,Null,Null);
Options[EXEDITLib_TLB.exContextItemToolTip] := 'This is bit of text that shown when user selects the <b>Column</b> item.';
Add('Item',Null,Null,Null);
Options[EXEDITLib_TLB.exContextItemToolTip] := 'This is bit of text that shown when user selects the <b>Item</b> item.';
end;
end
|
168
|
By default, the control shows the Context(""). How can I display other items
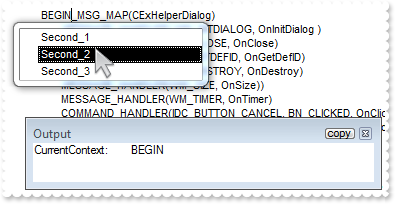
// OnContext event - Occurs when the user invokes the control's context window.
procedure TForm1.Edit1OnContext(ASender: TObject; Start : Integer;Context : WideString);
begin
with Edit1 do
begin
OutputDebugString( 'CurrentContext:' );
OutputDebugString( Context );
ActiveContextItems := 'Second';
end
end;
with Edit1 do
begin
with Context[Null] do
begin
Add('First_1',Null,Null,Null);
Add('First_2',Null,Null,Null);
end;
with Context['Second'] do
begin
Add('Second_1',Null,Null,Null);
Add('Second_2',Null,Null,Null);
Add('Second_3',Null,Null,Null);
end;
end
|
167
|
How can I show the control's sensitive context
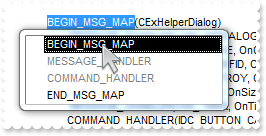
// DblClick event - Occurs when the user double clicks the left mouse button over an object.
procedure TForm1.Edit1DblClick(ASender: TObject; Shift : Smallint;X : Integer;Y : Integer);
begin
with Edit1 do
begin
ShowContext('DB');
end
end;
with Edit1 do
begin
with Context['DB'] do
begin
Add('BEGIN_MSG_MAP',Null,Null,Null);
Add('<fgcolor=808080>MESSAGE_HANDLER',Null,Null,Null);
Add('<fgcolor=808080>COMMAND_HANDLER',Null,Null,Null);
Add('END_MSG_MAP',Null,Null,Null);
end;
end
|
166
|
How can I provide different sensitive context
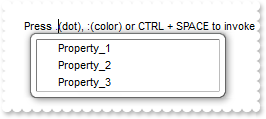
// Change event - Indicates that the control's text have changed.
procedure TForm1.Edit1Change(ASender: TObject; );
begin
with Edit1 do
begin
ShowContext(OleVariant(ChangeOnKey));
end
end;
with Edit1 do
begin
Text := '';
InsertText('Press .(dot), :(colon) or CTRL + SPACE to invoke the control''s context',Null);
with Context[Null] do
begin
Add('General_1',Null,Null,Null);
Add('General_2',Null,Null,Null);
end;
with Context['46'] do
begin
Add('Property_1',Null,Null,Null);
Add('Property_2',Null,Null,Null);
Add('Property_3',Null,Null,Null);
end;
with Context['58'] do
begin
Add('Method_1',Null,Null,Null);
Add('Method_2',Null,Null,Null);
Add('Method_3',Null,Null,Null);
end;
end
|
165
|
How can I change the control's background/foreground colors while the control is locked/read-only
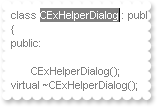
with Edit1 do
begin
Locked := True;
SelBackColor := RGB(128,128,128);
ForeColorLockedLine := RGB(128,128,128);
BackColorLockedLine := RGB(255,255,255);
end
|
164
|
How can change the color for selected text, when the control has no focus
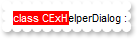
with Edit1 do
begin
HideSelection := False;
SelLength := 10;
SelBackColorHide := RGB(255,0,0);
end
|
163
|
How do I change the "Incremental Search" caption
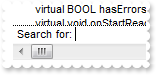
with Edit1 do
begin
Cursor[EXEDITLib_TLB.exIncrementalSearchArea] := 'exHelp';
Caption[EXEDITLib_TLB.exIncrementalSearchField,EXEDITLib_TLB.exCaption] := 'Search for: %s';
IncrementalSearchError := RGB(255,0,0);
end
|
162
|
How do I enable the scrollbar-extension, as thumb to be shown outside of the control's client area
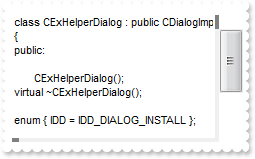
with Edit1 do
begin
ScrollBars := EXEDITLib_TLB.exBoth;
ScrollPartVisible[EXEDITLib_TLB.exVScroll,EXEDITLib_TLB.exExtentThumbPart] := True;
ScrollPartVisible[EXEDITLib_TLB.exHScroll,EXEDITLib_TLB.exExtentThumbPart] := True;
ScrollPartVisible[EXEDITLib_TLB.ScrollBarEnum($2),EXEDITLib_TLB.exExtentThumbPart] := True;
ScrollWidth := 4;
Background[EXEDITLib_TLB.exVSBack] := $f0f0f0;
Background[EXEDITLib_TLB.exVSThumb] := $808080;
ScrollHeight := 4;
Background[EXEDITLib_TLB.exHSBack] := Background[EXEDITLib_TLB.exVSBack];
Background[EXEDITLib_TLB.exHSThumb] := Background[EXEDITLib_TLB.exVSThumb];
Background[EXEDITLib_TLB.exSizeGrip] := Background[EXEDITLib_TLB.exVSBack];
end
|
161
|
How can I get ride of control's horizontal scroll bar
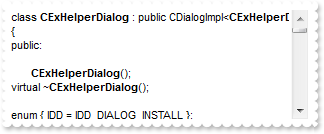
with Edit1 do
begin
AddKeyword('<b>CExHelperDialog</b>',Null,Null,Null);
Refresh();
ScrollBars := EXEDITLib_TLB.exVertical;
end
|
160
|
How do I specify the characters to close the sensitive context
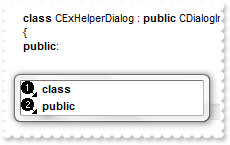
with Edit1 do
begin
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
AddKeyword('<b>public</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
with Context[Null] do
begin
Add('<b>class</b>','',OleVariant(1),Null);
Add('<b>public</b>','',OleVariant(2),Null);
Options[EXEDITLib_TLB.exContextAllowChars] := '_ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz';
end;
end
|
159
|
How do I sort items in the sensitive context
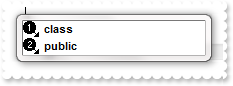
with Edit1 do
begin
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
AddKeyword('<b>public</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
Context[Null].Add('<b>public</b>','',OleVariant(2),Null);
Context[Null].Add('<b>class</b>','',OleVariant(1),Null);
Context[Null].Sort(True);
end
|
158
|
Can I add icons to the sensitive context
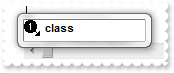
with Edit1 do
begin
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
Context[Null].Add('<b>class</b>','',OleVariant(1),Null);
end
|
157
|
How can I change the keys combination that invokes the sensitive context
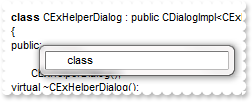
with Edit1 do
begin
ContextKey := 544;
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
Context[Null].Add('class',Null,Null,Null);
end
|
156
|
How do I enable or disable the sensitive context menu
with Edit1 do
begin
CodeCompletion := EXEDITLib_TLB.exCodeCompletionDisable;
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
Context[Null].Add('class',Null,Null,Null);
end
|
155
|
How can I add a sensitive context menu
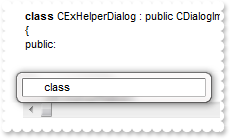
with Edit1 do
begin
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
Context[Null].Add('class',Null,Null,Null);
end
|
154
|
Can I use wild characters to define keys in your control
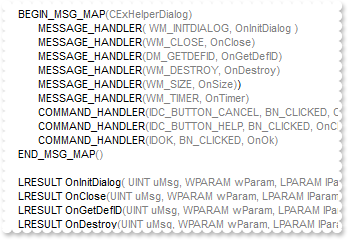
with Edit1 do
begin
AddWild('<fgcolor=808080>(*)</fgcolor>');
Refresh();
end
|
153
|
Can I use wild characters to define keys in your control
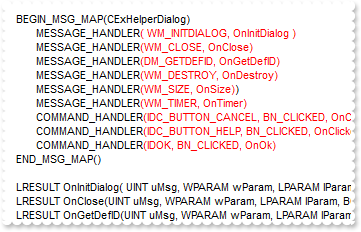
with Edit1 do
begin
AddWild('_HANDLER<fgcolor=FF0000>(*)</fgcolor>');
Refresh();
end
|
152
|
How can I remove or delete all expressions
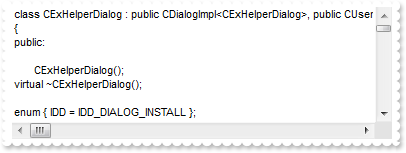
with Edit1 do
begin
AddExpression('(','<b><fgcolor=FF0000> </fgcolor></b>',')',OleVariant(False),Null);
ClearExpressions();
Refresh();
end
|
151
|
How can I remove or delete an expression
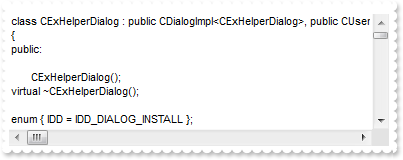
with Edit1 do
begin
AddExpression('(','<b><fgcolor=FF0000> </fgcolor></b>',')',OleVariant(False),Null);
DeleteExpression('(');
Refresh();
end
|
150
|
How can I add an expression
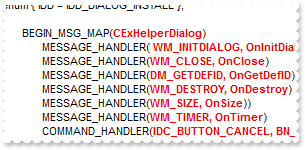
with Edit1 do
begin
AddExpression('(','<b><fgcolor=FF0000> </fgcolor></b>',')',OleVariant(False),Null);
Refresh();
end
|
149
|
How can I add an expression on multiple lines
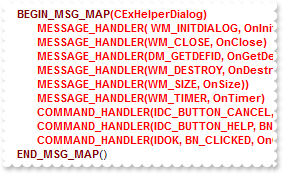
with Edit1 do
begin
AddExpression('<fgcolor=800000><b>BEGIN_MSG_MAP</b></fgcolor>','<b><fgcolor=FF0000> </fgcolor></b>','<fgcolor=800000><b>END_MSG_MAP</b></fgcolor>',OleVariant(True),Null);
Refresh();
end
|
148
|
How can I remove or delete all keywords
with Edit1 do
begin
AddKeyword('<b><fgcolor=FF0000>class</fgcolor></b>',Null,Null,Null);
ClearKeywords();
Refresh();
end
|
147
|
How can I remove or delete keyword
with Edit1 do
begin
AddKeyword('<b><fgcolor=FF0000>class</fgcolor></b>',Null,Null,Null);
DeleteKeyword('class');
Refresh();
end
|
146
|
How do I add a keyword that's not case sensitive
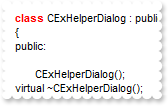
with Edit1 do
begin
AddKeyword('<b><fgcolor=FF0000>class</fgcolor></b>','','',OleVariant(2));
Refresh();
InsertText('ClasS\r\n',OleVariant(1));
InsertText('CLASS\r\n',OleVariant(1));
end
|
145
|
How do I add a keyword that's not case sensitive
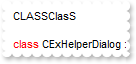
with Edit1 do
begin
AddKeyword('<fgcolor=FF0000>class</fgcolor>','','',OleVariant(1));
Refresh();
InsertText('ClasS\r\n',OleVariant(1));
InsertText('CLASS\r\n',OleVariant(1));
end
|
144
|
How can I assign a tooltip to a keyword
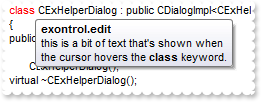
with Edit1 do
begin
AddKeyword('<fgcolor=FF0000>class</fgcolor>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
end
|
143
|
How do I add a keyword

with Edit1 do
begin
AddKeyword('<fgcolor=FF0000>class</fgcolor>',Null,Null,Null);
Refresh();
end
|
142
|
How do I add a keyword
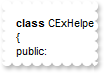
with Edit1 do
begin
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
end
|
141
|
How can I display a tooltip as soon as the user types a keyword
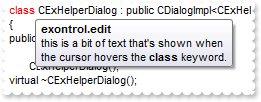
with Edit1 do
begin
ToolTipDelay := 1;
ToolTipOnTyping := True;
AddKeyword('<b>class</b>','this is a bit of text that''s shown when the cursor hovers the <b>class</b> keyword.','exontrol.edit',Null);
Refresh();
end
|
140
|
How do I change the color for a locked or a read only line
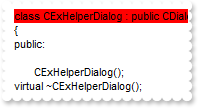
with Edit1 do
begin
ForeColorLockedLine := RGB(0,0,0);
BackColorLockedLine := RGB(255,0,0);
LockedLine[1] := True;
end
|
139
|
How do I lock or make read only a line
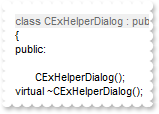
with Edit1 do
begin
LockedLine[1] := True;
end
|
138
|
How do I start overtyping
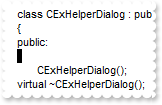
with Edit1 do
begin
Overtype := True;
end
|
137
|
How do I get the selection
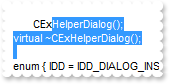
with Edit1 do
begin
GetSelection(sy,sx,ey,ex);
OutputDebugString( sy );
OutputDebugString( sx );
OutputDebugString( ey );
OutputDebugString( ex );
end
|
136
|
How do I select multiple lines
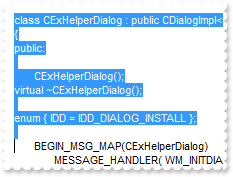
with Edit1 do
begin
SetSelection(OleVariant(0),OleVariant(0),OleVariant(10),OleVariant(0));
HideSelection := False;
end
|
135
|
How can I change the shape of the cursor when it hovers the selected text
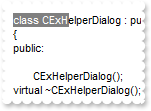
with Edit1 do
begin
Cursor[EXEDITLib_TLB.exSelectedText] := 'exHelp';
SelLength := 10;
HideSelection := False;
end
|
134
|
How can I change the shape of the cursor when it hovers the incremental search area
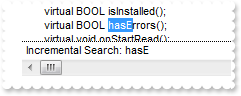
with Edit1 do
begin
Cursor[EXEDITLib_TLB.exIncrementalSearchArea] := 'exHelp';
end
|
133
|
How can I change the shape of the cursor when it hovers the line numbers area
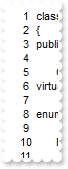
with Edit1 do
begin
Cursor[EXEDITLib_TLB.exLineNumberArea] := 'exHelp';
LineNumberWidth := 16;
end
|
132
|
How can I change the shape of the cursor when it hovers the bookmark area
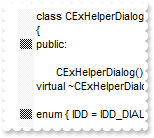
with Edit1 do
begin
Cursor[EXEDITLib_TLB.exBookmarkArea] := 'exHelp';
BookmarkWidth := 16;
end
|
131
|
How can I change the shape of the cursor when it hovers the edit
with Edit1 do
begin
Cursor[EXEDITLib_TLB.exEditArea] := 'exHelp';
end
|
130
|
How can I enable or disable OLE drag and drop operations
with Edit1 do
begin
OLEDropMode := EXEDITLib_TLB.exOLEDropAutomatic;
end
|
129
|
How can I change the descriptions for items in the control's context menu
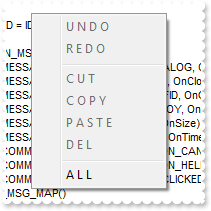
with Edit1 do
begin
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextUndo] := 'U N D O';
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextRedo] := 'R E D O';
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextCut] := 'C U T';
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextCopy] := 'C O P Y';
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextPaste] := 'P A S T E';
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextDelete] := 'D E L';
Caption[EXEDITLib_TLB.exContextMenu,EXEDITLib_TLB.exContextSelectAll] := 'A L L ';
end
|
128
|
How can I change the descriptions for fields in the Replace dialog
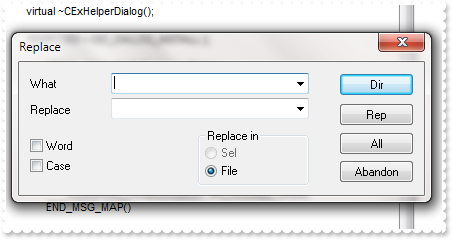
with Edit1 do
begin
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldFindWhat] := 'What';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldReplaceWith] := 'Replace';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldWordOnly] := 'Word';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldMatchCase] := 'Case';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldFindNext] := 'Dir';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldSelection] := 'Sel';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldWholeFile] := 'File';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldReplace] := 'Rep';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldReplaceAll] := 'All';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exFieldCancel] := 'Abandon';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exErrorTitle] := 'Title';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exErrorFindNext] := 'Failed!';
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exErrorFindNext] := 'Done';
end
|
127
|
How can I change the descriptions for fields in the Find dialog
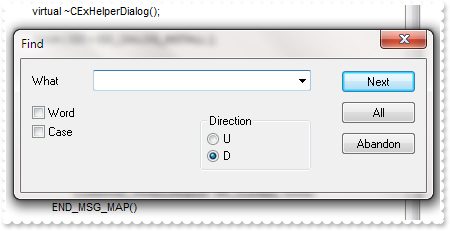
with Edit1 do
begin
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldFindWhat] := 'What';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldWordOnly] := 'Word';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldMatchCase] := 'Case';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldFindNext] := 'Dir';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldSelection] := 'U';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldWholeFile] := 'D';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldFindNext] := 'Next';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldReplace] := 'All';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exFieldCancel] := 'Abandon';
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exErrorFindNext] := 'Failed!';
end
|
126
|
How can I change the caption for the Replace dialog
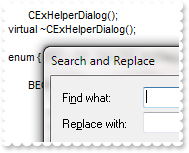
with Edit1 do
begin
Caption[EXEDITLib_TLB.exReplaceDialog,EXEDITLib_TLB.exCaption] := 'Search and Replace';
end
|
125
|
How can I change the caption for the Find dialog
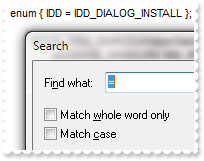
with Edit1 do
begin
Caption[EXEDITLib_TLB.exFindDialog,EXEDITLib_TLB.exCaption] := 'Search';
end
|
124
|
How can I move the cursor when user invokes the control's context menu
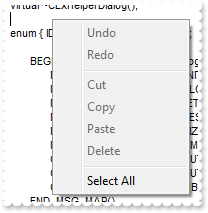
with Edit1 do
begin
RClick := True;
end
|
123
|
How can I disable indenting the selected text when the user presses the TAB key
with Edit1 do
begin
IndentOnTab := False;
end
|
122
|
How can I indent a line
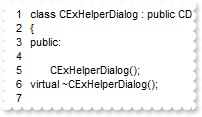
with Edit1 do
begin
LineNumberWidth := 18;
HideSelection := False;
SelectLine(3);
IndentSel(True);
end
|
121
|
How can I show or hide the control's splitter
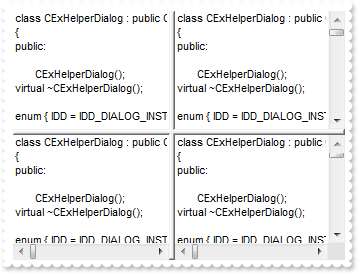
with Edit1 do
begin
AllowSplitter := EXEDITLib_TLB.exBothSplitter;
SplitPaneHeight := 128;
SplitPaneWidth := 128;
end
|
120
|
How can I select a line
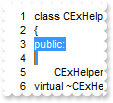
with Edit1 do
begin
LineNumberWidth := 18;
HideSelection := False;
SelectLine(3);
end
|
119
|
How do I change the font to display the line numbers
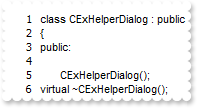
with Edit1 do
begin
LineNumberFont.Name := 'Tahoma';
LineNumberWidth := 18;
end
|
118
|
How can I change the height of the line
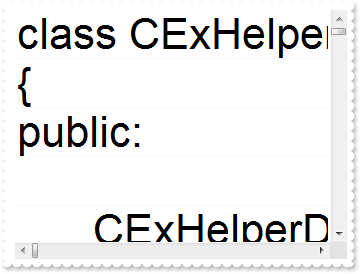
with Edit1 do
begin
Font.Size := 32;
DrawGridLines := True;
Refresh();
end
|
117
|
How can I show or hide the grid lines
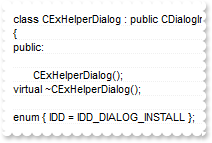
with Edit1 do
begin
DrawGridLines := True;
end
|
116
|
How do I highlight the position of multiple lines expression on the vertical scroll bar
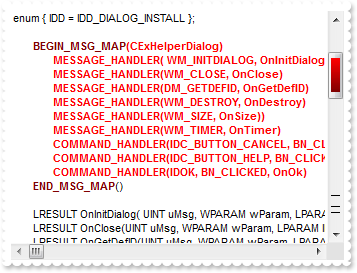
with Edit1 do
begin
AllowMark := True;
MarkContinueBlocks := True;
AddKeyword('<b>CAxWnd',Null,Null,Null);
AddExpression('<fgcolor=800000><b>BEGIN_MSG_MAP</b></fgcolor>','<b><fgcolor=FF0000> </fgcolor></b>','<fgcolor=800000><b>END_MSG_MAP</b></fgcolor>',OleVariant(True),Null);
MarkColor['BEGIN_MSG_MAP'] := $ff;
MarkColor['END_MSG_MAP'] := $80;
MarkColor['CAxWnd'] := $0;
Refresh();
end
|
115
|
How do I ignore \" in a string
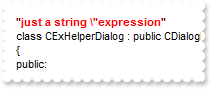
with Edit1 do
begin
InsertText('"just a string \"expression"\r\n',OleVariant(1));
AddExpression('<fgcolor=800000><b>"</b></fgcolor>','<b><fgcolor=FF0000> </fgcolor></b>','<fgcolor=800000><b>"</b></fgcolor>',OleVariant(True),Null);
IgnorePrefixInExpression['"'] := '\';
Refresh();
end
|
114
|
How can I change the color for the line number's border
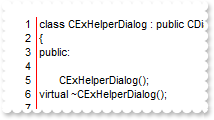
with Edit1 do
begin
LineNumberBorderColor := RGB(255,0,0);
LineNumberWidth := 18;
end
|
113
|
How can I change the color for the bookmark's border
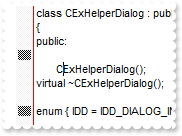
with Edit1 do
begin
BookmarkBorderColor := RGB(255,0,0);
BookmarkWidth := 18;
end
|
112
|
Can I display a custom icon or picture for bookmarks
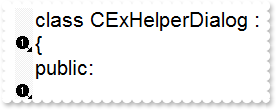
with Edit1 do
begin
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
BookmarkImage := 1;
Bookmark[2] := True;
Bookmark[4] := True;
BookmarkWidth := 18;
end
|
111
|
Can I display a custom icon or picture in the bookmark area
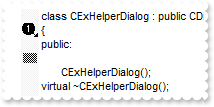
with Edit1 do
begin
Images('gBJJgBAIDAAGAAEAAQhYAf8Pf4hh0QihCJo2AEZjQAjEZFEaIEaEEaAIAkcbk0olUrlktl0vmExmUzmk1m03nE5nU7nk9n0/oFBoVDolFo1HpFJpVLplNp1PqFRqVTql' +
'Vq1XrFZrVbrldr1fsFhsVjslls1ntFptVrtltt1vuFxuVzul1u13vF5vV7vl9v1/wGBwWDwmFw2HxGJxWLxmNx0xiFdyOTh8Tf9ZymXx+QytcyNgz8r0OblWjyWds+m0' +
'ka1Vf1ta1+r1mos2xrG2xeZ0+a0W0qOx3GO4NV3WeyvD2XJ5XL5nN51aiw+lfSj0gkUkAEllHanHI5j/cHg8EZf7w8vl8j4f/qfEZeB09/vjLAB30+kZQAP/P5/H6/yN' +
'AOAEAwCjMBwFAEDwJBMDwLBYAP2/8Hv8/gAGAD8LQs9w/nhDY/oygIA=');
BookmarkImageLine[2] := 1;
Bookmark[4] := True;
BookmarkWidth := 18;
end
|
110
|
How do I remove the line's background color
with Edit1 do
begin
BackColorLine[1] := $ff;
ClearBackColorLine(1);
end
|
109
|
How do I change the foreground color for a line
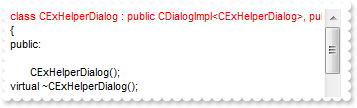
with Edit1 do
begin
ForeColorLine[1] := $ff;
end
|
108
|
How do I change the background color for a line
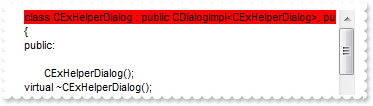
with Edit1 do
begin
BackColorLine[1] := $ff;
end
|
107
|
How can I add my own items in the control's context menu
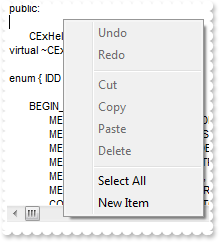
with Edit1 do
begin
ContextMenuItems := 'New Item';
end
|
106
|
How do I ensure that a specified line is visible
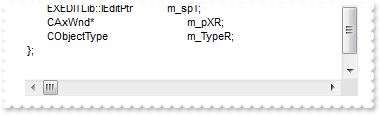
with Edit1 do
begin
EnsureVisibleLine(Count);
end
|
105
|
How can I programmatically perform a REDO operation
with Edit1 do
begin
Redo();
end
|
104
|
How can I programmatically perform an UNDO operation
with Edit1 do
begin
Undo();
end
|
103
|
How do I get the bookmarks as a list
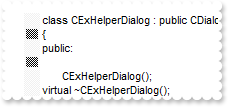
with Edit1 do
begin
Bookmark[2] := True;
Bookmark[4] := True;
BookmarkWidth := 16;
var_BookmarksList := BookmarksList;
end
|
102
|
How can I move to the previous bookmark
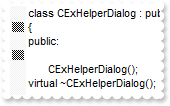
with Edit1 do
begin
Bookmark[2] := True;
Bookmark[4] := True;
BookmarkWidth := 16;
PrevBookmark();
end
|
101
|
How can I move to the next bookmark
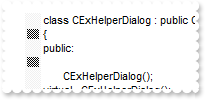
with Edit1 do
begin
Bookmark[2] := True;
Bookmark[4] := True;
BookmarkWidth := 16;
NextBookmark();
end
|